asdru
JCHJava Community | Help. Code. Learn.
•Created by asdru on 10/24/2024 in #java-help
Optimizing draw function for texured triangle
https://github.com/asdru22/3D-Graphics/blob/rasterization/src/main/java/geom/Triangle.java
My current implementation basically implements a rasterization algorithm from scratch, which isn't very efficent. But i don't know how i would otherwise make a triangles texture rotate with it
20 replies
JCHJava Community | Help. Code. Learn.
•Created by asdru on 9/19/2024 in #java-help
scanner blocking thread close
in a project im working on i have a client with a sender and receiver thread. When the server send the quit command im also sending the clients the "quit" message which should intterupt their sender and receiver threads. The receiver ends just fine but the sender (that has the scanner) is stuck waiting for the next line. What's the appropriate way to handle this? i tried closing the senders' scanner in the receiver among other things but nothing worked
24 replies
JCHJava Community | Help. Code. Learn.
•Created by asdru on 9/16/2024 in #java-help
keeping track of clients connected to server
i have a server class that implements the runnable method, and i want to keep track of the clients connected to it so i can disconnect all of them via server class method.
I've seen that a common practise is to use clientHandlers that are Runnable so that each client can run concurrently with the others, but i still dont get how to store the connected client object to the server (if its even possible)
5 replies
JCHJava Community | Help. Code. Learn.
•Created by asdru on 8/9/2024 in #java-help
convert n-tree to dictionary based on depth
I wrote this function to convert a tree into a dictionary where the depth is mapped to the nodes on that depth (i.e. 0->root)
is there a better way to do this?
5 replies
JCHJava Community | Help. Code. Learn.
•Created by asdru on 8/7/2024 in #java-help
isometric tile setup
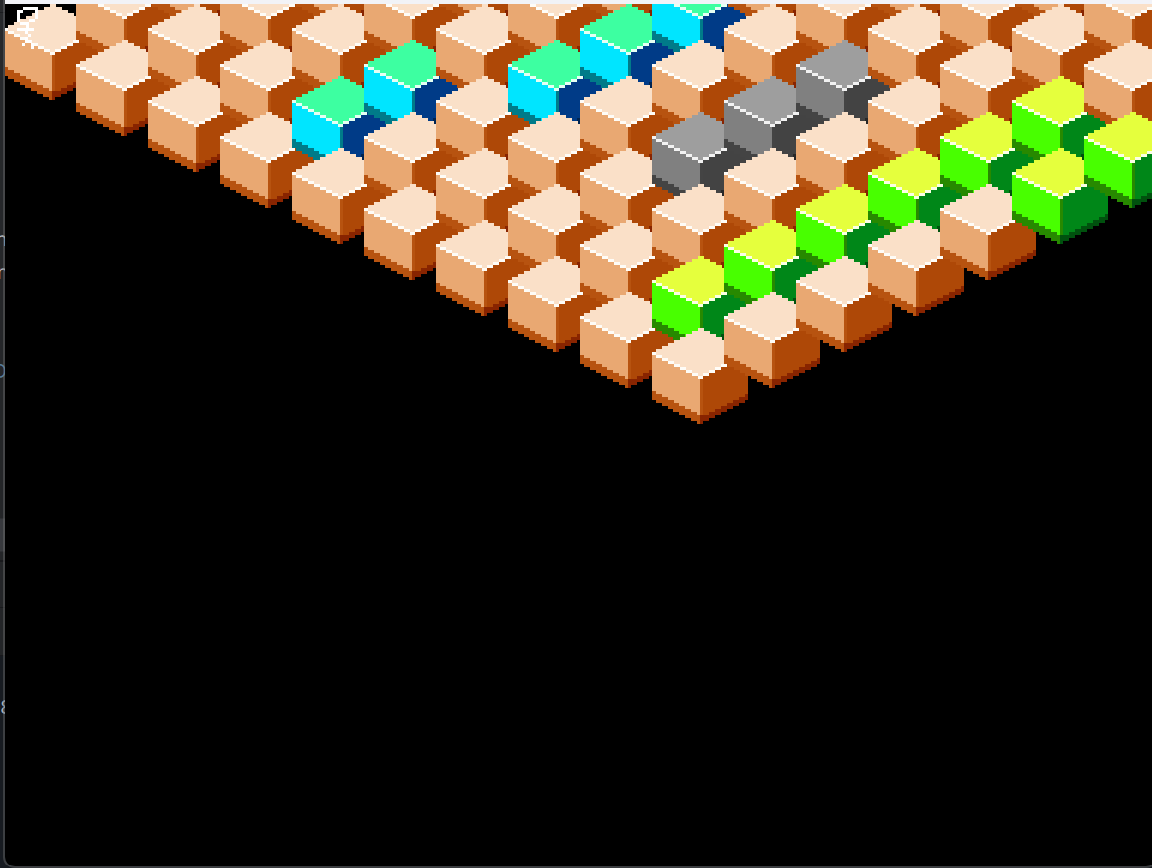
26 replies
JCHJava Community | Help. Code. Learn.
•Created by asdru on 5/4/2024 in #java-help
Filling array with same object reference
get characters returns this
return new PlayableEntity(gamePanel, "player",new Stats(100,20));
i thought this would make the playable entities inside of the array different intances of the same object, but for some reason all the changes i make the one playable entities in the array are applied to all other playable entities29 replies
JCHJava Community | Help. Code. Learn.
•Created by asdru on 5/3/2024 in #java-help
Build jar from maven project on github
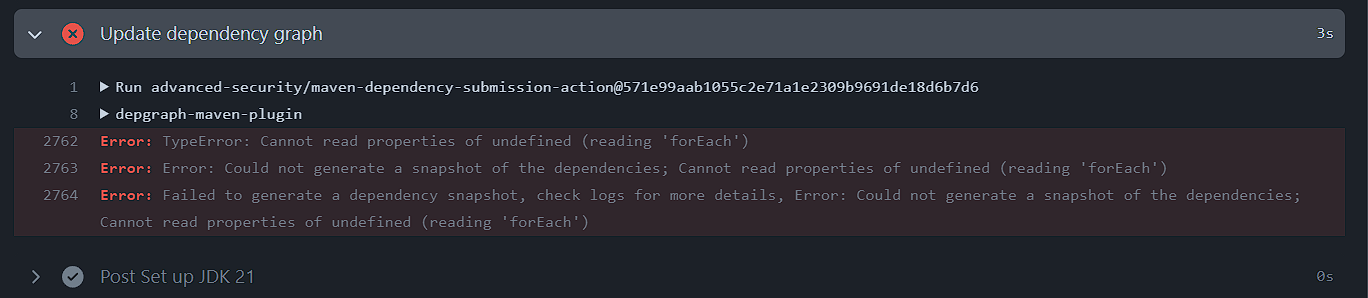
35 replies
JCHJava Community | Help. Code. Learn.
•Created by asdru on 3/19/2024 in #java-help
cant build javafx project
Java FX Packager: Can't build artifact - fx:deploy is not available in this JDK
this is the error i get but i dont understand what fx:deploy is26 replies
JCHJava Community | Help. Code. Learn.
•Created by asdru on 3/15/2024 in #java-help
Gson cannot be resolved to a type error randomly started appearing
i have this method that loads a json file into a class, which i dont exactly know it started throwing an error at this line
Gson gson = new Gson();
despite me not touching that function or the Util function. The wierd part is that if i rewrite that function in a runnable class like
it works. so i can say its not an issue with imports or my util function6 replies
JCHJava Community | Help. Code. Learn.
•Created by asdru on 2/29/2024 in #java-help
modules randomly stopped working on intelliJ

8 replies
JCHJava Community | Help. Code. Learn.
•Created by asdru on 2/16/2024 in #java-help
return string that gets put in the text in a scene builder text label
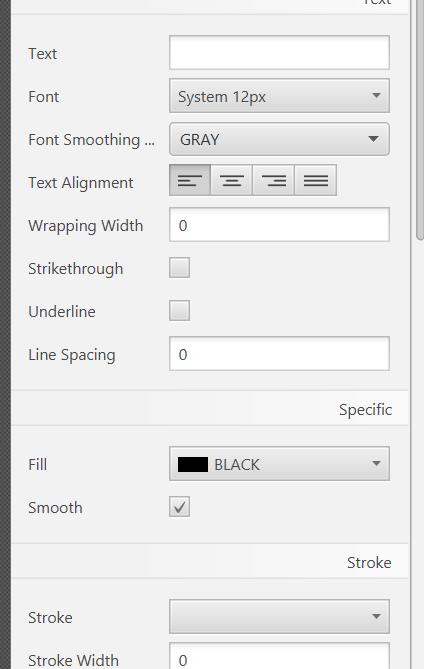
4 replies
JCHJava Community | Help. Code. Learn.
•Created by asdru on 2/7/2024 in #java-help
javafx fxml file not found
i get an error saying that
getClass().getResource("LoginAdminView.fxml")
at line 22 is null
i dont understand why since i have the same setup in the main class and that works. the only difference is that this class is in a subfolder and the main isnt. But i can't figure out what's wrong28 replies
JCHJava Community | Help. Code. Learn.
•Created by asdru on 5/22/2023 in #java-help
java.lang.ClassNotFoundException
I started getting this error regardless of what file i compiled, after a bit of research i managed to narrow down the issue to something wrong with my classpath in sistem variables, but hadn't had any luck fixing it
19 replies