Waffen
❔ Deep clone variable
How to create a complete, isolated copy of a variable to prevent the copy from changing when changed parent variable.
I have tried some variants:
1. The most easiest variant
2. Variant with 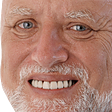
ICloneable
*logic still not changed
if (parent == clonedParent) <-- True
Still True
3. A little bit harder variant with ICloneable
if (parent == clonedParent) <-- True
Still True
Do you know any other variants to clone and isolate 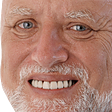
86 replies
❔ Problem with Dictionary<ulong, Image> (Strange work of memory access)
Concept of my system: A system that draws a picture with stickers, each of the stickers can be moved, there can be an unlimited number of stickers.
Code with explanations in comments:
Problem:
Each time you use this function, a new picture should appear with ALL stickers and the sticker that we moved to a new location. However, it happens that the sticker is duplicated and there are several of them.
The suggestion line
image.Mutate(x => x.DrawImage(stickerImage, new Point(sticker.PositionX, sticker.PositionY), 1f));
updates the Dictionary directly, though it shouldn't be, presumably because C# has a memory bug here.
I am using .NET 7.013 replies