❔ Deep clone variable
How to create a complete, isolated copy of a variable to prevent the copy from changing when changed parent variable.
I have tried some variants:
1. The most easiest variant
2. Variant with 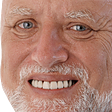
ICloneable
*logic still not changed
if (parent == clonedParent) <-- True
Still True
3. A little bit harder variant with ICloneable
if (parent == clonedParent) <-- True
Still True
Do you know any other variants to clone and isolate 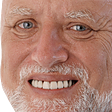
67 Replies
you could use structs which are copied on assignment, or use records that you can copy using
with {}
or use records that you can copy using with {}
Do you mean this variant?
no, i mean an actual
record
the
Clone()
version definitely doesn't return trueEro#1111
REPL Result: Success
Console Output
Compile: 747.068ms | Execution: 94.422ms | React with ❌ to remove this embed.
It is just example
My code is too big, i can't send it here
well then it's a bad example, no?
your "example" works perfectly fine
you're
new
ing up the Person
. that cannot be equal to the old referenceLet me try
the first "variant" doesn't actually do what's being asked, it's not making a copy
¯\_(ツ)_/¯
but this is also flawed
because in this instance,
r1 == r2
will still be true
just beause of a different reasoni interpreted his goal as wanting to make a copy, not just test equality
the way it's being tested leads me to believe there's more to this
true, then you'll get a deep copy too
Hm, thanks, let me try
record
before. But serialize then deserialize
method sounds very good.what are you actually trying to accomplish?
I need to make a copy of the UserProfile then make some changes to it then I need to compare oldProfile and newProfile and get this difference
Ok, it doesn't work. It made copy, but it still changes two variables
but you made the changes to it, surely therefore you know what differences there are?
idk what you mean by "it changes two variables"
it creates a new record with the same values as the first one, it doesn't change anything
Due to some I can't get these changes directly
that isn't copying anything, classes are by reference
let me try this method firstly
what, no
try anything else first
this does not mean they are the same object
if you want to compare that, use
.ReferenceEquals
not ==
changed example for you:
this means they have the identical contents
Ero#1111
REPL Result: Failure
Exception: CompilationErrorException
Compile: 612.249ms | Execution: 0.000ms | React with ❌ to remove this embed.
even so, how does that indicate it "changed two variables"
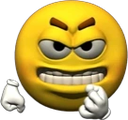
Ero#1111
REPL Result: Success
Result: ValueTuple<R, R, bool, bool>
Compile: 572.211ms | Execution: 117.820ms | React with ❌ to remove this embed.
it's just saying the two objects are equal according to the
==
operator which can be overridden (and is overridden by records to compare them by value)
instead of testing something else and making assumptions about other behavior, test the actual behavior you're trying to achievethe
with
thing will generally only do shallow copies, so probably not whats wanted eitherbased on the model posted earlier a shallow copy is sufficient
ask insufficient questions, get insufficient answers
¯\_(ツ)_/¯
I needed just a method how to do deep copy
there's no one correct answer
serialize then deserialize the best method for me i guess.
i guess
performance wise, its the worst u can do
Yes, but is there any other variants?
do it all recursively i guess
I'm not sure if this method is right for my problem
well, you want a deep copy
the easiest and most efficient way is to use the 2nd variant, after all u have to take care of references inside ur instances
What "2nd variant" are you talking about?
the
ICloneable
implementation and actually manually creating the copy inside the Clone
methodI've tried this variant already, it stil True
i quite literally proved that that is not possible
that's not possible
Okey
did u make copies of nested of mutable references as well?
==
tests reference equality for classes unless overridden
you're returning a new object, so it cannot be the same referencewell, unless u show exactly what u tried we cant tell what u did wrong, after all u implemented it urself
here u have only a shallow copy, because
box1.Items
and box2.Items
would reference the same List<object>
instance.
u would have to make a copy of the list as well.
and then u need to think about if need to make a copy of each element in the list as well,
and then again if take care about their referencesThanks a lot! I did not know how to correctly formulate my problem, what to correctly google the solution.
Deep clone through Serialize and deserialize helps me and takes
0.0758792
seconds. For my project, this delay is not a problem.i'm not sure how you benchmarked it but i'm very skeptical it took 70ms to serialize and deserialize a 2 field class
you should use something like https://benchmarkdotnet.org/
different hardware would give different results, also the (de-)serialization methods
not 70ms different
that sounds way too high
wouldnt alone reflection based and source generation based make quite a huge difference?
also the difference is currently 6ms and we dont know the actual structure of the data they tested with
It's just user data, it can't be that much
Unless the project or game is already hugely complex
Isn't it easier at all to just get the difference before adjusting an object? Or just newing up an object with replacements in the way of
that's essentially what the record suggestion was
Ah I missed that one then my bad was a lot of messages
What’s wrong with the 2nd option? Why did they resort to serializing and deserializing?
nobody knows
more to write i guess 😂
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.