sabercoy
Explore posts from serversCDCloudflare Developers
•Created by sabercoy on 10/15/2024 in #pages-help
How to set up auth for static files
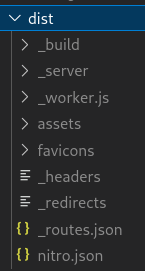
3 replies
Returning Errors and not throwing
I am messing around with actions and useSubmission and I have seen Ryan and the docs mention it is best to return, not throw, Errors
I dont understand the "so that errors can be used declaratively to render the updated page on the server" part
Isnt the use of actions in forms and useSubmission always on the client? How are we rendering and updated page on the server? Dont we look at the submission and change the page on the client accordingly? (granted this requires JS)
8 replies
ErrorBoundary reset() method
what is reset() supposed to do?
if you run this and getData() errors, it will be caught be ErrorBoundary and the fallback will be rendered
if you click the mutateDataAction button until getData() errors again, there will be an uncaught error
if you call the reset() method (onClick handler on the div) it just re-runs the fallback function
the docs say
The reset function will reset the error boundary and re-render the children.
if you call reset() then have getData() error again, it will not be caught
if you call reset() the children are not reran, the fallback is reran
what is the intended usage of reset()? and how can I make it to where multiple subsequent calls to getData() are handled properly if errors occur?1 replies
The combination of API routes and data loading functions
I have been trying out integrating my existing API routes with
cache
and action
deploying to cloudflare.
The goal is to have the benefit of 1) preloading route data 2) having an open API 3) and being able to easily log API accesses.
The examples with SS seem to want you to utilize server functions which would not be an open API and (I dunno) how I would log properly
If I call fetch
to an API route inside cache
with no use server
then it can be called on either client or server
I need cookies/credentials, so calling on client is good because the browser will pass them, but on server there is no credentials and CF errors
I can utilize use server
to make each call a server function that calls an API route, then extract the headers from the event and wire into the fetch
to make it as close as possible as if I called the API route from the browser
But this seems very non-ergonomic.. It seems as though deciding to use cache
and action
means not having a good time with API routes, and instead opting to using only server functions52 replies
Can cache be manually set?
Say I have a
export const getList = cache(...)
and I access the list using createAsync
in multiple places in the app
If a user chooses to delete the entire list in some section of the app and the request succeeds, the expected result for the list is now to be empty
To my understanding with the current model, the way to update the list to be empty would be to revalidate
it, probably in an action
, which would trigger another call to the server to get the new list (which is empty)
but say I want to not go to the server to get the obvious empty-list result. Is there a way I can manually set the list to empty?
I had thought I could use cache.set()
to do this, but upon using it nothing seems to happen (at least reactively)
I know it is frowned upon to want to manually mutate state in the UI and not re-ask the server, but I can think of a few scenarios where this is harmless and beneficial.
basically, I am wondering if there is an equivalent mutate()
function from createResource
for createAsync
14 replies