FredTheNoob
Explore posts from serversTTCTheo's Typesafe Cult
•Created by FredTheNoob on 3/17/2025 in #questions
Uploading files to uploadthing directly using fetch API or similar
I am currently trying to write a migration script that simply moves my local files over to uploadthing. I want to do this in a ts script using bun.
I tried doing it like this:
But that gave me an error.
Following the documentation I tried implementing the https://docs.uploadthing.com/api-reference/client#upload-files example and https://docs.uploadthing.com/api-reference/ut-api#upload-files without luck.
Anyone got an idea of how to do this?
2 replies
BABetter Auth
•Created by FredTheNoob on 2/19/2025 in #help
Forget Password endpoint always returns status code 200
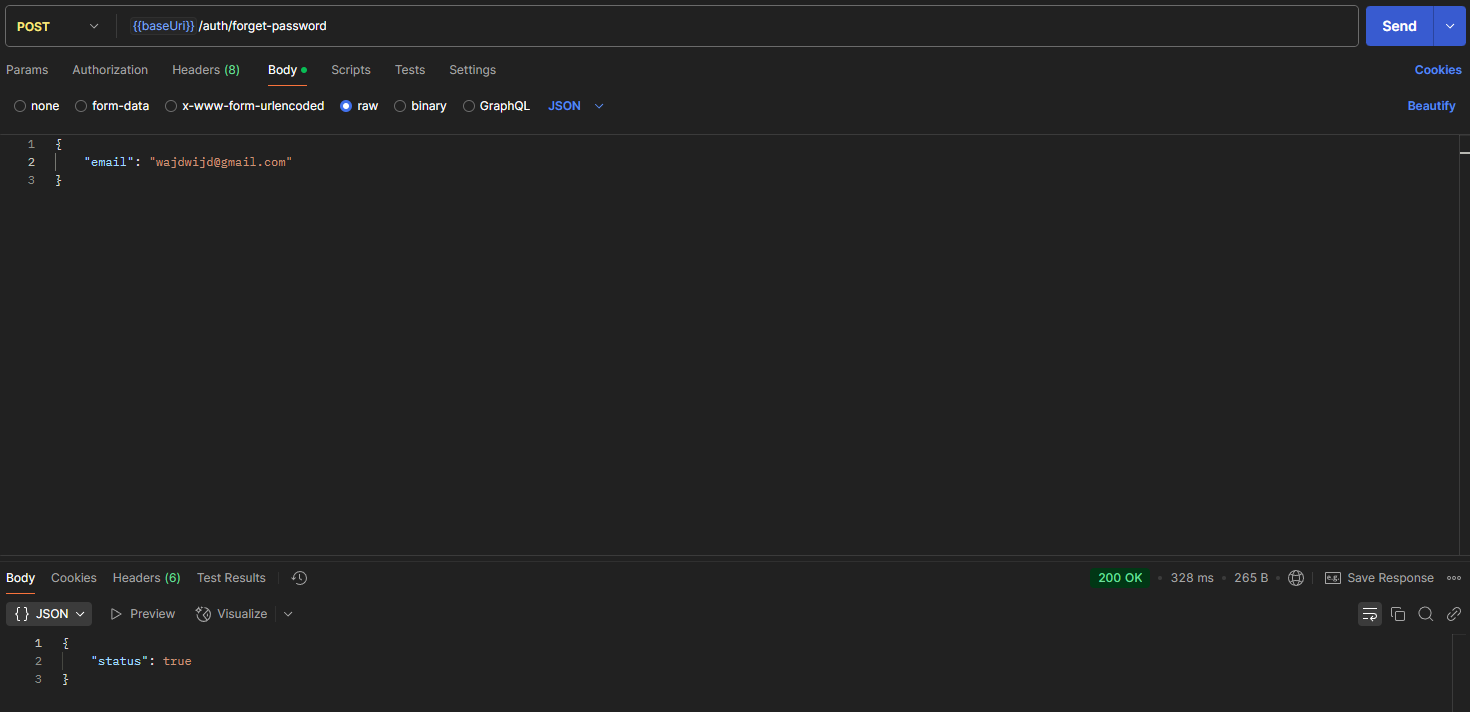
8 replies