Wolfman
Explore posts from serversMmalefashionadvice
•Created by Wolfman on 5/31/2024 in #questions-and-advice
Looking for critiques on groom suit
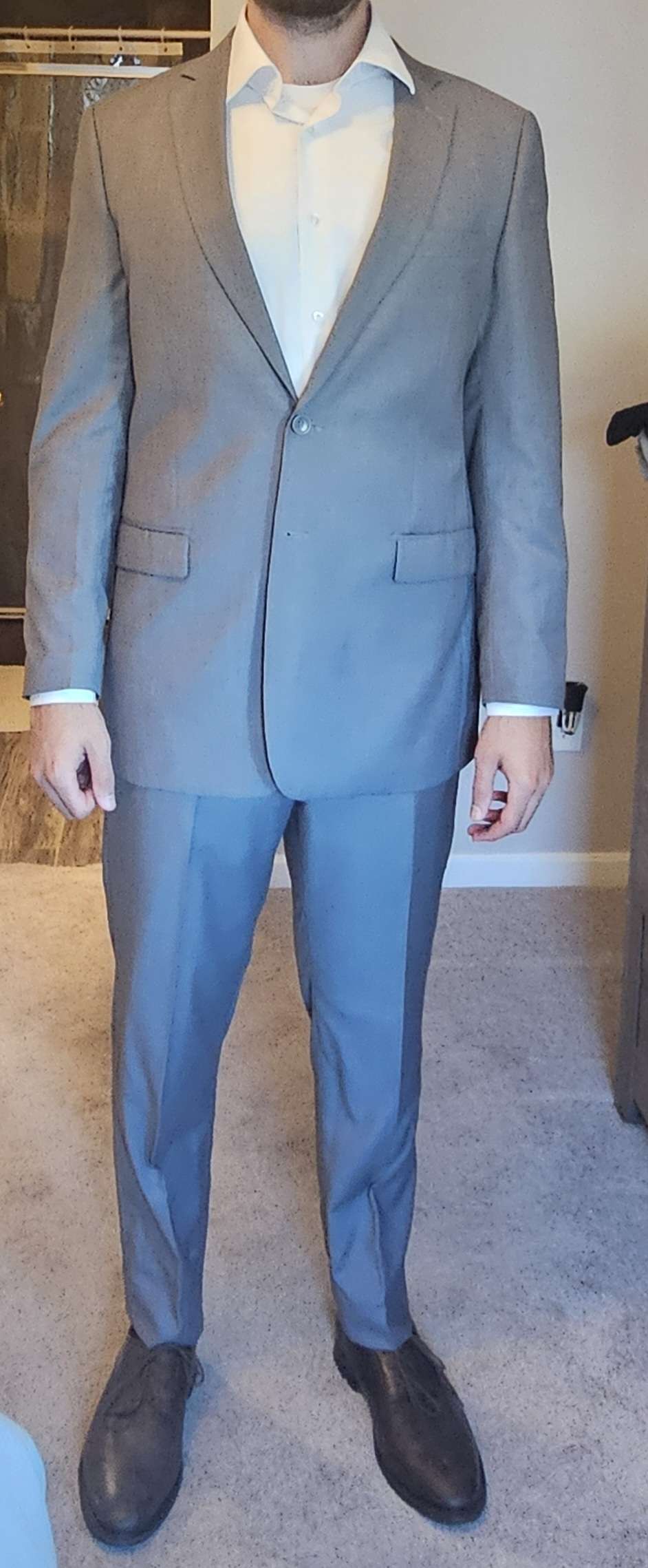
8 replies
Unhandled Exception: System.Runtime.InteropServices.COMException:Unknown error (0x8007203b)
I am currently working on a console app that goes through active directory and exports all the computer object to a csv document. This is what I have so far however I keep getting the error noted above when I hit around 3 million computers. Any help would be great!
26 replies