Perfect
Explore posts from serversTTCTheo's Typesafe Cult
•Created by Perfect on 7/23/2024 in #questions
Difference between SSR with streaming and new PPR
So today in react (often achieved through nextjs) we can use SSR to stream components onto the page and show a fallback while data is being fetched. I’m referring to the main concept in this explanation by Dan. (https://github.com/reactwg/react-18/discussions/37)
Server components are not even a part of this discussion - react can render a page on the server and use suspense to stream in pieces that may take longer. The alternative to this is just waiting for all of the page to be rendered after data is fetched which could result in a delay.
Now in NextJS, we have the idea of static and dynamic rendering. Static rendering happens at build time (or incrementally), and renders HTML that is served instantly from a CDN. No rendering is required at request time if a page is static. Dynamic rendering happens if any part of a page opts into dynamic behavior. So the page gets rendered every time a request comes in, and the user could be left waiting a while. If we go back to suspense, this helps set boundaries that can stream in when they are ready. Static parts of the dynamic page will still need to be rendered, right?
I’m trying to figure out what’s different about this dynamic rendering with suspense boundaries approach, and the new PPR pattern that will be released in the future.
https://nextjs.org/learn/dashboard-app/partial-prerendering
Any explanation or resources would really help as I’ve been confused on this all day.
7 replies
TTCTheo's Typesafe Cult
•Created by Perfect on 6/23/2023 in #questions
Truncating link
I want the behavior of the link you see at the top. For the link to truncate I had to set it to block but this means that it fills its container, so on hover works on spots it shouldnt and the border is not right. Inline-block did not fix because truncation stopped working, any ideas?
33 replies
TTCTheo's Typesafe Cult
•Created by Perfect on 6/6/2023 in #questions
Design Feedback
Im creating a link in bio tool to learn nextjs 13 app dir and just wanted some feedback on the design of a dashboard link so far. Something seems off to me but I am not sure what, maybe the colors of the icons or spacing? (too tight?) Thanks!
- icons might be too big?
- icon color too bold?
- spacing too tight?
- space between links too little?
19 replies
TTCTheo's Typesafe Cult
•Created by Perfect on 5/30/2023 in #questions
do something once server action is completed
Is there a way to achieve this (see title)? I’m using useTransition to start the action and need to close my modal once the action is completed successfully.
162 replies
TTCTheo's Typesafe Cult
•Created by Perfect on 5/23/2023 in #questions
Loading file or suspense?
So I have a basic dashboard page and want to show some loading state for a server component.
Instead of just putting UserLinks unwrapped on its own and using the
loading.tsx
file, I wrapped it in Suspense so that only that part of the component shows loading state (only where its doing async data stuff). Before doing this, the entire page showed whatever is in loading.tsx
so all the static text and stuff that could be shown right away was not.
I could have put the static stuff in the loading file, but it seems repetitive. Is this a better solution? It seems that next knows not to use the loading file if there is a Suspense in use.2 replies
TTCTheo's Typesafe Cult
•Created by Perfect on 5/9/2023 in #questions
SWR - If loading is false and error is undefined, what can we assume?
If
isLoading
is false and error
is undefined, can I assume data
is not undefined? (using typescript)
Is it ok to always have to use data like this data?.something
(since it is possibly undefined).
Is there a more standard way to know for sure when data
is ready to be used on the UI? Should I always do something like data && <Component/>
?
Thanks!1 replies
TTCTheo's Typesafe Cult
•Created by Perfect on 5/3/2023 in #questions
SWR - array of data in one request, one item from array in another
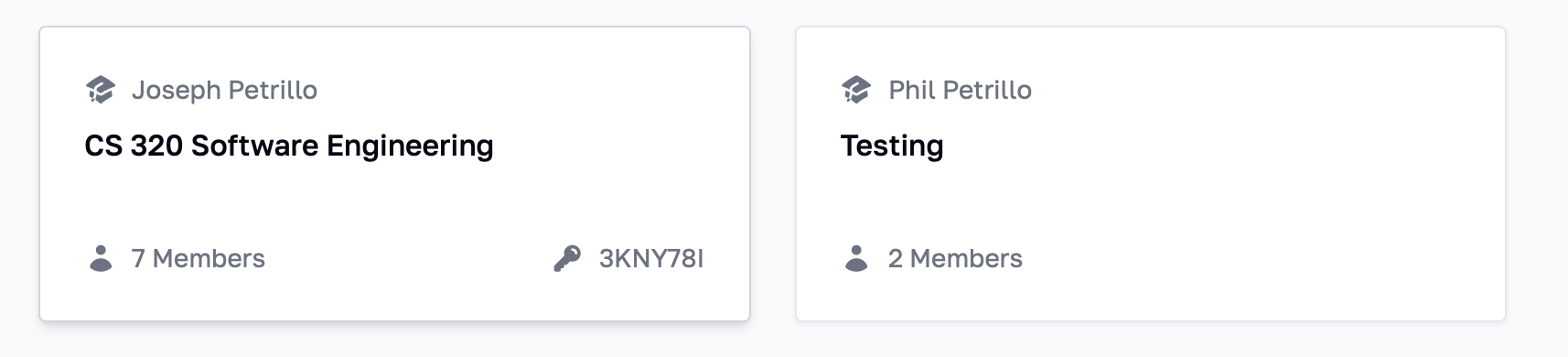
2 replies
TTCTheo's Typesafe Cult
•Created by Perfect on 4/30/2023 in #questions
UI for updating role
Got a quick question. I have a table displaying all the users in a course. Two actions can be performed on a user from the table.
1. They can be kicked from the course
2. They can be promoted to role "instructor" (if they are a student) or demoted to role "student" (if they an instructor).
I have the kick button currently in the ui, but I am not sure where to put the ui for the role changing part. Any ideas? Thanks!
https://cdn.discordapp.com/attachments/1095827961015455754/1102266025724088330/image.png
22 replies
TTCTheo's Typesafe Cult
•Created by Perfect on 4/28/2023 in #questions
Acceptable Layout Shift
Is this acceptable layout shift for a skeleton loader? Not sure how to handle the possibility of the course name going to two lines or not.
5 replies
TTCTheo's Typesafe Cult
•Created by Perfect on 4/27/2023 in #questions
Should a react layout component be responsible for initial required data fetching.
So I am building a dashboard for a course page. There are different routes that the course page can have. For example
/courses/9
/courses/9/settings
/courses/9/members
etc
Each of these pages shares the same header (includes course name, metadata stuff, and the tabs to change pages)
The role the user has in that course is fetched in the data the header uses to get its data.
To avoid repeating the header on every course page, I think making a layout could be a solution. However, I need the data the header uses in each of the pages as well. So, I guess since I’m using SWR I can just use the same api key and get the data instantly without another fetch, or I can figure or some other way. Does this make sense? Any ideas?
1 replies
TTCTheo's Typesafe Cult
•Created by Perfect on 4/27/2023 in #questions
More Elegant Way For Type
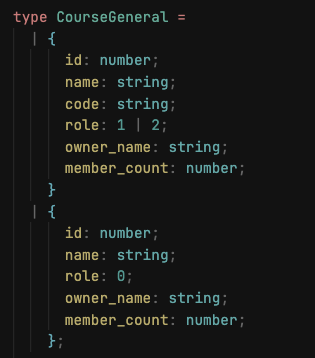
51 replies
TTCTheo's Typesafe Cult
•Created by Perfect on 4/26/2023 in #questions
Proper REST API Nesting
I feel like I am doing too much nesting. Do these routes look semantically correct overall? thanks!
GET
/courses
(get course details of courses you are enrolled in)
GET /courses/:course_id
(get specific course details, should this get everything related to the course?)
POST /courses
(create new course)
POST /courses/enroll
(attempt to join class only using course code)
DELETE /courses/:course_id
(delete entire course)
GET /courses/:course_id/members
(owner gets all members of their course)
PATCH /courses/:course_id/members/:user_id
(change role of user in course)
DELETE /courses/:course_id/members/:user_id
(leave a course)
PATCH /courses/:course_id/meetings/:meeting_id
(updated details of a meeting)
POST /courses/:course_id/meetings
(create a new meeting)
POST /courses/:course_id/meetings/:meeting_id
(join the queue for this meeting)
GET /courses/:course_id/meetings/:meeting_id
(get specific meeting details)18 replies
TTCTheo's Typesafe Cult
•Created by Perfect on 4/20/2023 in #questions
REST API Best Practice
As a general rule of thumb, should you always provide the same data for a resource back, if in one form it is an array, and the other is just that one resourse?
For example, if I have courses.
I could have a
/course
GET route that gets me back all of the courses for the user
I could have a /course/:id
GET route that gets me back a specific course
Should the /course
response contain an array of /course/:id
responses?
Think of a dashboard, where all of the user courses are displayed (/course
route is used). I am unsure if I should only return what the page uses, or everything?
Thanks!18 replies
TTCTheo's Typesafe Cult
•Created by Perfect on 4/19/2023 in #questions
Fastest way to get counts (need to redo schema?)
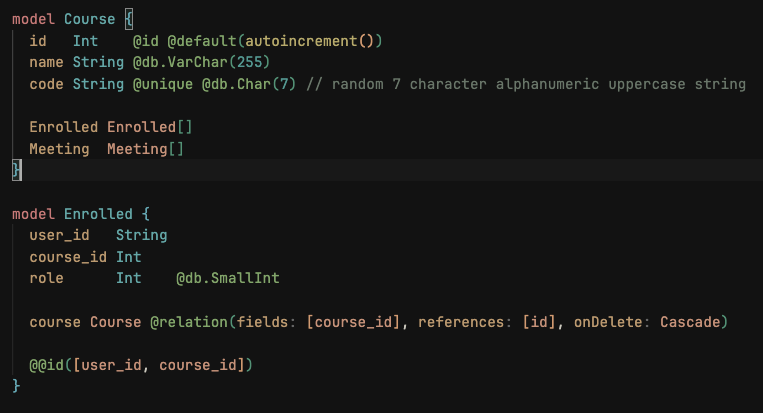
108 replies
TTCTheo's Typesafe Cult
•Created by Perfect on 4/12/2023 in #questions
Extending Error SWR
Does anyone know how to extend error type to make something like this example from SWR work?
https://swr.vercel.app/docs/error-handling#status-code-and-error-object
Very lost on how to do this after looking online for awhile. thx!
2 replies
TTCTheo's Typesafe Cult
•Created by Perfect on 4/4/2023 in #questions
Zod - instanceof z.ZodError
For some reason, the error is not being recognized as an instanceof Zod and I have no idea why. Anything wrong I am not seeing?
5 replies
TTCTheo's Typesafe Cult
•Created by Perfect on 3/25/2023 in #questions
Does my DB schema look correct?
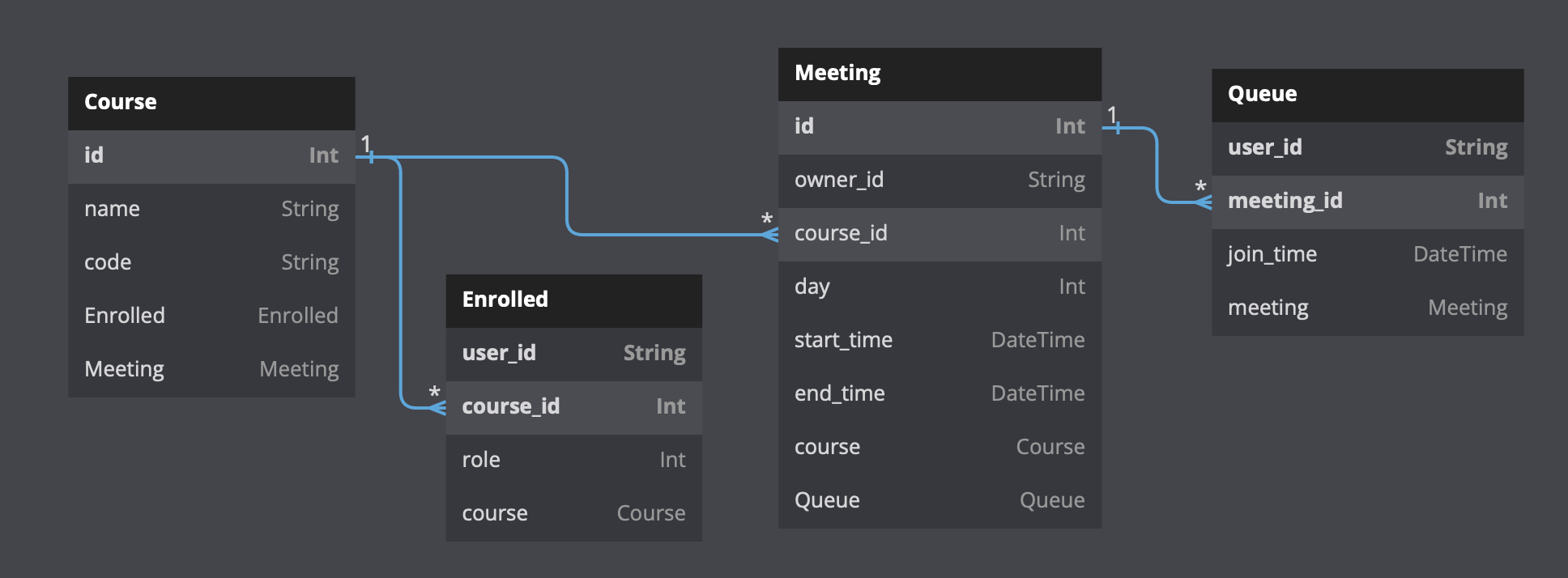
13 replies
TTCTheo's Typesafe Cult
•Created by Perfect on 3/17/2023 in #questions
identical urls page vs api
Noob question -
If my site has a page at /posts, but my api also has a /posts route. Is it possible to differentiate? Should I prefix all my api routes like /api/posts for example?
15 replies
TTCTheo's Typesafe Cult
•Created by Perfect on 2/5/2023 in #questions
Next Image Behavior
Hi, I am just curious if this looks like normal nextjs image behavior (the popping in of the images)?
https://rust-meta.vercel.app/raiding/calculator
Thanks!!
3 replies