Martacus
Explore posts from serversDTDrizzle Team
•Created by Martacus on 11/12/2023 in #help
Having trouble writing my sql statement in drizzle. Want to join two variables
Perfect
11 replies
DTDrizzle Team
•Created by Martacus on 11/12/2023 in #help
Having trouble writing my sql statement in drizzle. Want to join two variables
Now to get them as names
11 replies
DTDrizzle Team
•Created by Martacus on 11/12/2023 in #help
Having trouble writing my sql statement in drizzle. Want to join two variables
That works 😄
11 replies
DTDrizzle Team
•Created by Martacus on 11/12/2023 in #help
Having trouble writing my sql statement in drizzle. Want to join two variables
Oh I see my mistake
11 replies
DTDrizzle Team
•Created by Martacus on 11/12/2023 in #help
Having trouble writing my sql statement in drizzle. Want to join two variables
Using the given query I still get 2 results, two times the same relation but with a different entity attached.
I've tried doing:
But that still gives me
Alias "entity" is already used in this query
11 replies
DTDrizzle Team
•Created by Martacus on 11/12/2023 in #help
Having trouble writing my sql statement in drizzle. Want to join two variables
Oh wait, is it because I am not adding aliases?
11 replies
DTDrizzle Team
•Created by Martacus on 11/12/2023 in #help
Having trouble writing my sql statement in drizzle. Want to join two variables
I've tried:
But i get a
Alias "entity" is already used in this query
11 replies
DTDrizzle Team
•Created by Martacus on 11/12/2023 in #help
Having trouble writing my sql statement in drizzle. Want to join two variables
Yeah ofcourse:
11 replies
DTDrizzle Team
•Created by Martacus on 11/5/2023 in #help
Unable to read error message when inserting withdb.insert().values()
tyty
8 replies
DTDrizzle Team
•Created by Martacus on 11/5/2023 in #help
Unable to read error message when inserting withdb.insert().values()
In the schema I added
.defaultNow()
to them. Will updatedAt be updated on every edit to the row?
8 replies
DTDrizzle Team
•Created by Martacus on 11/5/2023 in #help
Unable to read error message when inserting withdb.insert().values()
Okay so my bad, I had wrong types for the createdAt and updatedAt. The error is very complicated and my eyes just couldnt really focus.
8 replies
DTDrizzle Team
•Created by Martacus on 11/5/2023 in #help
Unable to read error message when inserting withdb.insert().values()
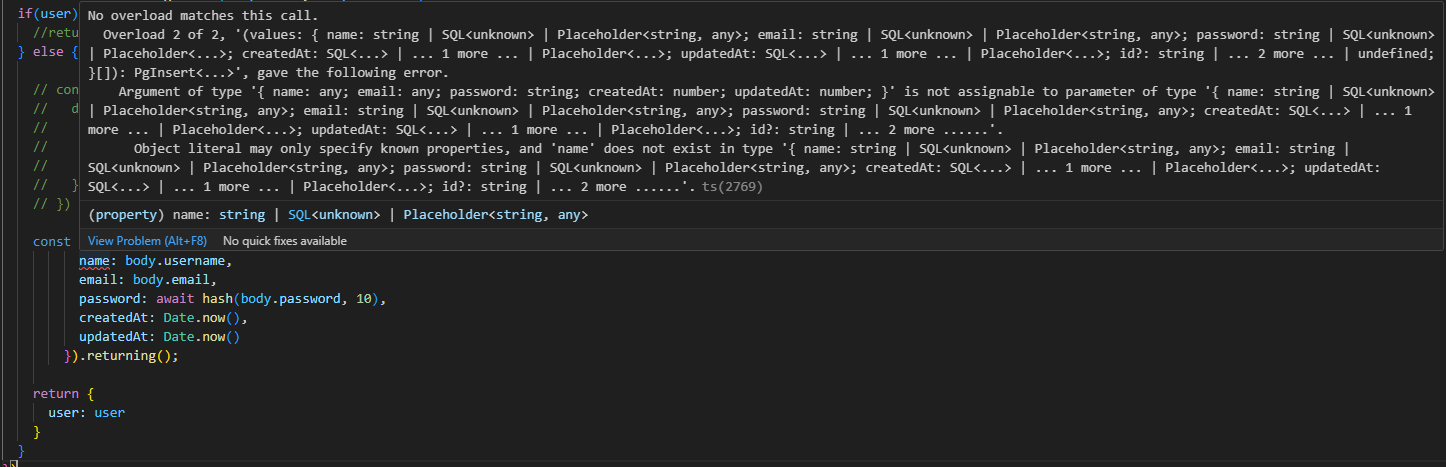
8 replies