snxxwyy
KPCKevin Powell - Community
•Created by snxxwyy on 12/10/2024 in #front-end
:has() alternatives
Hey, are there any polyfills or alternate methods for
:has()
for browsers that don’t support it? Thanks in advance20 replies
KPCKevin Powell - Community
•Created by snxxwyy on 12/10/2024 in #front-end
How does the cascade affect media/container queries?
Hey, if I’m catering for browsers that don’t support
@container
, I may do something like so-
Since the media query will run despite the support for @container
, if the browser supports containers, do they both run and the container query styles take over the media query ones due to it being lower in the cascade? Or does the media query styles activate as well and if so should you wrap it in an @supports not
block?
Thanks in advance4 replies
KPCKevin Powell - Community
•Created by snxxwyy on 12/10/2024 in #front-end
optimization | @font-face with <link>
Hey, i know pre-loading fonts or links of any sort of importance aids in optimization. If i have a local font that i have a
@font-face
declaration for, can i also include something like <link rel="preload" href="fonts/fontName.woff2" as="font" type="font/woff2/>
alongside it or am i unable to have both?
Thanks in advance.15 replies
KPCKevin Powell - Community
•Created by snxxwyy on 12/9/2024 in #front-end
Class overwriting styles it shouldn’t?
Hey, I’m trying to style a button with two classes with the
[class|=“”]
selector, if I add with-icon
and button
at the same time it overwrites all of the styles for the button class. This would totally make sense if both classes had the same properties in which case the cascade would handle which values are used, but it overwrites things in button
that with-icon
doesn’t have, such as background-color
. I want it to overwrite the display property only in this case, which I thought it would do.
It doesn’t do this when I use regular class selectors e.g .button
so it must be selector related, but this selector says ‘if the element has a class with that value or one that starts with that value”, this doesn’t sound like they should clash in any other way than expected?
https://codepen.io/deerCabin/pen/EaYyaqr
I’d appreciate any insight. Am i missing something? Thanks in advance.15 replies
KPCKevin Powell - Community
•Created by snxxwyy on 12/8/2024 in #front-end
display contents use cases
Hey, what are some use cases for
display: contents;
? To me it seems very similar to subgrid but since it comes with the reduced styling consequence, i don't really see any positives for it?
I'd appreciate any help, thanks in advance.19 replies
KPCKevin Powell - Community
•Created by snxxwyy on 12/6/2024 in #front-end
Drop down menu & position absolute sizing
Hey, I’ve never really looked too in depth into dropdown menus and decided it’s time haha, what’s an efficient way to “dock” them so they don’t sit off screen when the screen gets too small like in this example codepen?
I saw the recent video Kevin made on it however due to the lack of browser support in things like
anchor-positioning
, I was wondering about more general approaches.
Following that, I’ve never really noticed that position absolute makes elements min-content
or if not something similar, what is it that shrinks a position absolute element and how do you effectively expand them back to an intrinsic size without setting a width/min width which presents overflow issues in some cases?
https://codepen.io/deerCabin/pen/ogvbVKJ
Thanks in advance6 replies
KPCKevin Powell - Community
•Created by snxxwyy on 12/5/2024 in #front-end
revert vs unset & svg focusable attribute
Hey, i have a couple questions regarding the topics in the title-
1) mdn says the difference between the
revert
and unset
values is that revert
changes a set of styles back to the user agent styles whilst unset
changes a set of styles back to the inherited value and if not then the user agent styles. I don't understand logically the need for unset
since the default behavior would be to inherit a value if possible anyways right? unless there's more to it?
2) I've seen people add focusable="false"
to svgs to stop them being added into the tab order or being caught in a screen reader, i don't quite understand this since i don't believe svgs are focusable by default anyways?
I'd appreciate getting some help clearing those up, thanks in advance.6 replies
KPCKevin Powell - Community
•Created by snxxwyy on 12/4/2024 in #front-end
svg path creating space
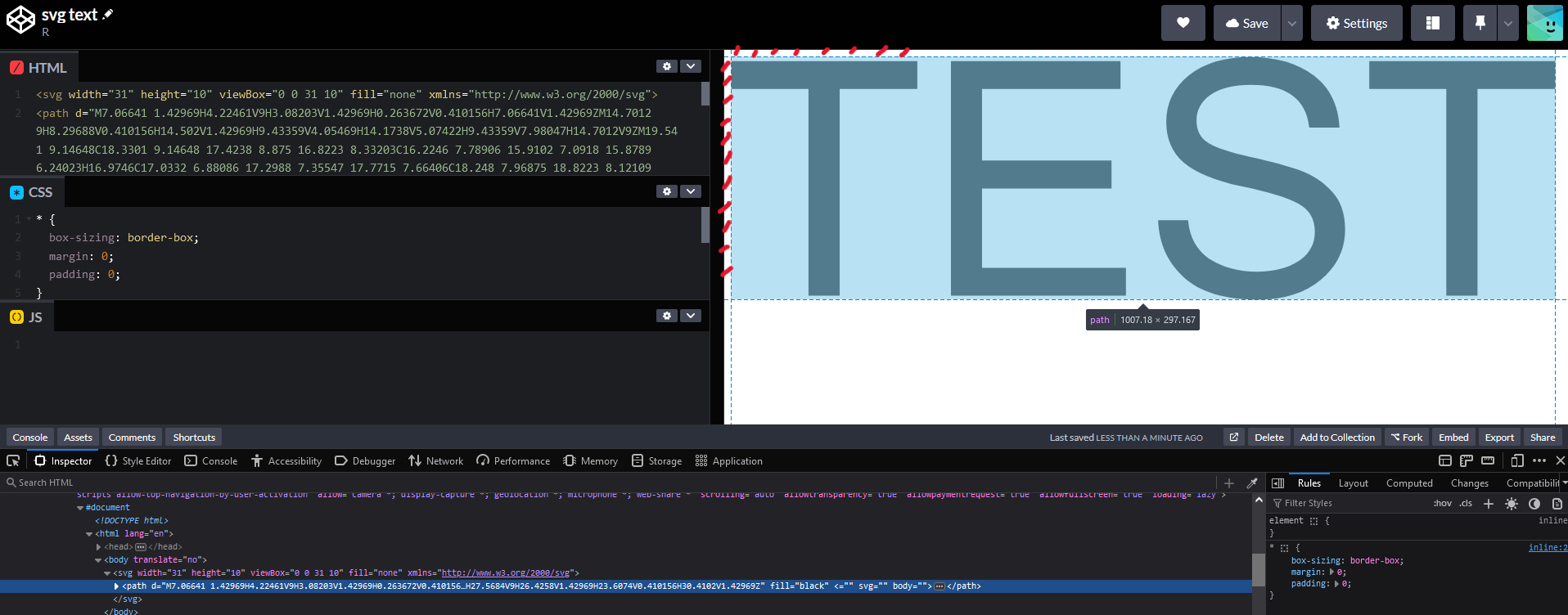
15 replies
KPCKevin Powell - Community
•Created by snxxwyy on 11/27/2024 in #front-end
How to correctly import fonts
Hey, if I have some
@font-face
declarations inside of a css file and import the css file in the html, I understand that this shouldn’t cause a problem because the assets wouldn’t load sequentially since they’re being imported through the html, thus not blocking the page render if it takes too long, but should I be importing fonts using a different method? Perhaps a link
tag if that exists for this? If so, how do i still get the benefits of @font-face
?
Thanks in advance8 replies
KPCKevin Powell - Community
•Created by snxxwyy on 11/27/2024 in #front-end
dialog questions
Hey, I have a couple questions regarding the
dialogue
element-
1) mdn recommends to avoid toggling the open
attribute to enable the dialogue and to instead use .show()
or .showModal()
in js. Why is this?
2) I’ve seen that .show()
makes the dialogue “non modal” and .showModal()
makes the dialogue “modal”. What’s the key differences?
3) mdn also says it’s recommended to use the autofocus
attribute on important content or the close button in the dialogue. I haven’t seen this attribute until now, is it a dialogue only thing or can you use it with other things?
I’d appreciate any help, thanks in advance.12 replies
KPCKevin Powell - Community
•Created by snxxwyy on 11/27/2024 in #front-end
Overlay content method
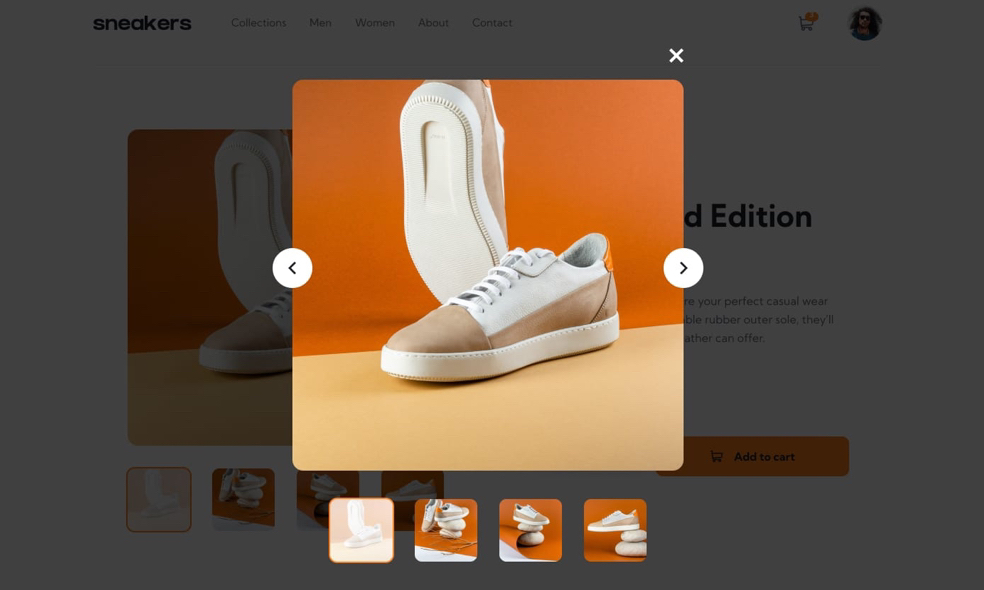
25 replies
KPCKevin Powell - Community
•Created by snxxwyy on 11/24/2024 in #front-end
nodes in js
Hey, i hear "nodes" floating around when people talk about certain things in js. But what exactly are they and how do they differ from elements? There are some methods that are for nodes and some that aren't e.g.
parentElement
and parentNode
and it seems pretty confusing since they at first sight seem to be doing the same thing?
I'd appreciate any info, thanks in advance.31 replies
KPCKevin Powell - Community
•Created by snxxwyy on 11/23/2024 in #front-end
dataset vs regular data methods.
Hey, what's the difference between using
dataset
and regular data methods such as setAttribute
? I know there's more to dataset
but i can't figure it out.
The only thing i see with dataset
is you can list all attribute names and values and have them put in an object but can't you also get attributes with .attributes
and .getAttribute
?
And for setting them rather than doing element.dataset.xyz = "xyz"
can't you use setAttribute
?
I'd appreciate any info. Thanks in advance.9 replies
KPCKevin Powell - Community
•Created by snxxwyy on 11/22/2024 in #front-end
title attribute | tooltips
Hey, I’m aware that if you add the
title
attribute to an element it adds a tooltip with that text on hover. What is the reason you may want to add this?
Thanks in advance31 replies
KPCKevin Powell - Community
•Created by snxxwyy on 11/21/2024 in #front-end
solving overlapping stacking contexts.
Hey, In this example i have two divs that are
containers
. they both each create a new stacking context. The red box overlaps the text, however if i'd like the text to overlap the red box, i can't do that unless i add something like position: relative
and z-index
to the text. It makes sense to do so but since the text element doesn't use position: relative
i'm adding it for the sake of overlapping content which doesn't feel right? I assumed the z-index: -99
on the red box would have solved it but since they're their own individual stacking contexts it has no effect.
https://codepen.io/deerCabin/pen/OJKexQj
Is there a more appropriate way to solve this sort of thing? Thanks in advance.5 replies
KPCKevin Powell - Community
•Created by snxxwyy on 11/15/2024 in #front-end
dl vs ul/ol
Hey, could somebody please explain the difference between a description list and general list and common use cases for a dl? Is the only difference that dl should be used for list items with a description?
Thanks in advance.
19 replies
KPCKevin Powell - Community
•Created by snxxwyy on 11/15/2024 in #front-end
inset behaviour
Hey, I have a
position: fixed;
header and a .wrapper
class for this example. I wanted to center the header on the page with the inset
property (top, bottom, left, right works too). However when i use inset: 1em auto 0 auto;
which i assume to have the top, bottom, left and right order, it doesn't work, instead i have to do inset: 1em 0 auto 0
which doesn't make sense to me. the 1em
is to space it from the top of the page but since i don't want any space at the bottom i would put 0
instead of auto
but that stretches it for some reason which also confuses me.
That only works if the .wrapper
class is directly applied to the header. If it's wrapped in it my flex
on header doesn't work and the content seems to get really long if you hover over it in dev tools.
I'd appreciate any help explaining this. Thanks in advance.
https://codepen.io/deerCabin/pen/BaXbqpP85 replies
KPCKevin Powell - Community
•Created by snxxwyy on 11/13/2024 in #front-end
@media | print, screen & only
Hey, i have a couple questions for some
@media
rules-
1) What type of things/content on your site would warrant changing in regards to the print
rule? essentially, what would you want to change when printing out a page of your site?
2) i've heard the screen
rule represents exactly what it says, being a screen of a certain size. That makes something like @media (min-width: 500px)
different since it refers to a window size right? but aren't they technically the same?
3) What does the only
rule really do? i've heard it hides media queries from older browsers but why is that? is it for support reasons?
Thanks in advance.60 replies
KPCKevin Powell - Community
•Created by snxxwyy on 11/12/2024 in #front-end
forms | action and method
Hey, i have a couple of questions about the
action
and method
attributes for forms-
1) With action
, what necessarily does sending data to other html/pfp files etc do? I can imagine it's so you can use it with js that's hooked up to the page it's sending to to either use it in a query or display it on the page. This makes sense to me since i imagine you'd have to use a database or something similar to retrieve data between pages without this functionality. let me know if i'm wrong though please.
2) With the GET
method, what's the function of appending the data to the url? What does it allow you to do?
3) When would you use GET
vs POST
? I assume POST
when sending the form data somewhere with action
. With GET
, the answer depends on the answer to question 2) but it would confuse me if you had a GET
method with a link in action
as where is the data going at that point if you're getting data from somewhere else?
I'd appreciate any help clearing this up, thank you in advance.31 replies
KPCKevin Powell - Community
•Created by snxxwyy on 11/11/2024 in #front-end
quick css questions
Hey, i just a few quick questions
1) is there a way to select an element based off of whether it's a certain count of one on the page? I know
nth-child
and nth-of-type
do this, but only if the elements are siblings. If i wanted to select the 3rd p element on the whole page for example, would that be possible? maybe it's with that of
selector that's floating around?
2) I've noticed that if you have a list inside of another list, the child's ul/ol
element will have it's user agent margin removed, what causes this? is it because of the way sub-lists work? https://codepen.io/deerCabin/pen/wvVRoVG
Thanks in advance.18 replies