nullopt
DIAdiscord.js - Imagine an app
•Created by nullopt on 5/6/2024 in #djs-questions
Best way to get StringSelectMenuInteraction value from ButtonInteraction?
I have a StringSelect and a Button as my components.
I want the user to select a value from the StringSelect, and then press the button to confirm.
This is my current setup:
14 replies
DIAdiscord.js - Imagine an app
•Created by nullopt on 3/31/2023 in #djs-questions
Trying to start a scheduled event
I'm running into an error when trying to start a scheduled event:
Error:
Creation Code:
Starting Code:
I am manually able to start the event from the UI, but not from code?
12 replies
DIAdiscord.js - Imagine an app
•Created by nullopt on 3/25/2023 in #djs-questions
Is there a way to auto subscribe a set of users to a GuildScheduledEvent?
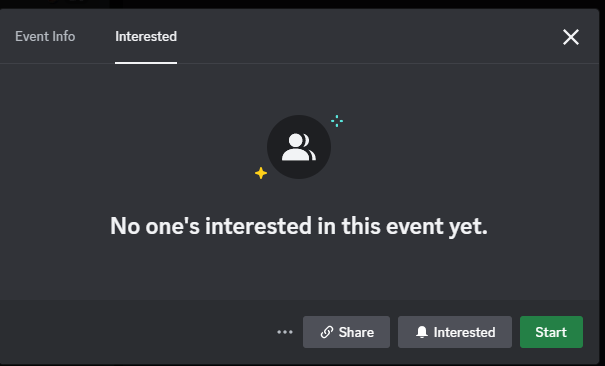
3 replies
DIAdiscord.js - Imagine an app
•Created by nullopt on 12/30/2022 in #djs-questions
guildMemberUpdate only firing on 2nd event
So I have subscribed to the
guildMemberUpdate
event, but it is not being called the first time I assign a role to someone. Only when I remove that role is it firing. From then after it works perfectly.
8 replies
DIAdiscord.js - Imagine an app
•Created by nullopt on 12/30/2022 in #djs-questions
Client.on(guildMemberUpdate) - Check who added the role
Is there a way to check if a user or a bot added a role to a user?
I want staff to be unable to self-assign certain roles, and only allow them to be assigned via the bot
4 replies
DIAdiscord.js - Imagine an app
•Created by nullopt on 12/12/2022 in #djs-questions
deferUpdate while waiting for api response
After the initial CommandInteraction, I .deferReply while I wait for an api response, then I .editReply once it's complete.
I then have a ButtonBuilder attached that allows the data to refresh. So I .deferUpdate while the data is refreshing, then I .update once complete.
My issue is that it fails on the .deferUpdate as .deferReply has already been called.
Is there a way to resolve this?
(The API is external and sometimes takes more than 3 seconds to resolve, so the interaction times out if the .deferUpdate is not called)
5 replies
DIAdiscord.js - Imagine an app
•Created by nullopt on 12/11/2022 in #djs-questions
Update original Interaction reply from ButtonInteraction
How can I edit the original interaction reply from within a ButtonInteraction handler?
29 replies
DIAdiscord.js - Imagine an app
•Created by nullopt on 11/15/2022 in #djs-questions
MessageComponentCollector within a MessageComponentCollector
I have an embed with a SelectMenuBuilder attached.
If an invalid selection is made, I want to reply to the interactor (user) with an ephemeral message that contains a ButtonBuilder to join a waitlist.
6 replies
DIAdiscord.js - Imagine an app
•Created by nullopt on 11/14/2022 in #djs-questions
Message.attachments.size or Message.embeds.length always returning 0
Hi all, currently trying to check if a message contains an image. Using the
messageCreate
hook.13 replies