Key-value Field with dot in key
Maybe I am blind/stupid, but why isn't it possible to save a key value in a
KeyValue
field with a dot in the name? I have just this simple field:
and of course I cast it in the model to array:
So keys like "Test" or "Test 2asdas" or "Test 3!" are working and I can save them without errors.
But as soon as there is a dot inside like "Test." or "Test Value. XY" then I get: "No synthesizer found for key: "1""
Context: I use this field to create a mapping of values to IDs. For example, I want to map certain brand names like "Apple" or "Google" to IDs. However, my source dataset also contains brands with a "." in their name, such as "O.B.":
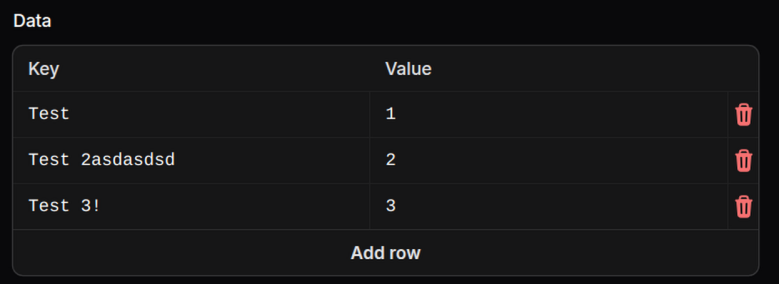
31 Replies
I think these are used you see
@toeknee Sorry, I don't understand what you mean?
The dots are likely interfering with the statePath which also uses dots to denote the components in the form. It’s an internal mapping.
As ;er awcodes and depending if we are using dataget // Arr:get that treats . as a nested array.
I'd recommend stripping out . and replacing with
Well, I was already thinking into this direction, but
beforeStateDehydrated
isn't working
I tried
Why not just add it in the model as the mutator?
Well this is just a simplified example. In my real usecase, I am using the keyvalue field inside a repeater
and I get an error when saving when using beforeStateDehydrated. So the problem occurse before
So use ->formatStateUsing(fn($state, $record) => )
Thats the worng position in lifecycle.
But on the model you could use:
Which would return data with the .'s replaced.
Maybe we are speaking of different problems. The user enters the name like "O.B." into the KeyValue Field. So formatStateUsing or mutators are too late / too early. The error occurs on saving
Ok so do it on the set
That is assuming the values save to the DB ok which I suspect they should
This is again too late. The error isn't from the model, but from the field.
But my first try on beforeStateDehydrated is wrong. I thought I get the string in beforeStateDehydrated, but I get already the wrong data:
If you want to do it on the key value field:
mutateDehydratedStateUsing()
I did jsut test the saving though through a KEyvalue field and it tried to save to the db as expected
set
test
= { "test.test": "testvalue" },
At which point you could mutate it on the setDataAttribute on the saving to the DB.Well thats funny.
This is without any extra code, just
KeyValue::make('data')
So when creating a new record, setting the value to test.test
it works and is saved without problems. Then editing and adding something without a dot, it works as well.
Then creating a new record without a dot, saving and adding the same test.test
again, I get the error
Thats how its saved
So yes that's how it's saved... but did you add the attribute above? Which will adjust it from . to _ on saving?
to your model?
Where should I add this? To the model?
Yes
It is a Standard laravel Attribute caster

Thats now the whole model:
Are you on laravel 11
this is the latest fork of the demo app
laravel/framework v11.31.0 The Laravel Framework.
But once again, I dont think this is a problem on the model part of laravel, but on the filament part
Works. without any setDataAttribute
That will, just doing it on the model is easier for future proofing
This is it working
Not sure if modifing data just to make it work for a specific form isn't they way to go imho. Because on alll other places, the Dot is just fine. In the import Command, on the output, the API's where I have to use the data. So just the form is the problem, not the data, the storage or the formating
But anyway, thanks for your help and time. Maybe I will sort it out otherwise
Well then you need to just make sure the dots are put back in on save, and replaced on get
Yeah. The idea is to fix this with some kind of "Using" method. But none of them are triggered early enought. I get the error before
beforeStateDehydrated
You will, because that's filling it we use php to get the values likely, so you would need to use the getStateUsing method because the state is what is filled I beleive.
Method Filament\Forms\Components\KeyValue::getStateUsing does not exist.
None is triggered before the error:
KeyValue::make('data')
->mutateDehydratedStateUsing(fn ($state) => dd($state))
->afterStateUpdated(fn ($state) => dd($state))
->beforeStateDehydrated(fn ($state) => dd($state))
->dehydrateStateUsing(fn ($state) => dd($state))
even
->afterStateUpdated(fn ($state) => dd($state))
->live(onBlur: true)
throws the error, when the field loses the focus. So as you can see, it has nothing to do with the save / model
but only on edit, not on create. weird bug