Precondition doesn't return an error message
import { ApplyOptions } from '@sapphire/decorators';
import { Precondition } from '@sapphire/framework';
import type { PreconditionOptions, PreconditionResult } from '@sapphire/framework';
import type { CommandInteraction, ContextMenuCommandInteraction, GuildMember } from 'discord.js';
import { container } from '@sapphire/framework';
import { Result } from '@sapphire/framework';
@ApplyOptions<PreconditionOptions>({
name: 'TicketModerate',
})
export class TicketModeratePrecondition extends Precondition {
public override chatInputRun(interaction: CommandInteraction): PreconditionResult {
return this.checkModeratePermission(interaction);
}
public override contextMenuRun(interaction: ContextMenuCommandInteraction): PreconditionResult {
return this.checkModeratePermission(interaction);
}
private checkModeratePermission(interaction: CommandInteraction | ContextMenuCommandInteraction): PreconditionResult {
const member = interaction.member as GuildMember;
if (member.permissions.has('Administrator') || interaction.guild?.ownerId === interaction.user.id) {
return this.ok();
}
const { prisma } = container;
return Result.fromAsync(
prisma.ticket.findFirst({
where: {
channelId: interaction.channelId,
status: {
not: 'CLOSED'
}
},
include: {
category: {
include: {
moderatorRoles: true
}
}
}
}).then(ticket => {
if (!ticket) {
return this.error({ message: '❌ Cette commande ne peut être utilisée que dans un ticket actif.' });
}
const hasRole = ticket.category.moderatorRoles.some(
role => member.roles.cache.has(role.roleId) && ['MANAGE', 'READ_WRITE'].includes(role.permission)
);
if (!hasRole) {
console.log('❌ Vous n\'avez pas la permission d\'utiliser cette commande.');
return this.error({ message: "❌ Vous n'avez pas la permission d'utiliser cette commande." });
}
return this.ok();
})
);
}
}
declare module '@sapphire/framework' {
interface Preconditions {
TicketModerate: never;
}
}
import { ApplyOptions } from '@sapphire/decorators';
import { Precondition } from '@sapphire/framework';
import type { PreconditionOptions, PreconditionResult } from '@sapphire/framework';
import type { CommandInteraction, ContextMenuCommandInteraction, GuildMember } from 'discord.js';
import { container } from '@sapphire/framework';
import { Result } from '@sapphire/framework';
@ApplyOptions<PreconditionOptions>({
name: 'TicketModerate',
})
export class TicketModeratePrecondition extends Precondition {
public override chatInputRun(interaction: CommandInteraction): PreconditionResult {
return this.checkModeratePermission(interaction);
}
public override contextMenuRun(interaction: ContextMenuCommandInteraction): PreconditionResult {
return this.checkModeratePermission(interaction);
}
private checkModeratePermission(interaction: CommandInteraction | ContextMenuCommandInteraction): PreconditionResult {
const member = interaction.member as GuildMember;
if (member.permissions.has('Administrator') || interaction.guild?.ownerId === interaction.user.id) {
return this.ok();
}
const { prisma } = container;
return Result.fromAsync(
prisma.ticket.findFirst({
where: {
channelId: interaction.channelId,
status: {
not: 'CLOSED'
}
},
include: {
category: {
include: {
moderatorRoles: true
}
}
}
}).then(ticket => {
if (!ticket) {
return this.error({ message: '❌ Cette commande ne peut être utilisée que dans un ticket actif.' });
}
const hasRole = ticket.category.moderatorRoles.some(
role => member.roles.cache.has(role.roleId) && ['MANAGE', 'READ_WRITE'].includes(role.permission)
);
if (!hasRole) {
console.log('❌ Vous n\'avez pas la permission d\'utiliser cette commande.');
return this.error({ message: "❌ Vous n'avez pas la permission d'utiliser cette commande." });
}
return this.ok();
})
);
}
}
declare module '@sapphire/framework' {
interface Preconditions {
TicketModerate: never;
}
}
12 Replies
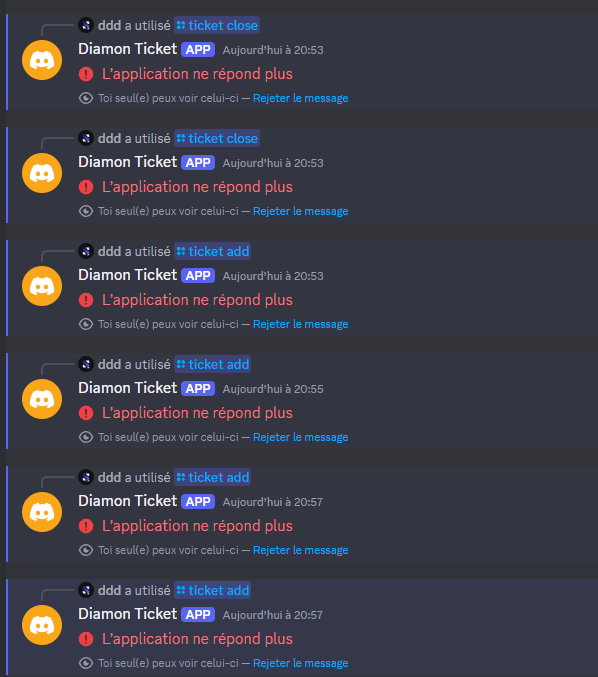
preconditions do not automatically send messages, in fact, sapphire as a whole never does. You have to implement this yourself with
Yeah but I already have that
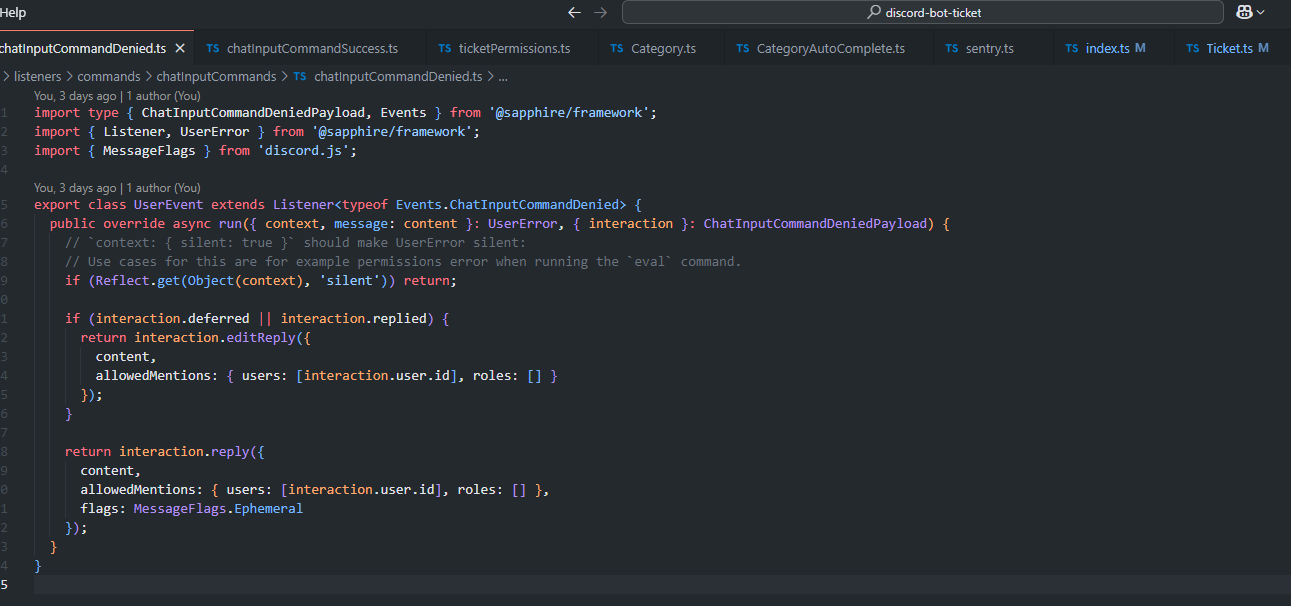
My precodition are working for the config command for example

import { ApplyOptions } from '@sapphire/decorators';
import { Precondition } from '@sapphire/framework';
import type { PreconditionOptions, PreconditionResult } from '@sapphire/framework';
import type { CommandInteraction, ContextMenuCommandInteraction, GuildMember, Message } from 'discord.js';
import { checkAdministrator } from '../lib/util/functions/permissions';
@ApplyOptions<PreconditionOptions>({
name: 'AdminOnly',
})
export class AdminOnlyPrecondition extends Precondition {
public override chatInputRun(interaction: CommandInteraction): PreconditionResult {
return this.isGuildAdmin(interaction.member as GuildMember);
}
public override contextMenuRun(interaction: ContextMenuCommandInteraction): PreconditionResult {
return this.isGuildAdmin(interaction.member as GuildMember);
}
public override messageRun(message: Message): PreconditionResult {
return this.isGuildAdmin(message.member as GuildMember);
}
private isGuildAdmin(member: GuildMember): PreconditionResult {
return checkAdministrator(member)
? this.ok()
: this.error({ message: "Bien essayé, mais tu n'as pas les permissions nécessaires pour effectuer cette commande." });
}
}
declare module '@sapphire/framework' {
interface Preconditions {
AdminOnly: never;
}
}
import { ApplyOptions } from '@sapphire/decorators';
import { Precondition } from '@sapphire/framework';
import type { PreconditionOptions, PreconditionResult } from '@sapphire/framework';
import type { CommandInteraction, ContextMenuCommandInteraction, GuildMember, Message } from 'discord.js';
import { checkAdministrator } from '../lib/util/functions/permissions';
@ApplyOptions<PreconditionOptions>({
name: 'AdminOnly',
})
export class AdminOnlyPrecondition extends Precondition {
public override chatInputRun(interaction: CommandInteraction): PreconditionResult {
return this.isGuildAdmin(interaction.member as GuildMember);
}
public override contextMenuRun(interaction: ContextMenuCommandInteraction): PreconditionResult {
return this.isGuildAdmin(interaction.member as GuildMember);
}
public override messageRun(message: Message): PreconditionResult {
return this.isGuildAdmin(message.member as GuildMember);
}
private isGuildAdmin(member: GuildMember): PreconditionResult {
return checkAdministrator(member)
? this.ok()
: this.error({ message: "Bien essayé, mais tu n'as pas les permissions nécessaires pour effectuer cette commande." });
}
}
declare module '@sapphire/framework' {
interface Preconditions {
AdminOnly: never;
}
}
export class TicketCommand extends Subcommand {
public constructor(context: Subcommand.LoaderContext) {
super(context, {
name: 'ticket',
description: 'Gérer le ticket actuel',
preconditions: ['IsInTicket','GuildOnly', 'IsInTicket'],
runIn: CommandOptionsRunTypeEnum.GuildAny,
subcommands: [
{
name: 'add',
chatInputRun: 'chatInputAdd',
preconditions: ['IsInTicket',"TicketModerate"]
},
{
name: 'remove',
chatInputRun: 'chatInputRemove',
preconditions: ['IsInTicket',"TicketModerate"]
},
{
name: 'close',
chatInputRun: 'chatInputClose',
preconditions: ['IsInTicket',"TicketModerate"]
},
{
name: 'switchpanel',
chatInputRun: 'chatInputTransfer',
preconditions: ['IsInTicket',"TicketModerate"]
},
{
name: 'rename',
chatInputRun: 'chatInputRename',
preconditions: ['IsInTicket',"TicketModerate"]
},
{
name: 'addrole',
chatInputRun: 'chatInputAddRole',
preconditions: ['IsInTicket','TicketManager']
},
{
name: 'unclaim',
chatInputRun: 'chatInputUnclaim',
preconditions: ['IsInTicket','TicketModerate']
},
{
name: 'autoclose',
chatInputRun: 'chatInputAutoClose',
preconditions: ['IsInTicket','TicketModerate']
},
{
name: 'claim',
chatInputRun: 'chatInputClaim',
preconditions: ['IsInTicket','TicketModerate']
},
{
name: 'closerequest',
chatInputRun: 'chatInputCloseRequest',
preconditions: ['IsInTicket','TicketModerate']
}
]
});
}
export class TicketCommand extends Subcommand {
public constructor(context: Subcommand.LoaderContext) {
super(context, {
name: 'ticket',
description: 'Gérer le ticket actuel',
preconditions: ['IsInTicket','GuildOnly', 'IsInTicket'],
runIn: CommandOptionsRunTypeEnum.GuildAny,
subcommands: [
{
name: 'add',
chatInputRun: 'chatInputAdd',
preconditions: ['IsInTicket',"TicketModerate"]
},
{
name: 'remove',
chatInputRun: 'chatInputRemove',
preconditions: ['IsInTicket',"TicketModerate"]
},
{
name: 'close',
chatInputRun: 'chatInputClose',
preconditions: ['IsInTicket',"TicketModerate"]
},
{
name: 'switchpanel',
chatInputRun: 'chatInputTransfer',
preconditions: ['IsInTicket',"TicketModerate"]
},
{
name: 'rename',
chatInputRun: 'chatInputRename',
preconditions: ['IsInTicket',"TicketModerate"]
},
{
name: 'addrole',
chatInputRun: 'chatInputAddRole',
preconditions: ['IsInTicket','TicketManager']
},
{
name: 'unclaim',
chatInputRun: 'chatInputUnclaim',
preconditions: ['IsInTicket','TicketModerate']
},
{
name: 'autoclose',
chatInputRun: 'chatInputAutoClose',
preconditions: ['IsInTicket','TicketModerate']
},
{
name: 'claim',
chatInputRun: 'chatInputClaim',
preconditions: ['IsInTicket','TicketModerate']
},
{
name: 'closerequest',
chatInputRun: 'chatInputCloseRequest',
preconditions: ['IsInTicket','TicketModerate']
}
]
});
}
I have a ChatInputSunCommandDenied too
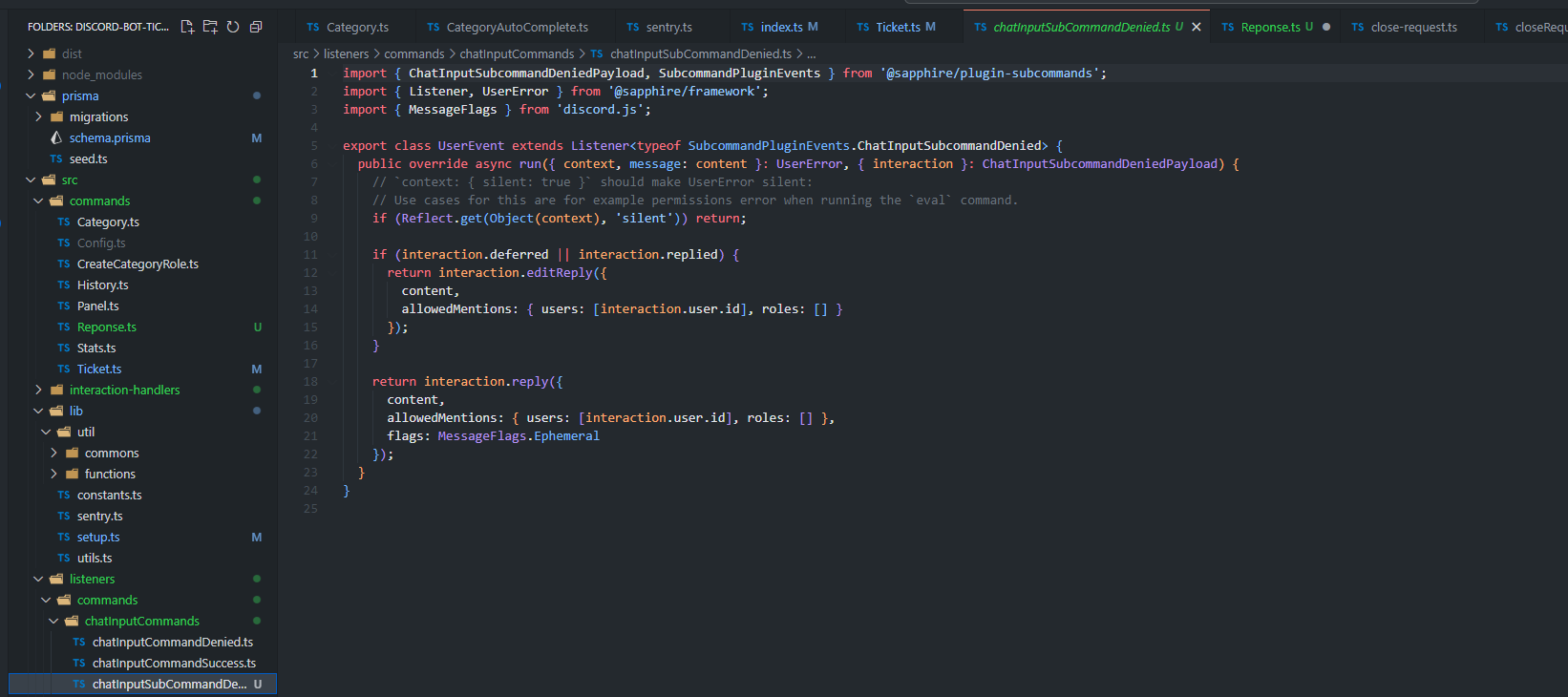
sun?
Sun ? I didn't understand what you mean sorry
Solution
Fixed, the problem was from my chatInputSubCommandDenied
you said SunCommandDenied just above and in case you copypasted it that wouldve been a typo
yeah I just seen that sorry
Thank you for your help
Have a nice day
Enjoying hard Sapphire