Custom page with resource record error
I have a custom page:
public static function getPages(): array
{
return [
'ticketWithComments' => Pages\TicketWithComments::route('/{ticketId}/ticketwithcomments'),
…
];
}
And I try to pickup the ticketId and fill the form with the following code:
public function mount($ticketId): void
{
$this->ticket = Ticket::findOrFail($ticketId);
$this->form->fill($this->ticket->attributesToArray());
}
But I’m getting error: Typed property App\Filament\App\Resources\TicketResource\Pages\TicketWithComments::$ticket must not be accessed before initialization
What am I doing wrong?Solution:Jump to solution
```php
use Filament\Resources\Pages\Page;
use Filament\Resources\Pages\Concerns\InteractsWithRecord;
class ManageUser extends Page...
11 Replies
You need to define it on the class
public $ticket;
I'm sorry, but I don't understand it.
This is my complete code:
class TicketWithComments extends Page implements HasForms
{
use InteractsWithForms;
use InteractsWithRecord;
public ?array $data = [];
public Ticket $ticket;
protected static string $resource = TicketResource::class;
protected static string $view = 'filament.app.resources.ticket-resource.pages.ticket-with-comments';
public function form(Form $form): Form
{
return $form
->schema([
TextInput::make('title')
->autofocus()
->required(),
TextInput::make('personable.full_name')
->required(),
])
->statePath('data')
->model($this->ticket);
}
public function mount($ticketId): void
{
dd($this->ticketId);
$this->ticket = Ticket::findOrFail($ticketId);
$this->form->fill($this->ticket->attributesToArray());
}
}
I already did defined it on the class?you are declaring public as Ticket but your class need torun before hitting it. For example is you do: Public $ticket; does it work without the delcartion of the class?
I'm trying to understand what you mean. Where do I have to run the class? and do I do that with public $ticket; ?
Maybe you have a working example for me?
This is how you do it @Rick Doetinchem
Thanks Matthew! It's working but also after I deleted the use InteractsWithRecord trait. I think I used 2 separate method to retrieve the model.
I have it working with Ticket::findOrFail But how do you do this if you want to use the resolveRecord with the InteractsWithRecord trait like documented in the filament documentation on https://filamentphp.com/docs/3.x/panels/resources/custom-pages#using-a-resource-record
Right now I'm getting this error (see attached image) with this code:
class TicketWithComments extends Page implements HasForms
{
use InteractsWithForms;
use InteractsWithRecord;
public ?array $data = [];
protected static string $resource = TicketResource::class;
protected static string $view = 'filament.app.resources.ticket-resource.pages.ticket-with-comments';
public function form(Form $form): Form
{
return $form
->schema([
TextInput::make('title')
->autofocus()
->required(),
TextInput::make('personable.full_name')
->required(),
])
->statePath('data')
->model($this->ticket);
}
public Ticket $ticket;
public function mount(int | string $record): void
{
$this->ticket = $this->resolveRecord($record);
$ticketAttributes = $this->ticket->attributesToArray();
$this->form->fill($ticketAttributes);
}
}
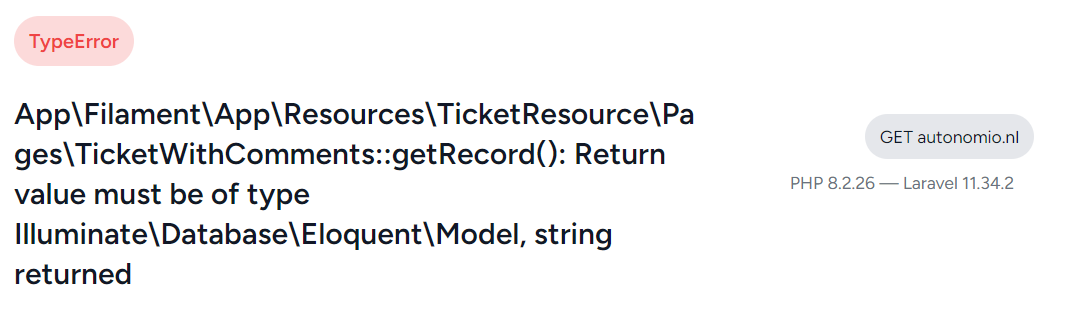
nono, without ineractswithrecord, you can use resoleRecord
try this:
Okay, that doesn’t answer my question. I am curious about how to use resolveRecord() and InteractsWithRecord() correctly, as mentioned in the Filament documentation.
If I'm not mistaken, you have to have
public Ticket $record
and this will resolve it automatically
Also you URL param has to be named {record}
Solution
Code like this worked for us and our demo cases