All other relations drop when creating a new one
export const homologationSchemas = createTable(
"homologation_schemas",
{
id: serialUuid(),
schema: jsonb().$type<AnyStateSchema>().notNull(),
name: varchar().notNull(),
description: text()
},
(hs) => ({
schema_index: index("schema_idx").on(hs.schema),
})
);
export const homologations = createTable(
"homologations",
{
id: serialUuid(),
name: varchar().notNull(),
productId: uuid("product_id"),
stateSchemaId: uuid("state_schema_id").notNull(),
},
(h) => ({
state_index: index("state_idx").on(h.state),
})
);
export const products = createTable(
"products",
{
id: serialUuid(),
name: varchar().notNull(),
}
)
export const homologationSchemaRelation = relations(homologations, ({ one }) => ({
stateSchema: one(homologationSchemas, {
fields: [homologations.stateSchemaId],
references: [homologationSchemas.id]
}),
}),
}))
// When I created this relation, the relation above just stops working (vscode doesn't show the property `product`, but it returns the data)
export const homologationProductRelation = relations(homologations, ({ one }) => ({
product: one(products, {
fields: [homologations.productId],
references: [products.id],
relationName: "relationname"
})
}))
// idk
export const productHomologationsRelation = relations(products, ({ many }) => ({
homologations: many(homologations, { relationName: "relationname" })
}))
export const homologationSchemas = createTable(
"homologation_schemas",
{
id: serialUuid(),
schema: jsonb().$type<AnyStateSchema>().notNull(),
name: varchar().notNull(),
description: text()
},
(hs) => ({
schema_index: index("schema_idx").on(hs.schema),
})
);
export const homologations = createTable(
"homologations",
{
id: serialUuid(),
name: varchar().notNull(),
productId: uuid("product_id"),
stateSchemaId: uuid("state_schema_id").notNull(),
},
(h) => ({
state_index: index("state_idx").on(h.state),
})
);
export const products = createTable(
"products",
{
id: serialUuid(),
name: varchar().notNull(),
}
)
export const homologationSchemaRelation = relations(homologations, ({ one }) => ({
stateSchema: one(homologationSchemas, {
fields: [homologations.stateSchemaId],
references: [homologationSchemas.id]
}),
}),
}))
// When I created this relation, the relation above just stops working (vscode doesn't show the property `product`, but it returns the data)
export const homologationProductRelation = relations(homologations, ({ one }) => ({
product: one(products, {
fields: [homologations.productId],
references: [products.id],
relationName: "relationname"
})
}))
// idk
export const productHomologationsRelation = relations(products, ({ many }) => ({
homologations: many(homologations, { relationName: "relationname" })
}))
3 Replies
Aparently, the schema works just fine, but the properties under
A query like that would return something like
with
drop
const results = await ctx.db.query.homologations.findMany({
with: {
stateSchema: {
columns: {
id: true,
name: true,
description: true,
}
},
product: true,
},
columns: {
id: true,
createdAt: true,
updatedAt: true,
comments: true,
name: true,
type: true,
},
orderBy: [desc(homologations.updatedAt)]
});
const results = await ctx.db.query.homologations.findMany({
with: {
stateSchema: {
columns: {
id: true,
name: true,
description: true,
}
},
product: true,
},
columns: {
id: true,
createdAt: true,
updatedAt: true,
comments: true,
name: true,
type: true,
},
orderBy: [desc(homologations.updatedAt)]
});
[
{
id: '42093116-0d62-4225-9fa5-a1509262d8f1',
createdAt: 2024-12-03T14:12:22.719Z,
updatedAt: 2024-12-03T14:12:22.719Z,
comments: null,
name: 'nombre homologacion',
type: 'new',
stateSchema: {
id: '42093116-0d62-4225-9fa5-a1509262d8f1',
name: 'nombre esquema',
description: null
},
product: {
id: '5e1eceb0-133a-46dc-972d-82775b4ab35d',
name: 'nombre producto',
brand: 'marca producto',
model: 'modelo producto',
manufacturer: 'fabricante producto',
description: null,
comments: null,
origin: null
}
}
]
[
{
id: '42093116-0d62-4225-9fa5-a1509262d8f1',
createdAt: 2024-12-03T14:12:22.719Z,
updatedAt: 2024-12-03T14:12:22.719Z,
comments: null,
name: 'nombre homologacion',
type: 'new',
stateSchema: {
id: '42093116-0d62-4225-9fa5-a1509262d8f1',
name: 'nombre esquema',
description: null
},
product: {
id: '5e1eceb0-133a-46dc-972d-82775b4ab35d',
name: 'nombre producto',
brand: 'marca producto',
model: 'modelo producto',
manufacturer: 'fabricante producto',
description: null,
comments: null,
origin: null
}
}
]
But the infered returned type drops all relations
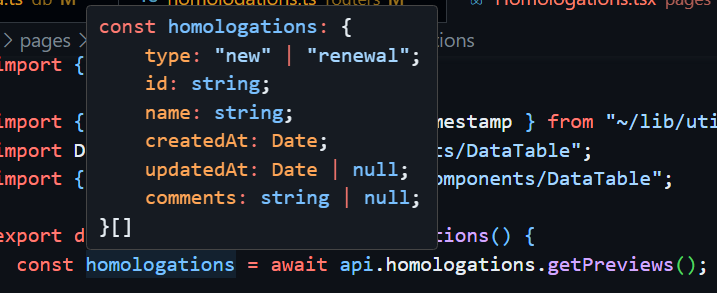
nvm you shouldn't define relations on one table using two different variables