Nuxt auto-imports with Nested component
How to make auto-imports work with Nested component? Say you have this inside
components/SomeComponent.ts
file
The SomeComponent
works in SFC, but not the nested one
6 Replies
Hi @TheSadMidDeveloper. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
To make auto-imports work with nested components in Nuxt, you need to understand how Nuxt scans and registers components. Based on the provided knowledge sources, here's what you need to know:
1. By default, Nuxt only scans files at the top level of the
components/
directory Docs > Guide > Directory-structure > composables > How Files Are Scanned (https://nuxt.com/docs/guide/directory-structure/composables#how-files-are-scanned).
2. For nested components, you have two options:
a. Re-export the nested components (recommended):
You can re-export the nested components from the main component file. This way, Nuxt will be able to auto-import them.
b. Configure the scanner to include nested directories:
You can modify the nuxt.config.ts
file to scan nested directories.
For your specific case, where you have a nested component defined within the same file, the auto-import system might not recognize it automatically. Here's what you can do:
1. First, try explicitly importing the nested component in your Vue file:
2. If that doesn't work, you might need to modify how you're exporting your components. Instead of using Object.assign
, try exporting them separately:
3. If you want to keep your current structure and make it work with auto-imports, you might need to use a local module to register the nested component. You can use the addComponent
function from @nuxt/kit
as described in the Docs > Guide > Directory-structure > components > npm Packages (https://nuxt.com/docs/guide/directory-structure/components#npm-packages) section:
Then, include this module in your nuxt.config.ts
:
This approach should allow you to use <SomeComponent.Test />
in your templates with auto-imports.
Remember, these are suggestions based on the available information. The exact solution might depend on your specific Nuxt version and configuration. If these approaches don't work, you might need to consult the latest Nuxt documentation or community resources for more specific guidance.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1214688654199496797> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
More info
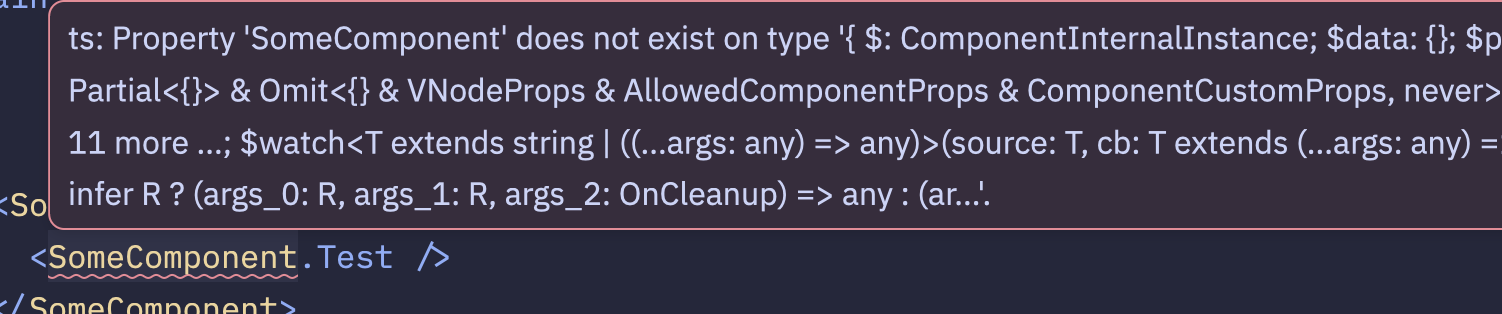
@kapa.ai Doing something like
SomeComponent.Test = Test
in your suggestion will break the typesLet me check the knowledge base for you again. One moment! :books:
----------------------
You're right, and I apologize for the oversight in my previous suggestion. You're correct that assigning
Test
to SomeComponent.Test
can indeed break the TypeScript types. Let's explore a more type-safe approach to handle nested components in Nuxt.
Based on the available knowledge sources, there isn't a direct solution to this specific issue. However, we can consider a few type-safe alternatives:
1. Use a separate file for the nested component:
Instead of defining both components in the same file, you could split them into separate files. This way, Nuxt's auto-import feature can handle them individually.
Then you can use them in your template like this:
2. Use a type-safe compound component pattern:
You can create a compound component structure that maintains type safety:
3. Use the resolveComponent
helper:
As mentioned in the Docs > Guide > Directory-structure > components > Dynamic Components (https://nuxt.com/docs/guide/directory-structure/components#dynamic-components) section, you can use the resolveComponent
helper provided by Vue:
This approach requires that you've properly registered the SomeComponentTest
component, possibly using the addComponent
method in a local module as described in my previous answer.
These approaches should help maintain type safety while working with nested components in Nuxt. However, it's important to note that the best solution may depend on your specific use case and project structure. If you're still facing issues, you might want to consider opening a discussion on the Nuxt GitHub repository or seeking help from the Nuxt community forums for more tailored advice.
kapa.ai: Based on the knowledge sources provided, there isn't specific information addressing how to handle nested components in a type-safe manner while maintaining auto-imports in Nuxt. The available documentation doesn't cover this particular scenario of defining nested components within a single file and preserving TypeScript types.
To properly answer this question, we would need more detailed information about Nuxt's handling of complex component structures and TypeScript integration. Without this information, I cannot provide a definitive solution that ensures both type safety and proper auto-importing for nested components defined in a single file.
If you're encountering type issues with the nested component approach, you may want to consider alternative component structuring methods or consult the latest Nuxt documentation and community resources for more up-to-date guidance on handling complex component scenarios with TypeScript.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1214688654199496797> Kapa.ai is still learning and improving, please let me know how I did by reacting below.