Get another writeline on second itteration
Im storing a username and password (fejk ones) in an array and using this for-loop i want the second pass to display "Pick a password", how do i go about this?
string[] user = new string[2];
for (int i = 0; i < user.Length; i++)
{
Console.WriteLine("Pick a username: ");
user[i] = Console.ReadLine();
}
}
99 Replies
It would be best to not use an array to store that data and not use a loop
Yeah, why not just have two separate parts?
Infinitely more readable and your question actually requires a lot of extra work
create a User class that will store the user and password
example
This was not their question at all
They use an array to store the two strings, not two individual users
Using a class would be a solution, though
But yeah, spoonfeeding bad
But if you're interested, here is the bad solution if you didn't use a class:
pls don't do this
it's not right to do this, store different things in one array :sadge:
Indeed, hence why my first question pointed out to just use two different calls rather than some array
But I think you confused the array with two distinct users that are being stored
If this isn't a homework question I will eat my hat
It's also pretty basic so I suspect a lot of the suggestions here are possibly out of reach without explaining more programming concepts
I doubt it
A ternary operator is not hard to understand
But regardless, my point is still that they should not overcomplicate their code so much and they should just make two individual calls as per my initial message. The rest should not apply apart from being "something to learn about"
If this is homework, then my answer would not make much for spoonfeeding since it's basically just me pointing out their overcomplicated code and the reason why it is so
Then again I would not be surprised if OPs question was from a paper that was given. Teachers tend to share horrible code to their students
Im actually supposed to create a login form using a menu, where i store username and password in separate arrays, then i login and also have an option to quit... iv tried everything i feel like, this is my code so far.. my if statement is not working at all. any ideas?
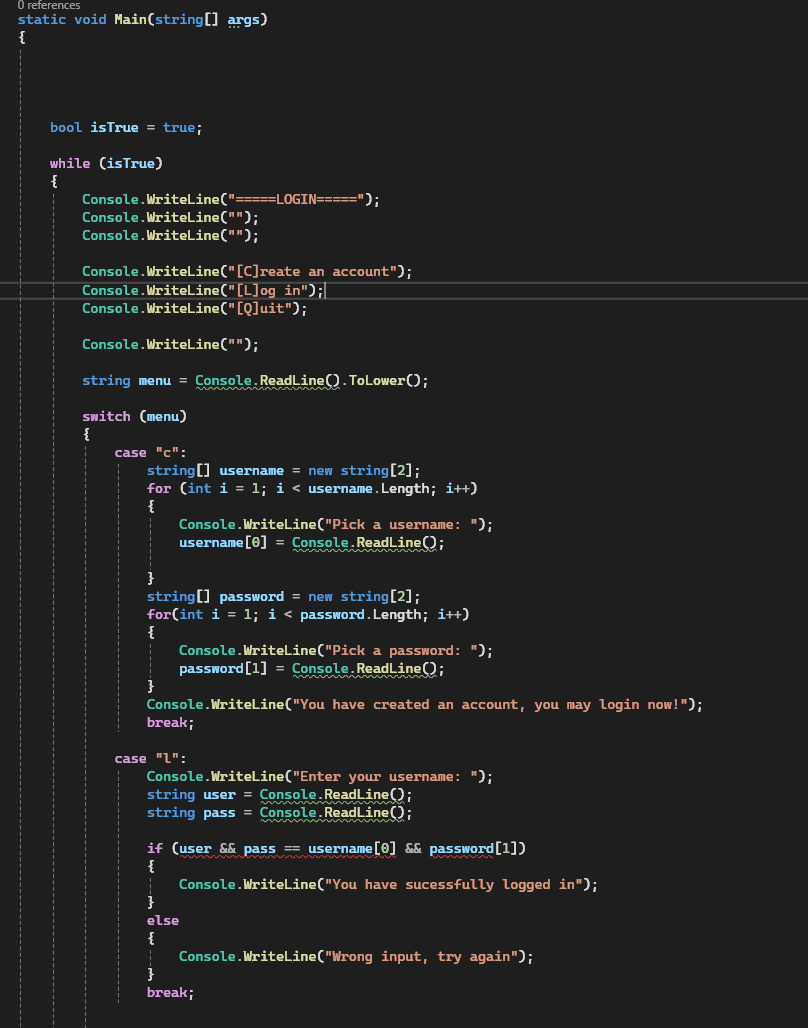
You don't need arrays here, get rid of them
Just make two string variables, one for
username
and one for password
The same way as with menu
I understand what your saying but my teacher has it as a requierments to store it in arrays, even tho its not the best option as you've said
You already have this actually. It's just that only one part has it
That sounds stupid
Is the reason because you must store multiple users?
That could be the only logical reason at least
Also, the reason your if-statement fails is because you check
user
like a boolean. This does not work because it is a string here
I think what you want here is the ability to register multiple users and to log in with one, is it?well the assignment just says to register your account, than login but also that all usernames and passwords should be stored in 2 separate arrays, so im guessing thats what she meant.
Okay, considering this is homework I can kind of see the point
Normally you would make a class, but I guess this is done at a later stage so for now we have each thing in its own array
And in this case you'd probably have multiple arrays for multiple users
Firstly you must understand the scope of things in your code
Can't do comparison this way btw

Yes I mentioned
Right now you have the arrays inside a case, for example. You can't read these in other case labels
The fix is to either put them above the
switch
, or outside the method entirely.oh i see, makes sense
In your case I'd just put them above the switch
Sorry, correction, put them above the
while
because then the array will keep existing every time this loop restarts
The next thing is being able to register multiple users
Right now you just loop through the array, but you should keep track of the index when you add a new user
Because you don't make two usernames, then two passwords. You specify a username and a password, and then put them in the array
What you can do is after your array creation you make an int
type which specifies the index of the array
int
types are numbers
Then in your c
case you ask for username and password, and you store them in the array based on that number
The syntax is the same as what you have already, but instead of 0
you pass the name of the variable
Then, when you are done you want to increment the number. You do that by doing myNumber = myNumber + 1
(yes, keen eyed developers, we know this can all be written better. This is for another time)
Also, I suggest you increase your arrays to a size of 50 or something. If your index is higher than the size of the array, your program will crash
There are ways around this. Also for another time
Anyway. Start with that. See if you can fill in both arrays once with each added user
@Yukonヌ
And feel free to share the result or ask more questions
Also, if you do want to share your code, then maybe use this site instead of sending screenshots: https://hatebin.com/Really appriciate the help, gonna get right to it after i take out the doggies, once again thanks for taking your time ♥️
No problemo
I intentionally tried not spoonfeeding here but if you get really stuck I can also just give examples
na its good, I wont learn anything but just getting "fixes" shoved in my face, rather just get some guidance for now :Ok:
Im even more confused now actually, since moving it outside of the while loop im not prompted to select from the menu before beginning, now i start with entering a username/password, how do i get around this?
You only need to move your array definitions outside of the while loop
So the
string[] username = new string[2]
(and change it to new string[50]
to be sure that it won't break too quick)
I assume you did more than thathaha yes I did 🗿
https://hatebin.com/jpbsrtuiqp
Yes. So code still works apart from your if-statement, right?
It would be a good idea to do this now
yea exactly, cant compare string to string[]
Your arrays exists so it can have multiple usernames and passwords, so now it's time to make use of it
See if you can make that work and feel free to ask questions
what i cant figure out is so that my for loops dont go through the whole array, im prompted to put in 50 usernames etc
You don't have to loop the array
Right now you have a for loop that runs until (in the case of username) runs until
i
is bigger than username.Length
.
Maybe you are confused by what Length
is here. It will always be 50, not the number of strings you addedoh yea that is true ... yea its the whole length of the array right, makes sense
If you want more control, you can also use a
List
. this is basically the same as an array, but it tracks its elements differently.
Ans also, its length will be the number of elements added
But in your case you don't need to loop it like you have now.
You do need to loop it eventually, but in my initial set of messages I mentioned that you should add a numeric variable that keeps track of it and increments with each added username/password
Basically this variable will be used instead
And if you use a List none of this is needed, but I suggest you try this first
Especially since the syntax of a List is more complex
A list uses an array internally, but with each added element it resizes the array to grow bigger and allow for more elements pretty much
Just useful to know, but try it with an array first.yea iv read about lists but seems like my teacher wants it to be stored in arrays, and they kinda confuse me abit. I did read about making an integer to keep track but im not sure how to attach it to the array
You don't attach it, it's a separate thing
But you must understand that the size you create the array at is the same size that
Length
returns
I think this confused you, did it?
Either way, the integer is just a basic number that tracks the actual size of the array
When you add a username and password, increment it by 1. This is now the new size according to the integer
You could even improve your code and ensure it doesn't exceed the array's Length
value, because it would otherwise crash the program
This can be done with a simple if-statement before you ask for the username and password
Oh, and the integer indirectly also specifies the index at which to add the username and password
Your current loop does the sameyea im really lost now, dont get me wrong I really appriciate you trying to explain im probably just too stupid, I cant get my head around what to do with the for loop and the int variables to let the user set the array size by input
Get rid of the while loop
Make an integer next to where you make the arrays
When you ask for username/password, just ask those and then store them in the array on the index of the integer
Basically this
oh so now i want to make a for loop and use index right?
You don't need loops yet
You just add it to the array using that integer variable as the index
so just ask the user to input username to store it then
usernames[index] = Console.ReadLine();
Yes, that works
Do the same for password, and then make sure to increment
index
In a proper application you'd store both of these in a class, and then put the class in an array. This way you only need 1 arrayI see, im getting use of unassigned local variable 'index' error on usernames[index] = "Foo";
nvm figured it out ...
😁
Do i need to use a for loop now or can i just in the login case compare with an if statement?
In the login statement you'd use a loop using the integer variable as the length
And inside you would compare the username. If that matches you compare the password. If that matches you log in.
https://hatebin.com/soeawndgss still dont understand how to use the for loop in this case, im not able to use the Length property
You only need to use 1 index, not two
Also, you correctly increment the index, but you must do this when you add a new entry
Also, you don't have to do
i = index;
. Just check the array using i
as the index rather than index
and do the comparisons as you had beforeI see but if im only using one index, how does it know to store username/password in the correct array?
That's why you have two arrays
But right now you store the password in the usernames array
username goes to the usernames array, password into the passwords array
You can use the integer variable for the index in both cases. You increment it by 1 after you inserted both pieces of data
Gotcha 👌 il continue tomorrow hopefully I get It to work, it's been doing my head in but it's fun 😊
All good, if it's getting too confusing then I can always give you parts
Morning, I think im doing something wrong with the loop here, or the if statement, https://hatebin.com/vkkiqhmrfb
am i not supposed to use the loop in the c case? so it stores, if u want to give example maybe that will make me understand abit easier
it seems to work now, the storing and comparing in the l case. https://hatebin.com/guykomazwl
I added another while loop in the login case to repeat the loop if user inputs wrong username/password. https://hatebin.com/npgqbpyonz
it also says in the assignment that user data is supposed to be saved in (RAM) to allow login but it does not need to be saved permanently, this is confusing me, you got any ideas?
also seems like im only saving one username and password, i cant create several than use each of them
Storing in RAM just means that you store it in a variable
Anything you do in the app uses RAM for storage, to a degree
"saving permanently" means saving in persisting storage. This is, for example, through a database or saving in a file
Because these changes stay available even after youe xit your application
So, regarding your code:
You currently use the index wrong. In the for-loop at the bottom you should make use of the
i
variable. Also, instead of incrementing index
you should increment i
. Index should only increment when you add a user, which in this case should be after line 41
Other than that it's a matter of putting your if-check on line 70 into the for-loop you created and then iterating the array until you either found one that is matching or the loop ends
Note that this means your else
at 77 should be outside of the loop, since it only ends if no user was foundbut you cant put an else statement outside an if statment right? their related, or im understanding this wrong
or do you mean another if statement like this? https://hatebin.com/zrqfkgzuoz
You don't need the else-statement
Just the body of code inside
The idea is that a loop compares the username/password that you pass with each array entry.
If it succeeds, you logged in and you can
return;
out of it
If it fails, it will end the for-loop because nothing was found and you can trigger the code below itu mean like this? https://hatebin.com/mmycwwpurb still my for loop is not correct i assume since now nothing seems to get stored, if you just wanna give me some examples im ok with it, il just review it untill i get the hang of things. I feel bad taking so much of your time
No, now you seem to be missing the whole flow of cases you had previously?
Also, your comparison still uses
index
rather than i
aah my bad, just showed that part of the code. Here's the whole thing https://hatebin.com/frdbaaydcs
73:name == usernames[index] && pass == passwords[index]I told you the issue here
47:passwords[index] = Console.ReadLine();I told you the code has to do something after this line
78:break;This one might be confusing, but since you are in a for-loop, this break actually only exists the loop. You want to use
return;
here to end the whole method since you're logged in.
Otherwise you log in, but it also logs that it failedyes after readline, iv got index = index+1 this should add it to the array right? and in the comparison i compare with i not index. im still not able to store more than the latest username/password.
https://hatebin.com/gsmphoripb
nvm i added the index=index+1 om the l case, its supposed to be when creating the account obviously, seems to work now, am able to store multiple accounts and use em
You put it in the wrong case
It is supposed to go into case 'c' because that's where you make the accounts
yea exactly, thats what i changed and now it seems to work
The whole point is to create the account, then increment the index to the next entry for the next account (and also to track the length)
So what is the code now?
Yes that looks basically perfect 👍
What you could do next is ensure your arrays don't overflow by checking
index
against usernames.Length
/passwords.Length
thanks for all the help, my brain feels like mush i tend to overthink alot... So basically make an if statement in the c case, like
if (index < usernames.Length && index < passwords.Length)
?
https://hatebin.com/wvluszthoj
this seems logical to me but does not work lol 😅One sec, having lunch
The check is fine but you need to do it before you put anything in the database
It's not a matter of stopping the increment, you want to stop the whole flow
So if the if-check passes, you want to write to the console that there's no more room
Then, the last line is
continue
This is similar to break
But where break exists out of a loop early, continue restarts it early
And in this case it will go back to your while-loop
Also, technically your if-statement only needs to check one of the arrays. The reason is because they will always be the same size. It's up to yougotcha, this seemd to do the trick https://hatebin.com/gdnzfznhhv
Yes, looks perfect
You can also make a "guard clause"
In this case it's basically your if-check, but reversed (
if (index == usernames.length)
)
If it passes, break early, otherwise continue the code
It removes a step of nesting which makes your code cleanerThanks for the tip, im happy so far, started c# last week, currently studying for webdevelopment and just finished html/css ... gotta say c# feels harder to get a grasp on but I guess in time it will be easier. Cant express how greatful I am that you take your free time to help a newbie like me 😁
Like with any language you just have to keep making things and keep learning
It took me years to learn C#, then years to learn proper C#
Honestly, in your case you make mistakes because you (probably) never programmed before, have you?
Well, I hope the program makes sense, especially since you wrote it all yourself
I see, yea it takes time to get decent at it, that much I understand. It does make sense except for the i variable in the for loop, how is index related to i here? It sounds more logical to me that i should be comparing name and pass to username[index] and password[index] rather than username[i] etc. I dont get how the i variable is connected to the index of my array
index
is your own variable and the only purpose is to indicate to the code where your array ends
You use it to indicate where you place the next username/password, but you can also use it to indicate in the for-loop where it ends because there are no more usenames
i
in this case is purely for the for-loop to loop through the array. It increments until it is the same value as index
, in which case it ends because the array would no longer have values
You don't use index there because it already has a purpose, and you can't change the purpose because it holds data about how big your array is
Again, with a List this would not be required but an array's size is not directly corresponding to how many items it has, so we must track this ourselvesoh I see, thanks for the explanation ❤️
i also added another case to handle unknown inputs for my menu, using default, is this a good option? https://hatebin.com/qfqcrfynos
Yes, this is exactly how you do this
I read that default should always be in the last case, I currently have it in the first case but that doesnt matter right? seems to work either way
The default case handles any case that is not handled by one of the cases above
I'm not sure if it matters here what the order is
I know there is a different switch type wher eit does matter, but the general rule is that it's at the bottom
Now that you have done this, perhaps you want to try adding some methods to handle your cases?
alright cool, what do you mean when you say handle my cases?
my next assignment is to make a library for books, where user and register/login and look for books and borrow them or add books, using search algorithms and methods. So il probably implement classes/methods here instead.
You have cases for different keyboard keys in this case
If your switch cases does not handle any case, then default is used if it exists
Yes that is definitely a good case where you do this
Hi gotta quick question regarding list and count. Im working on building a library and being able to rent books, this is just a snippet of what im having a issue with right now. I want the book.Count to update when someone rents a book, so the books gets removed and the remaining books are orderd from 1 to book.Count, what am i doing wrong here?
https://hatebin.com/sqkvjmsnqw
i tried having Id = book.Count aswell but doesnt seem to help
What is the issue?
I want the book = Id to update with the element position after I remove a book, right now if I remove the first book, the book with Id 2 gets in first place so to remove it I have to press 1
its kinda hard to explain hope you understand
A list updates itself to get rid of the item. Unlike an array which replaces it with the default value, being
null
in this case
I don't understand what you mean though
If you want the book, just get it and then remove it from the list
Also, pls fix this int rentBook = int.Parse(Console.ReadLine());
part
$tryparseWhen you don't know if a string is actually a number when handling user input, use
int.TryParse
(or variants, e.g. double.TryParse
)
TryParse
returns a bool
, where true
indicates successful parsing.
- Avoid int.Parse
if you do not know if the value parsed is definitely a number.
- Avoid Convert.ToInt32
entirely, this is an older method and Parse
should be preferred where you know the string can be parsed.
Read more hereso if i run the program now, and I input 1, I will remove the first book, new iteration shows books with ID 2-10, now I want to be able to press 2 to remove the first book of the updated list
Oh, you want the index to be based on the book?
exactly
Well right now it's based on list index, not id
So if you want this you could use a for-loop, and compare the Id of the book on an index against the index you gave
Then, as before, you use
i
from the for-loop to remove the book on the given index.
There are easier ways, like using LINQ, but I'd say you try this firstI see, not sure I fully understand how to implement this :/
Same as the previous code
I'd say create the loop and try to do as much as you can
Iv tried googling cant seem to find any info, everyone gives linq as the option for this.
You can use LINQ, but the syntax is very complex because it uses a new style you haven't used yet
I don't see why you can't just use a loop. Have you tried?
I mean yea im just so confused with what to iterate exactly in the for loop
You iterate each book by accessing it the same way you have done before, by using the indexes (
[i]
) and then comparing the int you gave through user input against the idhttps://hatebin.com/hksgpejcom not getting it to work at all now
I don't get how this is so hard to be honest
You made a loop here that does exactly what you need
You go into the index of the array, but in this case you don't get a string but an object
So instead of comparing directly, get the id like you did before from the object and compare this against
rentBook
Just make sure you break;
out again so you don't continue loopingIv compared the rentBook if its equal to the book[i].Id, this is what you meant right? To compare so that the user input equals one of the ID's. I dont know why its so hard, but its confusing the hell out of me, since accessing the property in an object is something iv never done before. https://hatebin.com/uiwkztdcdh
That looks good
Just the
else
is wrong because now it breaks out as soon as it doesn't match
That has to go outside of the loop, but in this case you need to add an additional bool that checks if a book was found
Because your break;
breaks out of the loop and will otherwise also log the wrong choicebut if it goes outside of the loop i cant check the index? or am i wrong? you mean something like this, https://hatebin.com/bhadwluksr ?
Set the bool when it is found inside the loop and send a message, then outside the loop check if it's set, otherwise it was not found and the alternative method should be send
I decided to not have books removed, I added a method instead for IsRented: https://hatebin.com/fskenomquh
Sorry I have been super busy
This looks good, I see you have used a lambda
Which is more advanced
Does this work?
Your code seems to look better every time you share it