Displaying in App isn't working as intended.
Hello can someone pin point what I am doing wrong here?
This is supposed to display each equipment slot, Type of slot ( Head, chest, Legs ) with the item name or as empty.
It only displays Head: Empty For each slot ( they are all empty )
Here is how I initialize my Equipment Class
Here is how I get fill my List of Slots
Here is EquipSlot if needed
Thanks for looking appreciate it!
public void DisplayEquipment()
{
Console.WriteLine("\n=== Equipment Status ===");
var slots = EquipmentSlots();
foreach (var slot in slots)
{
string slotName = slot.SlotType.ToString().PadRight(10);
string itemName = slot.Item != null ? slot.Item.Name : "Empty";
Console.WriteLine($"{slotName}: {itemName}");
}
Console.WriteLine("=====================\n");
}
public void DisplayEquipment()
{
Console.WriteLine("\n=== Equipment Status ===");
var slots = EquipmentSlots();
foreach (var slot in slots)
{
string slotName = slot.SlotType.ToString().PadRight(10);
string itemName = slot.Item != null ? slot.Item.Name : "Empty";
Console.WriteLine($"{slotName}: {itemName}");
}
Console.WriteLine("=====================\n");
}
public class EquipInventory
{
public bool TwoHanderEquipped;
public EquipSlot HeadSlot { get; private set; }
public EquipSlot ChestSlot { get; private set; }
public EquipSlot LegsSlot { get; private set; }
public EquipSlot FeetSlot { get; private set; }
public EquipSlot HandsSlot { get; private set; }
public EquipSlot MainHandSlot { get; private set; }
public EquipSlot OffHandSlot { get; private set; }
public EquipSlot Ring1Slot { get; private set; }
public EquipSlot Ring2Slot { get; private set; }
public EquipSlot NeckSlot { get; private set; }
public EquipSlot BackSlot { get; private set; }
public EquipSlot WaistSlot { get; private set; }
public EquipInventory()
{
InitializeSlots();
}
private void InitializeSlots()
{
HeadSlot = new EquipSlot(EquipmentSlotType.Head);
ChestSlot = new EquipSlot(EquipmentSlotType.Chest);
LegsSlot = new EquipSlot(EquipmentSlotType.Legs);
FeetSlot = new EquipSlot(EquipmentSlotType.Feet);
HandsSlot = new EquipSlot(EquipmentSlotType.Hands);
MainHandSlot = new EquipSlot(EquipmentSlotType.MainHand);
OffHandSlot = new EquipSlot(EquipmentSlotType.OffHand);
Ring1Slot = new EquipSlot(EquipmentSlotType.Ring1);
Ring2Slot = new EquipSlot(EquipmentSlotType.Ring2);
NeckSlot = new EquipSlot(EquipmentSlotType.Neck);
BackSlot = new EquipSlot(EquipmentSlotType.Back);
WaistSlot = new EquipSlot(EquipmentSlotType.Waist);
}
public class EquipInventory
{
public bool TwoHanderEquipped;
public EquipSlot HeadSlot { get; private set; }
public EquipSlot ChestSlot { get; private set; }
public EquipSlot LegsSlot { get; private set; }
public EquipSlot FeetSlot { get; private set; }
public EquipSlot HandsSlot { get; private set; }
public EquipSlot MainHandSlot { get; private set; }
public EquipSlot OffHandSlot { get; private set; }
public EquipSlot Ring1Slot { get; private set; }
public EquipSlot Ring2Slot { get; private set; }
public EquipSlot NeckSlot { get; private set; }
public EquipSlot BackSlot { get; private set; }
public EquipSlot WaistSlot { get; private set; }
public EquipInventory()
{
InitializeSlots();
}
private void InitializeSlots()
{
HeadSlot = new EquipSlot(EquipmentSlotType.Head);
ChestSlot = new EquipSlot(EquipmentSlotType.Chest);
LegsSlot = new EquipSlot(EquipmentSlotType.Legs);
FeetSlot = new EquipSlot(EquipmentSlotType.Feet);
HandsSlot = new EquipSlot(EquipmentSlotType.Hands);
MainHandSlot = new EquipSlot(EquipmentSlotType.MainHand);
OffHandSlot = new EquipSlot(EquipmentSlotType.OffHand);
Ring1Slot = new EquipSlot(EquipmentSlotType.Ring1);
Ring2Slot = new EquipSlot(EquipmentSlotType.Ring2);
NeckSlot = new EquipSlot(EquipmentSlotType.Neck);
BackSlot = new EquipSlot(EquipmentSlotType.Back);
WaistSlot = new EquipSlot(EquipmentSlotType.Waist);
}
public List<EquipSlot> EquipmentSlots()
{
return new List<EquipSlot>
{
HeadSlot,
ChestSlot,
LegsSlot,
FeetSlot,
HandsSlot,
MainHandSlot,
OffHandSlot,
Ring1Slot,
Ring2Slot,
NeckSlot,
BackSlot,
WaistSlot
};
}
public List<EquipSlot> EquipmentSlots()
{
return new List<EquipSlot>
{
HeadSlot,
ChestSlot,
LegsSlot,
FeetSlot,
HandsSlot,
MainHandSlot,
OffHandSlot,
Ring1Slot,
Ring2Slot,
NeckSlot,
BackSlot,
WaistSlot
};
}
public class EquipSlot
{
public EquipmentSlotType SlotType { get; set; }
public ItemObject Item { get; set; }
public EquipSlot(EquipmentSlotType slotType) { }
}
public class EquipSlot
{
public EquipmentSlotType SlotType { get; set; }
public ItemObject Item { get; set; }
public EquipSlot(EquipmentSlotType slotType) { }
}
5 Replies
At a glance, everything seems fine
Your best bet would be to use the debugger, I think
Yeah, far as I can tell, the code could be improved somewhat, but by all means it should work
Ah

Your constructor is empty
It does not set the slot type
Nor does it set the item
ah, thanks! my bad for something so trivial!
i didn't show the item assigning part, but it works with that small mishap
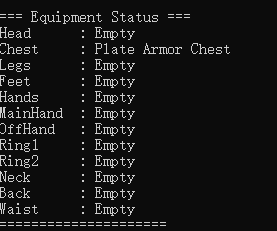