WPF login system
Hey all! hope everyone's doing well. I wanted a bit of help on a PRS system that I'm developing for a personal project. I want to make a login system for it using JSON but i've never actually read/wrote to a json file. im using the Nuget library as i've been told it would be best for me so i'm sort of just going with that. could someone help me on how to read from the json file and where exactly to start with the verification of it. my code and window has been attached below as screenshots. would really appreciate anybodys help!
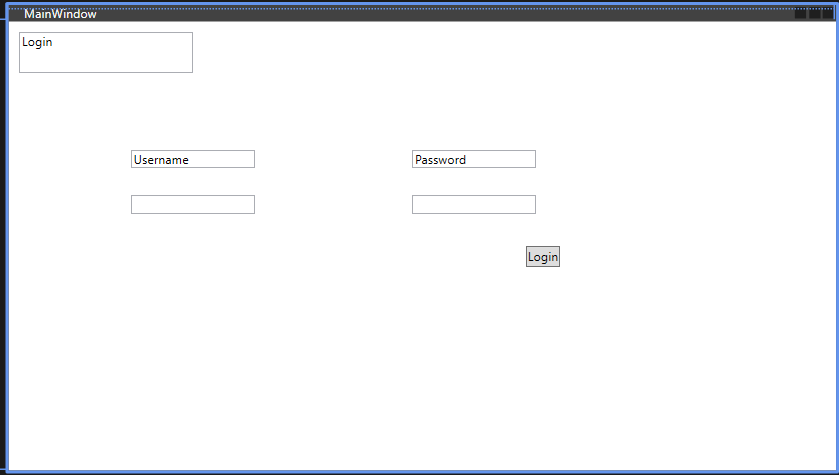
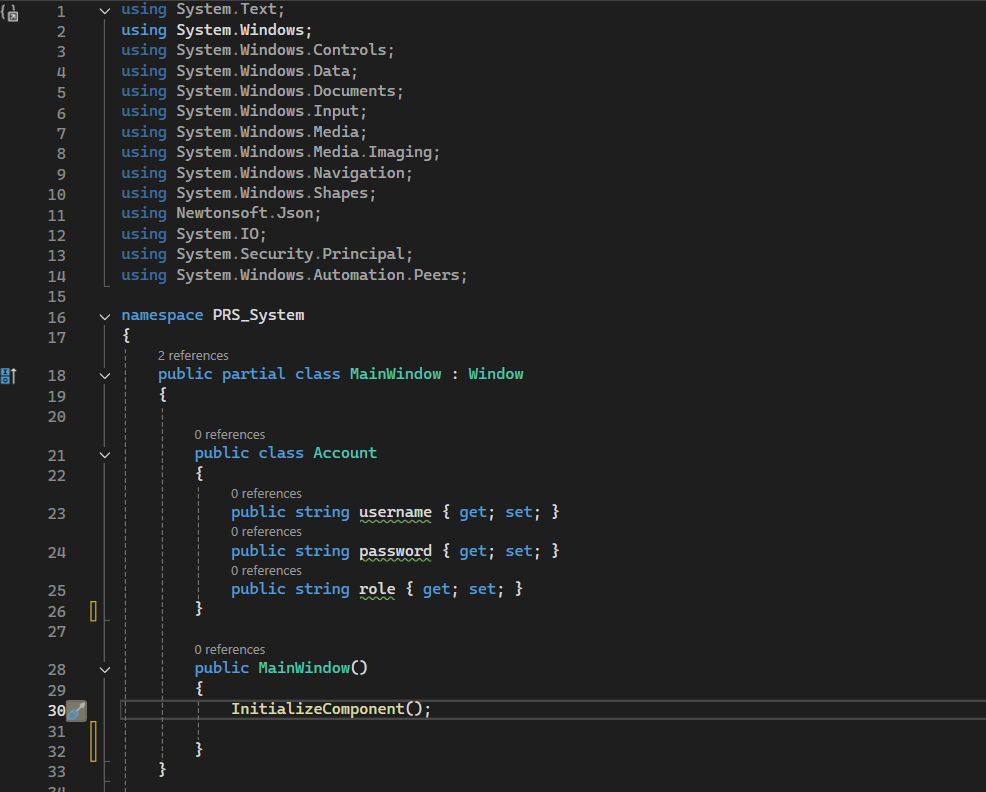
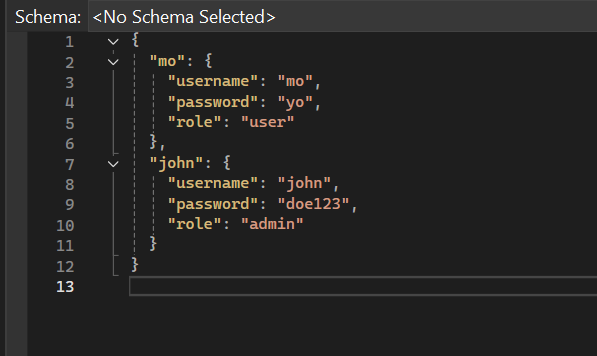
16 Replies
This is my solution
using Newtonsoft.Json;
wow thank you so much! i'll put this in and see how it runs and modify accordingly. could you explain what the first line means because i've never actually seen something like that before to be honest. also the "var json = JsonConvert.SerializeObject(this, Formatting.Indented);" could you please breifly explain that too if thats okay? 🙂
Can you paste into chat gpt and send what he replied?
yeah sure
for the readonly line: This declares a readonly string that holds the file path for storing the login data (login.json). In your program, we will change this to "accounts.json" since you are working with accounts.
var json = JsonConvert.SerializeObject(this, Formatting.Indented);:
This line converts the current object (referred to as this) into a JSON string.
JsonConvert.SerializeObject() is a method provided by the Newtonsoft.Json library that turns an object into a JSON string.
this refers to the current instance of the LoginData class (i.e., the object whose data you want to save).
Formatting.Indented makes the JSON output nicely formatted with indentation, which improves readability. Without this, the JSON would be compressed into a single line.
The result of this conversion is stored in the variable json, which will contain the string representation of the object in JSON format.
@GooBad
@GooBad
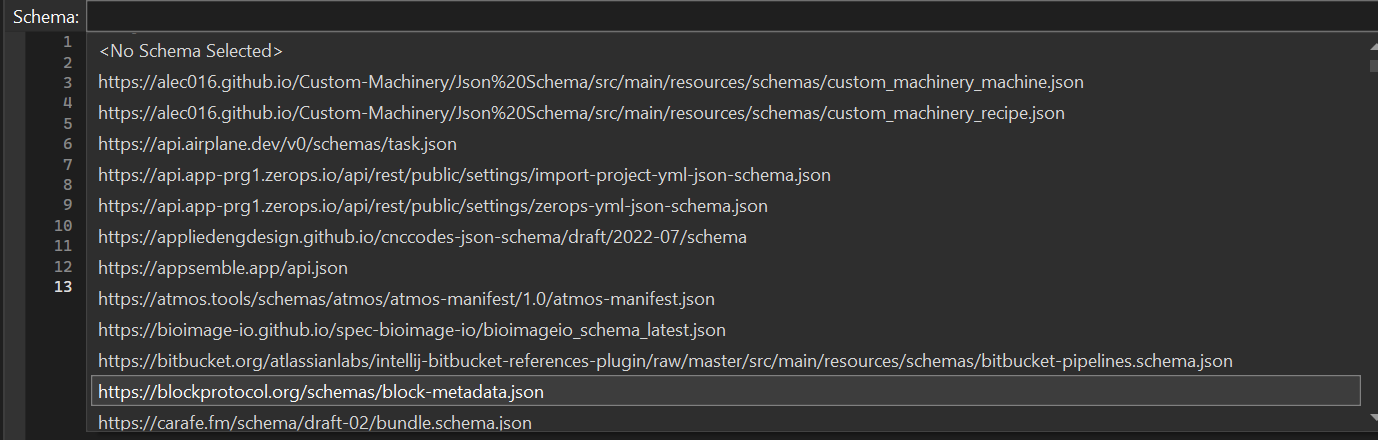
do i have to choose a schema?
because for some reason my code is not running its saying it cant find my file in the directory
You should post the error you got
wait sorry ignore me
i fixed it
Newtonsoft.Json.JsonSerializationException: 'Cannot deserialize the current JSON array (e.g. [1,2,3]) into type 'PRS_System.MainWindow+LoginData' because the type requires a JSON object (e.g. {"name":"value"}) to deserialize correctly.
To fix this error either change the JSON to a JSON object (e.g. {"name":"value"}) or change the deserialized type to an array or a type that implements a collection
i got this error however ^
Whats your error? Can you post errors in format like that:
So its readable in discord
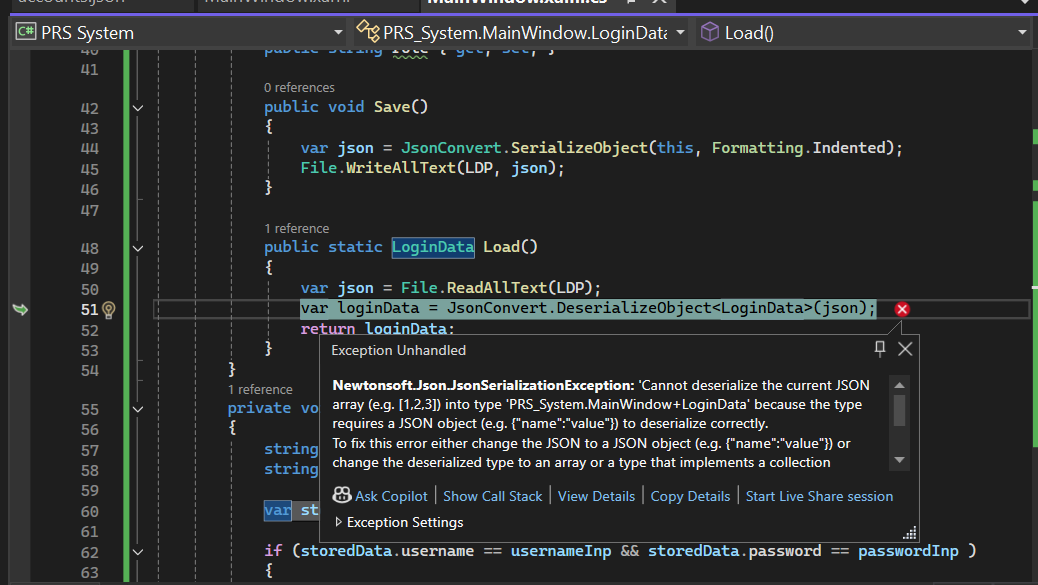
okay sorry!
but this is a screenshot of it
I suggest you try to resolve this error with chat gpt first :))
Send him code and error
Just FYI, there's zero need to use Newtonsoft nowadays
System.Text.Json
is built-inim honestly considering not using it now im getting so confused
about this serialisation and deserialisation
Serialization: turns an object into a JSON string
Deserialization: turns a JSON string into an object
It's really that simple
Angius
REPL Result: Success
Console Output
Compile: 619.469ms | Execution: 82.178ms | React with ❌ to remove this embed.