β Is it possible to Parse a string and identify it's type?
I want to scan an index of an array and identify if it's an operator such as (+,-,*) or if it's an operand (doubles). Is this possible?
101 Replies
basically in my if statement i'm triyng to identify if the string at the top of the stack is an operator or operand
My guess would be an if else statement to check the operators? else it's an operand?
You can use an if/else, you can use a switch, sure
nice
Also by any chance, do you know how i can identify the length of an array?
Does C# have a CBL for such a method?
uh
.Length
?
Or .Count
, I can never remember which is arrays which is listsHmmm
Are attributes not a part of the object that we create?

What attributes?
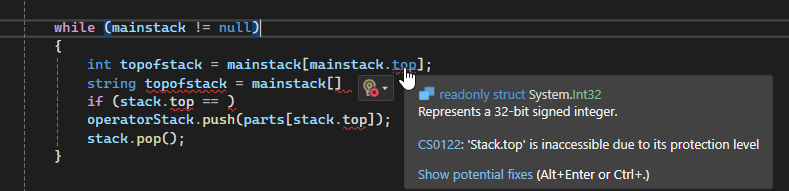
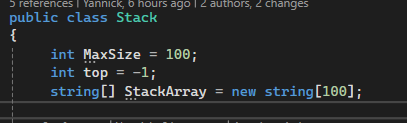
I'm trying to access the attribute "top"
But it says it's not accessible because of it's protection level even tho the class is public
it's private
oh i have to set the attributes to public if i want to use them?
C# defaults to lowest access if no access is specified
I see
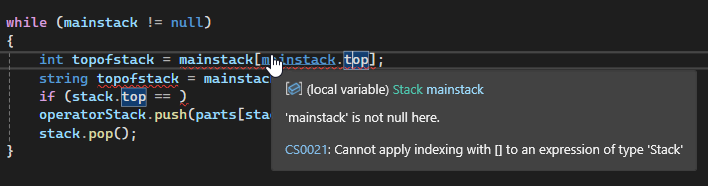
I don't get the type error?
top is of type int
Does
Stack
have an indexer?indexer?
This tells me that it does not:

Yes
You can't just get an element from anything with
[9]
or what have you
That thing must have an indexer
Yout StackArray
field in Stack
is an array, so that one would have an indexer by default
You could do mainstack.StackArray[mainstack.top]
But not mainstack[mainstack.top]
Not unless you implement an indexer:harold:
Using Indexers - C#
Learn how to declare and use an indexer for a class, struct, or interface in C#. This article includes example code.
Got another related question i think
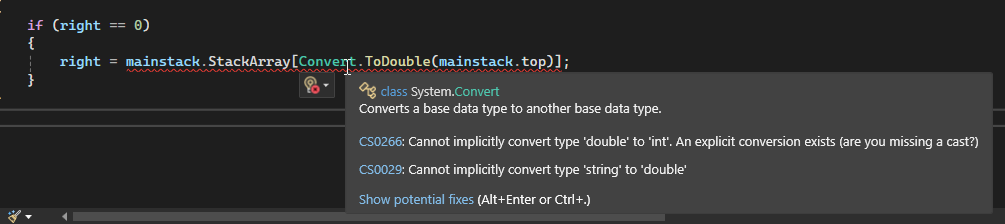
I'm trying to set right which is a double to the value at
mainstack.StackArray[mainstack.top]
Can i not convert a string to a double?
"Cannot implicitly convert type string to double"
I'm not entirely sure what that meansYou can parse a string into a double, yes
But
mainstack.top
is not a string
And you cannot index arrays with doublesmainstack.top
is an integerYes
Can i convert int to double?
Sure
But you cannot use a double to index an array
Ahhh
So i have to convert it first and then assign it to right?
uh
You use ints to index arrays
mainstack.top
is an intyea
Why would you convert anything here?
AAAh
`
Is that what you meant?
That seems better, yes
nicee very smart of you
Also, it's generally better to use
double.TryParse()
since it handles the string not being a double betterAngius
REPL Result: Success
Console Output
Compile: 521.251ms | Execution: 59.278ms | React with β to remove this embed.
Ahh i see
C# is so confusing sometimes
I don't really understand why some errors occur
Here for example. It says it doesn't exists, even tho it's clearly public ?


Does it exist in the same class?
Noo it's in a differenty class
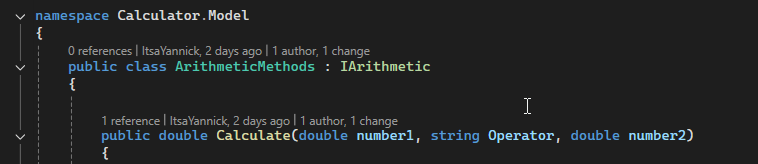
Well there you go, then
But both the class and the method are public
Sure
But this method is a member of a specific class
You can't just reference it and not the class
If the method is static, you just do
Class.Method()
If the method is not static, you need an instance of the class first
Aaaah right i completely forgot about that
π§
It worked!
nice
Got another question
:sadcat:
Shoot
I'm trying to read/write of files.
One
input.txt
and output.txt
. They are located in
C:\Users\aljom\Documents\Plugg Programmering filer\OOP\Assignment1\Assignment1\Calculator\Calculator\bin\Debug\net6.0
.
in my code i set my filepath to
It couldn't find them, not exactly sure what i messed upRight-click on each of those files, go to properties, set them to be copied always or copied if newer
In my folder on my pc?
In Visual Studio

Do you mean in the code?
No
Or should they be in the same file location as my solution explorer?
Ideally, place them next to the
.csproj
Then right-click them
And do what I mentioned
When doing so, they will be automatically copied to that .bin
folder when the project gets built and ran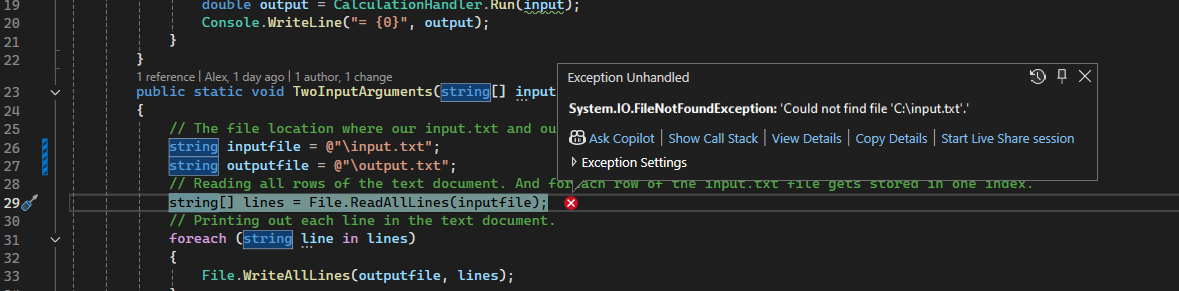
Remove that
\
Or replace it with ./
\
refers to the root, the root of the drive in this case
No slash or ./
will refer to the current working directoryOoh
ok the code ran! i'm not sure tho where it got placed
the copies that is
Probably in that
.bin/debug/......
Yeah i found them!
now all that remains is fix the code since it's not outputting wha ti want xD
I got a quick question about foreach loop and StreamWriter
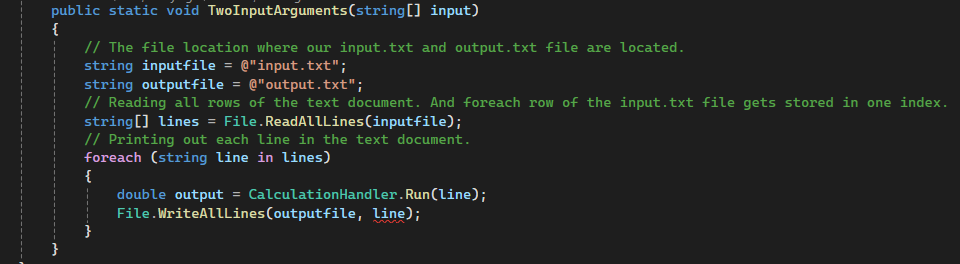
when applying a foreach loop
WriteAllLines
It expects an array of lines
ooh
In that case, are there any substitution to it?
Or would i have to take my double and convert it into an array of lines?
There are many options
You can keep appending to the file in the loop
Could use a stringbuilder to build a single string and use
WriteAllText()
Could store the calculated results in another array/list and write those as lines
And that can be done with either a loop like here, or LINQ
Plenty of choicesOh damn i didn't even think of that hehe
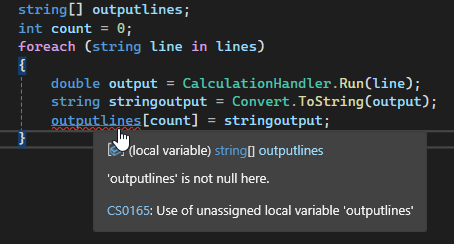
Shouldn't
outputlines
be defined inside my foreach loop?outputlines
has no value
It's empty, uninitialized
You have a space that says "apple crates go here"
You're trying to put an apple in a crate
But there are no crateshaha okay that makes sense
I just assume it auto initialized like in C
Nope
Nice works now!

Can the string 2.5 not be converted to decimals?
(or even double, i tried making it double)
I'm assuming you live in a country that uses a comma as decimal separator π
Just need to pass
CultureInfo.InvariantCulture
as the second parameter
Or any other culture that uses a period
Alternatively, you can set the culture of the whole threadHmmm
How do i pass
CultureInfo.InvariantCulture
as a second parameter?Just... do?
Assuming
Convert.ToDecimal()
takes it
?
ohh
A parameter
I thought it was pass it as an argument
$structure
Or rather everything inside () are arguments
Here's the naming reference
Aah i see!
I'm a little unclear how to handle exceptions
`
Remember when I mentioned the
TryParse()
method?
Like that
So you don't get exceptions but a nice bool
you can check with a regular if
$tryparseWhen you don't know if a string is actually a number when handling user input, use
int.TryParse
(or variants, e.g. double.TryParse
)
TryParse
returns a bool
, where true
indicates successful parsing.
Remarks:
- Avoid int.Parse
if you do not know if the value parsed is definitely a number.
- Avoid Convert.ToInt32
entirely, this is an older method and Parse
should be preferred where you know the string can be parsed.
Read more hereWell i think in our assignment we want to get an exception
Basically when the user tries different things
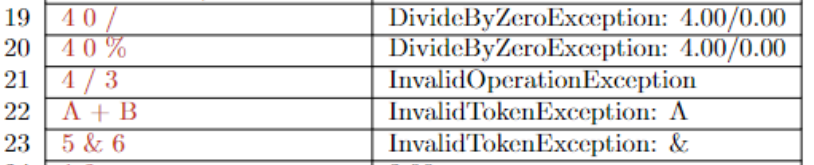
It should output the exception into the output file
Ah
Then yeah, you handle exceptions with a try/catch
But the thing in the image here I think is about creating and throwing your own exceptions
Yes, most likely
Okay i watched a few videos and tried again
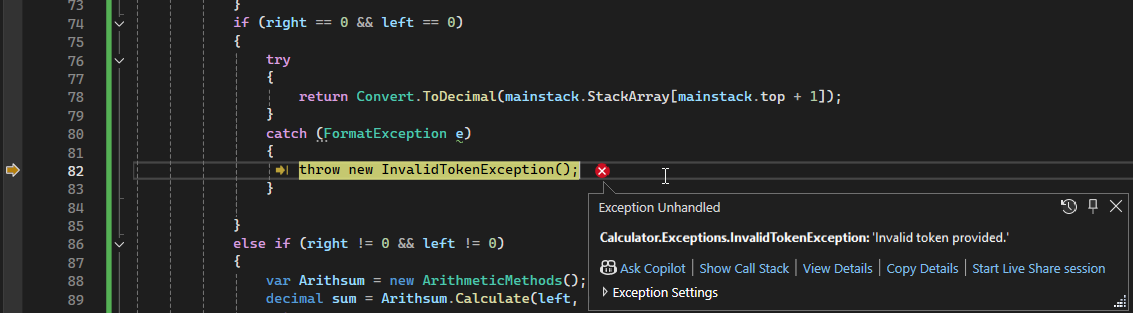
However i have nooo idea how to return it? xD