How to type a generic selection function
I've been trying to implement a generic select function but can't figure out how to type it
Something like this:
What type should I use to replace
Something
and SomethingReturn
I tried a combination of
and multiple combinations of PgSelect
, PgSelectBase
but can't get it to work properly
Any help appreciated12 Replies
@alexb Use the
SelectedFields
type (T extends SelectedFields
)@Mario564 thanks
SelectedFields really help but I'm still having issues
It works unless I add a
where
condition
ie:
return db.select(selectFields).from(users) // works
return db.select(selectFields).from(users).where(eq(users.id, '1111')) // returns "any"
Where is the
SelectedFields
being imported from? I'm looking at the source code and had forgotten there's one for each dialect, so you have to use the correct one for whatever dialect you're usingfrom
import type { SelectedFields } from 'drizzle-orm/pg-core'
That should work
Are you still explicitly defining the return type for the function?
Nope, letting typescript infer it
Attaching a more concrete example
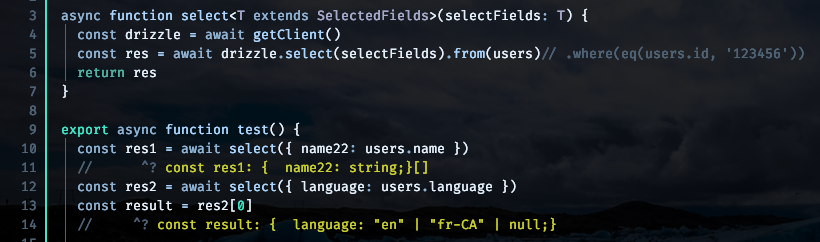
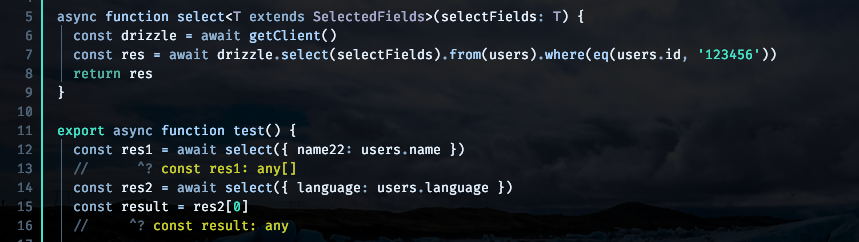
Couldn't find a clean solution to this problem, but I did come up with a workaround
Nice thanks!
yeah this is exactly the workaround I used
That'll do, I feel like this is a pretty standard use case though, surprised there's no clean way to do it :/
There probably is but it's not documented anywhere at the moment
The select types are also very complex so that certainly doesn't help
Thanks for your help! I'll update the thread if I ever find a better solution
Alright, glad to have helped!
Have you found any better solution? I too have similar kinda requirement