setInterval()
Steps to reproduce:title of the document should be changing as new messages every second.
But it doesnt happen here ,only on the initial refresh after 1 sec the title changes and remains num
82 Replies
Can anyone help me out with this.
At first glance that should work.
Presumably you are changing the value of "2" otherwise you won't see any change.
As a quick test, this works, updating the title with a random number every second:
I think closure is causing issue- You might want to try the code below; it works
function startUpdatingTitle() {
let messageCount = 0;
setInterval(()=> {
messageCount += 1;
document.title =
(${messageCount}) New Messages;
console.log(document.title);
}, 1000);
}
startUpdatingTitle();
This may not be relevant but it is also worth mentioning that this won't visibly work if you are testing this on a Codepen or similar.
This is because the output is being displayed in an iFrame so the document.title being updated will not be the global title, just the iframe contents title.
works fine for me
Why are you using setInterval instead of setTimeout? After the first change, all subsequent runs will set the same title, so you won't see anything else happen.
Why it doesnt change the static data though there is an interval it has to keep it blinking.It blinks only for the refresh then remains num
setTimeout occurs only once, but the requirement here is for every interval.
Why would it blink? That code will just update the value every second, not make it blink.
But every interval sets the exact same text. The code shared by Chris and Sanan update with different text, but your original code just updates with the exact same thing, so you won't see anything change.
You would have to set it to be empty before setting the message.
You could do that by including an setTimeout within the setInterval
But even then it wont actually be empty as the browser will give it the default title whilst it is "empty".
If you want a blink, you have to alternate between an empty string and the text that you want to be blinking.
Maybe something like this will get closer :
But the empty brackets will cause a slight jump between content as the "2" will take up more space.
(I'm not convinced that any of this is a good idea though. I personally. would find it very annoying)
yea
this means a toggle between browsers title and our title
so you mean with the same text we cant see that interval changeing the same text again and again.
If you could put the number at the end you would avoid the "jump" which might make it more acceptable
Exactly. It is just like updating the DOM, unless you add a timeout of some sort, the change is instant.
You must alternate with an empty or partial string to see it blink. Alternating a value with an identical value doesn't look like anything happened. I don't know why you expect a moment of blankness when there is a change. That is not something built into the system.
its not blink actually its like on/off
This works because there is a change in title every interval.
Mean while this doesnt have any effect the code wont update the title every second
yea we are updating the DOM only in 1sec .change is instant you mean we cant see the static text change?
i will be the downer here: how about you ... don't blink the number?
it's annoying, irritating, distracting and the user can't hide it
if something is asking for my attention, it better be very important
with that said, it is working: every second you're setting it to the same exact string
you are saying this is working?
yes, it is
nopes
if you close the blackets, it works
No i have closed it .it works only for the refresh and then remains static i cant see the updation
because ... you're always changing to the same value
every second, it's the same string
not a different one
Yes that was the discussion above.
then change to a different string
is that the thumb rule ?
that if anything is same we cant see in the DOM UPDATION
i want every second the same string should show that it has updated in the DOM. which is not possible
this should do it
it is, but if you change from the same to the same, you won't see a difference
stand up, and walk 0 steps forward and then 0 steps back
tell me how many times you've walked
Only standing is the task here nothing can be done apart from this.
no, you're saying "change to the string
a
"
and then you say "change to the string a
"
and you then say "change to the string a
"
guess what? you're going to get a
what did you expect? potatoes? a tv?
if you always set the same value, you always see the same value only
you have to set a different value to see a differenceyea that trigger or changing point i should see na
Okay okay
your example has has change in title
my code has a bug
this should work
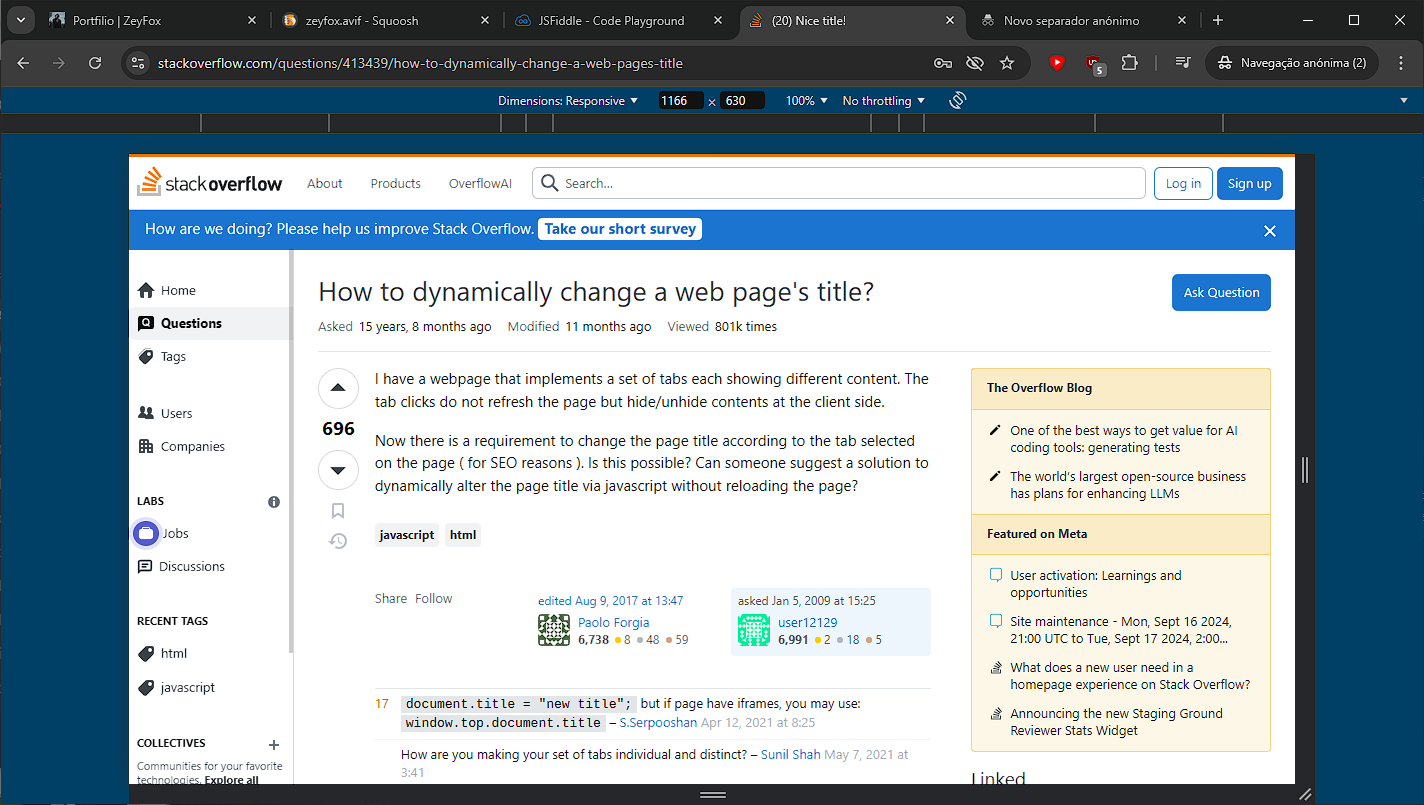
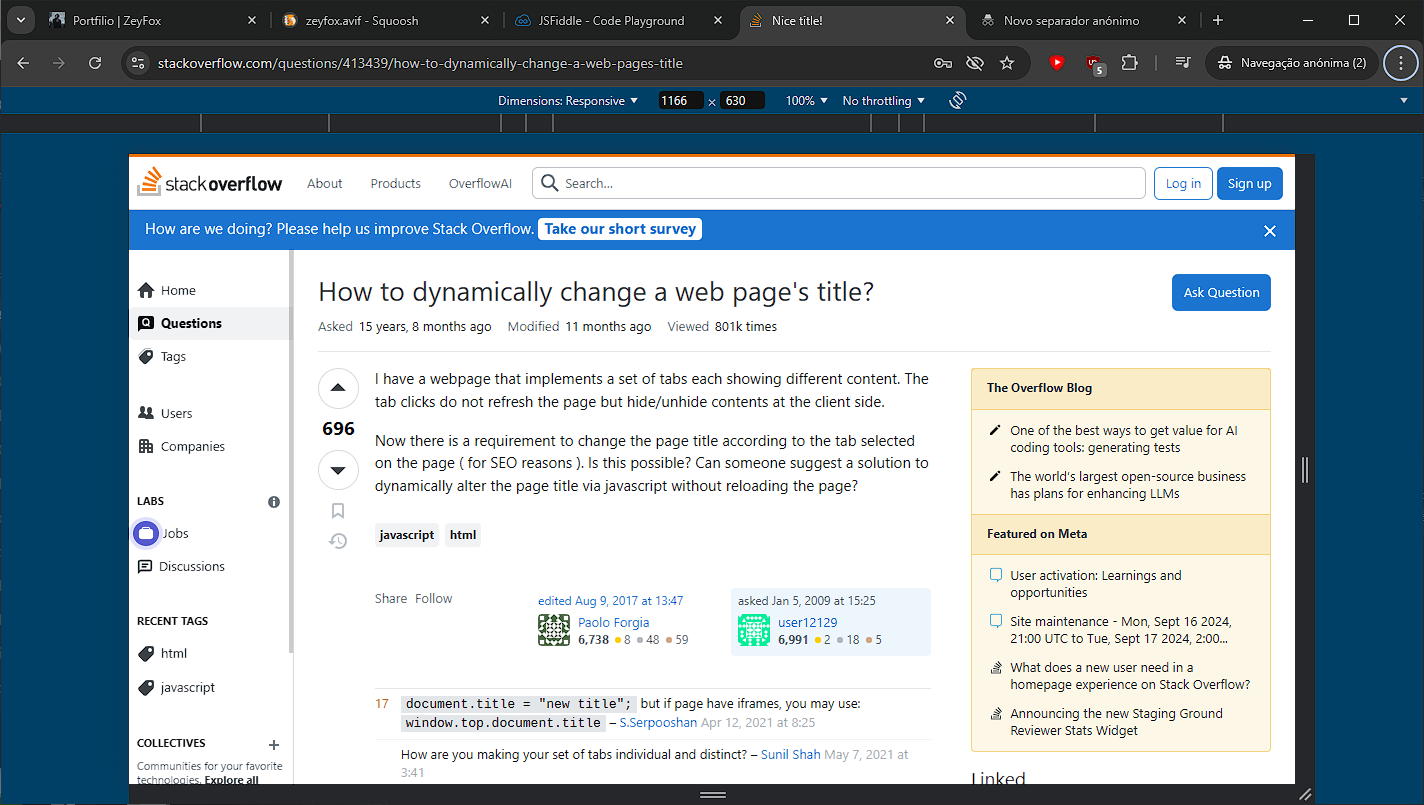
yes, i opened stackoverflow because it wasn't working, and i had to triple check for sanity
this is the equivalent of typing
1 + 1
in your calculator >.>means?
have you ever typed something super basic, like 2+3 in the calculator?
ofcourse why?
that's what i did, and im defending myself before you judge me >.>
hey i didnt judge you
infact i appreciate all of your efforts.
you know what i mean
but hey, it works, and it is just as irritatingly annoyingly annoying as i imagined
as of now i know only this
seriously i am not getting what are you talking about sanity and all
i am confused here as well
i just meant that my first example had a bug, and i was checking why it didn't work
and i opened stackoverflow to be sure that i was doing it right
then i used the page to test the code and get it to work
AHH sanity means you are saying checking 100 times that 1+1 is 2 or not
yes, because im getting 3, so, something wasn't adding up
(by "3" im mean that i was getting an unexpected result)
Great
did you look over stackoverflow for the logic or you coded on your own ?
i looked how to set a title on the document
Stack Overflow
How to dynamically change a web page's title?
I have a webpage that implements a set of tabs each showing different content. The tab clicks do not refresh the page but hide/unhide contents at the client side.
Now there is a requirement to cha...
this, it is what's in the browser
Thats great.
Thank you one and all for the explaination.
It was really a great help
you're welcome
do you understand my code?
Thats why i asked how did you write in a simple way
well, it is nothing extraordinary
i just use a boolean that im changing between true and false, and it decides if it shows the value or not
i always miss to write the boolean it wont flash to me
if it is to show the number, it just uses a template string
yes, which is why i said that you're changing into the same value, always
do you have any basic use cases to practice for this kind of stuff.
nope
it's just logic and experience
and planning, but i plan it all in my head
but you should learn a tiny bit of software engineering and learn how to do basic system analysis and how to make a data flux diagram
and also learn how to think in terms of splitting a task for an algorithm
yes agreed underated skill
it's sorely lacking
yea
most people that go to school don't learn anything related to this
trying to improve each day
so, you show them a new problem and they crumble like a spaghetti stick
string?
you know what i mean
?
you know, spaghetti isn't that strong
any pressure and it breaks
talking about myself?
no, fresh developers that come out of school
yea to make pasta
yeah, but now imagine someone who's developer skills are like that string of pasta
Including me
if it's laying on the table, it handles pressure okay
if you change the environment and just hang it a bit outside the confort of the table, it crumbles into nothing in moments
yEs pasta sticks
yes, that's what i meant
it doesn't mean i don't need help
but if i need help, it means im in serious serious trouble and what i need help with is absolutely niche or absolutely basic and idiotic
So overall you are saying if the problem or a feature is given to you, you will analayze in your brain how to do it.Then you write the logic on your own and here if you need help it will be kind of related to basics only.
mostly that, yes
what if that feature is new?
all features are new
Initial algorithm/analyze it in your brain would be difficult right?
to start with that feature itself would be like pasta sticks because we wont be having any idea about it like how to do it and all
not at all
in this case, it is something really simple
but something more complicated, i may do it on paper or in excalidraw
meaning in this post you are talking about,SetInterval.
I was asking about new features how would you approach.
i would need to know the system first, in and out and everything
and then i would need to know more about the feature: what's expected, what i have to put and where, how it will look like, how it should work, any specific functionality for this feature...
a lot of things
And then?
System Design?
and then, i plan on how i will implement everything and implement it