Beginner question: classes and constructor code?
So I've read this section a few times and it is not going in. I understand declaring regular variables, but this page in my book is talking about "public class MyNumber" then later goes on to say MyNumber numb = new MyNumber, what is the purpose of this and what is going on? Why can't they just stick to int a = 123, what is the point in all this new class and horrible syntax?
34 Replies
It reminds me a bit of enums from C which I also hated
I just found a really useful Youtube vid actually, it seems like classes are basically for times when an object requires more complexity than just 1 value, such as an employee, like employee ID, name, date of birth, etc - so that makes more sense now to me. Would appreciate any further input still if there's anything I should know...
C# classes are very different from C enums
The closest thing in C to C# classes are structs but they are still very different
For why you use classes, pretty much for encapsulation
Maybe an introduction to OOP would be helpful to you @proc
Classes are a concept of Object Oriented Programming and are used to model, well, objects. There are more concepts that work off of that idea.
Here's an official introductory tutorial on C# classes and how they relate to OOP:
https://learn.microsoft.com/en-us/dotnet/csharp/fundamentals/tutorials/classes
Classes and objects tutorial - C#
Create your first C# program and explore object oriented concepts
a critical idea is that classes aren't just a way to bundle related values together. they also let you bundle behaviour alongside the values it operates on. that is, a class can 'own' functions/methods
this makes it a lot easier to organise your codebase when you have 500 functions and 5000 bits of data floating around. with classes you can reasonably assume 'oh, the code for updating someone's username is probably in the User class'. it also means classes can hide away ugly implementation details from the rest of the world, and in turn the world doesn't have to care about how the User class works; it can just assume it does
as for the 'MyNumber numb = new MyNumber()' stuff. this is actually very similar to 'int a = 123'.
the only reason we don't have to repeat 'int' twice in that example is because ints are given special treatment by c# for convenience.
it was done this way partially as a response to c++ (i think), where there are about 5000 ways to create a new object. many of which are not obvious at all. in contrast c# has the new keyword and nothing else, so it makes it very easy to tell when new objects are being made
Really useful feedback all - thanks for this, makes a lot of sense now. One thing I don't now understand, having read onwards a bit and also watched a few videos on the same topic - is the constructors. I don't see what the purpose is of declaring a new class with a few variables in it, then directly below is creating a constructor which is the same name, and then each individual variable with this.name = name, this.value = value, etc etc. All videos explain that this is necessary but doesn't really explain why they are doing it in the first place...
For me it makes it appear very long-winded and of no purpose, as in the examples they are using you could probably create the same output with only a few lines of code (e.g. not even declaring a class, and only declaring variables by themselves). Is it meant to be for much larger programs that this becomes useful? Or is it really that tedious all the time?
Imagine you are using someone's libraby and want to create some object from that library, how would you know what values should you provide for that object for proper initialisation? In this case you check constructor for more information
Also you can provide in constructor something simple like int or string, but inside of that constructor you could create more complex objects out of more simple ones from it's parameters (sry for eng)
Yes, constructors are very verbose, full agreement here. Thankfully, there are ways to reduce that verbosity. Take
we can use a primary constructor instead
or we could skip the constructor altogether, ensure that our properties are given a value without it thanks to the
required
keyword, and use the initializer syntax instead:
this way, we can create a new instance like so:
Thanks for this - it's this part I couldn't understand what was happening:
Name = name;
Birthday = birthday;
ShoeSize = shoeSize;
To me it seemed redundant so I didn't know what the point of this was...
It's not redundant
You set the property value to the value of the parameter
Just like, say
Your example there makes more sense to me, but this one for example (i think he's called Bro Code on Youtube) he puts this code snippet. So the class part I completely understand what he is doing, and why - but the constructor part, is the brackets after "public Car (" arguments being passed to this constructor? And why does it have to be this.make = make; - etc - that part just seems pointless to me............
i put asterisk next to the bit which to me just seems pointless and can't get my head around...... really sorry if this is a stupid question!
I get that in future code, you could then put Car car1 = new Car - but why the constructor, what is that doing?
A constructor is a special method that gets called when a new instance of the object is created
When you have a constructor like
Car(String make, String model, int year, String color)
you're forcing everyone that attempts to create an instance of the that object to pass these arguments
These lines are just assigning the class's fields to the arguments passed to the constructorNice so immediately that makes sense to me now, just the this.make = make etc - I can't get
this.make
is refering to String make;
that lives in the objectIs "this" part of the C# language - e.g. it's not something the programmer has arbitrarily made up, it's a function or something
Yeah, it's a keyword in C#
this
refers to the current instance
of the object
You can omit it actually
For example
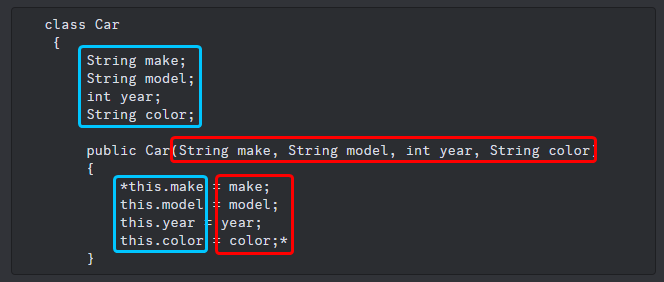
this
in this case is only needed because the name of the field is the same as the name of the parameterAlso, why is the tutorial using public fields :sad:
Usually, field names would be prefixed with
_
, so private string _make;
They're not public tho?Nvm, I'm blind
:Kek:
C# defaults to lowest access when no access modifier is given
Yeah ik, idk why I thougth they were
public
:OMEGALULiguess:What I want to question is why it uses
System.String
instead of string
Probably java developer
I really appreciate the feedback and help as I am learning it just feels like something isn't clicking with that - so you said it's assigning the class's fields, to the arguments passed to the constructor ...........let me just churn that through my thick skull for a sec...........
The other way around
It's assinging the class fields to the
values
of the passed in arguemnts
Not assigning to the actual parametersOh wait I think I might get it
It's like passing to functions in C where it might be void dothis (int a) - but you could pass it any variable with any name, it's only called a in that function -
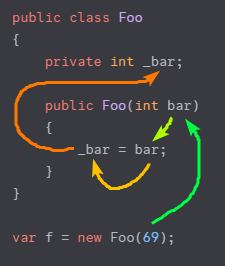
Yes
Ok the penny might have dropped there
works just fine
Ok ok ok that makes sense........ I really don't know why that just wasn't making any sense at all
Probably just because the names were the same on both sides it just looked like what the hell is this code trying to accomplish here
Legends , thanks again for this guidance !
o7
Anytime :Ok: