Help styling an component via props using TailwindCss.
Reusable button component:
usage:
I feel like there is something i'm not aware of.
At the browser nothing is being properly rendered, it does says it have the necessary classes, but the style isn't being applied:
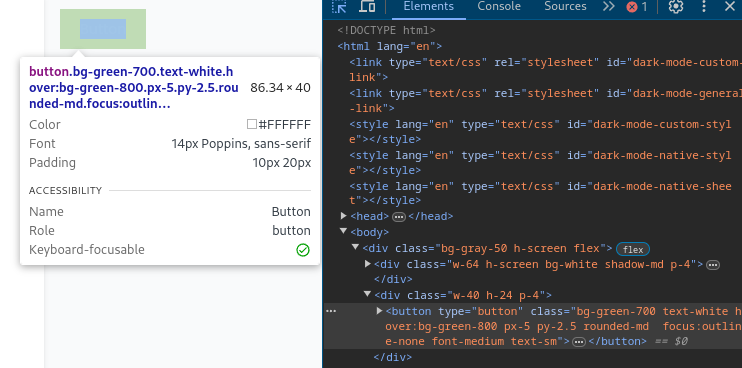
16 Replies
what do
buttonSizeStr
, buttonRoundedStr
etc look like?They take the property type value and return the proper tailwind class, example:
this doesn't work
Tailwind needs to see class names as a whole in your file to know what to compile
for example you need to write
text-green-700 hover:bg-green-800
in your buttonColorStr
instead of just green
.yep cva is great for this exact use case! Can recommend
This doesn't quite make sense to me, because the console.log shows the full class name for me:
bg-yellow-700 text-white hover:bg-yellow-800 px-5 py-2.5 rounded-md focus:outline-none font-medium text-sm
i'll take at look
I'd assume you meant tailwind needs to know the class at compile time, and base() is only getting it's value at runtime?yes
what tailwind does is it looks trough all your files and finds tailwind classes in strings
and your classes are dynamically generated and tailwind can't know what these values will be on runtime
and because of that it can't generate classes for you
makes sense, i"ll look for another option
@mrVinicius this page explains it very well: https://tailwindcss.com/docs/content-configuration#class-detection-in-depth
Content Configuration - Tailwind CSS
Configuring the content sources for your project.
And this is your issue: https://tailwindcss.com/docs/content-configuration#dynamic-class-names
Have a look at e.g. shadcn-solid implementation of a button and their tailwind config:
https://github.com/hngngn/shadcn-solid/blob/main/packages/tailwindcss/ui/button.tsx
GitHub
shadcn-solid/packages/tailwindcss/ui/button.tsx at main · hngngn/sh...
shadcn/ui, but for Solid. Contribute to hngngn/shadcn-solid development by creating an account on GitHub.
yeah, thanks yall
I ended up with something similar, as i decided to go with cva. I tried pandacss but not my thing.
only thing i'd change is to either make
variantClass
a function or call buttonStyles
directly in the class
attribute
spreading props in the component body will stop them from being reactivelike this id imagine:
i'll test this properly later, or else i'll use
splitProps
Yeah this is fine or use
splitProps
if typescript complains