Using sapphire paginator is it possible to customize the pages names ?
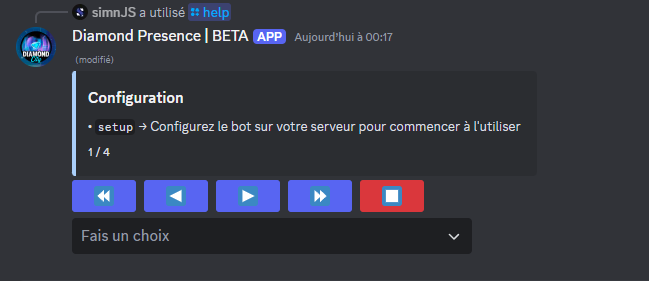
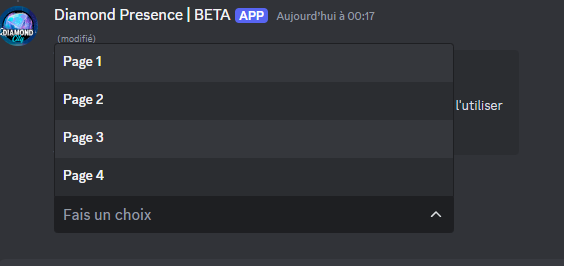
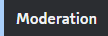
Solution:Jump to solution
```typescript
import { PaginatedMessage } from "@sapphire/discord.js-utilities";
import { Command } from "@sapphire/framework";
import { inlineCodeBlock } from "@sapphire/utilities";...
34 Replies
@simnJS you can do it like this
Yeah but how can I use a custom name for each page instead of an index
Maybe like that, right? @simnJS
Are you sure selectmenuoption exist ?

Solution
It's "setSelectMenuOptions" not 'setSelectMenuOptionsperPage'
Oh yes, sorry, I'm a little tired. 😅
The command doesn't work with that
I doesn't have logs
nothing
but it doesn't work
If i remove
it work correctly
I tried to logs all of my value all is defined
Oh I'm idiot
it's fine

it work now
Thank you @Swiizyy for your help
Pas de soucis mdr je viens de voir que tu est francais 😂
Ahah super, tu l'as pas vu à mon anglais catastrophique
ptdr
Leur doc pour sapphire utilities est trop mal foutu impossible de trouver ca au premier coup d'oeuil
j'ai le meme mdr vive le traducteur mdr
c'est vrai qu'il faut chercher un peut mdr
ptdr blagues-api je l'ai déja utilisé sur des projets
c'est golri
ouais c'est pas mal 🙂
Et ce genre de message de refus c'est ou que ca se custom ?

ptdr tu viens de me faire découvrir "userMention"
j'ai toujours fais <@id>
c un peu pareil en vrai
oui 😂
J'dois etre malchanceux

ca marche pas de mon côté
@simnJS
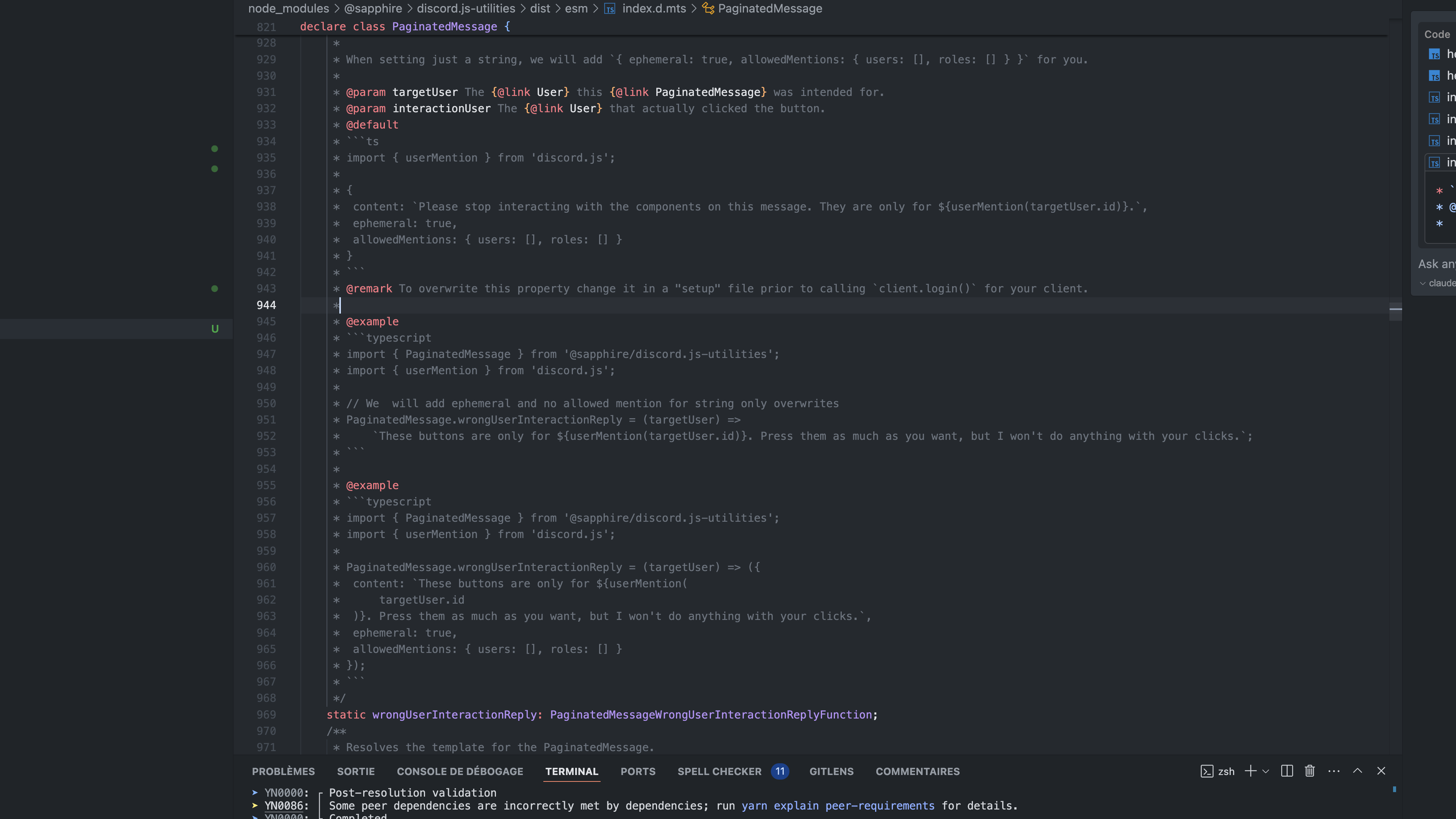
ahhhhhhhhhhhhhh
Faut que tu setup sa avant que sa appelle client.login :/
ok
relou okay
un peu

j'ai trouver autre chose
directement dans ton instance
de paginatedMessage
ah beh oui mdr
bien vu mdr
Bon bah super
merci pour tout
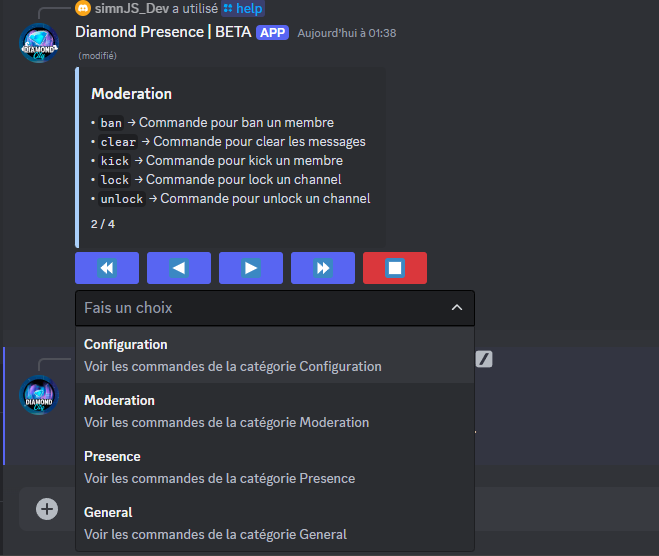
C'est mieux
quand même
Ah oui mdr
Toi qui a pas mal utilisé sapphire, niveau performance ca marche bien ?
oui ca marche vraiment bien