Hydration Mismatch. Unable to find DOM nodes for hydration key
Hi everyone
After create my "card" to list all projects I starting having the error
Hydration Mismatch. Unable to find DOM nodes for hydration key
, initial I suspect that is because I'm trying to use createPagination
primitive, but after comment the code related with the pagination the error persists.
I will leave the code on the thread24 Replies
routes/projects/index.tsx
components/cards/project.tsx
It may be because you're destructuring props
Already try both ways
If I click here it's working
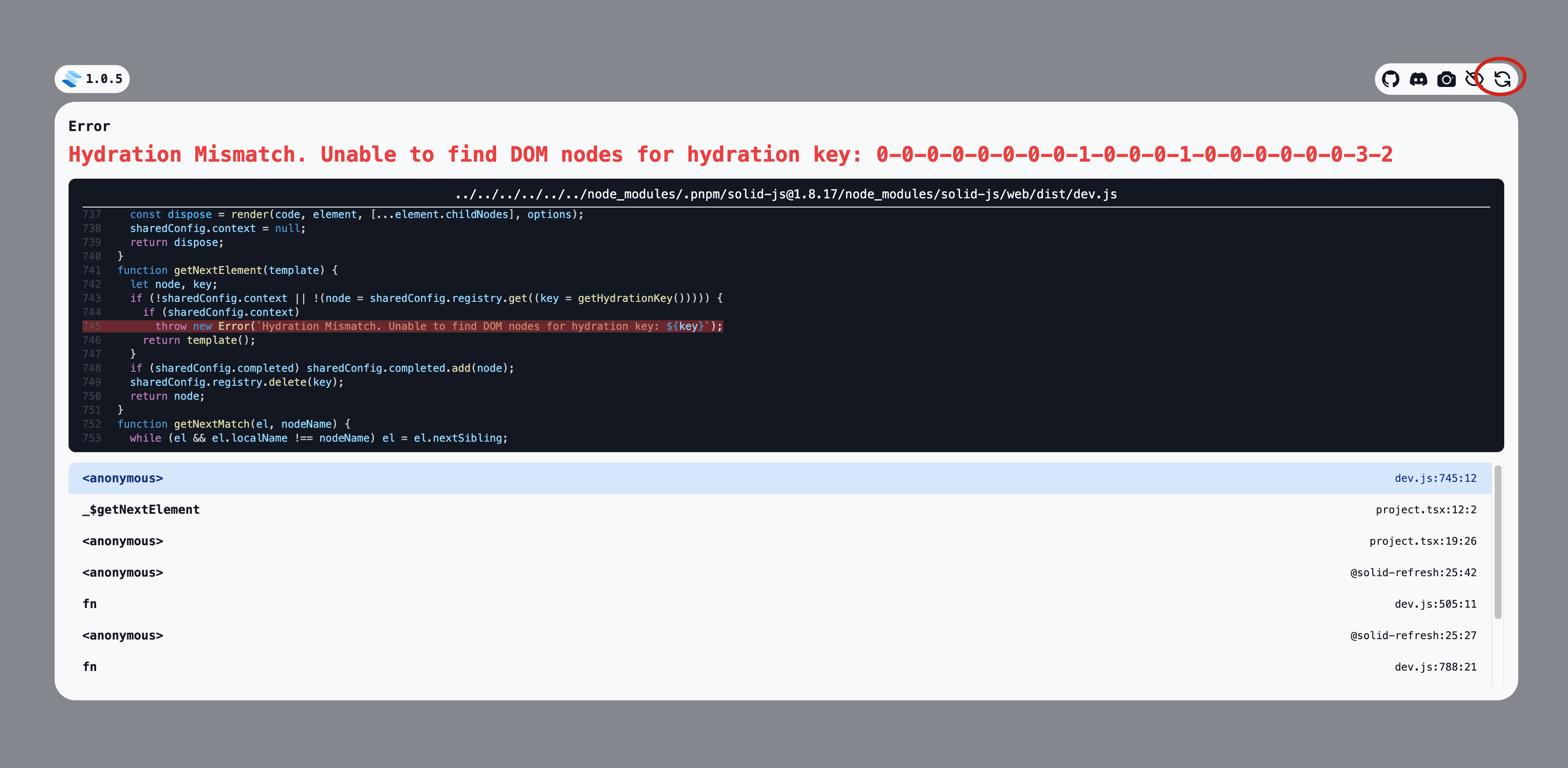
But only after click, if I refresh the page the error appears again
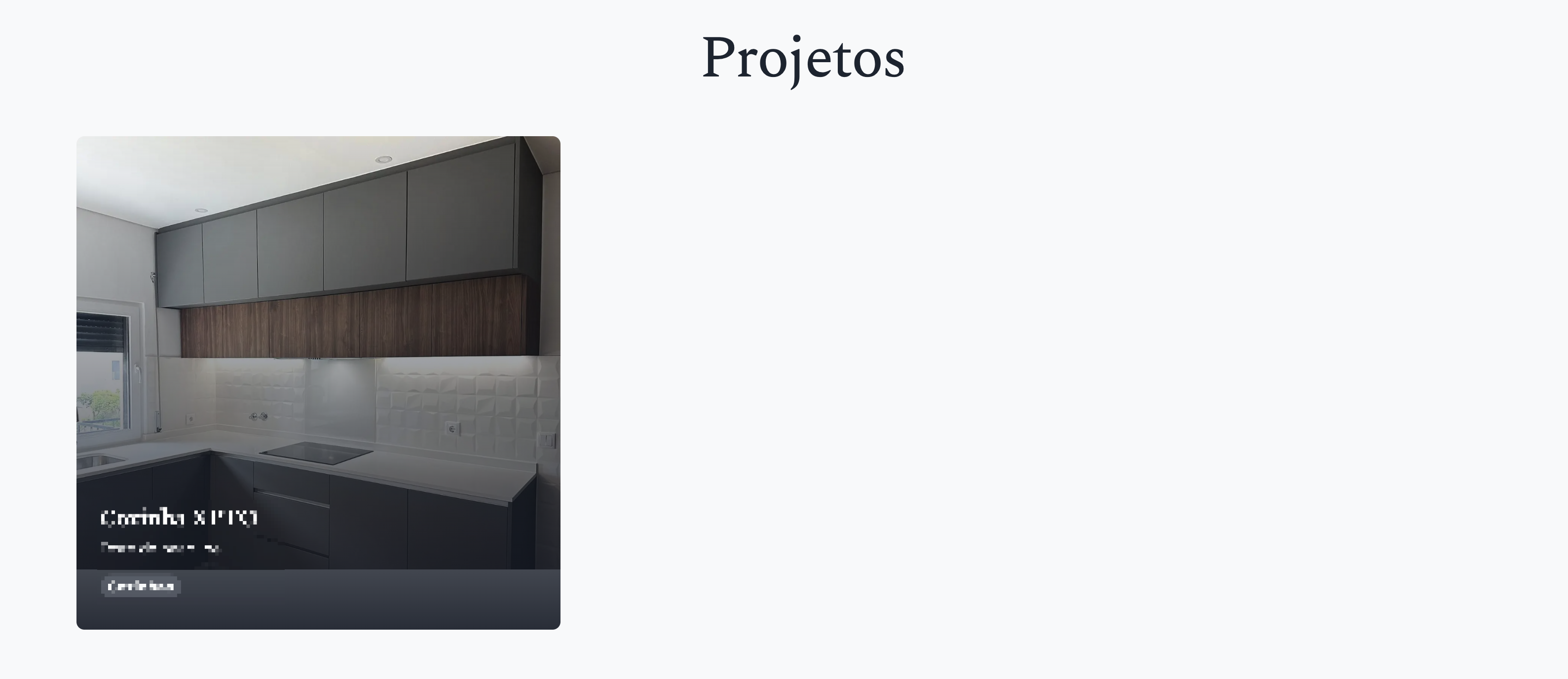
Using a top-level fragment could also be causing it. You'll still want to avoid destructring props though
Try changing the fragment to a div or something
but I'm not destructuring props now
Destructuring props is just a bad practice with Solid, as it can break reactivity
Yeah I understand that, but if you see the code that I send now, I'm using
props.<property>
Yeah, my other suspicion is the top-level fragment
But everytime I refresh the page I have that error
Already try too and the error keeps appearing
Hmm do you have a Suspense in
app.tsx
?Yes
Initially I suspect ti's because I'm trying to do pagination with the
createPagination
primitive (I'm using createAsync
and on the documentation they are using createResource
), but after comment that piece of code the erro keeps happeningfwiw
createAsync
is just createResource
under the hood. What happens if you return some dummy data inside createAsync
instead of calling getProjects
?I think I found the problem
I remove this
<a>
inside the main <a>
of the card and worksOhhh interesting
Yeah i think solid won't usually let you do
<a>
in <a>
, maybe that check isn't being done on the server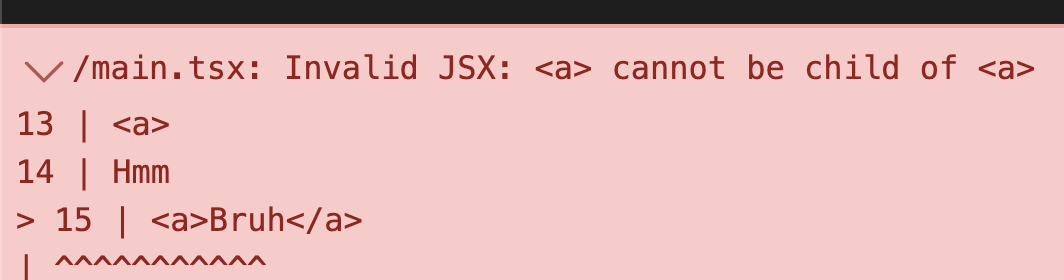
Thanks @Brendonovich
Now only need to figure out how to handle the pagination and put tabs to filter the content
Any suggestion for handle query parameters like page and in this case filters
It’s like a portfolio and I want to filter by service, showing like a tab for each service
I'd just use
useSearchParams
and read/write through itThanks
Also for anyone else finding this through search.
I always use Suspense in components which have a createAsync primitive.
This solves most of the hydration issues.
Additionally you can also use a Show component so that children will only render once the promise has resolved.
tbh the validation is pretty basic. it's probably better to pursue server/client comparison instead.
it could also be worth/easy to just validate the ssr result which should catch cases like this one.
But you add the suspense on the usage or inside the component as first element of the return?
In the same component which has the createAsync.
They’re supposed to work together and this way you don’t suspense at the top of your app all the time. So any of the components which not rely on async data can be mounted right away e.g. layouts.