API integration issue in .NET core framework.
Hello everyone.
I am a beginner of .NET core and I need to integrate other platform inside of .net core project.
I have this other site API docs and have credentials.
I need to request with token on header for this request, but not sure how can I implement this feature.
Many thanks.
72 Replies
HttpClient Class (System.Net.Http)
Provides a class for sending HTTP requests and receiving HTTP responses from a resource identified by a URI.
You can use this class to send http request
public Stream HttpRequest(string url, string token, string method, JObject requestObj)
{
string baseUrl = url;
string finalUrl = baseUrl;
HttpWebRequest httpWebRequest = HttpWebRequest.CreateHttp(finalUrl);
httpWebRequest.Method = method;
httpWebRequest.Headers.Add("Authentication", token);
httpWebRequest.ContentType = "application/json";
using (var streamWriter = new StreamWriter(httpWebRequest.GetRequestStream()))
{
streamWriter.Write(requestObj);
streamWriter.Flush();
streamWriter.Close();
}
WebResponse webResponse = httpWebRequest.GetResponseAsync().Result;
var response = webResponse.GetResponseStream();
return response;
}
@K.F
JObject
? HttpWebRequest
? Tf is this?sample
It's a shit sample
dont mind it
It's doing the opposite of helping
is it wrong @ZZZZZZZZZZZZZZZZZZZZZZZZZ
It's a sample of how to write shit code that uses deprecated APIs
Yes it is
HttpWebRequest has been deprecated long ago
deprecated hahaah yah
sorry
JObject is just getting rid of type safety for no reason
It's some 2000-ass code, the question was about .NET Core
oh my
JObject is old?
It was never necessary to begin with
so what do you suggest
generic type?
And, yes, .NET has a built-in Json serializer too, so Newtonsoft is not needed
A generic would be better, yes
but it is not dynamic
Also, your code uses
.Result
instead of being async
Why the fuck would you want to use dynamicfor easy code
For shit code
hahahha
That's slow and impossible to reason about
really?
oh my
i wish i never met Newtonsoft
Newtonsoft doesn't use
dynamic
far as I know
It was good when .NET had no built-in Json serializer
Now it's just largely unnecessary, and slower than the native serializer
Not inherently bad thoi see. but how do you know its slower?
Benchmarks
oh
Thanks
@ZZZZZZZZZZZZZZZZZZZZZZZZZ
how bout this
private static string HttpPostRequest(string url, string data, string authorization)
{
string finalUrl = url;
HttpRequestMessage httpRequestMessage = new HttpRequestMessage() { };
httpRequestMessage.Content = new StringContent(data, Encoding.UTF8, "application/json");
httpRequestMessage.Method = HttpMethod.Post;
httpRequestMessage.Headers.Add("Authorization", authorization);
httpRequestMessage.RequestUri = new Uri(finalUrl);
HttpClient httpClient = new HttpClient() { Timeout = TimeSpan.FromMinutes(5) };
HttpResponseMessage httpResponseMessage = httpClient.SendAsync(httpRequestMessage).Result;
using (StreamReader reader = new StreamReader(httpResponseMessage.Content.ReadAsStream()))
{
return reader.ReadToEnd();
}
}
Thanks @douchebag , @ZZZZZZZZZZZZZZZZZZZZZZZZZ , @blueberriesiftheywerecats
I will implement with this way and then let you know.
And many thanks @douchebag š
Yeah don't use the code above
what's matter @ZZZZZZZZZZZZZZZZZZZZZZZZZ ?
Not at my PC right now so can't elaborate, but it's not good code
It's using
.Result
so it's blocking, it does a bunch of unnecessary stuff with the request, a bu ch of unnecessary stuff with the response
And in the end produces a strong instead of something more usefulI am getting response from third-party api as JSON.
In this case StreamReader is good choice?
It's unnecessary
Chances are, you can just use
await client.GetFromJsonAsync<T>()
and get a proper object backbasic work flow is like this.
First, get an access token with your credentials.
Second, get data from the API with an authentication request with an authentication token.
But responses are all JSON type and need to send it to frontend side as JSON type.
I'd like to make this as service on project but most important is which structure could be best for this implementation.
I am new .NET and have experience with REST API according to tutorials.
Stack Overflow
Await on a completed task same as task.Result?
I'm currently reading "Concurrency in C# Cookbook" by Stephen Cleary, and I noticed the following technique:
var completedTask = await Task.WhenAny(downloadTask, timeoutTask);
if (completedTas...
var completedTask = await Task.WhenAny(downloadTask, timeoutTask);
if (completedTas...
@K.F change it to await
Make sure you have async function
Yes, I am currently using async function on my side.
Check this one @K.F , there is also request header here
So you're a backend dev. Ic
Ok guys, bye
Yes, as far as I checked HttpClient Class is good choice, I guess.
There is also this library that helps you write meaningful and compact code for requests https://github.com/reactiveui/refit
GitHub
GitHub - reactiveui/refit: The automatic type-safe REST library for...
The automatic type-safe REST library for .NET Core, Xamarin and .NET. Heavily inspired by Square's Retrofit library, Refit turns your REST API into a live interface. - reactiveui/refit
private async Task AuthenticateAsync()
{
using (var httpsClient = new HttpClient())
{
Console.WriteLine( _username);
var response = await httpsClient.PostAsync($"{_uri}/identity/realms/fintatech/protocol/openid-connect/token", new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("username", _username),
new KeyValuePair<string, string>("password", _password),
new KeyValuePair<string, string>("grant_typpe", "password"),
new KeyValuePair<string, string>("client_id", "app-cli")
}));
Console.WriteLine("authentication response", response);
response.EnsureSuccessStatusCode();
var authResponse = JsonConvert.DeserializeObject<AuthResponse>(await response.Content.ReadAsStringAsync());
_accessToken = authResponse.AccessToken;
_refreshToken = authResponse.RefreshToken;
}
}
Here is my approach to get auth token.
With postman, I tested it with my test credential and received tokens but I am getting 400 error with current code.
uri: https://platform.fintacharts.com
username: [email protected]
password: kisfiz-vUnvy9-sopnyv
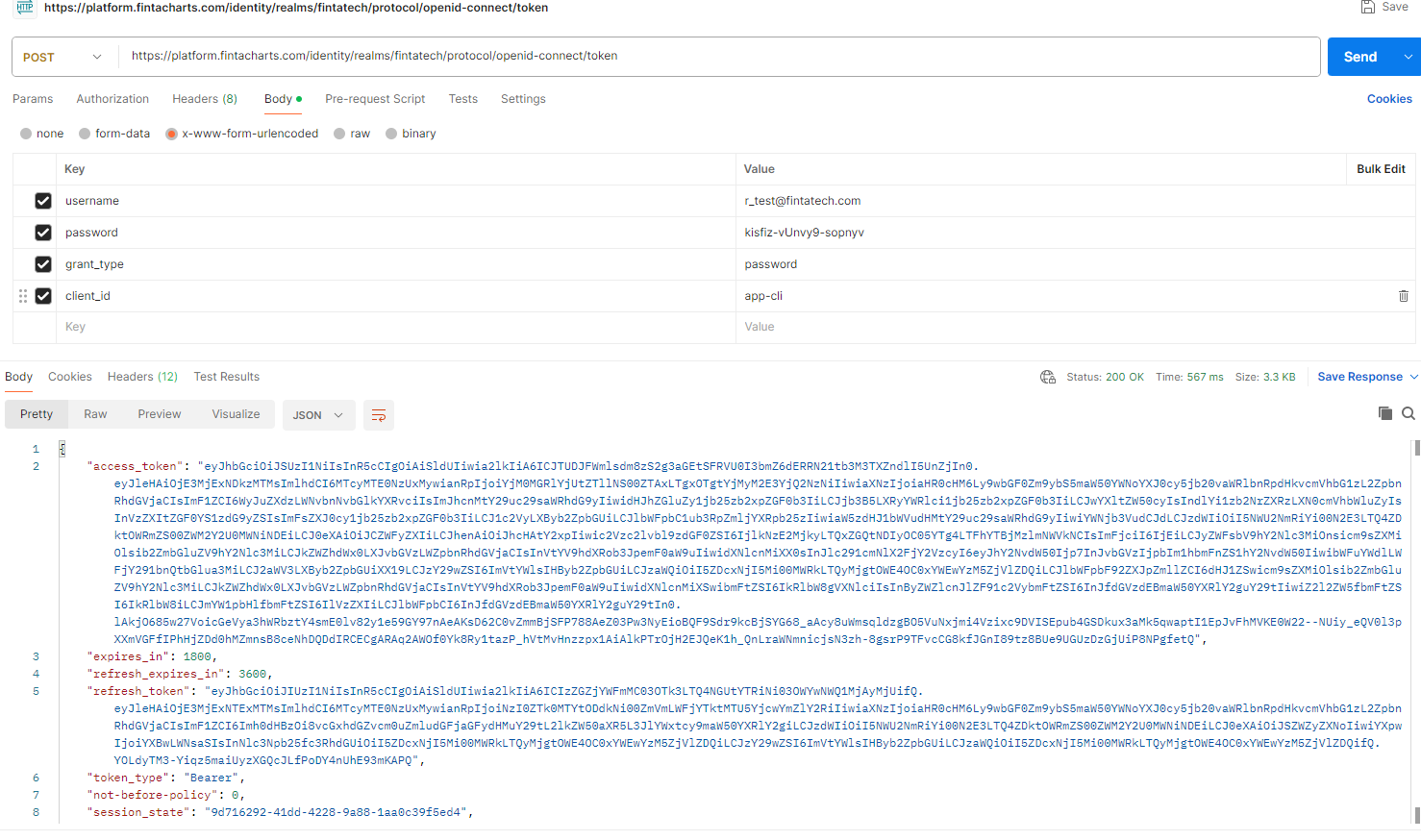
Its 200?
Wym
And also you leaked your password and refresh token so you better change those
Oh you mean with your code its 400
400 means you have wrong request
Check response, there should be problem written
And your uri seems wrong
Okay thanks. let me check again.
I checked console but there is empty
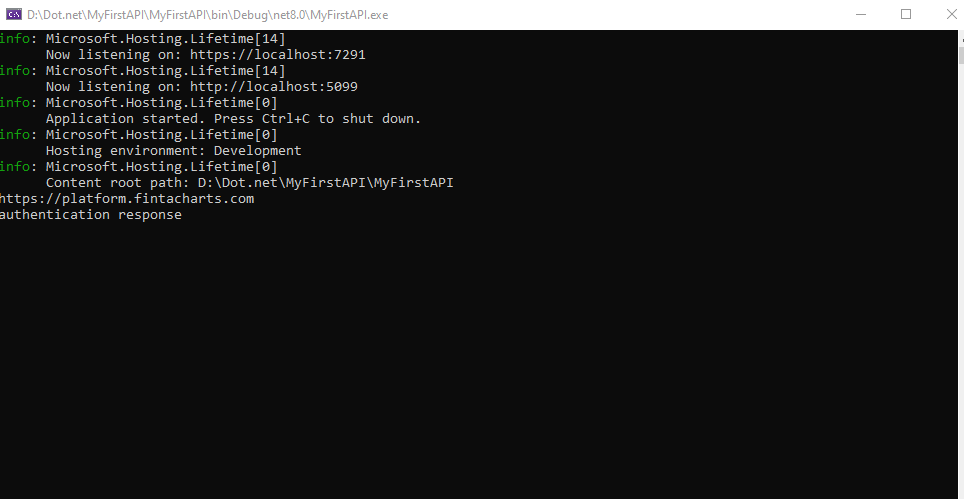
Are you sure you are sending your request to correcut uri?
Oh i see
You misspelled grant_type
@K.F
Awesome to figure out it. š But still not get auth token
But it appears 400 error is disappeared
What is the issue?
authentication response is empty
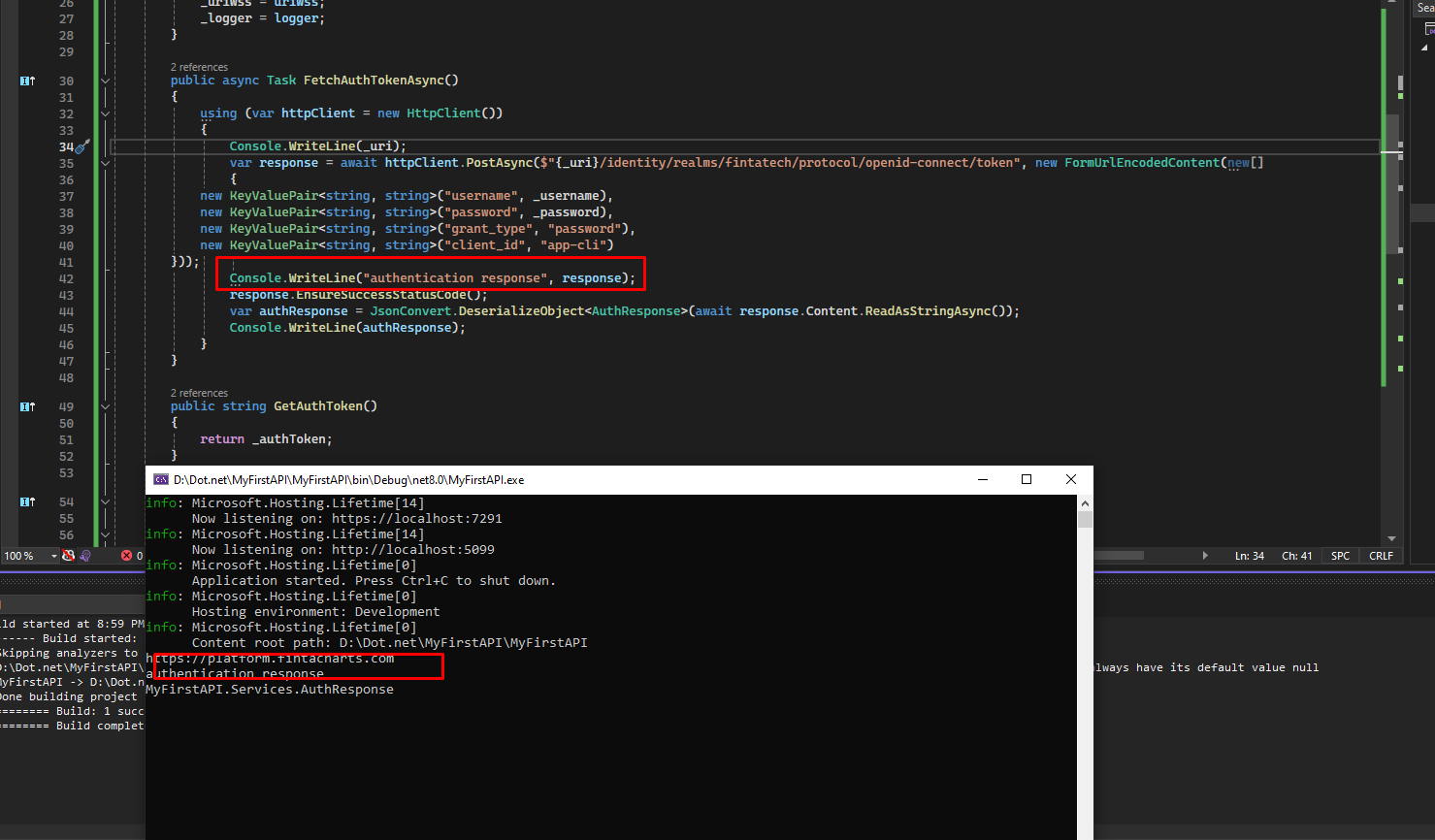
Well, c# cant just convert your object to string, you either override ToString method in AuthResponse or use debugger(better approach)
Im sure you have response
$debug
Tutorial: Debug C# code and inspect data - Visual Studio (Windows)
Learn features of the Visual Studio debugger and how to start the debugger, step through code, and inspect data in a C# application.
Im not sure if type have tostring overrided
So just use debugger and check
well still not getting result.
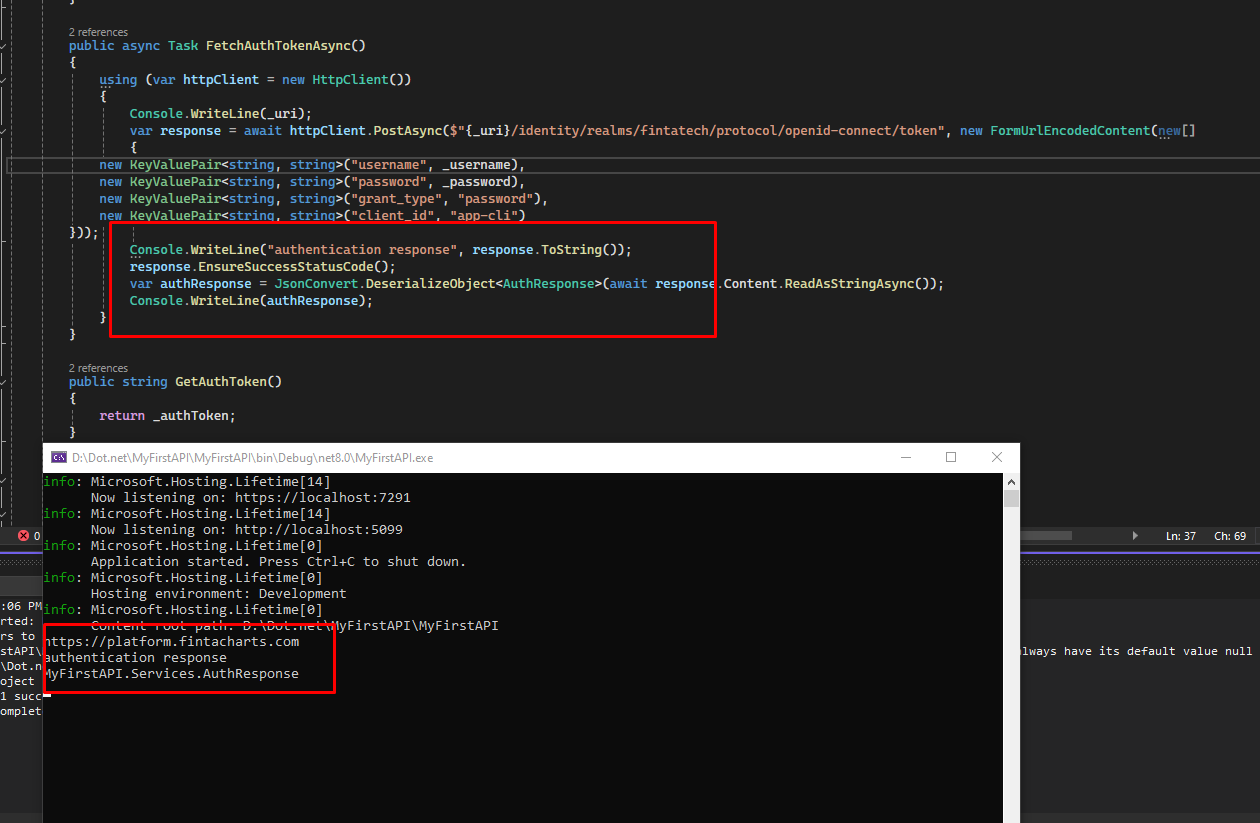
No, Console.WriteLine automatically calls the ToString method, and because its not overrided you wouldnt see anything
Use debugger to see everything in your variables
I am afraid if it is possible to debug for web api
Yes
You just have to put breakpoint before return
And then run debug
I mean before or just on Console.WriteLine(authResponse)
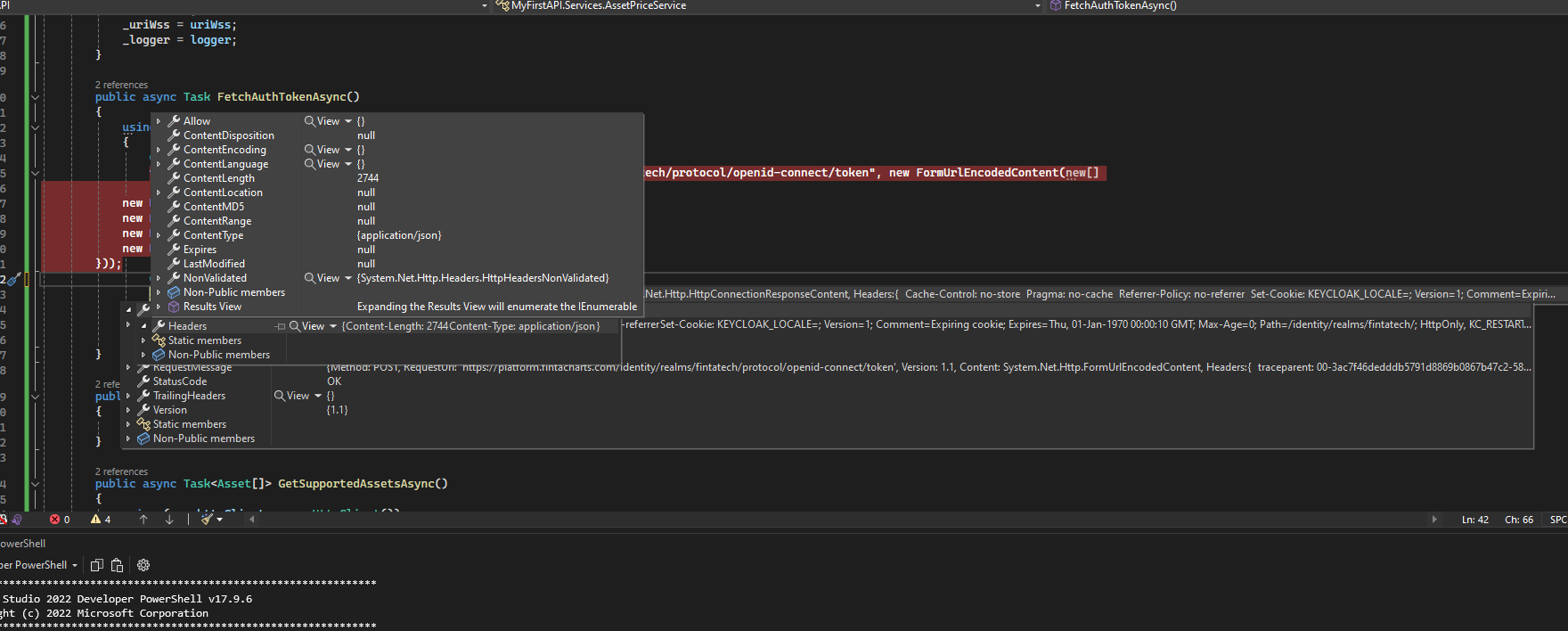
Yes, I am getting nothing as I debugged
Well i see that content length is 2744
Which means there is something
Check authResponse
authResponse is null
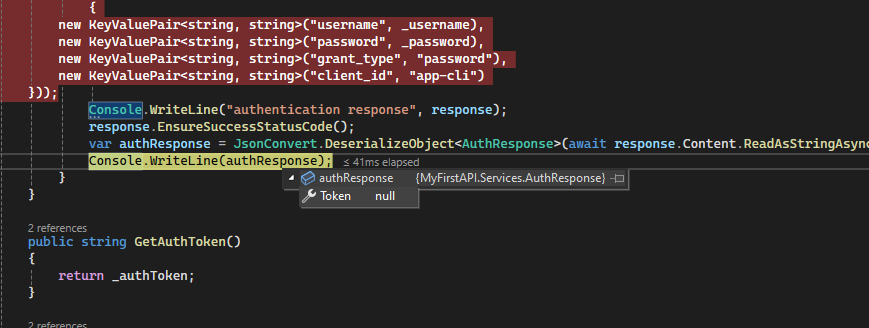
Yes, because it has wrong definition
To be able to deserialize from json your class must have properties that have the same name as json fields
In your case it should be smth like
Or you can use JsonPropertyName attribute if you want to change your properties name in c# but still get the value from json
Fantastic, you are the god of .net. š
It worked
š and how am i still jobless
I can't believe you are jobless now
jorking?
š
seems like yelling me
Lol i think no one wants to accept my resume because im still underage
well, are you still student?
Not yet, i mean i just graduated from school
I see, I can work with you but let me your expectation
JsonConvert
Any reason to use Newtonsoft?JsonSerializer.Deserialize<model>(jsonString);