Message commands help
Hey I started my journey with sapphire today and can't seem to make message command's work
This is my code so far
Slash command's work but message commands doesnt seem to work
Solution:
Jump to solution
Okay I fixed it, the problem was indeed very silly
I found out the code wasn't running after the
canRunInChannel
function, I checked what's the function was doing and it was a perms error
```ts
async canRunInChannel(message) {...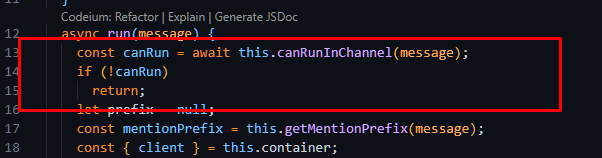
31 Replies
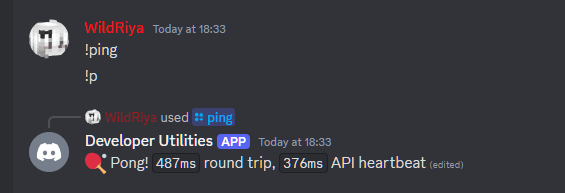
1. Which version of
@sapphire/framework
are you using?
2. What's your file/folder structure?
3. Did you use the CLI to generate your bot?
4. What's your main
(CJS) or module
(ESM) property in package.json
5. Are you using TypeScript? And if so, how are you compiling and running your code? That is to say, what are your build and startup scripts?
- Did you remove your output folder and rebuild then try again?
6. Is your problem related to message commands? Did you add loadMessageCommandListeners
to your SapphireClient
options
Remember that if you are new to @sapphire/framework
it is important that you read the user guide.1, 2, 3, 4, 5 :this:
1.
5.2.1
2. Image attached
3. No
4. "module": "src/index.ts"
5. Am using Bun
6. Yes I did add loadMessageCommandListeners
to my SapphireClient
options
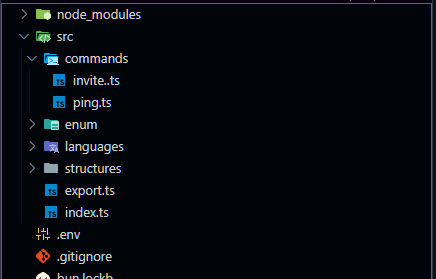
do you also have
"type": "module"
in the package.json?Yes I do
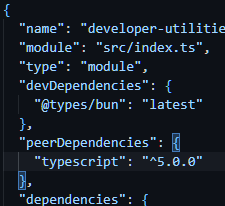
can you compare your own setup to this repo? this is a confirmed working setup https://github.com/sapphiredev/node-bun-example
GitHub
GitHub - sapphiredev/node-bun-example: A bot starting point with bu...
A bot starting point with bun for dev and node for prod - sapphiredev/node-bun-example
Yes my setup is identical
just curious, is the bot receiving message, i.e do you have message intent enabled?
I have enabled intents; the bot will not run unless I have enabled them in the portal and also defined them while defining the
Client
.
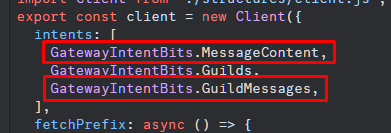
can you try creating a listener to see if your bot is getting those messages
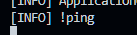
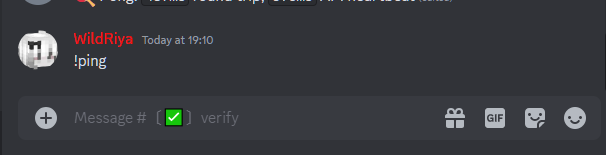
Anymore suggestions?
can i actually see the ping command?
and are you sure the prefix is !
Yes prefix is "!"
to your listener can you add
console.log(this.container.stores.get('commands'))
to see if the command gets loaded at all?
s!ev this.container.stores.get('commands')should give something like that
lemme try
command is loaded
can you do the same for listeners to check that the following are all there:
- CoreMessageCommandAccepted
- CoreMessageCommandTyping
- CoreMessageCreate
- CorePrefixedMessage
- CorePreMessageCommandRun
- CorePreMessageParser
can you can also try logging
this.container.client.fetchPrefix(message)
(with the message being from the listener) to see if that actually returns !
not sure what your problem is. It's probably something silly somewhere. Trying to find out what...
Yes every listener is there
[INFO] CoreInteractionCreate, CorePossibleAutocompleteInteraction, CoreChatInputCommandAccepted, CorePossibleChatInputCommand, CorePreChatInputCommandRun, CoreContextMenuCommandAccepted, CorePossibleContextMenuCommand, CorePreContextMenuCommandRun, CoreChatInputCommandError, CoreCommandApplicationCommandRegistryError, CoreCommandAutocompleteInteractionError, CoreContextMenuCommandError, CoreInteractionHandlerError, CoreInteractionHandlerParseError, CoreListenerError, CoreMessageCommandError, CoreMessageCommandAccepted, CoreMessageCreate, CorePrefixedMessage, CorePreMessageCommandRun, CorePreMessageParser, messageCreate
Prefex exists

okay in that case, can you try to debug to see how far sapphire code executes? Either with console logs or vscode debugger endpoints (the latter will be easier if you know how it works). The code will be in
node_modules/@sapphire/framework/dist/esm/optional-listeners/message-command-listeners
and then the .mjs
files starting with CoreMessageCreate
and from there you should be able to reason which event comes next by what gets emitted.
Some if check somewhere must be going wrong I suspectAlright lemme see
favna you should write a page on the guide on how to use vscode debugger, would help a lot
true tbh, though with the insistance (junior) devs have with using console logs I'm not sure how much it'll help ;-;
many use console.log because they dont understand how debugger works :kekw:
Solution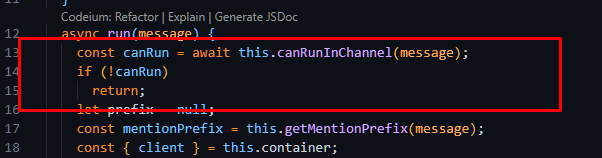
Okay I fixed it, the problem was indeed very silly
I found out the code wasn't running after the
canRunInChannel
function, I checked what's the function was doing and it was a perms error
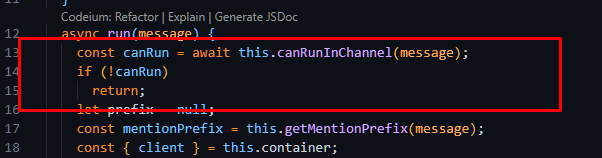
perfect! good to hear