Data from API is displaying in the console but not in the DOM, why?
Hey, I wanna display in the page the data from the api, but for some reasone, it is not be rendering.
api.jsx file
Movies.jsx file component where I wanna display the api data
I am exporting the Movies component in the App as the image you see below...
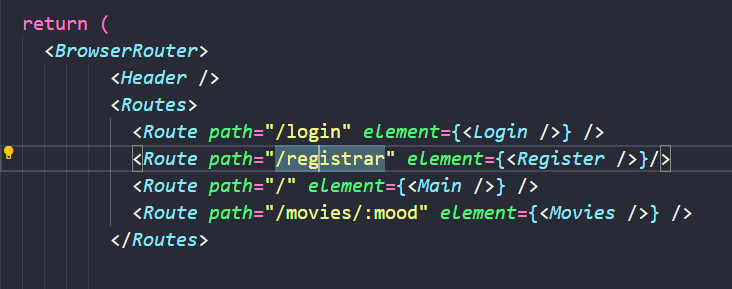
9 Replies
maybe show the reponse data
as well as what actually shows up on the page
here, how data looks like
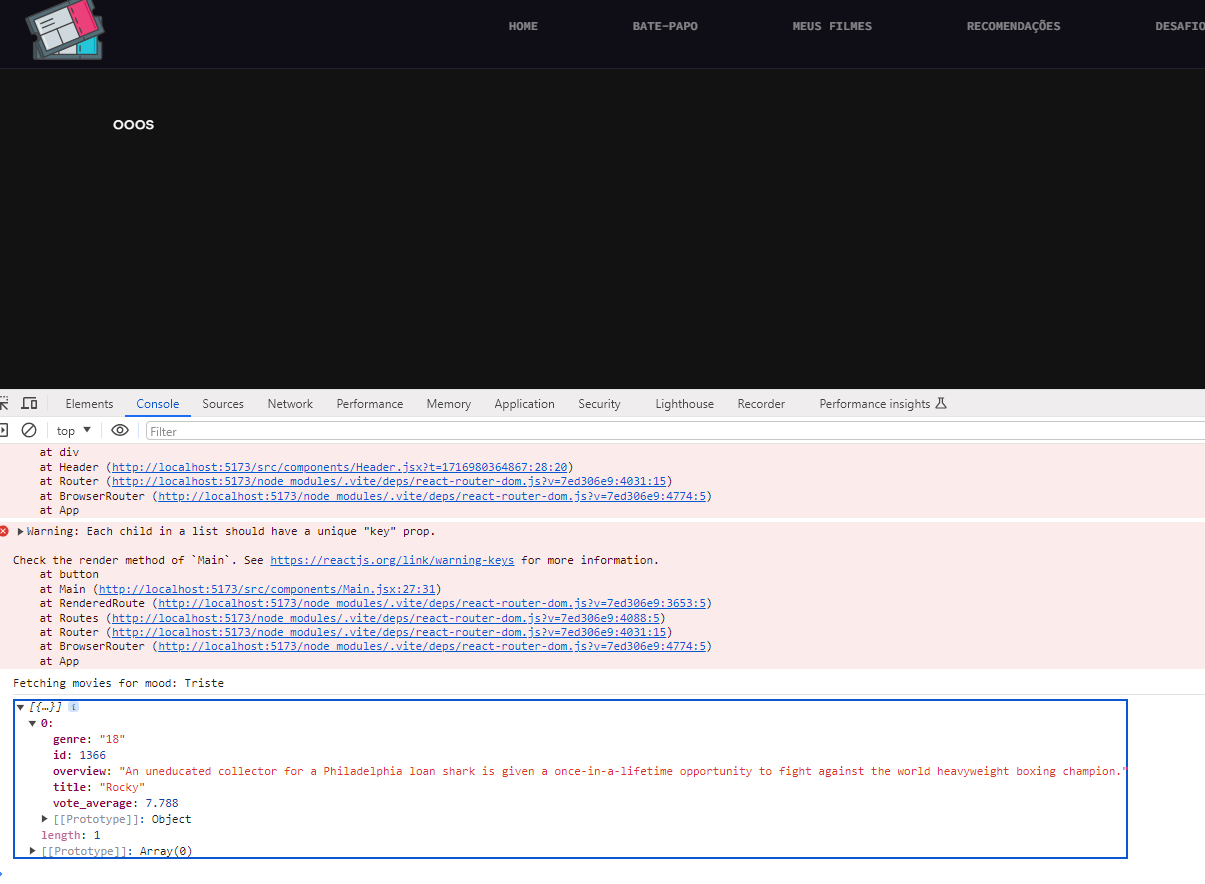
in the html, the ul tag is empty

is the moviesData logging out successfully?

btw u got a try catch block in getMovie() u dont need another one in useEffect
maybe try this idk
i dont see the issue here
nothing is be rendering. And if I reload the page, it is not be calling the api
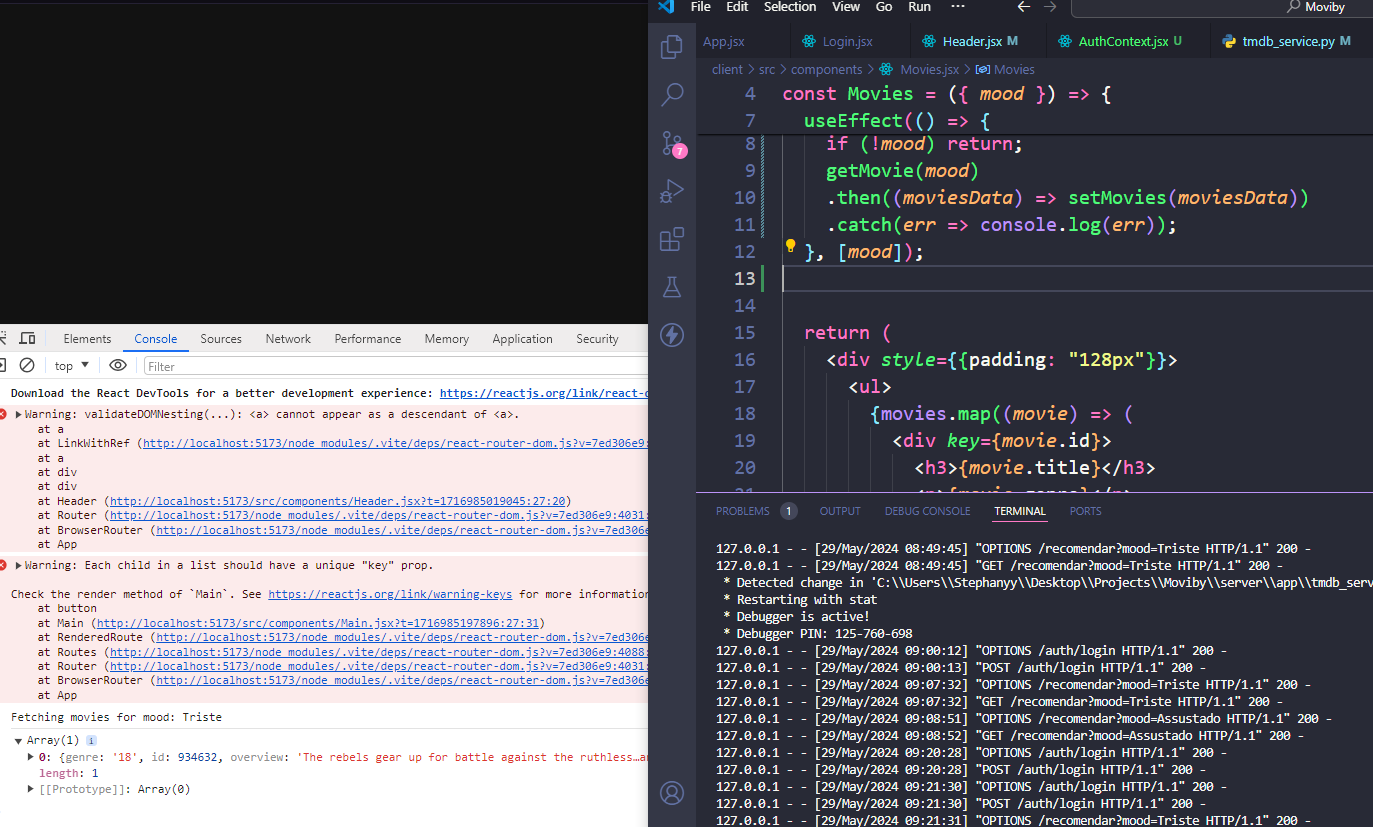
check if the movies array is empty. if it is, dont loop over the movies list. try this. probably not too important, just want to make sure
did it work?
not
try moving your "/" route to the very end of the Routes list
below movies/:mood
also how is it possible to pass mood as a prop and not use useParams? is it a new react feature?