Custom View Form Validation
I have a route that triggers a view that looks like this:
49 Replies
It has a component that looks like this:
But when I submit my form to a controller, I want to display the validation errors, how can I do this?
Why not just have the component implement forms? and not custom it?
Not sure if that would work in my use case.
So the reset password comes from an API call, the way we used to handle reset passwords is now jank, so we have gone the route of just having a custom blade view for it
But instead of a dead styling, im trying to keep filament styling
Why wouldn't it? On save, you can handle all the function calling your api?
I may be getting confused then, could you guide me where to look for info at setting this up?
This is my route
Why are you routing
I thought you had the page rendering already?
It was rendering with the route I had defined
So why have you changed the route?
you would likel yneed to clear your route cache for the new one to work if you created a new one
The docs say to create it that way?
So you absolutely can, but you already had the route? So you just needed to implment the extend
So if I undo back to what I had, it pointed to my controller, that looked like this:
But that cant find the component?
Unable to find component: [reset-password]
Think this is the blade part im getting stuck with though:
@livewire('reset-password', ['token' => request()->token, 'email' => request()->query('email')])
Tried that ^ still not working
Ok I am so confused with what you are doing here.
Just make a normal livewire component:
https://livewire.laravel.com/docs/quickstart
Then implement:
https://filamentphp.com/docs/3.x/forms/adding-a-form-to-a-livewire-component
And problem solved.
Laravel
Quickstart | Laravel
A full-stack framework for Laravel that takes the pain out of building dynamic UIs.
Now the page just hangs, no idea why its trying to claim so much memory:
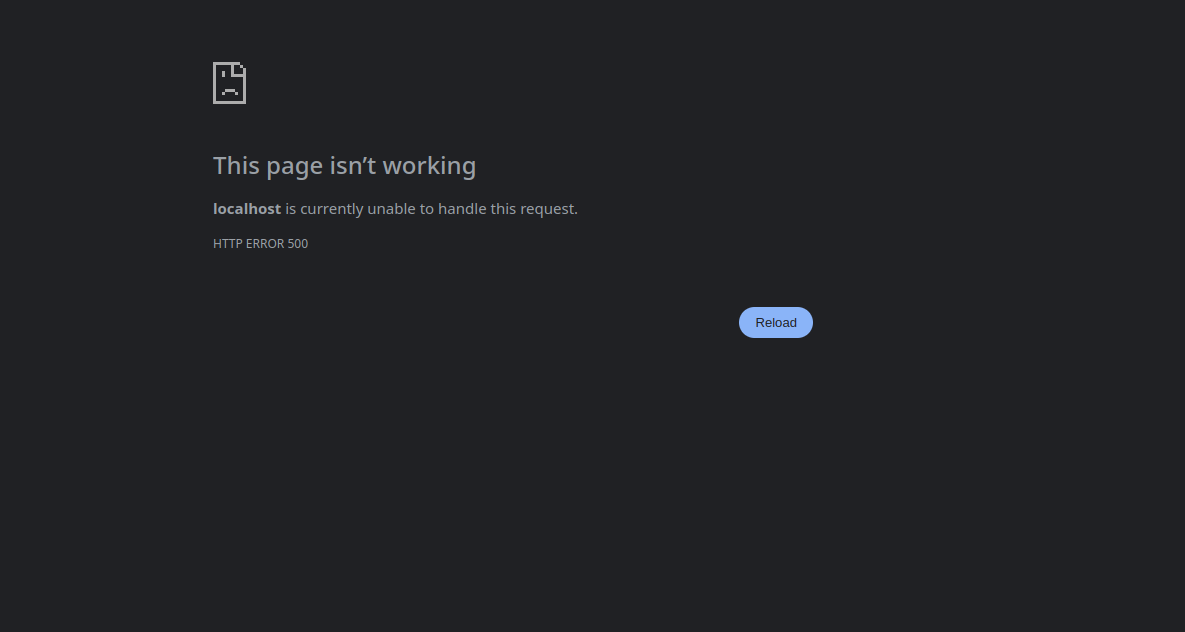
I re-done following this guide
That issue has been fixed, but now I have no styling:
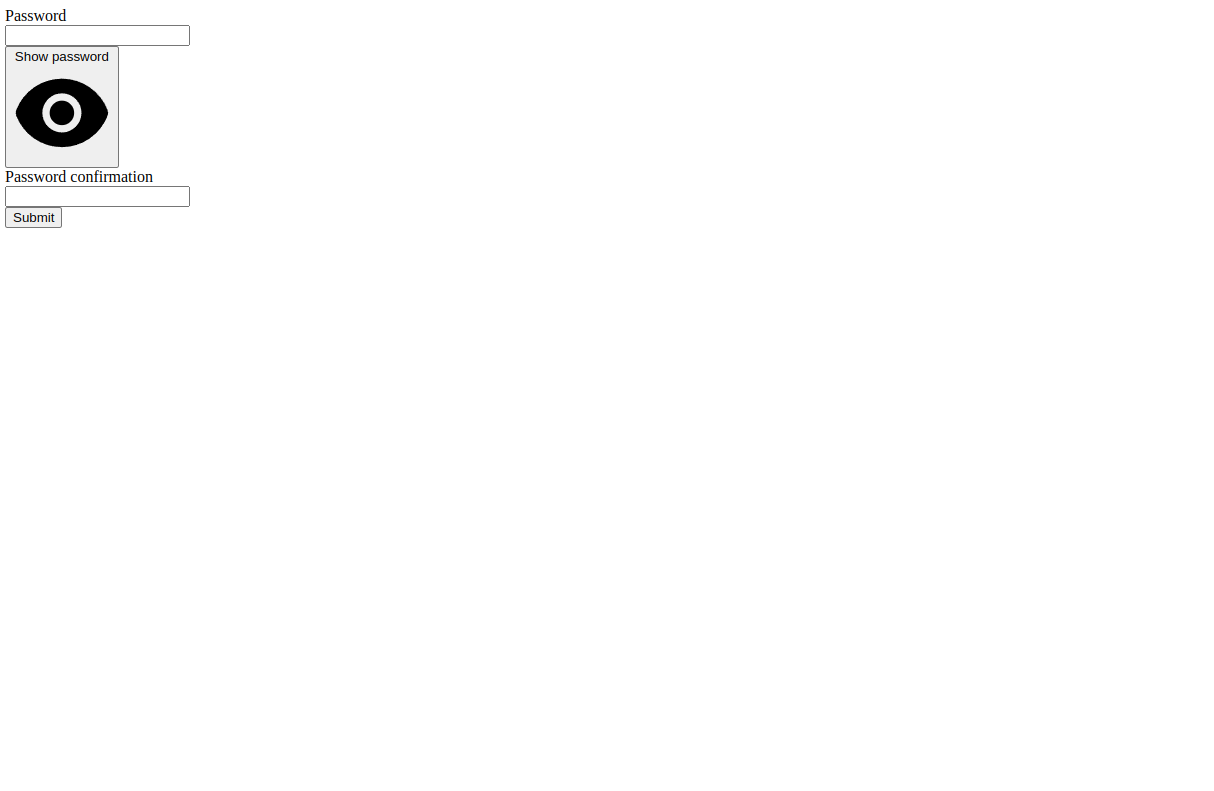
Followed, still no styling.
Trying to run npm run dev just hangs
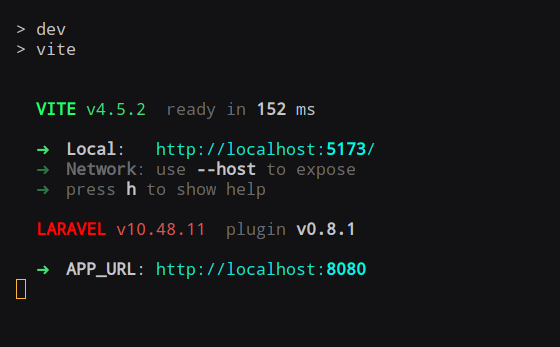
And attempted npm run build returns:
But I've ran the npm install etc that the documentation said to
IF it was followed the assets shoud be loaded for filament forms really...
so it can't find th efilament present... you must have it linked wrong
Literally gone through step by step on every document linked
It generated these js and css files etc, I've uploaded the app.blade.php file as instructed.
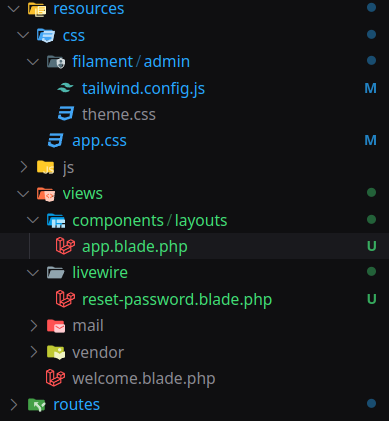
Ok... so in the blade are you rendering any css/js?
I have a feeling you don't have any app styling if it's all been setup correctly
I think its being a bitch around my docker containers
So I have the components/layouts/app.blade.php
By rendered blade view
That looks good, and you are sure the filament systels are loading if you check the network tab
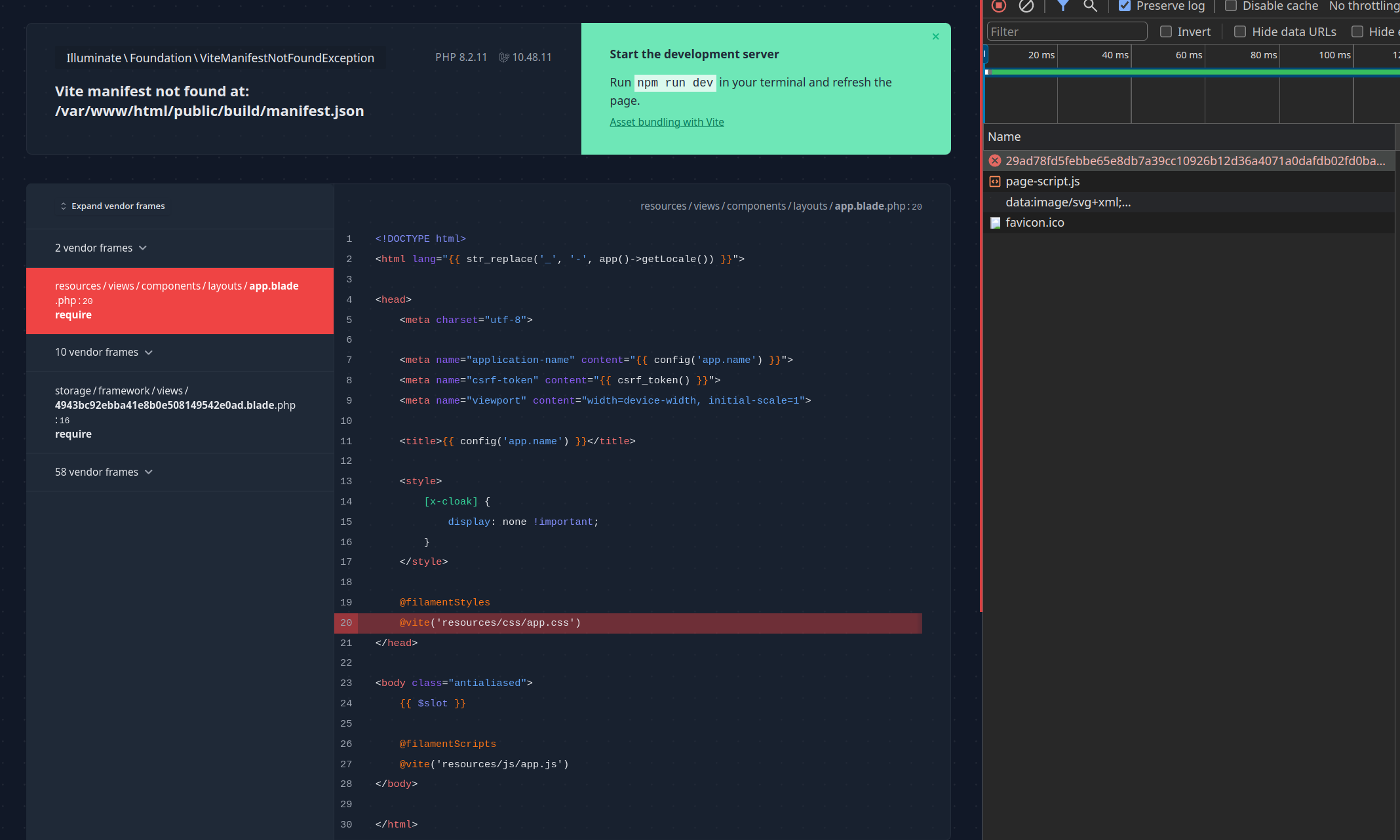
Okay, so npm run build has randomly just worked after failing about 20 times.
But now its blocked by csp, which I think is docker related
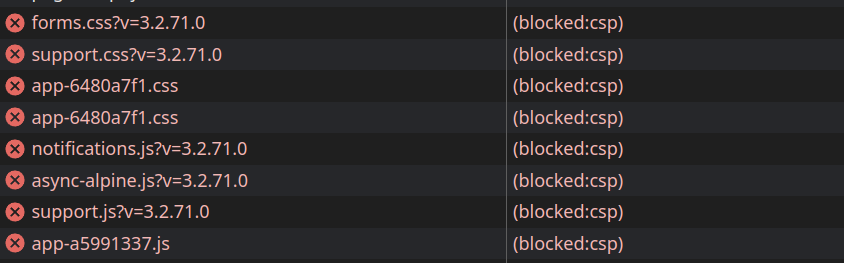
For some reason, isn't setting as localhost either
If its doing all this locally, its gonna be a right ball ache for prod
It is telling you... CSP. You need to ensure the app .env runs the same URL as the url you are accessing it from.
Its running from localhost like the rest of my application?
I've added that port to my docker-compose
This is more effort than it should be like
What is your .env app url?
If you were using Herd it'd be work 😉 But these are basic setup issues of your laravel dev instance
set to http://localhost:8080
I removed asset_url and now it loads them without blocking, but the styling doesn't exist
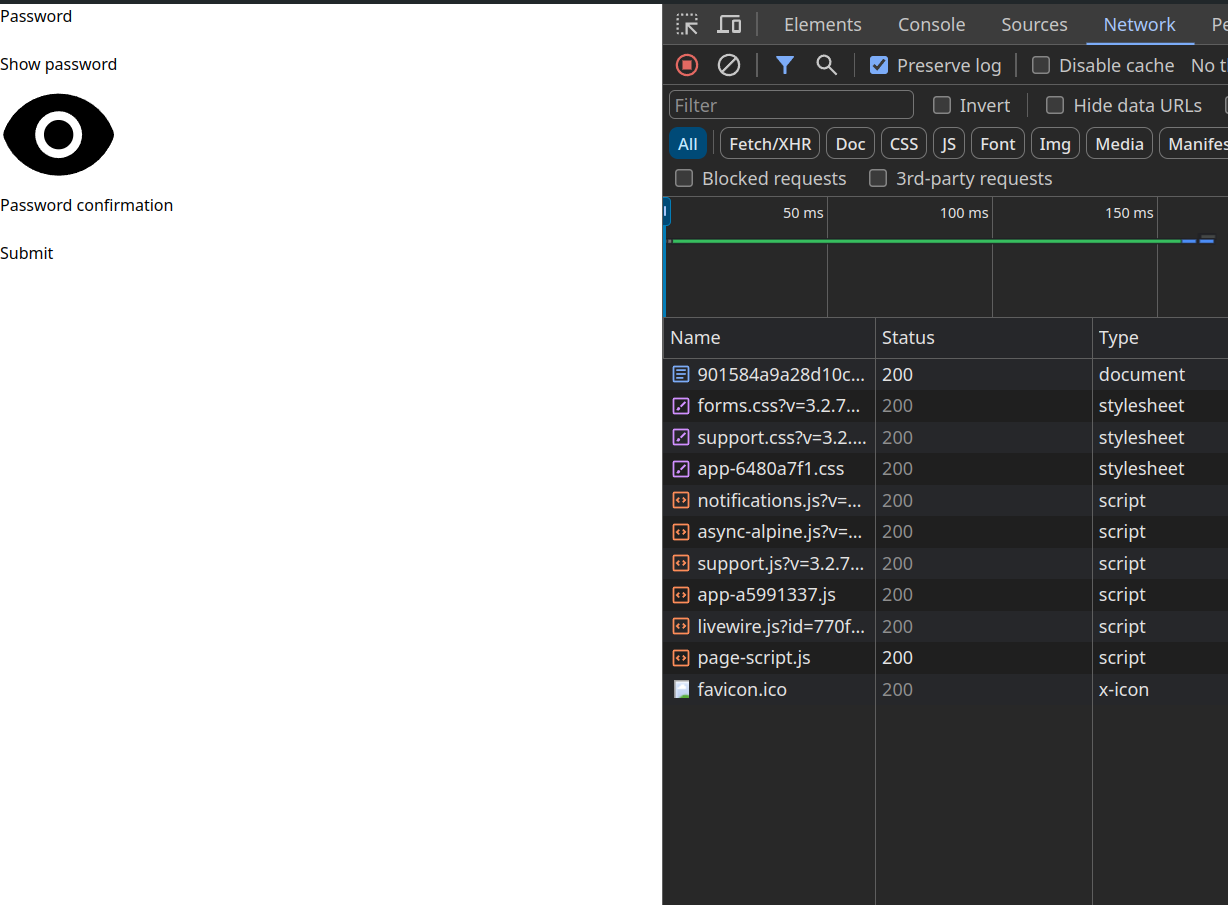
Did you resolve finding the tailwind preset?
That had to change into this:
If thats to pick up livewire.js then yeah, its in the list
if I try to go to localhost:5173, i get connection reset so it doesnt load
Like the styling does look like somethings being picked up, but not in the format other filament forms are
Unless its the theme css thats missing?...
I can see it in my manifest, but not in my network tab
Why not just use Herd for your app? so much easier!
run: npm run build, if it's still not picking it up provide your tailwind config as it'll be missing the inclusion of the filament design
Just not the way work has things setup, Ill mention it to look into
The theme does exist in here, but doesn't appear in the network tab still
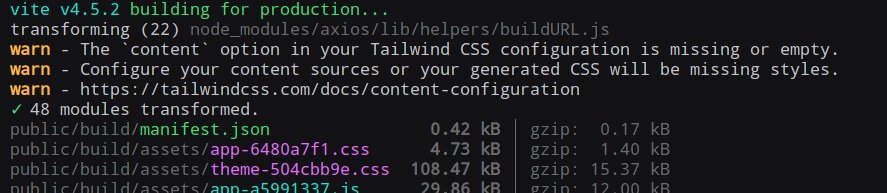
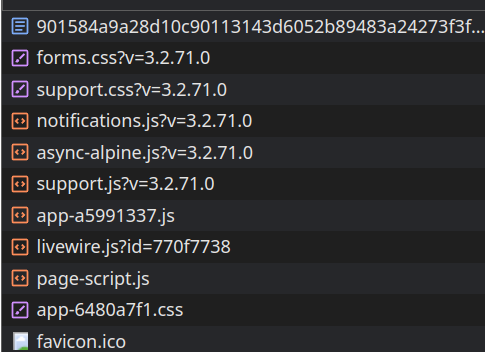
Adding this to my app.blade.php layout file
Has made the styling sort of better. now I need it in this sort of view
This is what I have

But I want it in this kinda layout:
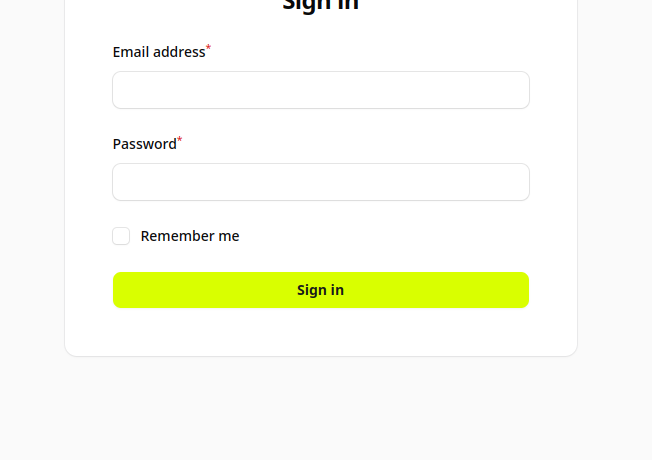
So copy the filament sign page html for that?
Finally working
Instead of extending component, I've gone down extending SimplePage instead
all works
Done and buried
Solution
Thank you for your help @toeknee Appreciate it 🙂
Perfect