Programmatically get columns from table
Hi all I'm wondering if it's possible to programmatically return columns from a table.
For example
In doing so, the result is this image posted below.
Is there a way I can do this programmatically or do I have to reference every value manually?
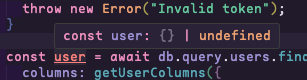
2 Replies
Hello, @NinjaBunny! You can find useful information here
https://orm.drizzle.team/learn/guides/queries/include-or-exclude-columns
Drizzle ORM - Learn
Drizzle ORM is a lightweight and performant TypeScript ORM with developer experience in mind.
I don't think this applies to relational queries as well