Phone Attribute and sendPasswordReset notification voodoo
I have this on my user model, this is super weird. In all cases, the user is created just fine, but if i try modify the phone number, the password reset email fails to send. As mentioned, in all cases, the user is created fine.
Solution:Jump to solution
@wyChoong modified the password reset method to ```php
class CreateUser extends CreateRecord
{
protected static string $resource = UserResource::class;
...
7 Replies
This is what the user creation is like https://gist.github.com/MACscr/89fd2f4ca6a7f132862ea5fd139270ed. I dont think its filament specific of an issue, but I am a bit dumbfounded. $data and $user look complete in the handleRecordCreation() method as well
i really dont know what magic is being used in sendPasswordReset email though. lol.
That sendPasswordReset method seems to send a notification that says "we cant find a user with that email address". This is at the same time it sends a notification that the user was created successfully, which it was. I have a pest test fails to verify that the password reset was sent. If comment it out like i listed above, it passes . 1) all user data looks fine and is created correctly 2) why would this reset notification even care about the phone field and its format?. its just super odd.
the password reset method in the test is just
use Filament\Notifications\Auth\ResetPassword as ResetPasswordNotification;
1. can you test the user create + notifiation without filament/livewire
2. what filament version are you on, could it be DB transaction?
@wyChoong Running latest filament. Now i just noticed in the mutator, if i set the phone number to 554-555-5555, it fails then too. So im thinking it might have something to do with livewire and serialization? I bet if i used a mutator on the 'name', i would run into the same issue
actually messign with name like so didnt cause any problems
though does fail to send the password reset
if the original name was 'bill'
Solution
@wyChoong modified the password reset method to and it does the job
That’s funny
The change was $data to $user only right?
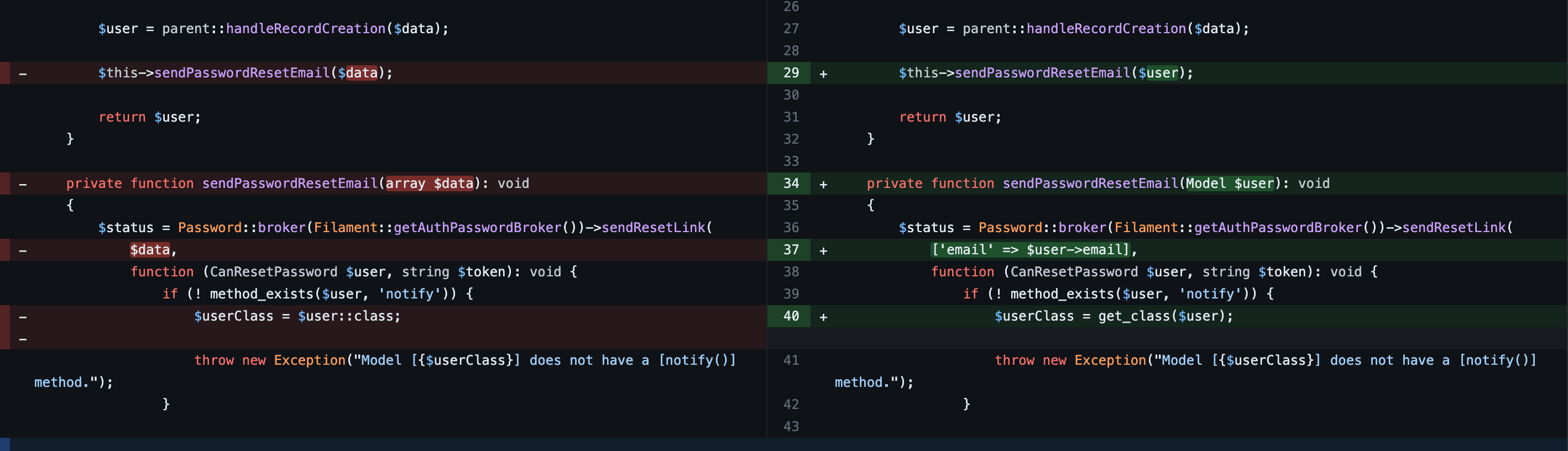
hmm, I thought i had a good explanation from chatgpt why it was causing problems before, but i cant seem to locate it. spent enough time on it already. lol