$fetch catches error, but not able to read body
Hi,
I must be missing something. I'm attempting to get a $fetch error and it's JSON body, but it simply returns 'POST (url) 422 Unprocessable Entity)
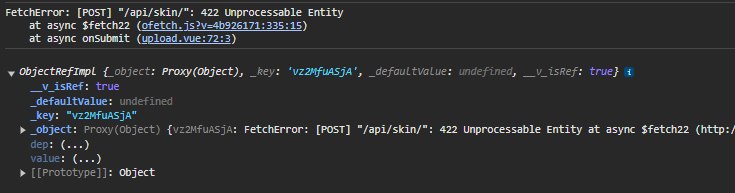
17 Replies
even with useFetch it somehow returns this, and the data maintains empty
within the 'network' tab I can see it just fine
@Kyllian
$fetch
does already to the conversion for your 😉
no need to check for response.ok and to convert to JSON as with the plain fetch apiGitHub
GitHub - unjs/ofetch: 😱 A better fetch API. Works on node, browser ...
😱 A better fetch API. Works on node, browser and workers. - GitHub - unjs/ofetch: 😱 A better fetch API. Works on node, browser and workers.
ah there is an ignoreResponseError, damn
that's so easy, thanks man
no problem 👍
it's so easy you overlook it haha
I have a similar problem, that the body is undefined on the server. In my store, I have the following function:
and on my server route server/api/offices/index.post.ts, i have the function:
However, the body is undefined on the server. What do i miss?
don't destructure 🙂
should be
const body
not const { body }
Its absolutely funny how its always the little things. Thanks!
This answer means it's not possible to get the error object from a $fetch request (you have to use ofetch)? I was hoping I didn't have to install another package for that... My use case is the following: there is no up-to-date package for Campaign Monitor (for Nuxt/Vue/Node) so I created a couple of API endpoints to call CM myself (like this: const response: string = await $fetch(
https://api.createsend.com/api/v3.3/subscribers/${listid}.json
, { method: "POST", body: body, headers: { Authorization: Basic ${authToken}
} });). When an error occurs, I only see "400 Bad Request" and a stacktrace but I'm missing the underlying reason (so I have to use tools like PostMan to check what the actual error is).ofetch and $fetch are the same ☺️
This answer means it's not possible to get the error object from a $fetch requestNo. try/catch it 👍
Use try and catch
I did do the try catch but the error object looks just like a string... Or can I pass "ignoreResponseError: true" as an option to $fetch (TS isn't recognizing it it seems...).
Or read err.data without the ignore option? Because TS also says it does not know .data and I don't really know of which type the error is.
This is the whole thing actually...
Throw the error with createError
Instead of the "Internal error", I would like to "personalize" it according to the error message within the 400 response.
Im on my phone but something like:
Catch(e) {
Throw createError({ status: 400, statusMessage: ‘error msg: ${e}’ })
I don’t have back ticks here. So obviously use back ticks 😂
Sure 🙂 Gonna try to see if I get more details from the error itself...
Not really what I was hoping for 😦 This is what was returned (I put an extra try..catch in my page, calling my own API endpoint):
And this is what I see in Postman (status is 400 Bad Request)...
Just found this online...
Didn't know that was a thing (now I have to find the type of that error :)). Sorry for the question (but maybe the answer can help others).