24 Replies
this code works just fine
I think the
inArray
function from drizzle does that for you
same result
Jan 11, 12:50:21PM error: PostgresError: syntax error at or near "ELSE"
seems to be an issue with the template stringCall
toSQL()
at the end and lets check what's producing
if i remove the
sql
and and the inArray to put it how it is in the OP, then i get this
which looks correct
missing quotes after the Id after "THEN" but same result when i add back
if i copy in the params it works just fine
its gotta be something to do with the ending of the last part bcause if i remove the else clause, it says the syntax error is at or near "END"this also works
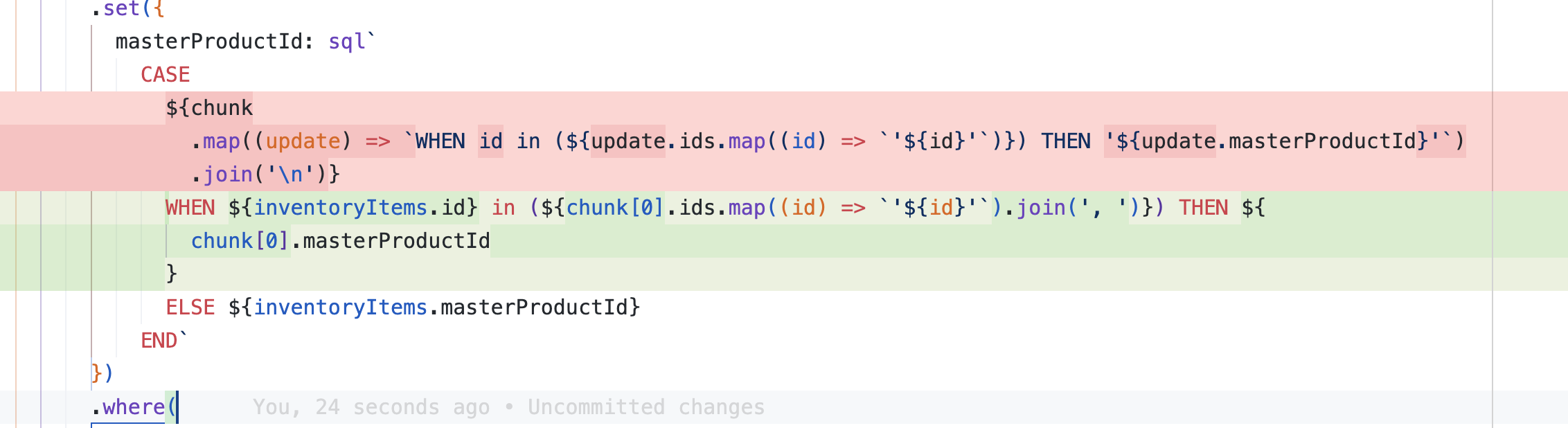
but only for one record
narrowed down the problem to being that it fails when i do the mapping of multiple rows joined with
.join(', ')
i think it might be a bug or something?Man, one sec, I'm confused. What is chunk?
This is wrong
This is wrong too
chunk is a just an array of {ids: string[]; masterProductId: string}
maybe its becuase im trying to do ".join" on a
SQL
object so it stringifies it
how else would you plug in multiple dynamically though
why wrong? when you hardocde in those parameters it works
logged output when i make chunk
of length 1:
looks correct and plugging in that value instead of the ${chunk....} code makes the query work
and again, works without the mapping, even without the sql operatorRemember that drizzle is trying to pass you input as parameters and it makes wrong syntax if you pass it strings with objects like this
more succinctly:
DOES NOT WORK
WORKS
not sure what the distinction is here
I'm kinda don't fully understand your setup or what you're trying to do
But you might need to return sql`` from your map function
yeah the problem is the .join('\n')
how can you build up the interior of the sql string without converting it to a string
fwiw i kinda struggled with something similar today https://discord.com/channels/1043890932593987624/1195077997431107728
ended-up doing stuff like
which looks wrong, but appears to be safe
I'll help you later tonight. If you're still struggling, can you ping me in about 6 hours?
@Hebilicious wrappiong it in sql.raw worked..?
using sql`` does not work
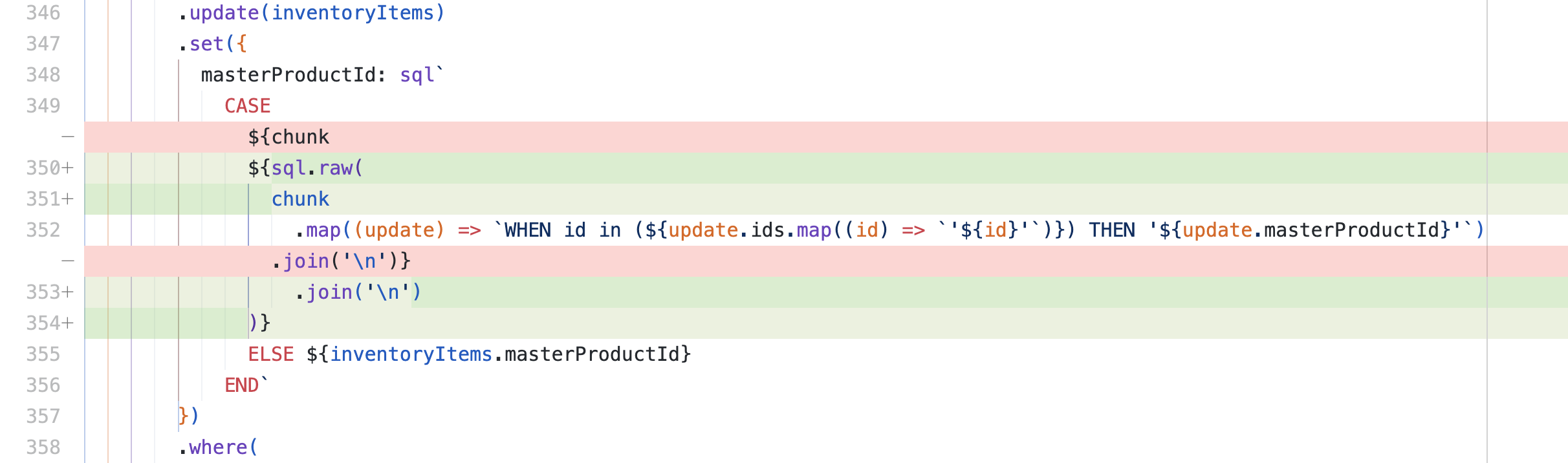
did that solve your issue ?:D
yes
weird behavior though:
i thought those were equivalent? @Angelelz if theyre supposed to be, maybe this is a bug after all
They are not equivalent, raw inlines the arguments in the query, making it susceptible to injection attacks
Let me look into it later and I'll let you know
Did you end up with a working (and perhaps non-susceptible to injections) solution to this? 🙂
no. stuck with the sql.raw answer
weird bc in theory the same code
simplest repro was here
Ait! Thanks for answering. Maybe Angelelz has come up with something, will check back a bit later 🙂
@jakeleventhal
Could this be helpful? Some very preliminary testing from my side, but it does seem to work for my "re-arrange
sort_order
for images in my gallery" use case.
so essentially identical code but
sql.join
instead of .join('\n')
i can try it outthis diff worked for me
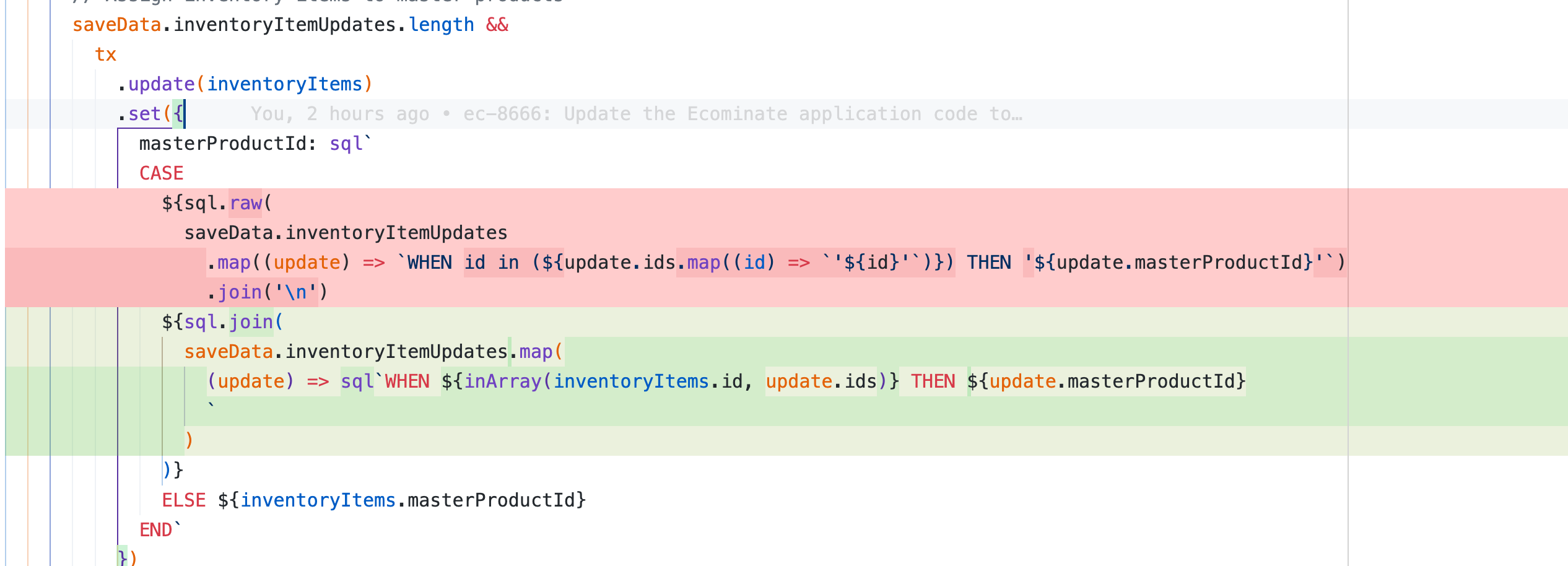