Dynamic component import
Hi there!
Can anyone tell me why this code works:
But not this one:
According to the documentation, I should use resolveComponent but this code snippet doesn't work either:
And same is true for a camelCase formatted component name.
13 Replies
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Ok, I can't "pass the component" because the component depends on the api response.
I moved these components inside the /global directory and it works perfectly 🙂
Thanks for your time
The final code snippet:
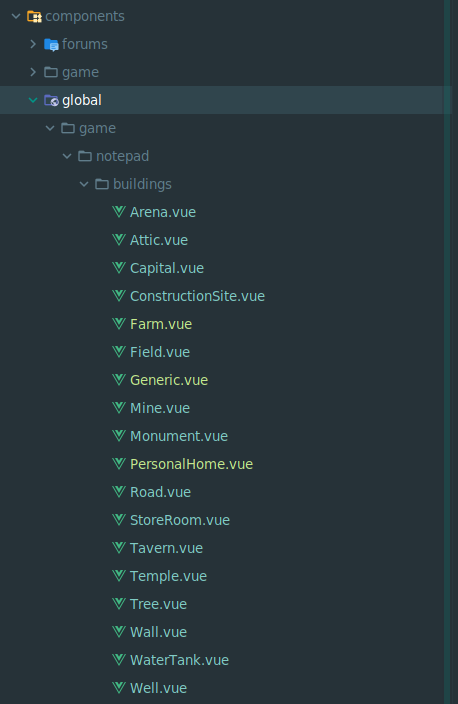
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
@orle
I continued to investigate on the "why this fails":
And surprisingly, it was due to the backtick, you can read more on this here: https://github.com/nuxt/nuxt/discussions/17411#discussioncomment-2568712
Using quotes makes it work but still, I can't get it work with any "dynamic" string:
As of today, I have dozens of different building types, it's a Nuxt2 app that I'm converting to Nuxt3 and previously, it worked perfectly.
So I would avoid a dozens of imports if possible as mentioned in your link
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Nope, the context is a browser game.
I have a "notepad" displayed at left displaying presences on the selected tile, it display the building but also maybe some mobs, characters or resources so the "building" is not a page.
Here is the template code of the TilePresence component which is using the buildings:
What are pages in this context are the "tabs" displayed on the notepad and changing the notepad view (game history, constructions, tile presence, ...)
My temporary working solution is this one but it's ugly as possible 😬
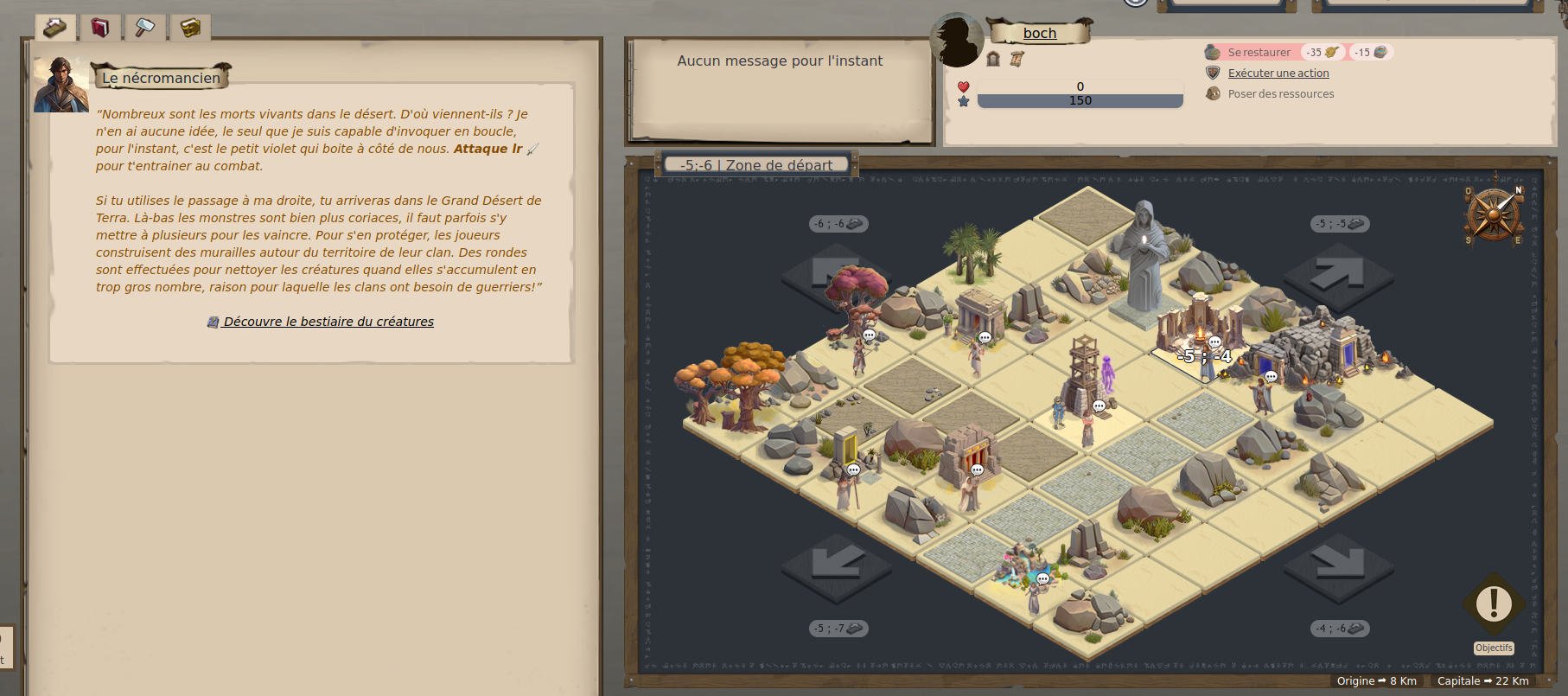
To be shorter, I may use the "Solution 1" of this SO post to not import and then map Building components one by one https://stackoverflow.com/a/72711085/11896411
@orle I make it work with a pretty solution, I post it here for others
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
This way, component are lazy imported and I don't need to maintain a mapping array for the new components 🔥
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
is the same of this? const newComponent = defineAsyncComponent(() => import(
~/components/path/to/component
)); ?
im currently using this, but the anything from the asyncComponent (props) is not beeing initializated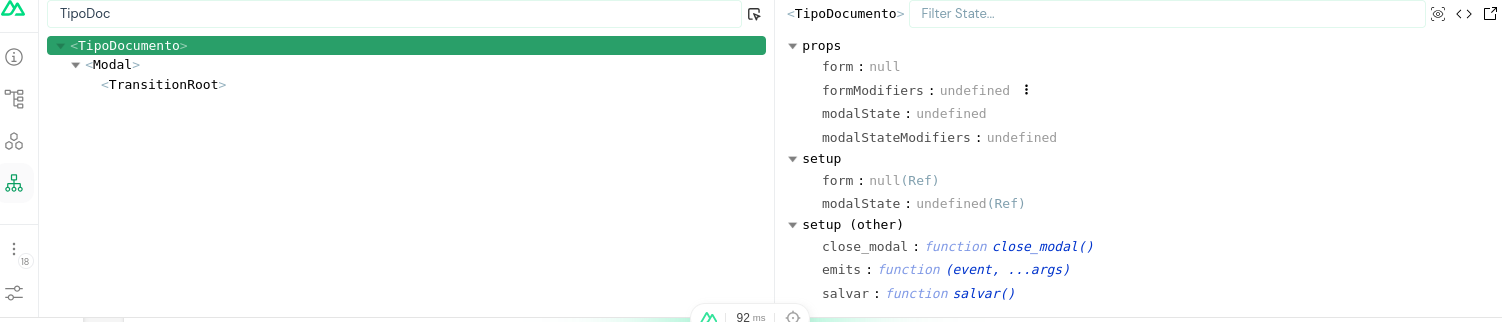
nvm, now is working XD. i just did a rollback and started again, probably mess something up on first time