Save an array state on a Custom Field
I'm trying to integrate a Google map drawing field that can draw polylines.
However, I can't figure out a way to save the data once the
polylinecomplete
event is triggered.
I can now draw the polylines (like the image attached).
My state consist of:
This is the code of my field view
Can you please shed some light?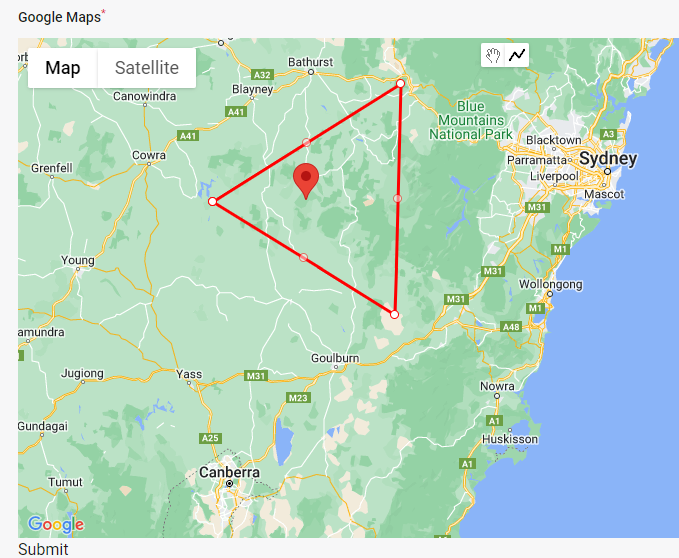
8 Replies
I would say to look at how #google-maps-location-picker is configured, essentially you'll need to trigger back a setter method
Tried it but no luck. There is no reactivity when an array is passed.
try:
@this.set('field', value);
here's the update
weird, so submitting the form carry out the additional polylines, but the
json_encode
didn't update while I draw themI think the issue here is that you're updating key inside state that's an array, i don't think alpine deeply watches the state (when entragled) (but i could be wrong)
anyhow, you can use the same aproach i've used in #saade-adjacency-list,
https://github.com/search?q=repo%3Asaade%2Ffilament-adjacency-list%20builder%3A%3Asort&type=code
I don't know if it'll help, but I do similar things in my #google-maps plugin, saving drawing layer JSON to a field on the form.
This gets done in drawingModified(), which is called from the various addOverlayEvents() cases.
https://github.com/cheesegrits/filament-google-maps/blob/main/resources/js/filament-google-maps.js#L714
GitHub
filament-google-maps/resources/js/filament-google-maps.js at main ·...
Google Maps package for Filament PHP. Contribute to cheesegrits/filament-google-maps development by creating an account on GitHub.
@Hugh Messenger , I've implemented your way. Question though, if I need just the coordinates, I just need to update the
statePath
?
Or the whole config array gets updated? Sorry, totally newb.I dunno, I'd have to see all your code and understand exactly what you are trying to do.