helpertext hide after selecting other select field
Why does the helper text for the "bank" field disappear when I choose the "expense type"?
Code
public static function form(Form $form): Form
{
return $form
->schema([
Textarea::make('detail')->maxLength(350)->required(),
DatePicker::make('date')
->format('Y-m-d')
->default(date('Y-m-d'))
->required(),
Forms\Components\TextInput::make('amount')
->numeric()
->inputMode('decimal')
->required(),
Select::make('expense_type')
->searchable()
->options([
'Operating Expense' => Account::where('account_type', Account::OPERATING_EXPENSE)->pluck('name', 'id')->toArray(),
'Direct Expense' => Account::where('account_type', Account::DIRECT_EXPENSE)->pluck('name', 'id')->toArray(),
'Overhead Expense' => Account::where('account_type', Account::OVERHEAD_EXPENSE)->pluck('name', 'id')->toArray(),
'Other Expense' => Account::where('account_type', Account::OTHER_EXPENSE)->pluck('name', 'id')->toArray(),
]),
Select::make('bank')
->searchable()
->options(
Account::where('account_type', Account::BANK)->pluck('name', 'id')->toArray()
)->afterStateUpdated(function (?string $state, ?Component $component) {
$balance = number_format((Account::find($state)->currentBalance(Carbon::yesterday(), Carbon::tomorrow()))[1], 2);
$component->hint("Current balance of account : {$balance}");
$component->hintColor($balance < 0 ? 'danger' : 'primary');
}),
]);
}
public static function form(Form $form): Form
{
return $form
->schema([
Textarea::make('detail')->maxLength(350)->required(),
DatePicker::make('date')
->format('Y-m-d')
->default(date('Y-m-d'))
->required(),
Forms\Components\TextInput::make('amount')
->numeric()
->inputMode('decimal')
->required(),
Select::make('expense_type')
->searchable()
->options([
'Operating Expense' => Account::where('account_type', Account::OPERATING_EXPENSE)->pluck('name', 'id')->toArray(),
'Direct Expense' => Account::where('account_type', Account::DIRECT_EXPENSE)->pluck('name', 'id')->toArray(),
'Overhead Expense' => Account::where('account_type', Account::OVERHEAD_EXPENSE)->pluck('name', 'id')->toArray(),
'Other Expense' => Account::where('account_type', Account::OTHER_EXPENSE)->pluck('name', 'id')->toArray(),
]),
Select::make('bank')
->searchable()
->options(
Account::where('account_type', Account::BANK)->pluck('name', 'id')->toArray()
)->afterStateUpdated(function (?string $state, ?Component $component) {
$balance = number_format((Account::find($state)->currentBalance(Carbon::yesterday(), Carbon::tomorrow()))[1], 2);
$component->hint("Current balance of account : {$balance}");
$component->hintColor($balance < 0 ? 'danger' : 'primary');
}),
]);
}
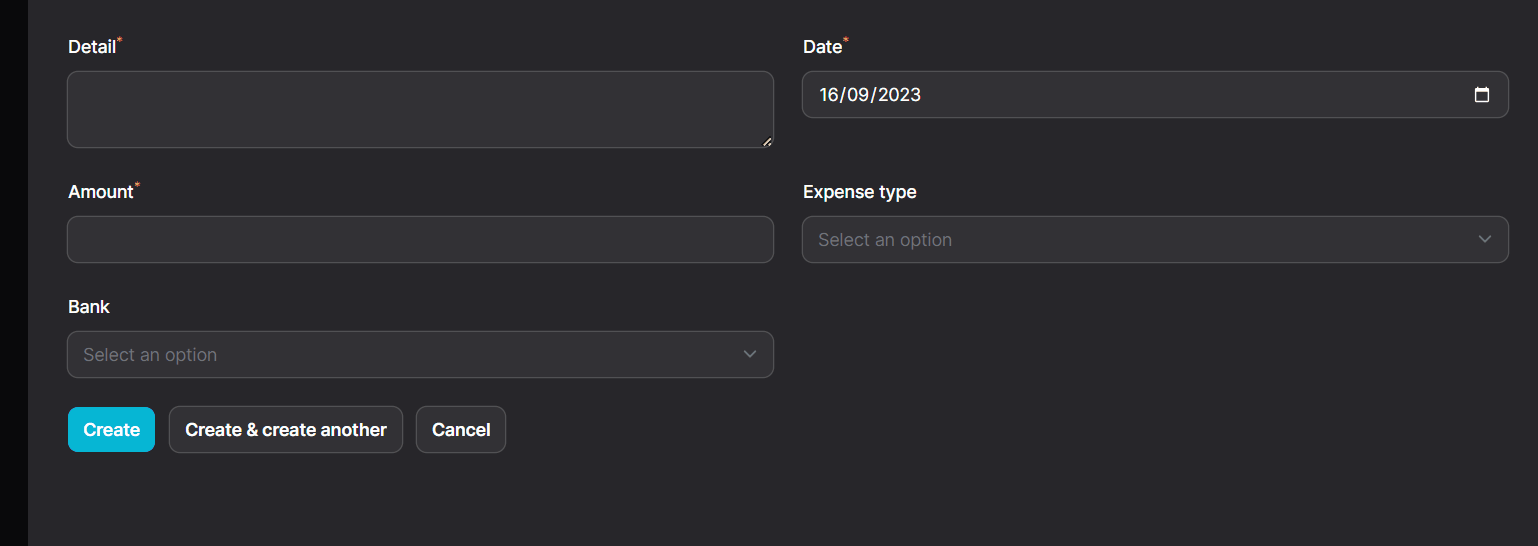
9 Replies
🧐
I think you need to move
hint()
and hintColor()
out of afterStateUpdated()
Select::make('bank')
->searchable()
->options(
Account::where('account_type', Account::BANK)->pluck('name', 'id')->toArray()
)
->hint(fn () => ...)
->hintColor(fn () => ...),
Select::make('bank')
->searchable()
->options(
Account::where('account_type', Account::BANK)->pluck('name', 'id')->toArray()
)
->hint(fn () => ...)
->hintColor(fn () => ...),
How are we supposed to obtain the balance variable in these functions?
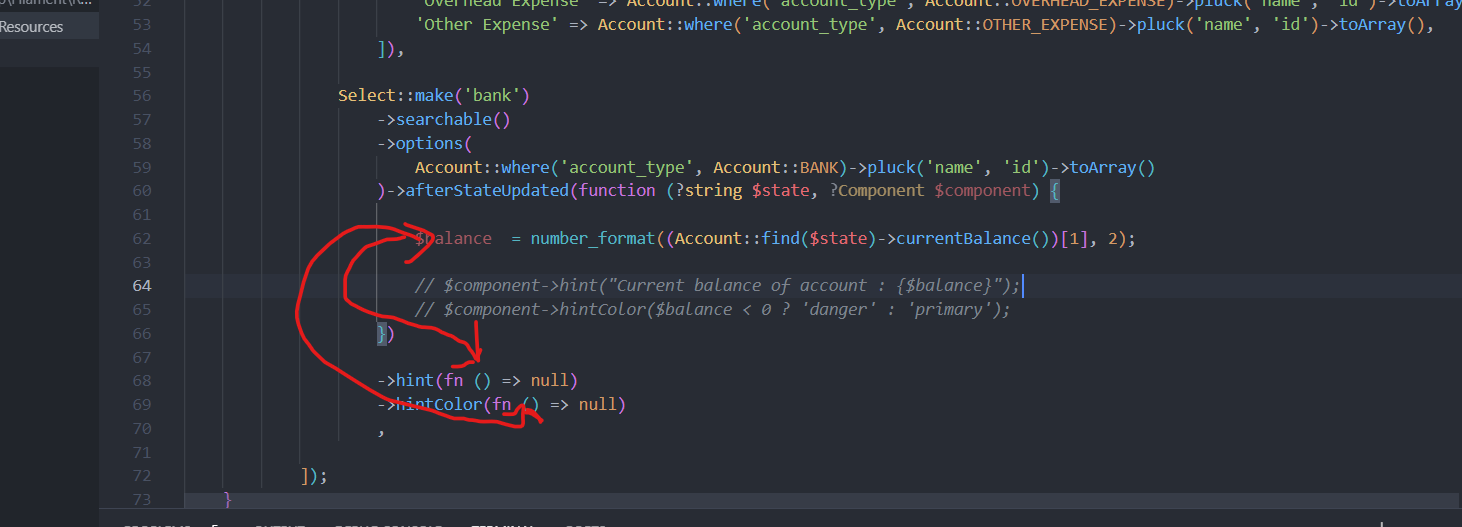
declare the variable before the
return $form
?Not Working 😢
public static function form(Form $form): Form
{
$hint = "";
return $form
->schema([
Textarea::make('detail')->maxLength(350)->required(),
DatePicker::make('date')
->format('Y-m-d')
->default(date('Y-m-d'))
->required(),
Forms\Components\TextInput::make('amount')
->numeric()
->inputMode('decimal')
->required(),
Select::make('expense_type')
->searchable()
->options([
'Operating Expense' => Account::where('account_type', Account::OPERATING_EXPENSE)->pluck('name', 'id')->toArray(),
'Direct Expense' => Account::where('account_type', Account::DIRECT_EXPENSE)->pluck('name', 'id')->toArray(),
'Overhead Expense' => Account::where('account_type', Account::OVERHEAD_EXPENSE)->pluck('name', 'id')->toArray(),
'Other Expense' => Account::where('account_type', Account::OTHER_EXPENSE)->pluck('name', 'id')->toArray(),
]),
Select::make('bank')
->searchable()
->options(
Account::where('account_type', Account::BANK)->pluck('name', 'id')->toArray()
)->afterStateUpdated(function (?string $state, ?Component $component) use ($hint) {
$balance = number_format((Account::find($state)->currentBalance())[1], 2);
$hint = "Current balance of account : {$balance}";
})
->hint($hint),
]);
}
public static function form(Form $form): Form
{
$hint = "";
return $form
->schema([
Textarea::make('detail')->maxLength(350)->required(),
DatePicker::make('date')
->format('Y-m-d')
->default(date('Y-m-d'))
->required(),
Forms\Components\TextInput::make('amount')
->numeric()
->inputMode('decimal')
->required(),
Select::make('expense_type')
->searchable()
->options([
'Operating Expense' => Account::where('account_type', Account::OPERATING_EXPENSE)->pluck('name', 'id')->toArray(),
'Direct Expense' => Account::where('account_type', Account::DIRECT_EXPENSE)->pluck('name', 'id')->toArray(),
'Overhead Expense' => Account::where('account_type', Account::OVERHEAD_EXPENSE)->pluck('name', 'id')->toArray(),
'Other Expense' => Account::where('account_type', Account::OTHER_EXPENSE)->pluck('name', 'id')->toArray(),
]),
Select::make('bank')
->searchable()
->options(
Account::where('account_type', Account::BANK)->pluck('name', 'id')->toArray()
)->afterStateUpdated(function (?string $state, ?Component $component) use ($hint) {
$balance = number_format((Account::find($state)->currentBalance())[1], 2);
$hint = "Current balance of account : {$balance}";
})
->hint($hint),
]);
}
declare a
public $hint
in your component, and use $this->hint
Using $this when not in object context
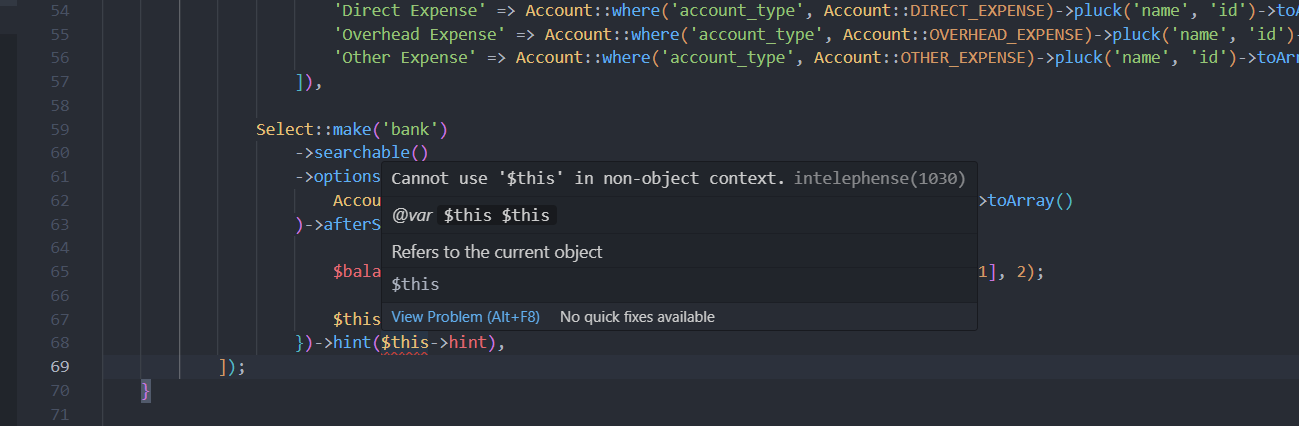
Same as you did before I think:
->hint(function ($state) {
$balance = number_format((Account::find($state)->currentBalance(Carbon::yesterday(), Carbon::tomorrow()))[1], 2);
return "Current balance of account : {$balance}";
})
->hint(function ($state) {
$balance = number_format((Account::find($state)->currentBalance(Carbon::yesterday(), Carbon::tomorrow()))[1], 2);
return "Current balance of account : {$balance}";
})
Thank you; your suggestion worked for me.