implementing generics with crud and classes
I am trying to implement a base class, that could be overwritten/extended with the help of generics, but I am running into an issue with my create function, where I kinda get blown into generics hell the more I look at the type error. If anyone could help or just tell me that creating something like this is impossible please let me know haha.
The issue I'm running into is in the create method where the values I'm passing in aren't happy with what it expects even though the
Insert
type is the inferred Insert of that specific table. Which doesn't make sense because update works as expected
Any help/suggestions will be greatly appreciated. Thank you in advance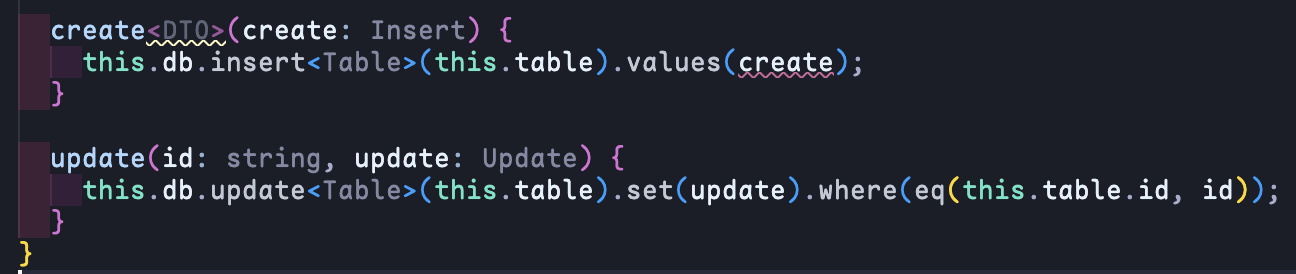
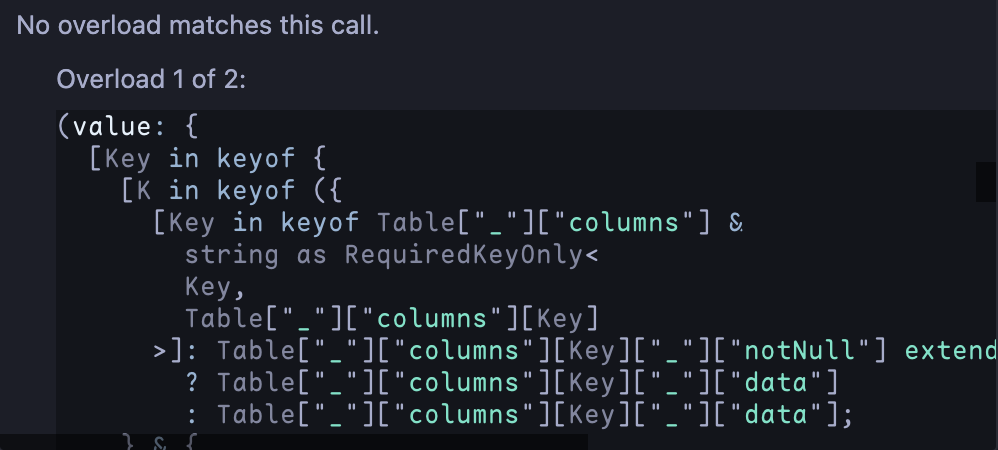
1 Reply
the type error is very long, if it's required I'll send the entire type haha
found this by just scrolling down a bit https://discord.com/channels/1043890932593987624/1147439990683488268