Deployment and logging issues (Maven)
Hello,
My program is running fine locally, but when I deploy it with Railway, I start seeing issues. I think the issue is primarily with grabbing the environment or database variable.
The reason I think it's some database/environment issue is because the logs on Railway says:
So it would appear that this part from main function around the JDA part is having issues:
I have double checked that the TOKEN variable is the same locally and on the Postgres database, so the issue is not the token itself.
I can't seem to figure out what is wrong. And also, how can I use the deploy log to my advantage for debugging? Locally, sometimes i'll just do System.out.println to check variables, etc., but this does not show up in the deploy logs.
72 Replies
Project ID:
e202dece-bdd8-491e-810f-9d28bf4c36e6
e202dece-bdd8-491e-810f-9d28bf4c36e6
Just realized I can use
for both dev and production, and once again, everything works fine locally, so something is going on with System.getenv on Railway
show us your service variables please
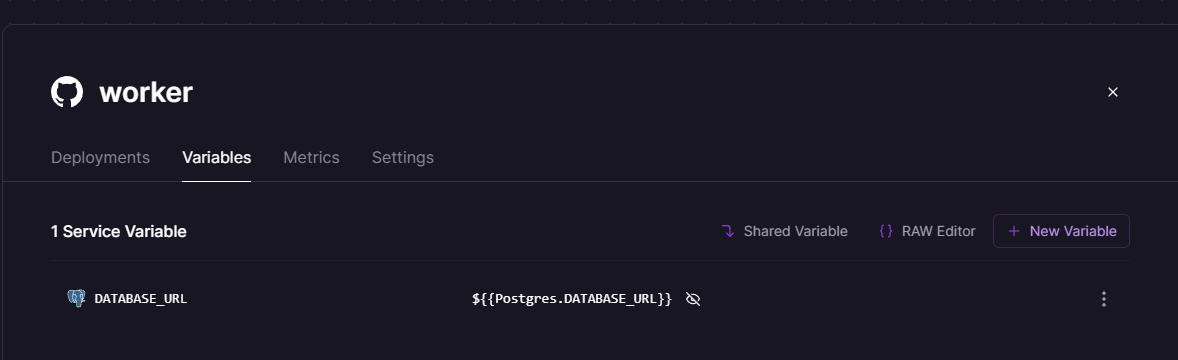
Are you Sure this is a correct url?
On some line in your code you check if the string starts with postgres://
Last I checked the one on your picture doesn't seem like it starts with that.
my undersatanding is that
System.getenv("DATABASE_URL") returns ${{Postgres.DATABASE_URL}}, which is pointing to this no?
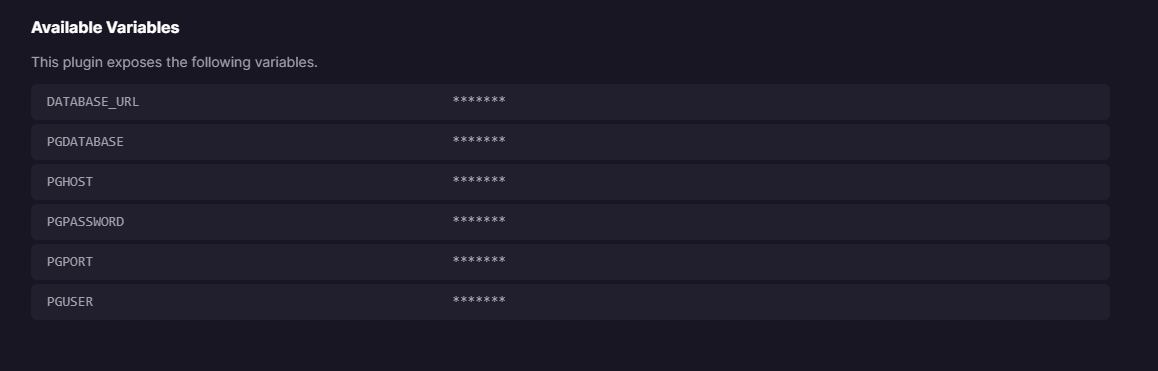

and given this, it does indeed start with postgresql://
unless my understnding is wrong
It seems like you have double postgres:// in the beginning but it might be wrong from my part
Oh you have a worker and then a seperate service where you define the database.
I think you might need to use shared variables?
interesting, could you explain this? it's my first time on railway
Scratch that. Seems like it would still work the way you have it.
https://docs.railway.app/develop/variables#reference-variables
hi, did you make sure you can connect to your databse and that it's returning something? If it was the environment variable, Java would thrown a connection exception.
You could also set your token in an environment variable to make sure that it's a database connection issue
All I can say for sure is that when I run it locally, without changing the code at all, it all works fine. So it seems that it is possible to connect to the database and retrieve/set things as needed. But once I deploy it to Railway, that's when the ssues pop up
i was just thinking abt trying this
also, instead of parsing the connection string, you can separately set the credentials, make the code a lot cleaner.
you are close, that is called a reference variable, it references the postgres plugins own
DATABASE_URL
variable, but while you see ${{Postgres.DATABASE_URL}}
in the railway website, railway will replace that with the DATABASE_URL
connection string from the database plugin when running your app
do a console log of this please System.getenv("DATABASE_URL")
however, you said you logs are not making it into the deployment logs, so make sure you are logging to stdout