Conditional findFirst
Is it possible to conditionally use findFirst, rather than having to chain the underlying model.
E.g.
Into either:
17 Replies
I'm not sure I quite understand what you're trying to acomplish. Do you want the table you're querying to be dynamic? You definitely do the second one. You can actually get your model types with
I'm not sure if that's what you're looking for.
I believe that should solve it thank you! We've just created a wrapper shorthand for some of the consistent query types we do, so we're just looking at adding things like this so that we can streamline our development.
Thanks for the snippet. Im trying to achieve similar result as Aidan, but using your example I couldn't get it to work.
It's showing "type { ... RelationalQueryBuilder<MySql2PreparedQueryHKT, Extract.... } cannot be used as an index type"
did you find a solution to this? I'm trying to implement the same thing, but I get that error as well
No I didn’t 😞
If you did find out please let me know!
I'll see if I can solve this solutiona nd share my findings
drizzle have several type helpers for this type of stuff
Can you show a code snippet of what you're trying to accomplish?
however when I hover over model, it is of type readonly ... table, and
this.db.query
will no accept a readonly type
this is the iceberg of the error
and it just spits out the entire table
I was thinking of doing this in the instantiation of the class like this
This is very similar to this other question. He implemented this exact thing https://discordapp.com/channels/1043890932593987624/1148695514821435443
I think if you type
private readonly name: typeof db.query
, you should be good to goI don't think this is the right thread seems like a different issue
also no typing it as private readonly doesn't work because the query itself won't work
ahhh I saw that one, but the issue in particular is coming from the this.db.query, which is something that thread/other person had not implemented yet, and I was trying to implement that specific aspect
Can you try typing name like this
private readonly name: keyof typeof db.query
I think I already tried that and ran into a similar issue that being this error blob which I have no idea what it means
Same thing here, did someone find a solution ?
a simple reproduction, get the TS error with two tables
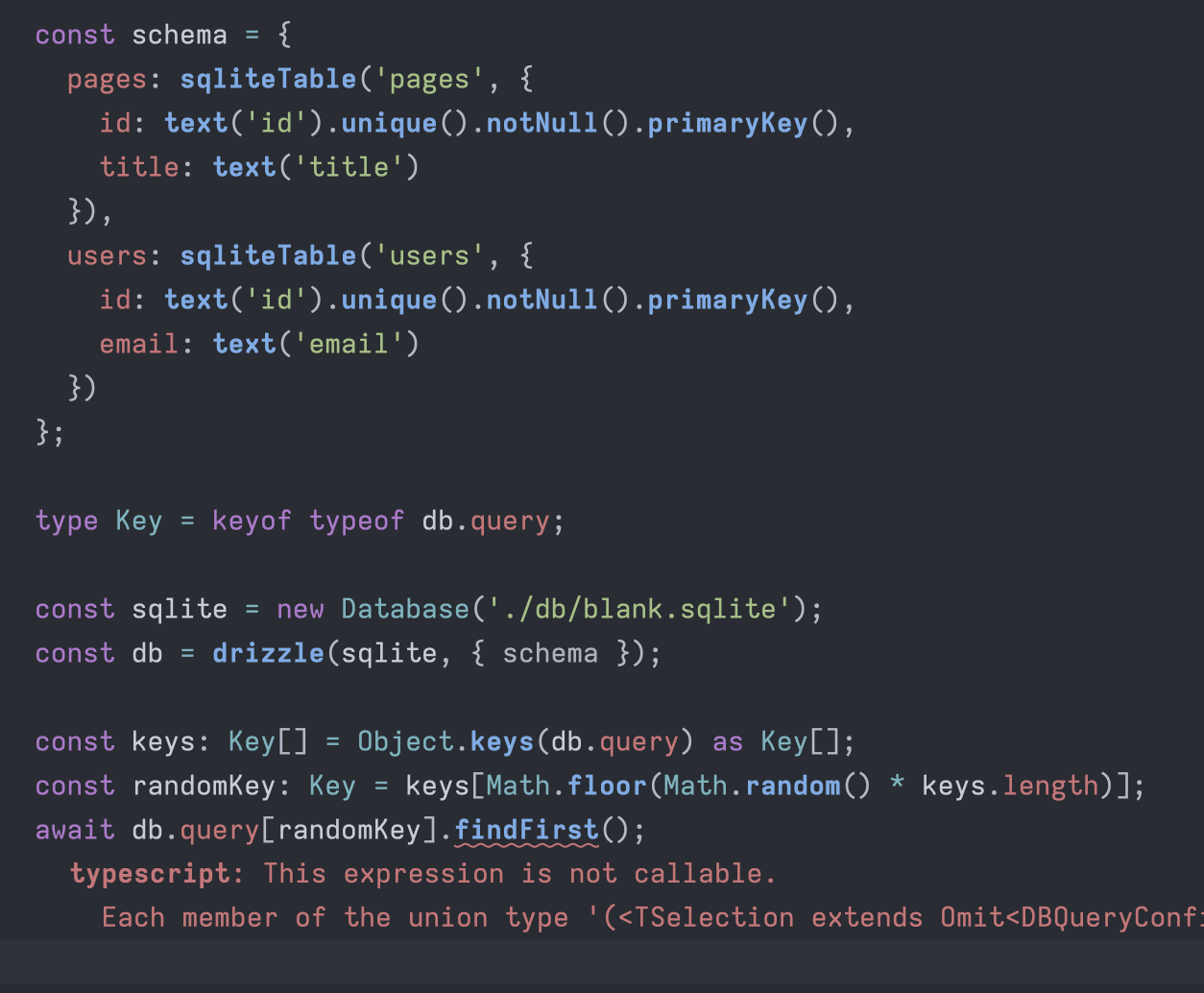
No error with one table only
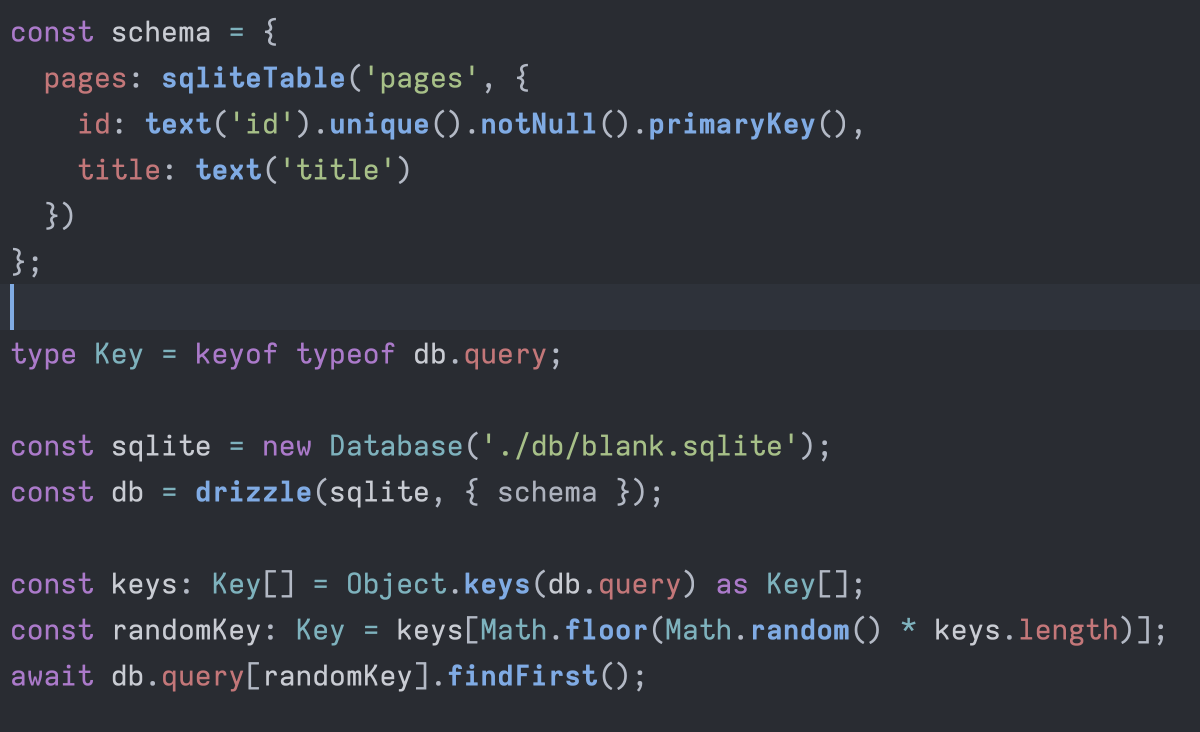