Missing required parameter for edit route redirect after creation error
I created a model EmploymentAnnouncement:
class EmploymentAnnouncement extends Pivot { use HasFactory; protected $table = 'employment_announcements'; // primary key protected $primaryKey = 'id'; protected $fillable = [ 'user_id', 'date', 'title', 'file_name', ]; public function user() { return $this->belongsTo(User::class, 'user_id', 'id', 'users'); } }The migration:
public function up(): void { Schema::create('employment_announcements', function (Blueprint $table) { $table->id(); $table->foreignId('user_id')->nullable()->constrained('users', 'id')->nullOnDelete(); $table->string('title'); $table->date('date'); $table->string('file_name'); $table->timestamps(); }); }
CreateEmploymentAnnouncement.php <?php namespace App\Filament\Resources\EmploymentAnnouncementResource\Pages; use Filament\Pages\Actions; use Filament\Resources\Pages\CreateRecord; use App\Filament\Resources\EmploymentAnnouncementResource; class CreateEmploymentAnnouncement extends CreateRecord { protected static string $resource = EmploymentAnnouncementResource::class; protected function mutateFormDataBeforeCreate(array $data): array { $data['user_id'] = auth()->id(); return $data; } }If I create a new EmploymentAnnouncement from the admin panel, It is created but then at the redirect I get the error attached to this post.
Solution:Jump to solution
Finally I found the answer to the problem thanks to @awcodes
it was solved here https://github.com/filamentphp/filament/issues/7592#issuecomment-1671997859
Basically somehow the models were extending the wrong class (Pivot instead of Model)....
GitHub
Missing required parameter for edit route redirect after creation e...
Package filament/filament Package Version ^2.0 Laravel Version ^10.10 Livewire Version No response PHP Version 8.2.6 Problem description After trying to create a model using the dashboard, the redi...
27 Replies
You haven’t created an edit page by the looks of it
I do have also an edit page
<?php
namespace App\Filament\Resources\EmploymentAnnouncementResource\Pages;
use App\Filament\Resources\EmploymentAnnouncementResource;
use Filament\Pages\Actions;
use Filament\Resources\Pages\EditRecord;
class EditEmploymentAnnouncement extends EditRecord
{
protected static string $resource = EmploymentAnnouncementResource::class;
protected function getActions(): array
{
return [
Actions\DeleteAction::make(),
];
}
}
And that’s declared within the resource?
yes
public static function getPages(): array
{
return [
'index' => Pages\ListEmploymentAnnouncements::route('/'),
'create' => Pages\CreateEmploymentAnnouncement::route('/create'),
'edit' => Pages\EditEmploymentAnnouncement::route('/{record}/edit'),
];
}
Yeah that looks good, so the edit employment route is looking for the record and it’s not being passed in.
Really strange looking at the code it seems fine from plain text reading
Did it definitely save?
yes.. it looks strange, I have other models which are very similar and those work, only with this one I have the issues..
Yes, it is saved
what happens if you put this on the create page class
protected function getRedirectUrl(): string
{
return $this->getResource()::getUrl('edit', ['record' => $this->record]);
}
?
The first SS is a dump of $this->record, the second is the error that I get after the creation (from the redirect) with the new code applied.
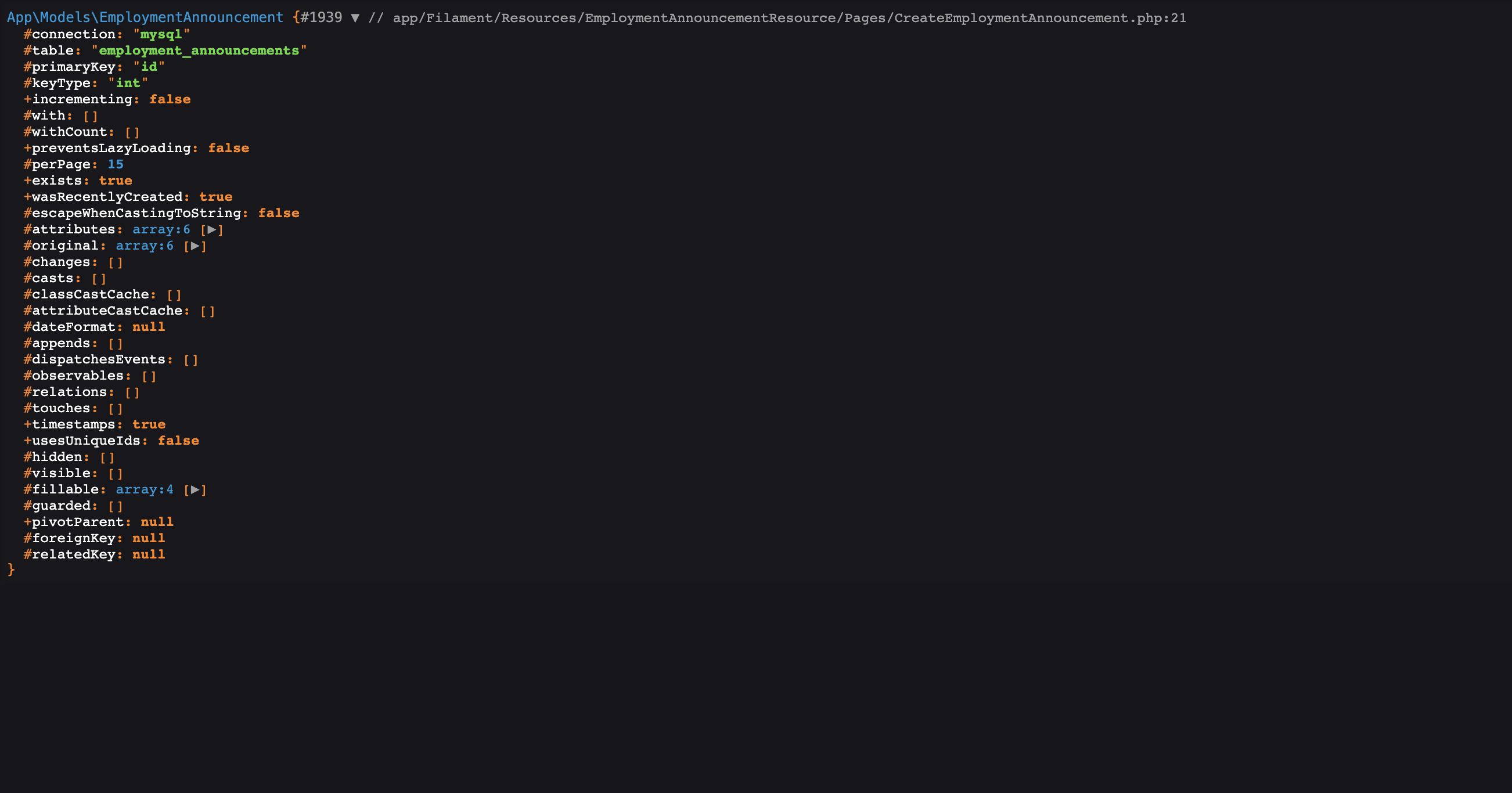
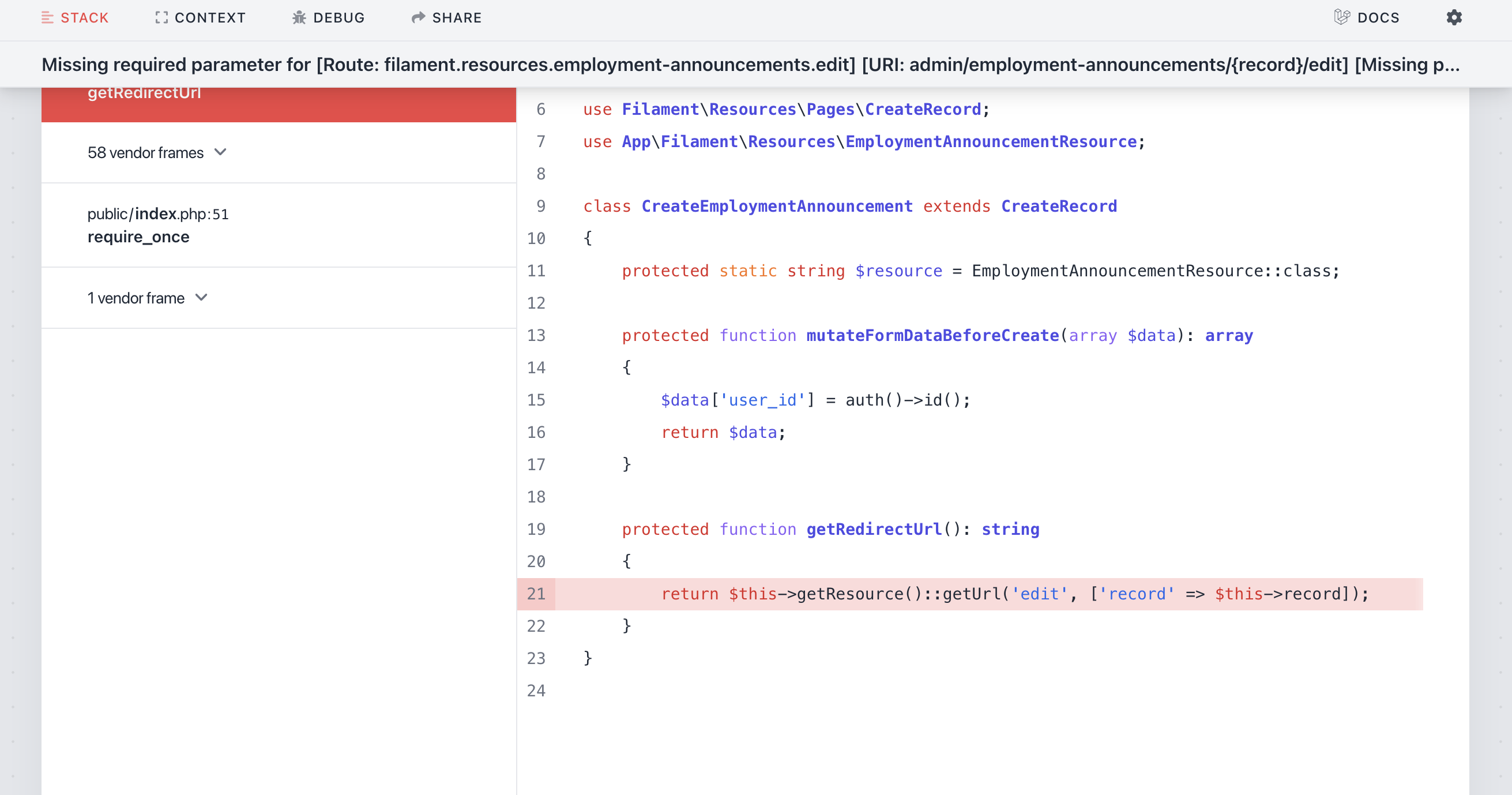
the attributes array is filled with the correct data that you expect?
have you tried php artisan cache:clear and composer dumpautoload ?
protected $fillable = [
'user_id',
'date',
'title',
'file_name',
];
yes
Tested and the same..
I mean in the dump SS if you expand the array "attributes" does it has the data that you put in the inputs?
Nope
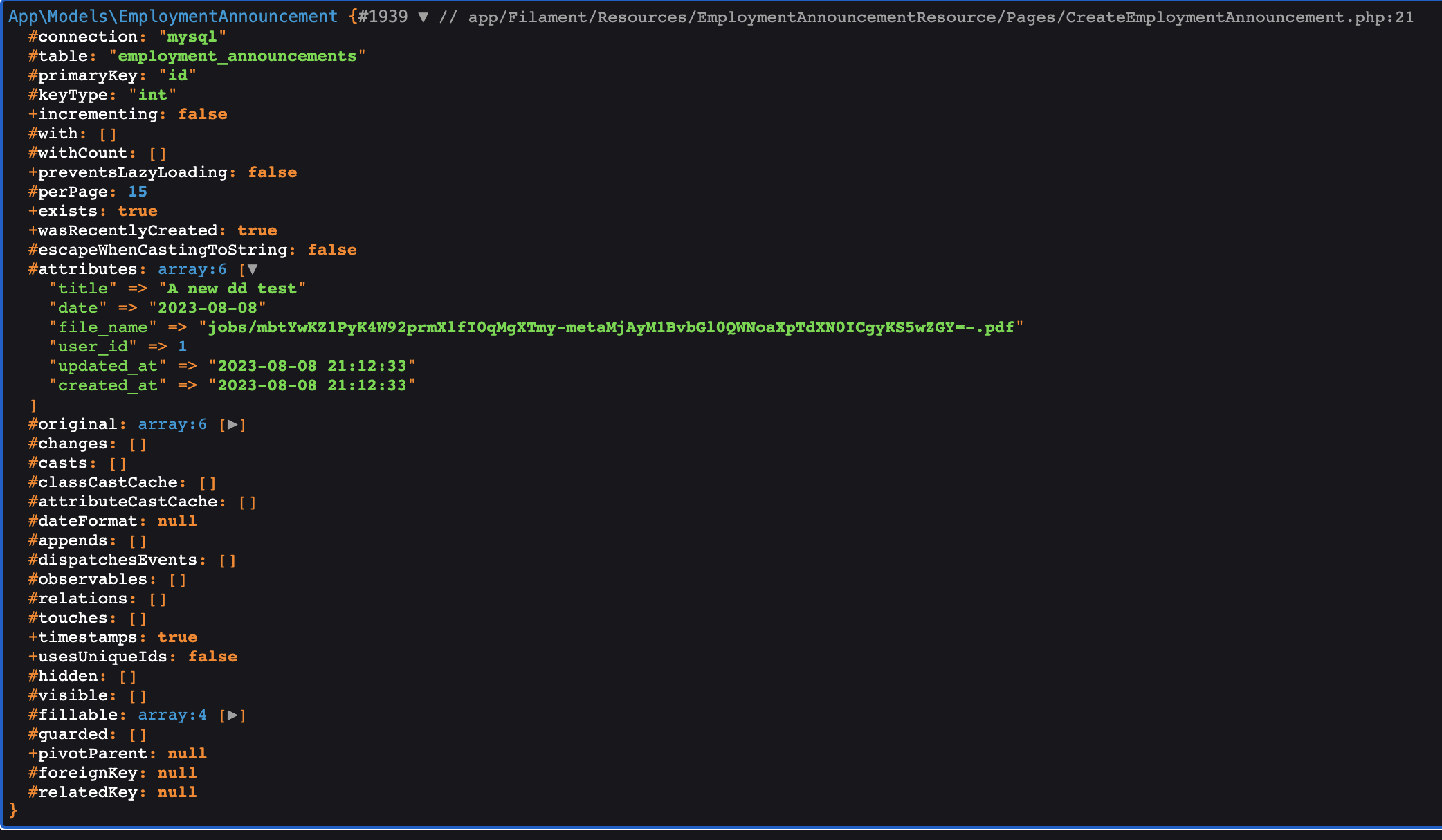
So the record object doesn't have the data of the recently created that you want to send to the edit page.
I guess so, the records are created in the db tho
maybe some class is overriding your create class of that resource, maybe some mappings are wrong :/
Where should I look ?
In the AppServiceProvider boot() this is what I have:
/**
* Bootstrap any application services.
*/
public function boot(): void
{
Filament::serving(function () {
Filament::registerNavigationGroups([
NavigationGroup::make()
->label('Administration')
->icon('heroicon-s-library'),
NavigationGroup::make()
->label('Content')
->icon('heroicon-s-pencil'),
NavigationGroup::make()
->label('Settings')
->icon('heroicon-s-cog')
->collapsed(),
]);
});
\RyanChandler\FilamentNavigation\Filament\Resources\NavigationResource::navigationGroup('Administration');
MediaLibrary::registerMediaInfoInformationUsing(function (array $information, MediaLibraryItem $mediaLibraryItem, MediaItemMeta $mediaItemMeta): array {
return array_merge($information, [
'ID' => $mediaLibraryItem->getKey(),
'Thumb conversion generated' => $mediaLibraryItem->getItem()->hasGeneratedConversion('thumb') ? 'Yes' : 'No',
]);
});
MediaLibrary::registerMediaInfoFormFields(fn (array $schema): array => [
...$schema,
SpatieTagsInput::make('tags'),
]);
FilamentNavigation::addItemType('Page Link', [
Select::make('page_id')
->searchable()
->options(function () {
return Page::pluck('title', 'id');
})
]);
FilamentNavigation::addItemType('Document Link', [
Select::make('document_id')
->searchable()
->options(function () {
return Document::pluck('name', 'id');
})
]);
}
If you have other custom create classes I would begin with those and dd the save method
if you have a repo link I can try to check it
Just saw the DM, you can put link here
cool, leave here the flow that I should take on the app, Ill check it when I finish dinner 🙂
Alright, thanks
1. php artisan make:filament-user
2. php artisan tinker
$user = User::first();
$user->assignRole('super_admin');
3. php artisan shield:generate --all
4. Head to /admin/employment-announcements/create
5. Create a new resource using some dummy data
I'm hoping that you don't have any issues with the MediaLibraryPro package which is licensed...
ok, I found the problem, the redirect method uses route model binding. But the method is called before persisting the data on the DB. If you try ['record' => '1'] (hard-coded) the error disappears
Alright but then why is this happening ?
Theoretically it should redirect by default to the edit page after creation, right ?
Also, I found that this issue I have on multiple models excepting one ("Page") but I couldn't find out why yet
to me the buildings create is giving the same error
thats very strange
The same to me, also other models like phones, news
For those who also want to reproduce the error locally I've made a simple public repo at: https://github.com/aflorea4/filament-missing-required-parameter-issue
Setup:
composer install
php artisan key:generate
php artisan migrate --seed
php artisan serve
Log in with:
localhost:8000/admin
[email protected]
password
Create a new EmploymentAnnouncement model from the admin panel.
GitHub
GitHub - aflorea4/filament-missing-required-parameter-issue
Contribute to aflorea4/filament-missing-required-parameter-issue development by creating an account on GitHub.
Also opened an issue here: https://github.com/filamentphp/filament/issues/7592
GitHub
Missing required parameter for edit route redirect after creation e...
Package filament/filament Package Version ^2.0 Laravel Version ^10.10 Livewire Version No response PHP Version 8.2.6 Problem description After trying to create a model using the dashboard, the redi...
Solution
Finally I found the answer to the problem thanks to @awcodes
it was solved here https://github.com/filamentphp/filament/issues/7592#issuecomment-1671997859
Basically somehow the models were extending the wrong class (Pivot instead of Model).
Thanks everyone.
GitHub
Missing required parameter for edit route redirect after creation e...
Package filament/filament Package Version ^2.0 Laravel Version ^10.10 Livewire Version No response PHP Version 8.2.6 Problem description After trying to create a model using the dashboard, the redi...
I've got the same issue on a model called "Page", however I've checked it's extending model as expected - Missing required parameter for [Route: filament.resources.pages.edit] [URI: admin/pages/{record}/edit] [Missing parameter: record].
The page itself seems to work fine if I change it to be an optional parameter:
'edit' => Pages\EditPage::route('/{record?}/edit'),
However no other resources have this issue
can you share more detail about the solution ?