Maximum call stack size exceeded while using createEffect
Hello! I'm trying to fetch data from API endpoint and fill in the store values using createEffect
But I get an exception. The exception is Uncaught (in promise) RangeError: Maximum call stack size exceeded. I can't get why it happens.
But I get an exception. The exception is Uncaught (in promise) RangeError: Maximum call stack size exceeded. I can't get why it happens.
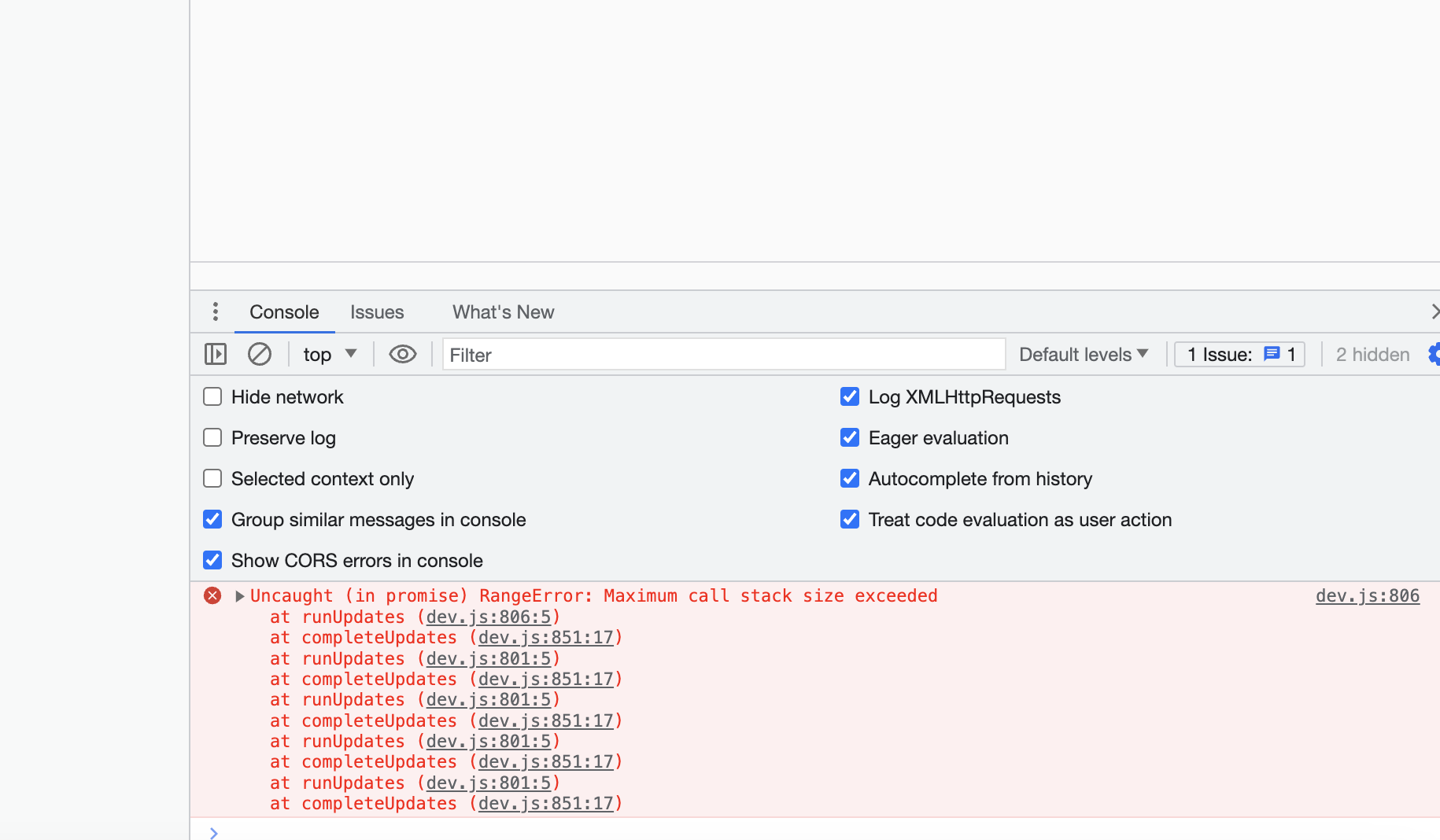
9 Replies
I used to use other approach to solve this task and it works well but it is not idiomatic:
Why does using createEffect here lead to infinite cycle?
I guess this is because you read and write formValues multiple times
well, if you setFormValues, you're updating that state, and it triggers the createEffect again.
which of course updates form values. which triggers the createEffect again.
since you read formValues in a createEffect, il will trigger the effect if it changes... but you change its value in the effect
Perhaps what you might want to do is a derived signal instead?
https://www.solidjs.com/tutorial/introduction_derived
Thanks to @lexlohr this code works
The thing is in that create effect expects getting true from inner func to stop triggering
... well, it's an other way to do this ...
but you should probably check out at derived signals like @eslachance said. Because, I don't think it's a good practice to edit an API data directly
Thanks for the answer! But why does changing the store trigger createResource when it must be triggered by changing of params.itemID?
I'm not seeing a dependency to params in your createEffect though?
not sure about the createResource