reordering based on condition
I have position column in table which I am reordering
Is it possible to reorder the position column with a condition that prevents reordering if the position value is 1?
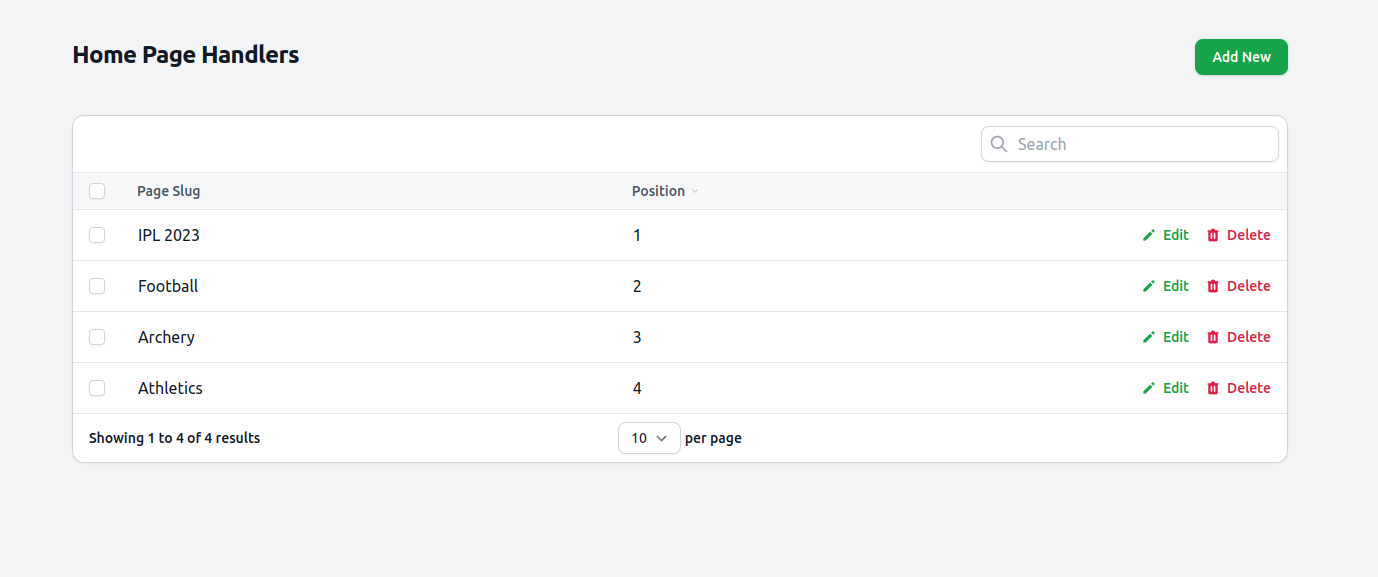
28 Replies
Hi. Do you mean you mean when you toggle on the reordering button (which I don't see in your screenshot) you want to be able to reorder all the rows EXCEPT the row with position 1?
sorry i shared wrong screenshot
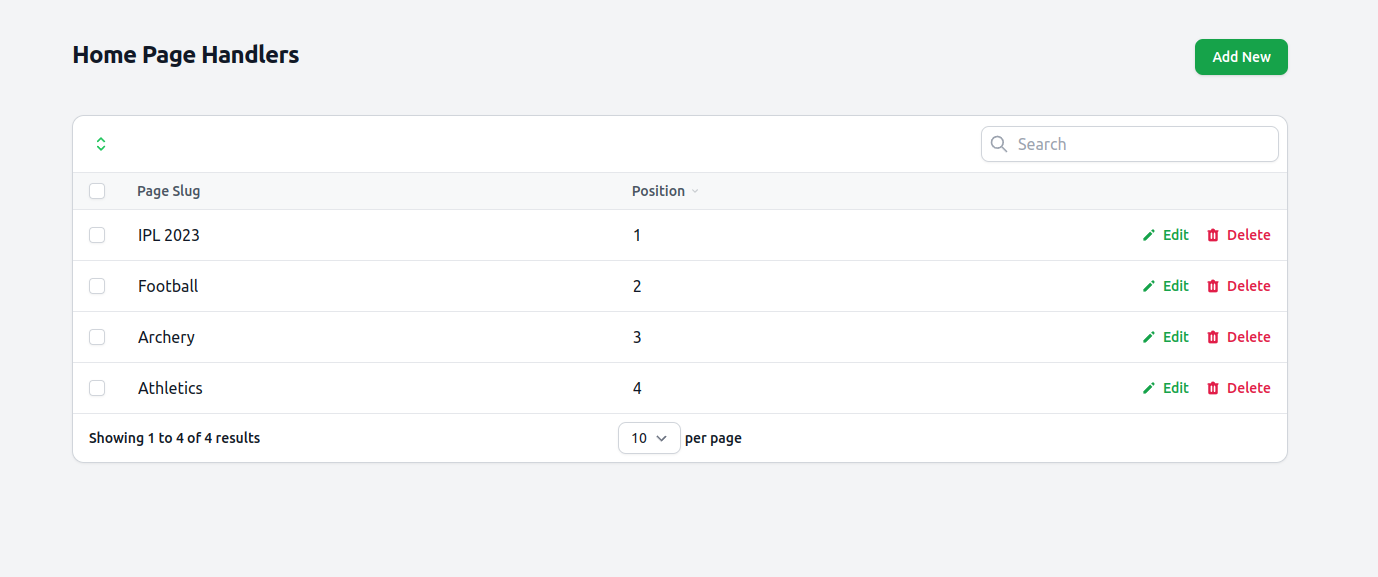
yes i want to reorder all rows except position 1
One way would be to override the reorderTable method in your ListHomePageHandlers class. I don't think you can disable the row so it won't move, but by overriding the reorderTable you can at least prevent it from actually reordering position 1.
There might be a better solution, but that's what my exhausted brain is coming up with
You can find the
reorderTable
method in CanReorderRecords
. You should be able to adapt that.
Just tested it out and it's actually pretty simple. Just add:
This will allow you to reorder any rows, but if it's position 1 it'll snap back and won't reorder. You could even add a Filament notification there and let your users know they can't reorder position 1yeah it is working if i am changing in vendor file but if i am adding this function in my list page it's not working
Can you share the full file?
Works for me. You need to include the entire code
I removed the relationship from this method
And you need to import the Str and DB classes
yeah its worked even though i dont have to add entire code...i dont know why that time it is not working
thanks
And don't forget to add a notification to let your users know why they can't sort position 1
Sure
Thank you
hey can you tell when you used this code do other rows can reorder ...in my case other rows cant be reordering with 1st row
And in $order we are getting ids from table...so how can we use $order[0] ! = 1
Correct. Isn’t that the point? If you don’t want position 1 to be able to move, then that also means that other rows can’t move into position 1, right?
Ok, I’m also assuming that “position” is your sort order column. Maybe position is a totally different column?
Do you have a position column and a sort column?
No that is not happening ..what i mean is when i am reordering position between 2 and 3 or 3 and 4....they are also not being reordered
no i have only position column
i am not sorting any other column
Ok. Thanks for clarifying. All the other rows were sorting for me. Did you copy paste my code? And as I mentioned, I had removed the relationship part so if this is a relationship then that could be a reason
And the reason this works is because the $order variable is just an ordered list of the new position numbers. dd($order) to see what I mean. It’s not a list of the model ids. So since position 1 should always be first (key 0 in the array), if there’s a different position number there then you know that position 1 was moved.
Here you mentioned you didn’t need to use the entire code…try the entire code.
I tried entire code and with relation part also
I think may be it's because you have newly created those...so primary key are starting from 1...may be you can delete some data then try reordering
I don't think that's the case, but let me check
Still working.
I'll send a video
I apologize...you are right. Hold on
@ba_mbi_07 Ok my apologies. I was wrong on what the $order was returning. It was returning the model's id. I was trying to do too many things at once. In this case I think you need to query the model and check if the model id in
$order[0]
is position 1 in the DB. Here's how I did it and now it does work:
You might be able to refactor that to avoid a separate DB call and only update it if the position isn't 1...but I'll leave that to you.
hi, this isnt related to the question but, if we modify anything in the vendor file, wont it go missing later (for example if my friend installs and runs the app on his ide)
Correct. Don't modify anything in the vendor file unless you want to just test something really quick. When you composer update or push to production you'll lose those changes. The above change isn't overriding a method in the vendor file, but inside their project. That you can safely do
any way to keep those changes eventho after composer update?
I mistyped above (fixed). The way to keep those changes is to override the method in your own ListXYZ class.
@black13kaiser So using this help topic as an example, OP has a HomePageHandlersResource. There is also a ListHomePageHandlers class. The code I shared above would need to be copied into that ListHomePageHandlers class. This would then override the vendor's
reorderTable
method. Then you can safely composer update because you haven't touched the vendors file@black13kaiser maybe checkout a refresher or tutorial on laracasts about OOP. That should help to make it more clear.
understood
thanks!
Hope that didn’t sound condescending, it wasn’t meant that way.
nah, at least now i know about laracasts
because the case here is im using a blog package, which creates three resources for me (blog, author and category). i created a custom page for reading the blog, and i added a read button at the vendor's blog resource
only then i discovered that it wont be saved on production/ new install
Fair enough. Get it done. 👍
its okay though..but I got the idea what i need to do from you
yeah i did same way...Thank you for your help 😃
hey i need more help this
I actually added my position 1 data to separate widget now when i am trying to reorder that its not reordering