❔ C# abstract classes & interfaces
So I normally code in java, and I was wondering, are there any major differences in terms of abstract classes and interfaces in C#, I thought C# had multi inheritance so then what’s even the point of interfaces.
47 Replies
C# does not have multiple-inheritance
thus, interfaces
C# does KINDA SORTA have multiple-inheritance, but not really, in the form of Default Interface methods, added in C#8
Oooh I thought it did oh well java has that too
But abstract methods would be better for that, even tho I still don’t see what the real difference is if they have default methods
I guess fields
interfaces are a decent bit more flexible
and no, abstract methods don't allow for multiple inheritance
interfaces (sorta) do
there's not a WHOLE lot of practical difference between base/overridable methods and interface default implementation methods
interface default implementations exist mainly because they didn't used to
and there was a decent use-case for adding support
For all practical intents and purposes, C# does not have multiple inheritance.
(I believe) default interface implementations are just a trick of the compiler
a class that implements an interface with a default implementation isn't actually INHERITING that implementation, the compiler is effectively copy/pasting it into the class
like a mixin
I think
The point of interfaces is to provide a contract that a type has to implement. Abstract classes provide base implementations and methods. They're completely separate purposes.
yeah
what interfaces REALLY allow for is polymorphism
"I, as a consumer, need something that does X. I don't care what or how."
and yes, you can accomplish the same thing with base/abstract classes, but that's not quite what those are for
base/abstract classes are mainly about de-duplicating logic and functionality
interfaces have neither of those things (default implementations notwithstanding)
Ye but
At that point you would never actually have to use abstract classes
I guess the difference is that abstract classes can have fields which makes them very different
But ye
But fair enough then they are the exact same as in java
abstract classes contain logic and state, yes
interfaces do not
Wdym logic and state?
I mean I see of interfaces as being a static thing
Like ye the methods aren’t static but like the interface itself is
Ok but if there are no special things in c# for that then I know it all
They're not about state, they're about what you can do with a class
You pretty much never have to use them unless you explicitly want to inherit behavior, for instance for base implementations of interfaces.
Well and one big thing is that abstract classes can inherit other classes, so you can actually limit the use. Like you can have EatingAnimal which extends Animal so only like Cat and Dog can override the eat method, unlike interfaces where you could make a house an EatingAnimal which is…. Cursed
Well, a house implementing that interface wouldn't make sense
A house could also inherit from Animal if it wanted to
Well not if it already inherits building
You could likewise have an
Animal
and IEatingAnimal : IAnimal
interface
yeahI guess that’s the point of only allowing inheritance of one class
until you want to include carnivorous plants in your model and now inheritance doesn't seem like such a good idea 😛
But then you run into the issue of a caravan being both a house and a vehicle. You'd ideally want to inherit from both
House
and Vehicle
, but you can't. However, you could implement both IHouse
and IVehicle
just fine.Eh ye but that’s def not something I would use an interface for
Because a house and a vehicle aren’t something you add to a thing
Its more of a thing on its own
IMovable would be better then
it's a thing that works as two different things
the granularity of your interfaces depends on your needs
Ye but like the class Vehicle def requires fields
And you can’t do that with an interface
So you’ll have a problem
I guess you can do abstract getters and setters but I don’t like those
Makes your code so big imo kind of stupid
Like at that point you should use a abstract class
Unless C# has a solution for this?
the class implementing the interface can implement it however it wants
interfaces define contracts, they aren't instantiable types
they tell a consumer how it can use the object, the implementation details are irrelevant
Ye so a House and Vehicle shouldn’t be an interface imo
then what should a caravan be?
because like thinker said, it's a house and a vehicle
for jeremy, trashed
if you have a function that does something with an object that works like a house, how would you pass it a caravan?
Or vice versa with a vehicle
if it has to be something set at runtime then it can't be inheritance
it must be composition
i would say it depends
this is going from meta-talk to details about implementation
(it could even be a data approach, object is registered somewhere both as a house and as a vehicle)
everything depends in programming
generally
it depends if it depends
can i? $itdepends
Lol why an elephant
because they are wise
Lmao
I guess
based on this (an actual series of books)
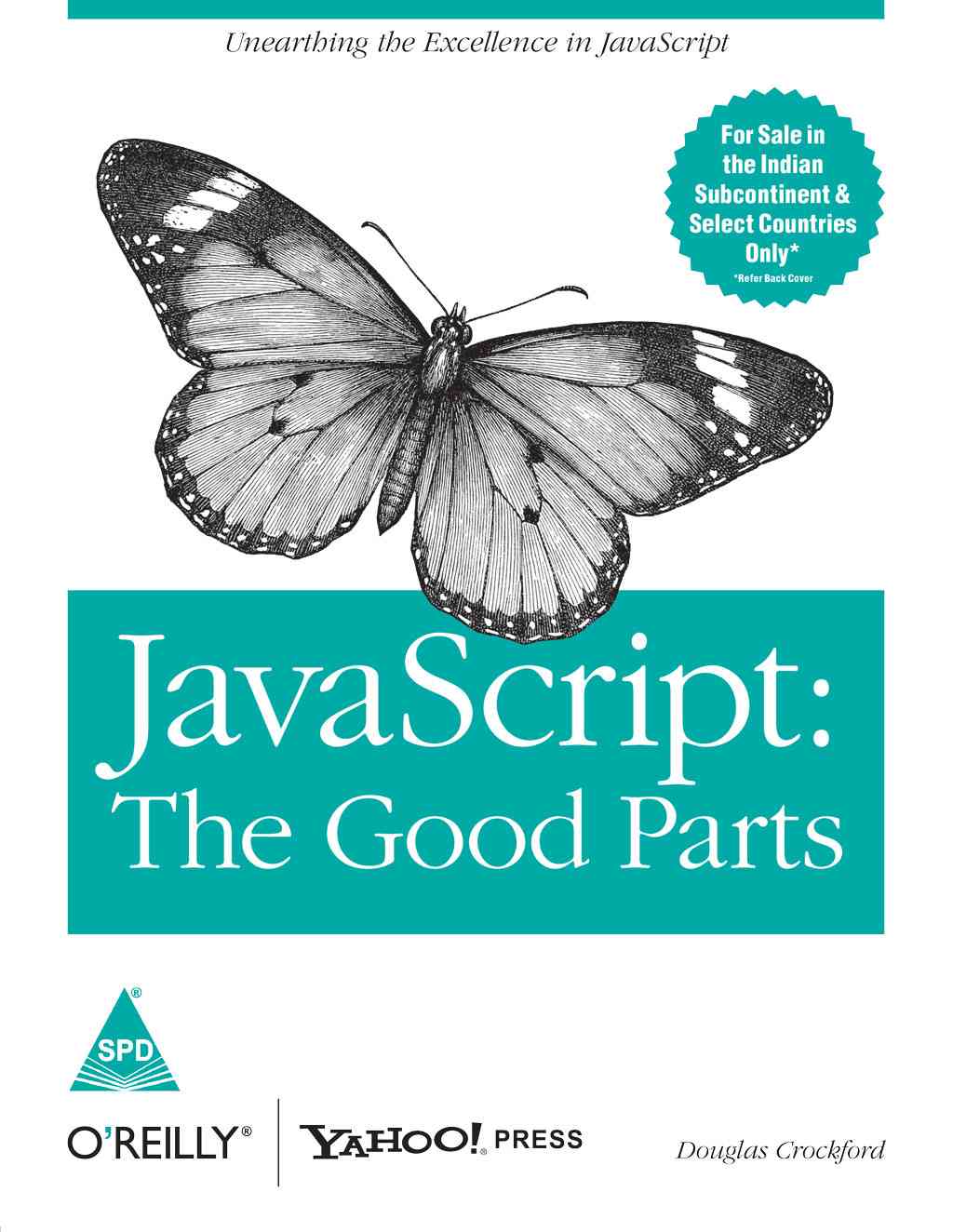
Abstract classes are good when you want to split up an implementation into substeps. You can define a sealed implementation of an interface method in an abstract class, that just calls into abstract or virtual methods and maybe adds some glue around those calls. That's basically all I typically use them for.
Defining abstract classes for mixing in some helper code is an overused malpractice in my opinion
this is called template method design pattern and it can be done using interfaces too since c# 8.0
you use abstract class for it in old c# version because interfaces don't support default implementations. Since they do you better use interfaces for that
I'd say we use abstract class over interfaces when we want to inherit instance variables like fields and properties
you can seal methods on interfaces?
no you do it without sealed then
Is this the name of a pattern? Because I've never heard this term.
here a very basic exmaple
AllCharsValid and IsValidLength get implemented by concrete validator. IsValid() is our template method that declares the structure of our algorithm without concrete implementation
That could just be an extension method 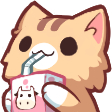
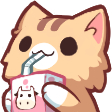
right
ok other example, suppose we have a fight method that always contains certain steps like draw weapon, aim, shoot, whatever. But each weapon does those steps differently. We can have template method fight that calls these methods which get redefined
it is the name of the pattern, yes
where you give general structure / order of algorithm but leave concrete implementation of the steps to concretions, the method that declares the structure and doesnt get redefined is a "template method"
its part of the original GoF patterns
GoF patterns?
Gang of Four
Also btw I don't think this has to do with the original question anymore
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.