❔ Design Pattern
This doesnt make sense to me, its clearly inside my library:
System.IO.FileNotFoundException: 'Could not load file or assembly 'System.Configuration.ConfigurationManager, Version=7.0.0.0, Culture=neutral, PublicKeyToken=cc7b13ffcd2ddd51'. The system cannot find the file specified.'
347 Replies
@Protagonist still there?
i have now moved to appsettings.json, and redoing my methods to work with json
this was old post ish
this is the library
this is the consuming console app
thats the config file, in json
I could easily change the
AddJsonFile
to be xml, or ini, or yaml, or whatever other format I want
Its a bit of turkey stuffing, but I hope you get the core concept here
@Protagonist lemme know if something isn't clear and you need a more in depth explanation.okay so without the consuming app console including the DI then it wouldnt function at all in the console app?
well, no, since there would be no
IConfiguration
registered.
as mentioned before, this is the modern way of doing things and it gives the consumer all the power
Deserilize class
Game class
@Pobiega I was just changing the methods to go to json instead of xml, does this look correct?
uhm
I kinda hate it, since you are not actually using strict models for your configuration.
I'd much prefer a
.Get<T>(section)
approach if thats all you want
also, this doesnt seem to be "configuration" at all
this seems to be straight up loading/saving application data
and for that, you can just use JsonSerializer
directly.I thought it was configuring since im not hardcoding anything and everything can be configured in the file
I had no idea about any of this so i was just searching too
what would i change to make this configurable?
you're not loading configuration as such
you are loading application state
thats better done with just raw json serializiaton
by application state do u mean how some variables will be changing?
for example health
Im not sure what to do from now on
also i get this error
System.IO.FileNotFoundException: 'Could not load file or assembly 'Microsoft.Extensions.Configuration.Abstractions, Version=7.0.0.0, Culture=neutral, PublicKeyToken=adb9793829ddae60'. The system cannot find the file specified.'
something is weird with your builds/nuget restores
you ahd the same for configurationmanager
anyways, json serializaiton is easy
the absolute simplest version is just this
so something like this?
what does your
World
class look like?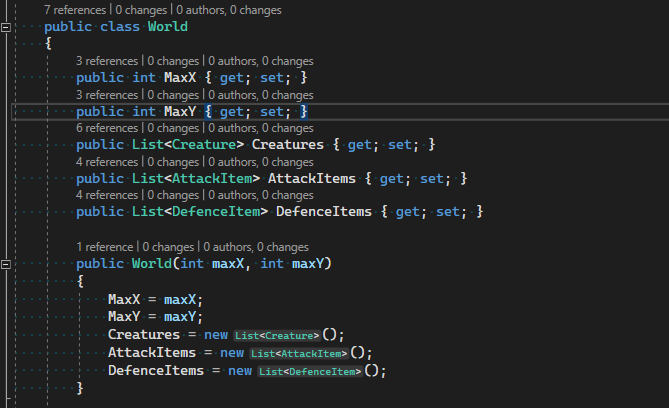
it includes methods aswell
thats fine
with that structure, you can literally store an entire world as a json object and deserialize it with a single line of code
is all you need
it will load creatures and attackitems and defenceitems too, from the json structure
And this part will work just fine if i do so correct?
:p
So the creatures and the worldobjects wouldnt need to be added to the world because it will already be added to it this way?
yes
And the appsettings.json file, what adjustments would have to be made to it
its important that your variables correspond to the right keys in the json file thou
so if the property on AttackItem is
string Name
it needs to be "Name" in the json
not "name"Oh okay i see thank you
i thought here it was just like the sectionName
no this is direct object deserialization
the structure and names of your json must exactly match your classes
that makes sense
but heres another question
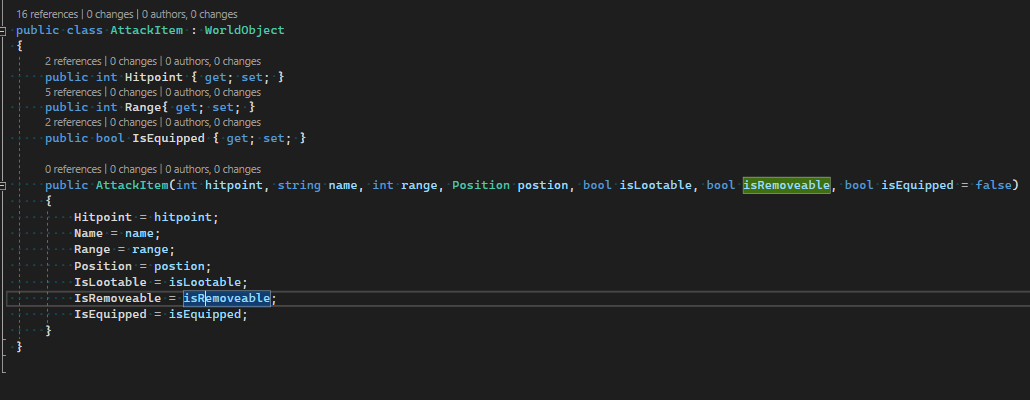
im inheriting from the worldobject class
thats fine.
so some properties arent in the attackitem itself
thats fine.
would the json file know this?
yes.
or rather, the deserializer doesnt care
AttackItem DOES have a
Name
property, even if its not declared inside AttackItem
thats how inheritance works
the child has the props of the parent, even if they are not declared thereeven if i dont specify it i can still say the attackitem has a name?
thats nie
nice
well yes, because it does
is valid
(except for the fact that you dont have an empty ctor, but thats not important here)
I didnt know that
thank you
what about the Position property in this case, im using x and y to map it in the json file
what would i name those
what is a
Position
?The position of the creature or worldobjects
so x and Y
?
yes
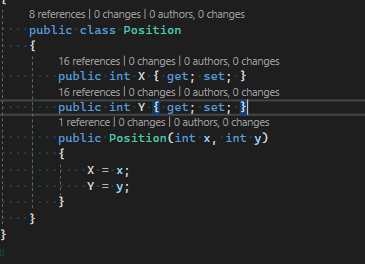
everything has a position
I see
does the placement of the properties matter or no
nope
just names and values
so i was just thinking the Creature has a IsDead prop, for the bools like IsDead, IsEquipped etc. Do i have to put like a default value to it in the json file
no
also do i have to mention all the properties of a class in there
they will by default have their default values, if left out of the json
nope, if you are fine with them having their default value you can leave em out
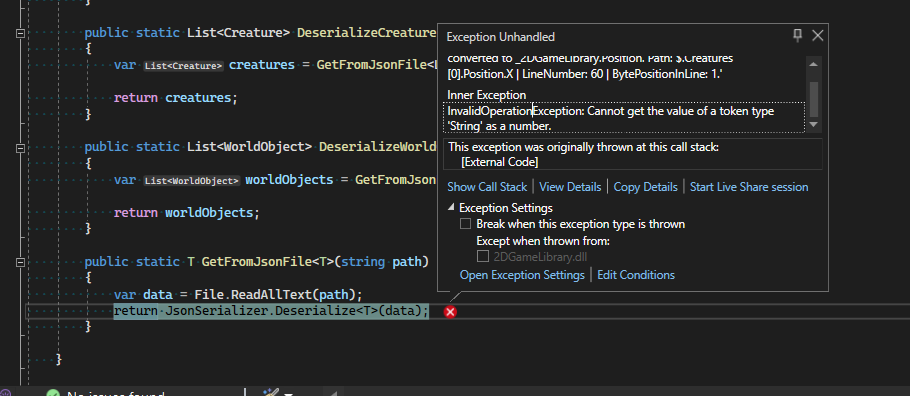
wouldnt i need to parse all the integers
no
oh sorry, you stringified your numbers
dont do that
etc
numbers should be numbers in json, not strings
Makes sense
"AttackItems": [
{
"Name": "sword",
"Hitpoint": 10,
"Position": {
"X": 23,
"Y": 25
},
"Range": 5,
"IsLootable": "True",
"IsRemoveable": "True",
"IsEquipped": "True"
},
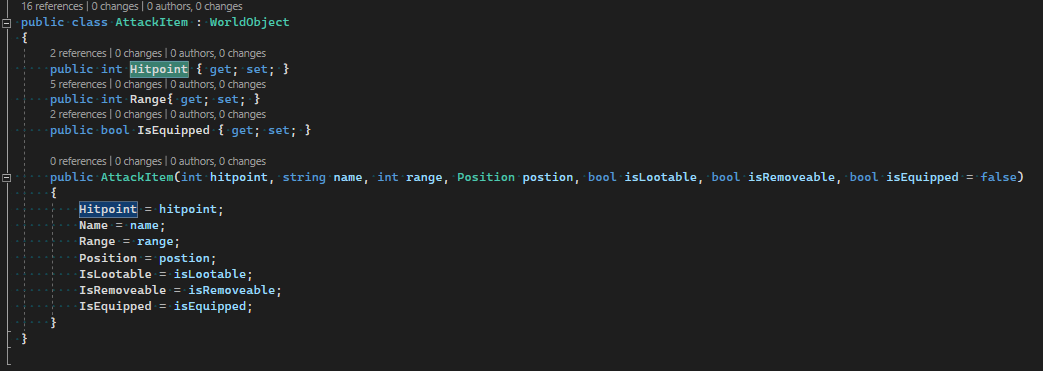
Everything matches here right?
System.InvalidOperationException: 'Each parameter in the deserialization constructor on type '_2DGameLibrary.AttackItem' must bind to an object property or field on deserialization. Each parameter name must match with a property or field on the object. The match can be case-insensitive.'
Your constructor doesnt match. Either make a parameterless one or one that matches the json
so it matches with the params in the constructor instead of the actual properties itself
It can do either
But the object must be constructed
That's the rules of C# itself
so why was it complaining earlier with the attack items naming
Exception thrown: 'System.Text.Json.JsonException' in System.Text.Json.dll
An unhandled exception of type 'System.Text.Json.JsonException' occurred in System.Text.Json.dll
The JSON value could not be converted to System.Collections.Generic.List
1[_2DGameLibrary.Creature].`
i dont put the IsDead inside the ctor but i tried earlier to give it a value in the ctor and still the same exception shows upLooks like the JSON doesn't match the class
Or it doesn't represent a list
But it does ive even made sure it does
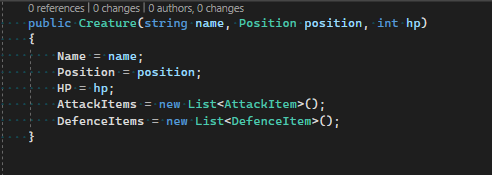
its specific to the creature section
does it not look accurate
And you're trying to deserialize this JSON to
List<Creature>
?Yes because the world has a list of creatures
World object*
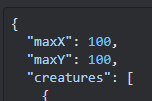
A
List<T>
doesn't have maxX
or maxY
properties
Nor does it have a creatures
propertyOne second
So you are not trying to deserialize it to
List<Creature>
?
But to a World
?well what im doing here is deseralizing the creatures then adding it to the world:
Deserialize it to a
World
Since it clearly is a World
It's neither
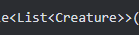
nor

so how would i add creatures and worldobjects to the world then
Deserialize the JSON to the
World
Add a creature to that
Done
I dont get it,
Theres a method that returns all the creatures from the json file and another with the of worldobjects, then we are adding all of these inside the world
what am i changing exactly
What?
You wanted to add a creature to the world, no?
So you take the world
Take the list of creatures in it
And add a creature to that list
You can't just pick and choose which part of the JSON you want to deserialize
You deserialize all of it, then you modify the parts you need
im new to all of this, can you show me a step towards the right direction code wise?
Here's an example
but you are making the things and world inside the code
the whole point of this is to make it configurable so everything is made inside the file then we just add them but in this case its hardcoded?
MockReadFile
returns a JSON string
Like what you would read from a file
Replace it with File.ReadAllText()
and therepublic static T GetFromJsonFile<T>(string path)
{
var data = File.ReadAllText(path);
return JsonSerializer.Deserialize<T>(data);
}
but this?
doesnt this read everything already?
Yeah, and it deserializes to a
T
That T
has to be a representation of the JSON
In my example, I could not use your GetFromJsonFile<List<Thing>>()
Because the JSON I'm trying to get is not a List<Thing>
It's a World
So only GetFromJsonFile<World>()
will work
The generic parameter in JsonSerializer.Deserialize<T>()
is not there to let you pick and choose which fragment of the JSON you want
It's to pass a type that represents the JSON as a wholei believe i have fixed these errors
thank you
although more errors on the rise now 🙂
kek
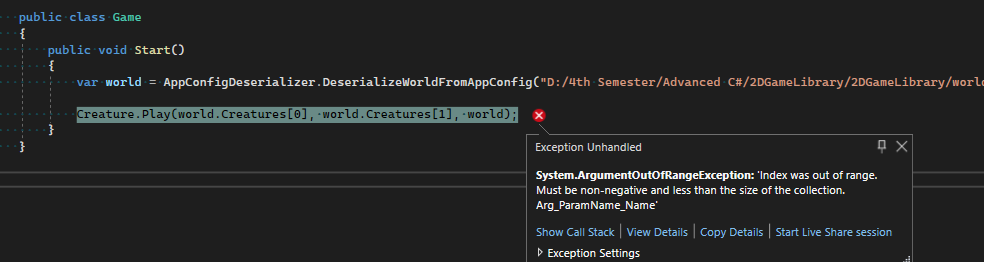
its because nothing is being added to the world
It's because there are no creatures
There are creatures in the json file
The error begs to differ
Use the debugger to see what
world
actually containsnothing in any of its lists
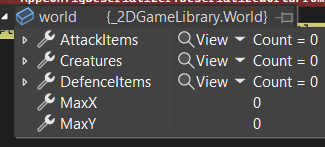
Naming matters
foo
is not the same as Foo
So use PascalCase
names in your JSON, like in your classes
Or use JsonSerializerOptions
to set up case-insensitive serialization"creatures": [
{
"name": "Steve",
"position": {
"x": 10,
"y": 10
},
"hp": 100,
"attackItems": [],
"defenceItems": [],
"isDead": false
},
{
"name": "Bob",
"position": {
"x": 20,
"y": 20
},
"hp": 100,
"attackItems": [],
"defenceItems": [],
"isDead": false
}
do these refer to the properties or the ctor's param names
Properties
You don't need the constructor for deserializing JSON
It always uses the default, parameterless constructor, and then sets the properties
Okay so i made the Creatures work
System.InvalidOperationException: 'Each parameter in the deserialization constructor on type '_2DGameLibrary.AttackItem' must bind to an object property or field on deserialization. Each parameter name must match with a property or field on the object. The match can be case-insensitive.'
this is what i get on the attackitems
prob will get the same on defence itemsTry just deleting the constructor?
"AttackItems": [
{
"Name": "sword",
"Hitpoint": 10,
"Position": {
"X": 23,
"Y": 25
},
"Range": 5,
"IsLootable": true,
"IsRemoveable": true
},
{
"Name": "dagger",
"Hitpoint": 10,
"Position": {
"X": 15,
"Y": 15
},
"Range": 5,
"IsLootable": true,
"IsRemoveable": true
}
],
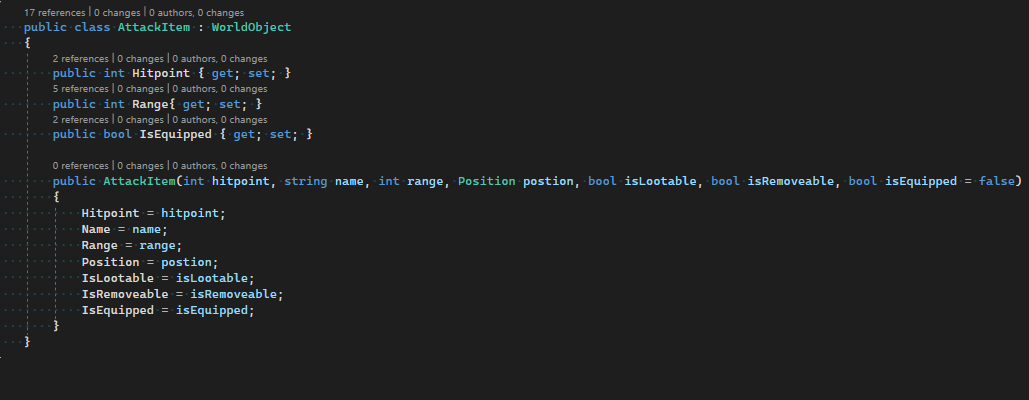
oof it worked
what does the ctor have to do
also it seems to have added everything twice
why did that interfere
Json serializer tries to use the default constructor and then sets the properties. In case there's no default constructor, one is provided, it has to be specifically set up. Every property needs to be set, there can't be more params than properties, and so on
I don't understand that fully
I just know it's a pain, so I don't use constructors for classes I'm serializing
required
properties do the jobi see, thank you
Everythings finally working, thank you guys ❤️
Now onto the next tasks zzzz
Btw you see for tracing and logging, is this wrong?
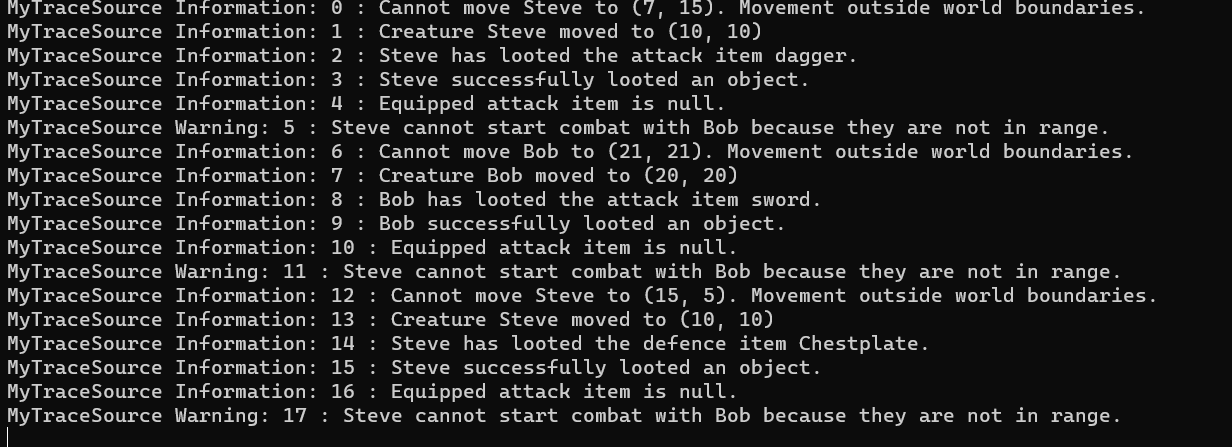
since it logs important things
Looks good to me
Depends on how you do this logging, though, I'd probably get fancy with changing the colour depending on the log level
oo that sounds nice
to me using even 3 of these would make sense if i had different types of creatures but i only have one type
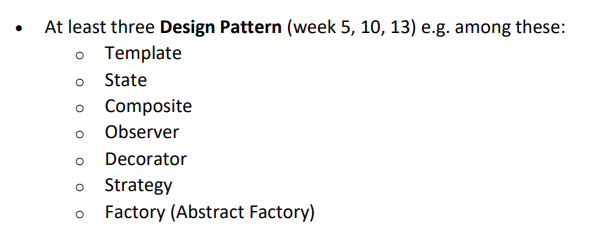
how else would i make these work
these are all my classes
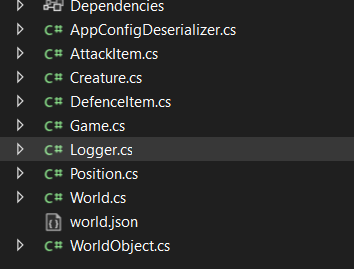
Also, to add on i dont think theres a reason but interfaces or abstract classes? should i add any here
This is getting dangerously close to "do my assignment for me" territory
i'd disagree, the logic was all done already, it was just configuration that i havent heard of and a question about if my logging was done correctly. Also im just asking for some insight on what design patterns would make sense since i only have one type of creature
The way i worded it made it seem otherwise
my apologies
Not the config stuff
The whole "what design patterns should I apply and how, and interfaces/abstract" stuff
Normally you pick design patterns before coding your entire app :p
oopsies
Not how but
I need to pick 3 design patterns
its the requiremnet but looking at most of them
they would only make sense
if i had more than one creature
Yeah, for a simple app like this most patterns would just make the code harder to read
can you suggest 3 that would make sense with what i currently have?
No.
That would fall under "do the assignment for you" umbrella
You need to think about your design and what patterns would make sense, that's the point
Yh youre right apologies
what if none of these apply to my application
but i would still need to use 3
does that mean id have to go back and update all the logic again
To some extent, yes.
But if you are ok with it being "bad" it won't be an entire rewrite
You could slap on a factory literally just for the sake of it, but it would be obvious that's what it was
If the pattern doesn't solve any problems, it tends to stand out
Does the pattern have to be for multiple classes or can it just be for one class
Right now i have
nothing to solve but my main class (creature class) has no use of any design patterns
but it does follow OOP
and SOLID principles
I mean, thats for you and/or your professor to decide?
you could just slap a "CreatureFactory" in there and call it done
but it would feel "slapped on"
🙂
the thing is its a requirement so i have to
if i do that im sure the teacher would point it out
what and why and how will be asked
then you might need to redo parts of your app.
Strategy pattern could be used to give your creatures different AI for example
For example if i add "Types" and they dont have anything special other than it being a certain type and thats all
would that count as useless or
could it contribute towards a factory or decorator design?
once again, thats up to your professor to decide.
IMHO If you just add a decorator pattern for the sake of adding one, that shouldnt count
you likely didnt learn anything
what if i add one for types of movement
so i have a move method
and if the creature can
swim
or fly
or crawl or walk
swim/fly/walk makes sense, if you add in obstacles
water/trees etc
what if we say crawl/walk/fly
how is crawl/walk different
other than just states of moving, nothing but what if it belongs to a certain type of creature?
maybe i could do like move +2 x and y with crawl
then isnt that just a prop on
Creature
?it is if it doesnt include any "benefits"
yup.
if i do this would it be okay?
¯\_(ツ)_/¯
sure
okay so, does this count as factory design pattern
satisfy*
yes
oo good
ive also added decorator class
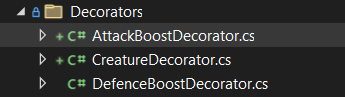
thats 2/3
i wanted to ask, i have a logging system.
I was wondering if that would technically count as an Observer design pattern
Looks like this:
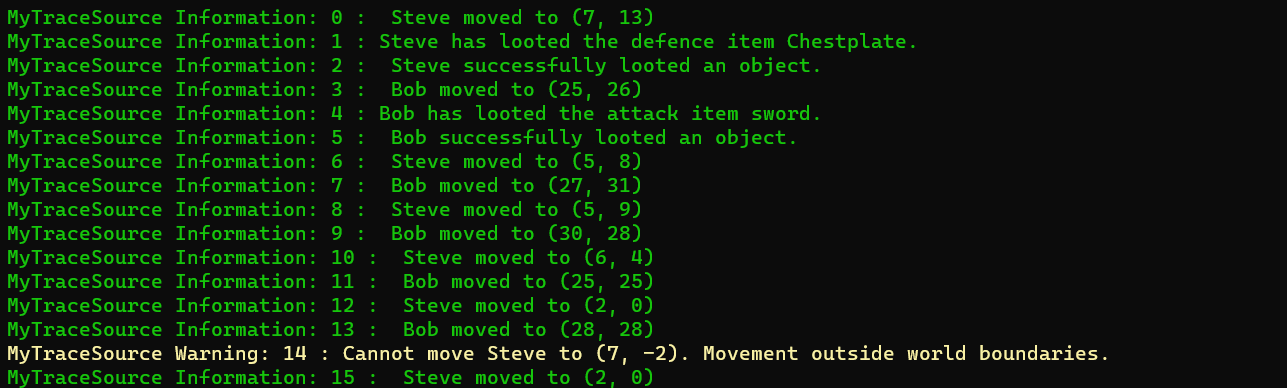
Does this count?
If so ive finally done 3/3
if you have a Subject that can notify a List of observers and update each observer then yes it should count as observer pattern. That is if you programmed towards abstractions and not concretions
and the observers gotta be able to add and remove themselves to the list of observers
"dragon" => new Dragon(name, position, hp) { Type = "Dragon" },
Type
should likely not be a string, and even if it should it should be set by the class (you don't want a Dragon
instance with a type of "Cheese"
), not as a settable field.
public string Type { get; } = "Dragon";
in your dragon class would do this.I agree that this is a code smell to pass the type as string to the Create method but I saw it implemented the exact same way when searching for factory pattern online. I think the best way would be to make the Create method or even the whole class generic instead:
I dont have any classes or anything, just a loggin system. So im assuming this wouldnt count towards an "Observer" Pattern but its still notifiying when things are done and updates the user in a way, so could it not be counted as a observer design pattern
even if its a string then putting something as "cheese" wouldnt count as a creature with the way i set the Create method up:
if its something other then these two it will throw an exception
also its better this way because of my json file
i can go back to these decorators anyway, im just wanting to know if the logging system will count towards an observer design pattern
I don't mean the input string
oh the type for Type itself
I mean the hardcoded string value assigned to the Type property in your initializers
That should not be specified here, imho
but i dont think it should be a param for the method
is that what you was thinking?
then i pass it to the creature
No, it should be set in the classes
Like so
public Dragon(string name, Position position, int hp) : base(name, position, hp + 50)
{
Type = "Dragon";
} this is how ive set it since type is in the creature class
} this is how ive set it since type is in the creature class
It should be marked abstract, or specified via an interface
And as a get only property
its just set as a property in the Creature Class:
should i just pass it in the dragons ctor?
that way it wont be hard coded anymore
thats one solution
also this? any insight on this
... how would logging be related to the observer pattern?
It's absolutely not
because technically its updating the user if updates that happen
maybe similar is the word
No.
Not at all.
That you are even trying to argue that tells me you have no idea what the observer pattern is :p
not arguing just thinking
your code calling Console.WriteLine is NOT an implementation of a specific pattern
That means even tracing wouldnt count correct
The thing is im stuck right now
ive chosen 2/3 patterns
strategy seems like a low hanging fruit
i tried doing strategy yesterday and my code blew up
you could have your creatures use a strategy implementation for their "thinking"
i tried doing it with movement
so two dragons might behave differently
i was thinking each type of creature will move differently
something like this
i implemented an interface called Imovement yesterday and a Fly movement and tried puttin in logic and so on, but then i remove it all cos it ended up getting confusing and not working
the thing is my Move implementation of the code is in the creature class, and i tried making a Defaultimplementation and make it so that creature uses it and then say that when it comes to dragon it will fly, so print out fly and move x + 2 and y+ 2 but this didnt end up working
would this be okay for strategy pattern
Strategy Pattern is not only inheritance but also composition. So to complete your code, we let a Creature HAVE a MovementStrategy which delegates the work to the concrete MovementStrategy:
Mhm i see, thank you
Dragon Class
FlyMovement Class
Play method in creature class
Move method in creature class, Movement is default movement where the implementation is
The FlyMovement is not being triggered
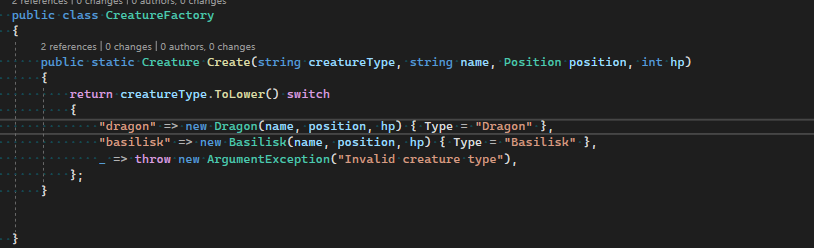
it hits dragon but it doesnt seem to take the method for flymovement and uses the defaultmovement instaed
wat
maybe this would be long to explain since it has many connected parts
little bit
I dunno, if you wanna read me in, what's the simplest you can condense the problem?
I set up a strategy pattern for the movement of a creature, each creature has a "certain movement"
okay
like a pre-defined sequence of movements
this is how the move method looks like
in the deafult movement ^
theres code but its too long to put
that's "Move
creature
within world
to position (x, y)
"?yes
k
the world has a position so does a creature
this is the flymovement
so if the creature flys
it will just move +2x and +2y and print the creature is flying
uhhh
was that confusing?
so, x/y is actually the creature's CURRENT position?
the position it's moving from?
and the creature moves 2 units diagonally on each tick?
x/y is a random movement from 0-5, and it gets added to the current creatures position
okay
so
so if i have creature with position 10,10 and x is 2 and y is 5, i should move to 12,15
what does
base.Move()
do^
well
then what the fuck is the hard-coded +2s?
So if u fly u basically have +2 movement
does that make sense
nah
what does
base.Move()
dookay, so x and y are the deltas
deltas meaning?
they're not x/y coordinates
they're a vector
a delta vector
an amount of change
yes
and movement is always positive?
it can be negative
then the +2s definitely don't make sense
it sounds more like what you want is to increase the RNG range
so, your RNG movement is +/-5 in any direction?
yes
the thing is it might not make sense, because moving - moves u down
with the +2s, you've turned it into +7/-3, with the bias on moving up-right
it sounds like what you REALLY want is for flying characters to have random movement of +/-7
i mean
now it sounds off
that's what I'm saying
😉
A creatures positon can never go beyond the worlds position, so if the world is 100,100, and the creatures position is 10,10 moving - just means moving down
now i get that the +2 might not make sense
so
but i could just add if statements
movement is not in any direction
well in my head i didnt think about right and left but technically it is every movement
which means that x and y can be negative
normally, anywhere form -5 to +5
it can be negative yes
exactly
if i do +2 to -5,5
thats -3,7
correct
but my point is
if the +2 is intended to make flying creatures move faster
that its pointless? 🤣
that's not what you wrote
well in this case what does it look like?
it seems like your intention was to give flying creatures +/-7 movement
you actually have given them +7/-3 movement
which is kinda nonsense
you're trying to bias your RNG generation at the wrong spot
if your goal is to generate a +/-7 value, you need to specify it that way to the RNG
you can't just adjust it after-the-fact to get the same result
ah so i inputted the wtong numbers
wrong
sort of
wherever your RNG call is that generates those numbers
you have to make the decision THERE
i wanna leave the -5,5 for rng
i can just change what the flymovement does
instead of
you'd want something like
or probably better yet, move this logic inside the Move method, so each create gets to define for itself how its movement works
i should move that logic to move yes, but this approach doesnt make sense to me
which one?
^
what doesn't make sense about it?
the random numbers
-creature.movementspeed
that's not random
that's a value defined for each creature
no no as in how would that work
for normal creatures, it'd be 5
for your flying creatures, you'd define it to be 7
where is this defined?
maybe some creatures could use 3
where you define it
wouldnt that just break the move
given that I wrote it as
creature.MovementSpeed
, my intention would be that you define it on the creature
how so?x and y properties?
what about them?
actually, better question
what x and y properties?
so instead of generating random numbers you want me to have const fields for x and y for each type of creature?
or maybe im understanding wrong
no
the way the method is set up i cant just call movement anyway
in creature class ^
I would want you to have a property
MovementSpeed
that is used to generate your random movement valuesso i wouldnt have to "hardcode" anything?
maybe?
maybe not
depends how you want to implement it
shouldnt this be done as a part of refactoring?
uhh, sure, you could call it that
after i finish all my code i can go into each and refactor to be better
but before i can refactor im having an issue thats not calling the flymovement method in the dragons ctor
its almost always using default
movement
okay
after i figure this issue ill apply the movement speed thank u
but i need some help figuring it out
the mvoement is not being reached do u know why?
depends
what's calling it
when calling move its called on a creature
and what calls that?
the method play
k
and
creature
is a Dragon
?yes
prove it
well in the json file i have it has type dragon then i deseralize it
okay, but that means nothing
it means you WANT it to be a
Dragon
you expect it to be a Dragon
IS it?its a creature
aha
but is it a
Dragon
?yes it should be
but IS it?
okay
that's great and all
no it says its a creature
but is
creature
a Dragon
how so?no
dragon is a creature
correct
but is
creature
a Dragon
?no it is not
how so?
creature is a creature
how so?
it's rhetorical, show me
because im calling creature instead of an instance of a dragon?
are you?
I'm not even sure what that means
well i am, but i thought in the method i showed im switching it to a dragon
again
it's rhetorical
I'm asking what
creature
is
show mewhat am i showing u exactly
the value of
creature
within the debugger
at the point where .Move()
is being calledwell first thing i notice
is the Type is null
when it should be dragon but it should of got that from the json file
is that why its not converting to a dragon instance
well, that just suggests more that there's some issue with your deserialization logic
or with something in between
now, you don't have just one creature, do you?
I'm guessing this is inside a loop over many creatures?
yes it is
well, make sure you're looking at ALL creatures in that loop
to see if your expected dragon is in there
also, you might consider swapping out your JSON file for one with only one creature, at least until this is debugged
but yeah, if there's no dragon in there, then either your deserialization is messed up, or you're screwing something up post-deserialization
or, your JSON is malformed
which might fall under "deserialization is messed up"
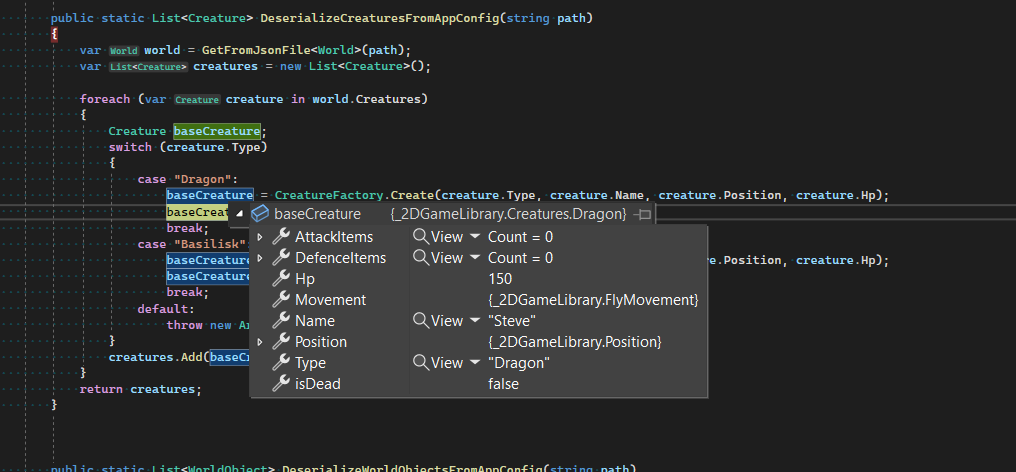
okay so this line here works fine it gets the type
and the movement
so, you were looking at the wrong creature
before that, it goes back to null
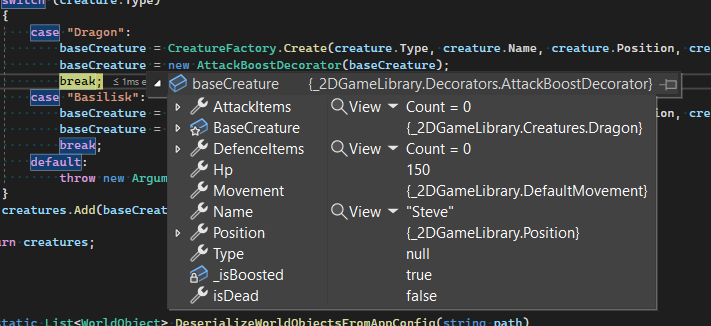
or that creature is getting lost
uhh
yeah
the Type
how
cause you told it to
you're replacing it
you just took the creature object that you verified was correct, and replaced it with a different object
you don't see where maybe the problem is?
aha
my mind totally went blank
😉
that makes sense now
🎉
your decorator appears to be malfunctioning
does this work?
define "work"
well before i was putting it in the object
will this still apply it to the basecreature?
apply what?
the decorator
it just adds +5 hitpoints to all attackitens
I mean
it does what you've coded it to do
the decorator pattern is perfectly valid
not my personal taste, but it's well-established
if you applying a decorator to this object makes it lose the properties you expect it to have
like
Type = "Dragon"
and Movement = FlyMovement
then yeah, I'd say it's not working
dig in and figure out whybefore that i should check if the movement is working
the fly that is
I thought we already established that it's not?
I thought that was the whole point of this conversation?
yh but now that the type is assigned to dragon it should
well not the type
but
you didn't change anything yet
did you?
we JUST established that the Type and Movement are wrong on account of the decorator
so you're going to go back and check again if they're still wrong, before doing anything to the decorator?
I just wanted to check if the creature was now pointing to Dragon
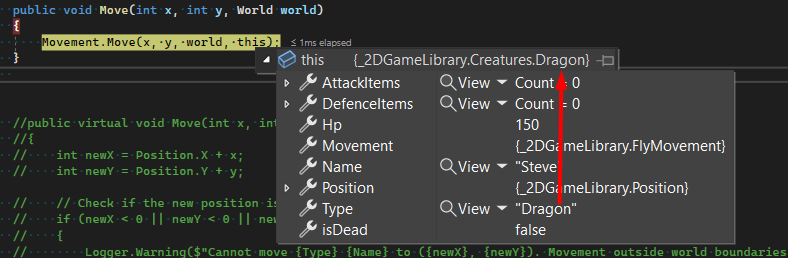
but i guess i should fix the decorator
its nearly 5am so excuse the brain deadness
I officially have no idea what's going on
Okay lets retrack
We wanted the flyMovement to trigger, but our creature was still of type creature and wasnt correctly being assigned to dragon
thats the most part
no
it was of type
AttackBoostDecorator
yes sorry ^
you said there was something wrong with the decorator class
cause that's what you showed me
you showed me that the
Dragon
object was getting deserialized correctly
and then immediately after, was getting wrapped in an AttackBoostDecorator
which you then showed was invalidYes
so
how would i call the decorator to the dragon now tho
what do you mean?
as in i want the attackdecorator class to be assigned to dragon class
im not sure of how i can apply the decorator now to it
i did it like this indtead
instead
there ive resolved my issues
Thank you
although now i need to fix flymovement
doesnt seem to add +2 to x and y
that's no longer a decorator
a decorator is supposed to wrap an existing object, invisibly, allowing it to affect the object's behavior
AttachBoostDecorator
presumably, wants to affect the attacking behavior of the creature it wraps around
meaning the other properties and behaviors of the object should be unaffected
meaning the decorator should be forwarding them from the wrapped object
which it seems to not have been doingi see
how would i apply it to my object then since im already applying something else to it
huh?
you apply it to your object the way you were already doing
that's the decorator pattern
you wrap an existing object
what other thing are you "applying" to it?
and how does that stop you from applying a decorator?
yes but then we had problems
right
because of your decorator
go fix your decorator
make it static?
no?
make it do what it's supposed to do
decorate the object it's been given
JakenVeina#1758
a decorator is supposed to wrap an existing object, invisibly, allowing it to affect the object's behavior
Quoted by
<@!137791696325836800> from #Design Pattern (click here)
React with ❌ to remove this embed.
JakenVeina#1758
meaning the other properties and behaviors of the object should be unaffected
Quoted by
<@!137791696325836800> from #Design Pattern (click here)
React with ❌ to remove this embed.
This first time ive done anything with design patterns so im not sure
so your saying with the correct implementation in the decorator, this code here should function as intended?
i was just doing things based off searching design patterns
yes, absolutely
both the
Dragon
creature and AttackBoostDecorator
are Creature
s
they should be indistinguishable, if you compare them, except that the AttackBoostDecorator
will exhibit a different behavior for attacking
whatever that means
when you call AttackBoostDecorator.Type
it should be returning the .Type
value of the Creature
that it's decorating
because that's not related to attacking
same for .Movement
the fact that it's not doing that means the decorator class itself is misbehavingah okay i think i see now
that's about the least efficient way to do it, but yes
would i have to override each property?
that would be a more-efficient way to do it
at least, memory-wise
so if i had a lot of properties i should always override or should i always almost override
whats best practice
I mean, I'm biased, cause my answer is gonna be "don't use decorators"
90% of the time
but IMO, overriding properties beats duplicating them
I.E. don't double your memory footprint
im just using for the sake of it being a requirement
but it doesnt sound too shabby
fair enough
also i do agree with overriding and saving memory
it's a decent exercise in how inheritance works
but i didnt know doing it like this doubles the memory
thats true
in a hand-wavy sort of way
your orignal object takes up enough memory to store 10 fields (let's say), plus a little bit for metadata about the object itself, like its type
if the decorator just duplicates all the fields, then, it also takes up enough memory to store 10 fields
I.E. creating a decorator and an object to be decorated allocates twice as much memory as just allocating the object
you can at least the extra memory needed to store the 10 fields
can't eliminate the overhead of the decorator object itself
cause
is equivalent to
I.E. it allocates a
string
field
and if that's on the base class, then both Dragon
and AttackBoostDecorator
have their own unique field
however
if you setup the base class as
and Dragon
as
and AttackBoostDecorator
as
then AttackBoostDecorator
doesn't allocate fields for each property
only Dragon
does
and AttackBoostDecorator
only allocates one field to store the whole decorated object
this does come with the assumption that the original instance being decorated ISN'T being used by anything else
but that is pretty much how the decorator pattern is accepted to workthats pretty nice
it seems like getting into the technicalities of memorys and meta date seems like a long/difficult thing
welcome to C#
ill prob pick up on them during internship or working
or maybe assing myself self study on that
maybe, maybe not
if you really want to take your knowledge to the next level, I recommend an actual technical book
at some point, you'll absorb about all you can through osmosis, and you'll need to take a more active role in developing your skills, in order to progress
thats true
If i may ask, since youve seen some of my code and so on
what would you say my level of C# is
Still beginner?
on the upper end
I see
the flymethod doesnt seem to be adding +2 to the x and y at all it just keeps it the same
but ill try figure it out tmrw
thank u for ur 

o7
this is strategy pattern and having hardcoded values is quite common in the redefined methods. Say you wanna create a Strategy for WeaponBehaviour because different weapons deal different amount of dmg. so we create an IWeaponBehaviour interface with DealDamage method or Attack or whatever. Then we create SwortBehaviour that attacks for 4, AxeBehaviour that attacks for 6, and DaggerBehaviour to attack for 3. It may seem weird to create extra classes for this but it makes more sense as the strategy gets more complex than that.
that wasn't really the question
I understood it as complaining about hardcoded code
no
sorry then
np
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.