❔ ✅ Splitting string without allocation
I'm writing a JsonConverter for Vector3 and I'd like to do this without allocating too much memory.
I've written this extension method to split the string as ReadOnlyMemory. I wanted to use ReadOnlySpan but I'm unable to use this type in IEnumerable<T>.
This is my implementation of Read for JsonConverter<Vector3>
I'd like to know if there are any ways to make my Split method better, and to somehow get my Read method to work.
Thanks for reading
11 Replies
Can't you just use the build-in
Split
?
I think the version of C# I'm using doesn't allow you to split a string with a span
since IEnumerable of span doesn't exist you can't do it with spans
I've heard ReadOnlyMemory is significantly slower than span, because it has to check the type of object stored
if you're satisfied with IEnumerator or just a for loop, make an iterator
make Current return a span
Also the use of Linq and IEnumerable makes me think you don't truly care about performance and allocations
GitHub
GitHub - cathei/LinqGen: Alloc-free and fast replacement for Linq, ...
Alloc-free and fast replacement for Linq, with code generation - GitHub - cathei/LinqGen: Alloc-free and fast replacement for Linq, with code generation
Ero#1111
REPL Result: Failure
Exception: CompilationErrorException
Compile: 617.981ms | Execution: 0.000ms | React with ❌ to remove this embed.
okay dude
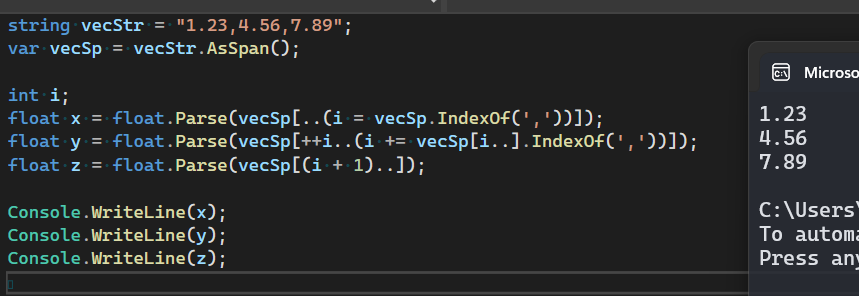
That's awesome. The whole point of this exercise is to learn more about how to use Span and Memory and associated types, I guess I need to keep practicing.
The next issue I have is supporting this functionality in a .NET Framework project. I've tried changing the LangVersion to 8.0 to support range and indexes but no luck. I also cannot parse float from a span. Since this is not related to the problem, I'll just end this here.
Thanks
this is faster without AI everywhere for me
?
what's confusing
??
why would you remove that
i'm sure they would have benefited greatly from that code
completely braindead
here you go @Samuel_. @Diddy wrote this one. this is a ref struct enumerator with 0 allocations
it works great and is probably really fast for what you want
you could benchmark this with and without AI
it's faster without for me
since the runtime probably knows best what to and what not to inline
here's the same slightly optimized
i made some more versions of the enumerables that take only a single
char
as the _split
parameter, since those are implemented differently for Span<T>.IndexOf
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.