ā Accessing the appsettings.json that resides in a ASP.NET Core 6 project from another project
Do I need to always pass that down as a constructor or is there any other way to do it. I need the database connection strings for my DAL project
43 Replies
your DAL project shouldn't access it, it should be fed in somehow
your APi project is the "core" of your application that assembles all the other parts
I understand the second part, but the first part is what I'm hung up on. Do I need to create a new class like AppSettingsContexts that gets populated in the API project that then goes into the DAL?
I'm trying to understand what the best practices are
why do you feel that you need the connection string in your DAL?
also, are you using something like EF or is this just
SqlConnection
or similar?I'm not using EF, so I guess that's part of why it's so hard for me to wrap my head around this. SqlConnection is what I want to use
Well, how EF works internally is that you configure a
DbContextOptions
object in your API
when you register EF
and then when the context needs to use the connstr, it gets it from the options objecthow does that make it's way to another project, via dependency injection? so on the controller level you need to pass that context down?
Yep, DI
not on controller level, as the DbContext is always injected
so it gets it from the service provider
The connection string gets passed as the context gets registered in the DI container
so if your project uses DI, you could just register a config object and inject that into your DAL where you need the connstr
alright cool.
so in my API program.cs file I have this configure service method, this is what it looks like currently
yupyup
and its your repositories that need the connection string, ye?
this is how I've laid out my project
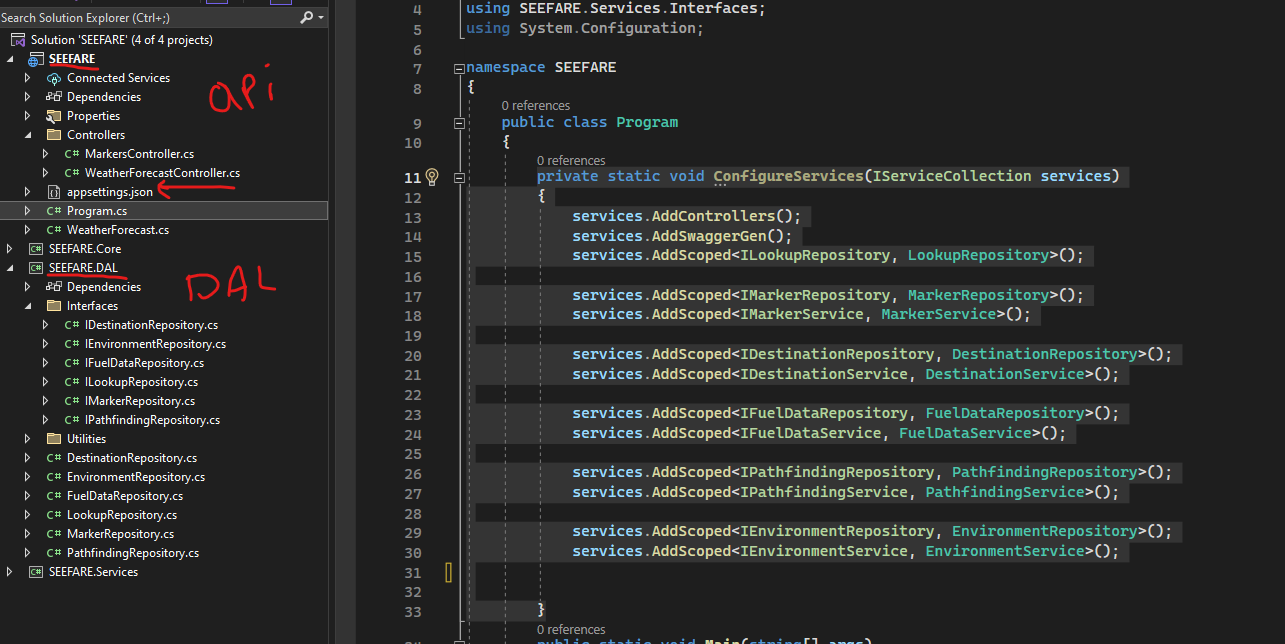
yupyup
this is one of my controllers
so in the API's program.cs config method, I would need to register a new class that I'll call something like AppContext, and populate it with the values from appsettings, then use Di on the controller level to the services, who would then pass that down to the DAL?
man this is rocking my brain right now
This is my currently flow:
API -> Services -> DAL
tada
if you wanna be fancy š
I'd have some
DatabaseContext
that wraps Dapper or whatever you're using (hopefully Dapper and not ADO), register that, and inject it into repositories/services
Or inject just the options, yeahIt's a fresh project and I'm relatively new to C# api's. I'm pretty sure I'd be using ADO (I've never used Dapper before) but I've got experience with ADO
My condolences
thanks for the help guys. last question @Pobiega , would I call ServiceCollectionExtensions.AddDataAccess from the ConfigureServices(IServiceCollection services) method?
I'm looking at dapper right now, it looks pretty cool
Yep
Say goodbye to manually mapping some data reader and other bullshit in a loop
Just a generic method, a class, bada bing bada boom, done
I see why it's cool
haha yeah
honestly it's so tedious
services.AddDataAccess("your string here");
extension methods my man
very cool stuff
you could even register your repos in there
its pretty common for "partial" applications like this to contain their own DI setup locally in an extension
and the API just calls all those extensionsYeah, that
.AddDataAccess()
could also be registering the repositories
And everything else from your DALif I wanted to pull the value of my connstring from my appsettings.json, how would I do that within the .AddDataAccess method?
that's where my confusion lies as well, how does it know between local/production environments
I want to host this API once I get it all flushed out so that's why I was having a hard time wrapping my head aroundit all
Asp puts it in the env object when it starts up
You can access this by injecting IEnvironment in ur classes
However, app settings should not be used for connection strings
Full stop
oh god
where else do I include the two connection strings then? one for local and one for the live server
your
ConfigureServices
method probably has access to the IConfiguration
, so get it from there?Far as env vs dev goes, you can add many config sources that make up a hierarchy. If conn string isn't found in appsettings, look for it in env vars. If it's not there, look for it in keyvault, etc
Ohh ya sry IConfiguration im dumb
Local dev is done using user-secrets production is done via keyvault or environment variables
App settings is not for secrets
Secrets should not be in appsettings
Safe storage of app secrets in development in ASP.NET Core
Learn how to store and retrieve sensitive information during the development of an ASP.NET Core app.
IConfiguration is what wires all these different providers up together
So ur app code doesn't care if it's in prod or dev mode
thanks for that. Reading the docs right now, but I currently don't see this directory/file. Do I need to create it?
%APPDATA%\Microsoft\UserSecrets<user_secrets_id>\secrets.json
specifically the UserSecrets directory
Use the secret manager
use
dotnet user-secrets
dotnet user-secrets
actually
š¤š
yeah I ran user-secrets init and I got a GUID back, which is good lol
alright sweet I see this here
C:\Users\jonat\AppData\Local\Microsoft
C:\Users\jonat\AppData\Roaming\Microsoft
I do not see a UserSecrets directory
alright I got it
lol
sorry
last and final question. when I deploy this, how will it know to look for a keyvault rather than my secrets
your secrets will not be there when its deployed, so it will fall back to environment variables or a keyvault
or however you configured it
love you guys, thanks a lot
went with the Di approach and it's gif related
don't forget to /close the thread