Invalidating queries with tRPC
Hi all! I am trying to do something similar to the screenshot:
I use useQuery() to fetch the initial data, and for each day, there are multiple start/end times with an id. Then, if any of them changes, I want to use mutate() and then somehow invalidate the query or optimistically update the data. I know how to do it by following React Query docs, but not 100% sure on how to execute this using tRPC.
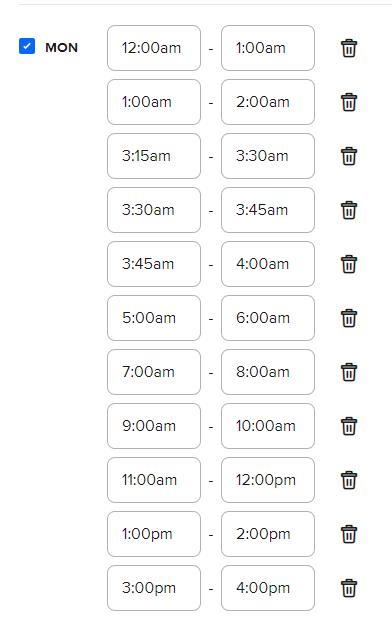
8 Replies
I'd imagine you'd do a
useQuery
to get the list, and then have a useMutation
for updating the times. When one of them is modified, you'd call mutate
with the new times and the id of the mutated time, and then use regular react query semanitcs to do the optimistic updateDo you mind if I DM real quick, I would like to show some code examples.
Thank you so much in advance
yeah go ahead
Here is solution for anyone wondering:
https://trpc.io/docs/useContext#helpers
useContext | tRPC
useContext is a hook that gives you access to helpers that let you manage the cached data of the queries you execute via @trpc/react-query. These helpers are actually thin wrappers around @tanstack/react-query's queryClient methods. If you want more in-depth information about options and usage patterns for useContext helpers than what we provide...
For example, if you want to do optimistic updates like such: https://tanstack.com/query/v4/docs/react/guides/optimistic-updates
Optimistic Updates | TanStack Query Docs
When you optimistically update your state before performing a mutation, there is a chance that the mutation will fail. In most of these failure cases, you can just trigger a refetch for your optimistic queries to revert them to their true server state. In some circumstances though, refetching may not work correctly and the mutation error could ...
Then you can do:
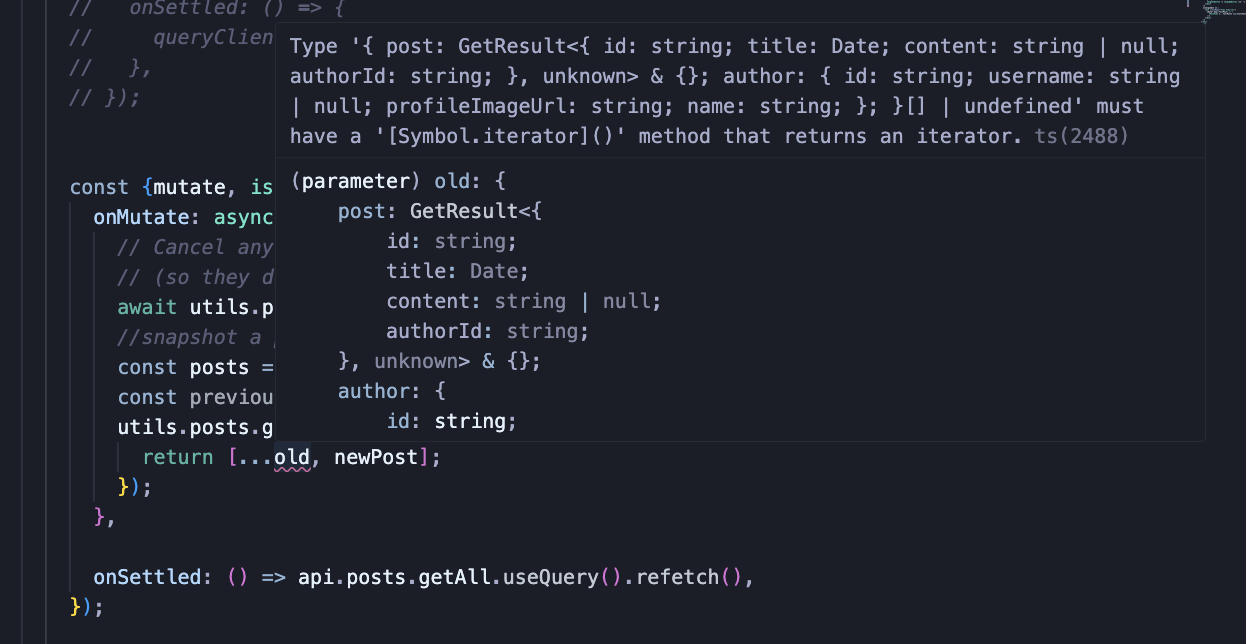
same error
here's my code
import { api } from "~/utils/api";
const utils = api.useContext();
heres how i got both utils and api. can you explain their differences please?