Ref
I am getting an interesting error when using a ref to select a canvas. Code works but just wondering if I am using ref the right way.
The error is
Variable 'canvasRef' is used before being assigned.ts(2454)
am I doing something wrong?
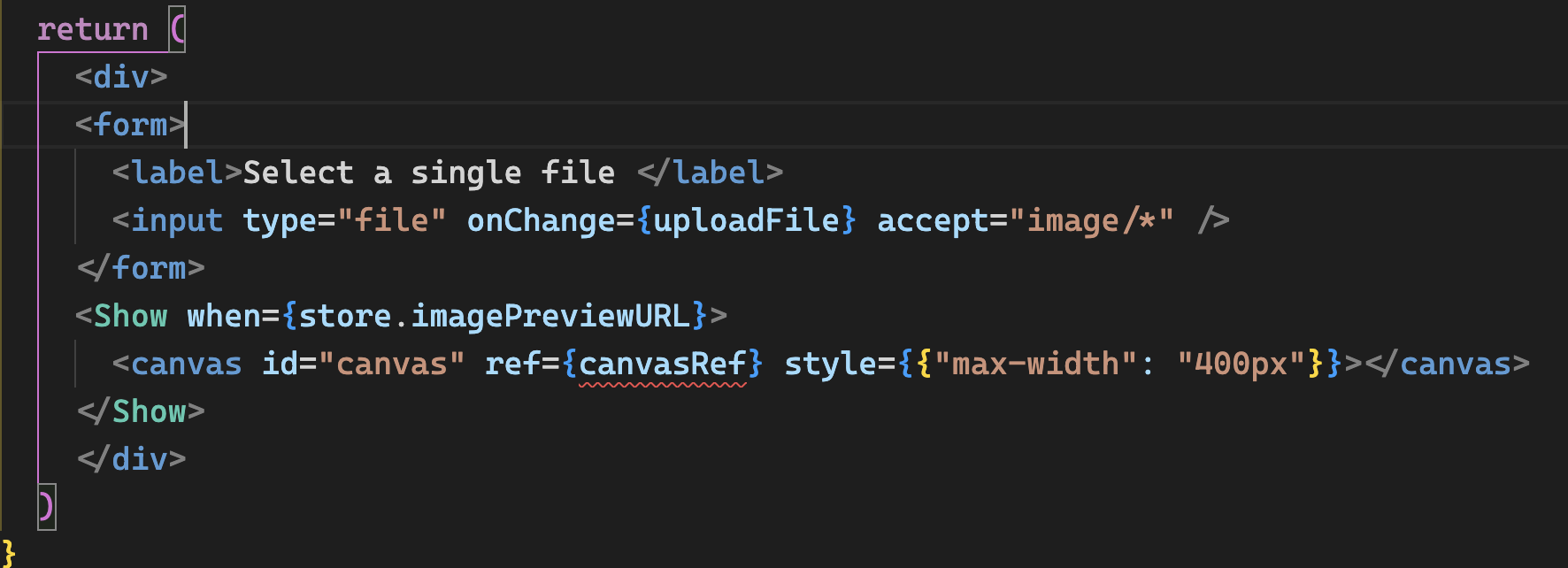
16 Replies
I am also open for other solutions if anyone got any good ideas 💜
Add "!" to make typescript happy - like
refs that are not signals imo are just a footgun
I am not exactly sure what you mean here, can you explain?
The kind of ref that you are using, which is just a simple variable that "works" thanks to the transform, is too problematic because it immediately causes type issues and it flat out doesn't work in some scenarios, the thing is it's not immediately obvious when it wouldn't work, so I see it as a footgun that should be deleted. If you use a signal as a ref that always works without problems.
how would one use a signal as a ref?
const [ref, setRef] = createSignal<HTMLWhatever | undefined> ();
<div ref={setRef} />
using the
setRef
function as the ref for the div? How does that work
wouldnt you want to use the ref
getterfundamentally a ref is simply a function that is called with an element, though sometimes it can look like something else. you pass it the setter so that it calls it with the element that you want, then you access it with the getter
thanks for answering btw - i am new to SolidJS - this week - i come from the world of React - lol xD
Like the other kind of ref if you think about it can't possibly work with a React-like transform, you are just passing "undefined" somewhere and somehow your variable changes?
Solid looks deceptively similar, but it's like a different world
a better world too
indeed, i am having to unlearn so much xD it's great
I see. I will try that see how it goes.
I am not sure but it its not working on my end
Can you make a playground?
yeah
thanks for following up btw
following this up it works thanks a lot. I forgot to add a () to the Accessor, my bad 😅