Collapse|Expand all nodes in tree
Hi, I'm making a simple web app in Blazor server. It's basically a tree structure with buttons to create, rename, delete, sort, etc.
Since a tree could be infinitely long, i render it using recursive component. The question is how can I expand/collapse whole tree at once?
My code is too long (even when simplified) so I'll post it in messages below
Thanks for help!
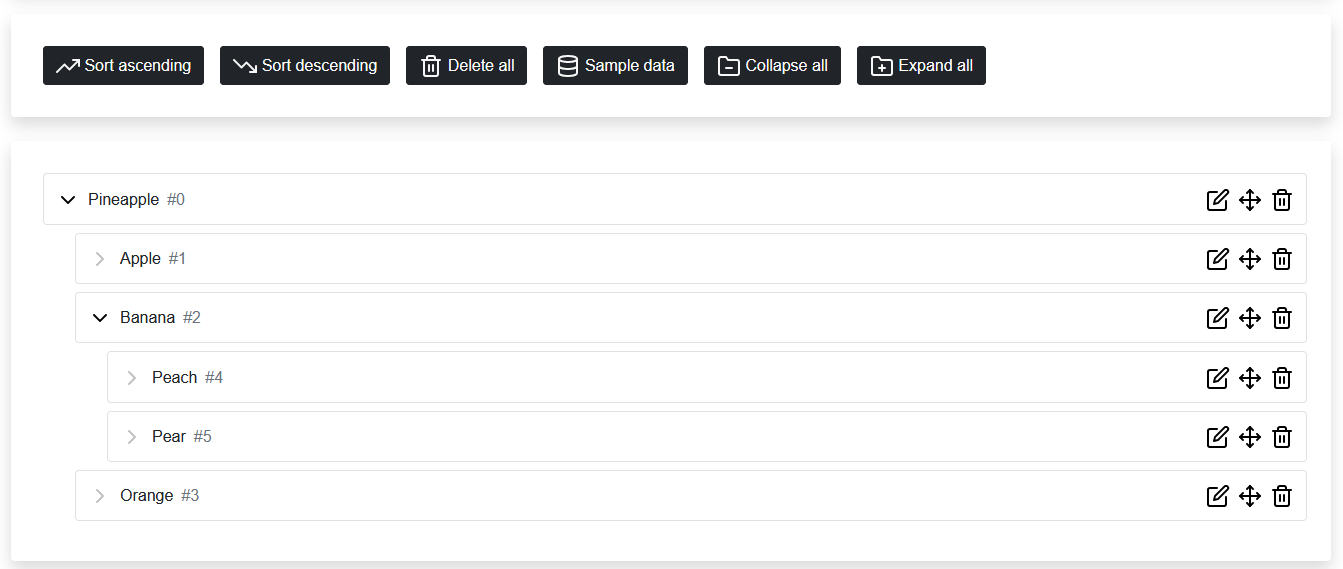
2 Replies
Index.razor
Tree.razor
and last one, TreeItem.razor
@foreach (var rootNode in NodeService.GetRootNodes())
{
<div class="...">
<ol>
<Tree Node="@rootNode"/>
</ol>
</div>
}
@foreach (var rootNode in NodeService.GetRootNodes())
{
<div class="...">
<ol>
<Tree Node="@rootNode"/>
</ol>
</div>
}
<li>
<TreeItem Text="@Node.Name"
Id="@((int)Node.Id)"
OnCollapse="@(() => _isCollapsed = true)"
OnExpand="@(() => _isCollapsed = false)"
IsCollapsed="@_isCollapsed"
CanBeExpanded="@NodeService.HasChildren(Node.Id)"/>
@if (GetSortedChildren() is var children && children.Any() && !_isCollapsed)
{
<ol>
@foreach (var child in children)
{
<!-- Here is recursion -->
<Tree Node="@child"/>
}
</ol>
}
</li>
@code {
[Parameter]
public Node Node { get; set; }
bool _isCollapsed = true;
List<Node> GetSortedChildren() { ... }
}
<li>
<TreeItem Text="@Node.Name"
Id="@((int)Node.Id)"
OnCollapse="@(() => _isCollapsed = true)"
OnExpand="@(() => _isCollapsed = false)"
IsCollapsed="@_isCollapsed"
CanBeExpanded="@NodeService.HasChildren(Node.Id)"/>
@if (GetSortedChildren() is var children && children.Any() && !_isCollapsed)
{
<ol>
@foreach (var child in children)
{
<!-- Here is recursion -->
<Tree Node="@child"/>
}
</ol>
}
</li>
@code {
[Parameter]
public Node Node { get; set; }
bool _isCollapsed = true;
List<Node> GetSortedChildren() { ... }
}
<div class="...">
<div class="...">
@if (CanBeExpanded)
{
@if (IsCollapsed)
{
<a @onclick="OnExpand">
<img src="img/expand.svg" alt="expand"/>
</a>
}
else
{
<a @onclick="OnCollapse">
<img src="img/collapse.svg" alt="collapse"/>
</a>
}
}
else
{
<img src="img/expand.svg" alt="expand" style="opacity: 0.25;"/>
}
</div>
<div class="p-1">
<span>@Text</span>
</div>
<div class="p-1 flex-grow-1">
<span class="text-muted">#@Id</span>
</div>
</div>
@code {
[Parameter]
public string? Text { get; set; }
[Parameter]
public int Id { get; set; }
[Parameter]
public bool IsCollapsed { get; set; }
[Parameter]
public bool CanBeExpanded { get; set; } = true;
[Parameter]
public EventCallback OnExpand { get; set; }
[Parameter]
public EventCallback OnCollapse { get; set; }
}
<div class="...">
<div class="...">
@if (CanBeExpanded)
{
@if (IsCollapsed)
{
<a @onclick="OnExpand">
<img src="img/expand.svg" alt="expand"/>
</a>
}
else
{
<a @onclick="OnCollapse">
<img src="img/collapse.svg" alt="collapse"/>
</a>
}
}
else
{
<img src="img/expand.svg" alt="expand" style="opacity: 0.25;"/>
}
</div>
<div class="p-1">
<span>@Text</span>
</div>
<div class="p-1 flex-grow-1">
<span class="text-muted">#@Id</span>
</div>
</div>
@code {
[Parameter]
public string? Text { get; set; }
[Parameter]
public int Id { get; set; }
[Parameter]
public bool IsCollapsed { get; set; }
[Parameter]
public bool CanBeExpanded { get; set; } = true;
[Parameter]
public EventCallback OnExpand { get; set; }
[Parameter]
public EventCallback OnCollapse { get; set; }
}
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View