sapphire-support
discordjs-support
old-sapphire-support
old-discordjs-support
old-application-commands-and-interactions
can i use preconditions in listeners
i have a few checks needed before running a group of different listeners, right now i have these checks in a helper function that i run at the start of each listener, but it would be nice to have them run automatically if i add a precondition
Solution:
no but preconditions are just glorified if checks so just extract the functionality to a function and call that function in both places
Listeners not registering?
I'm using sapphire with TS & TSUP, but registers aren't working...
listener code:
```ts
import { Events, Listener } from "@sapphire/framework";
import { ActivityType, type Client } from "discord.js";...
Solution:
could you try changing main to dist/main.js
error when trying to run bot
Solution:
edit it to
"typescript": "~5.4.5"
and run npm install/yarn/pnpm whatever...
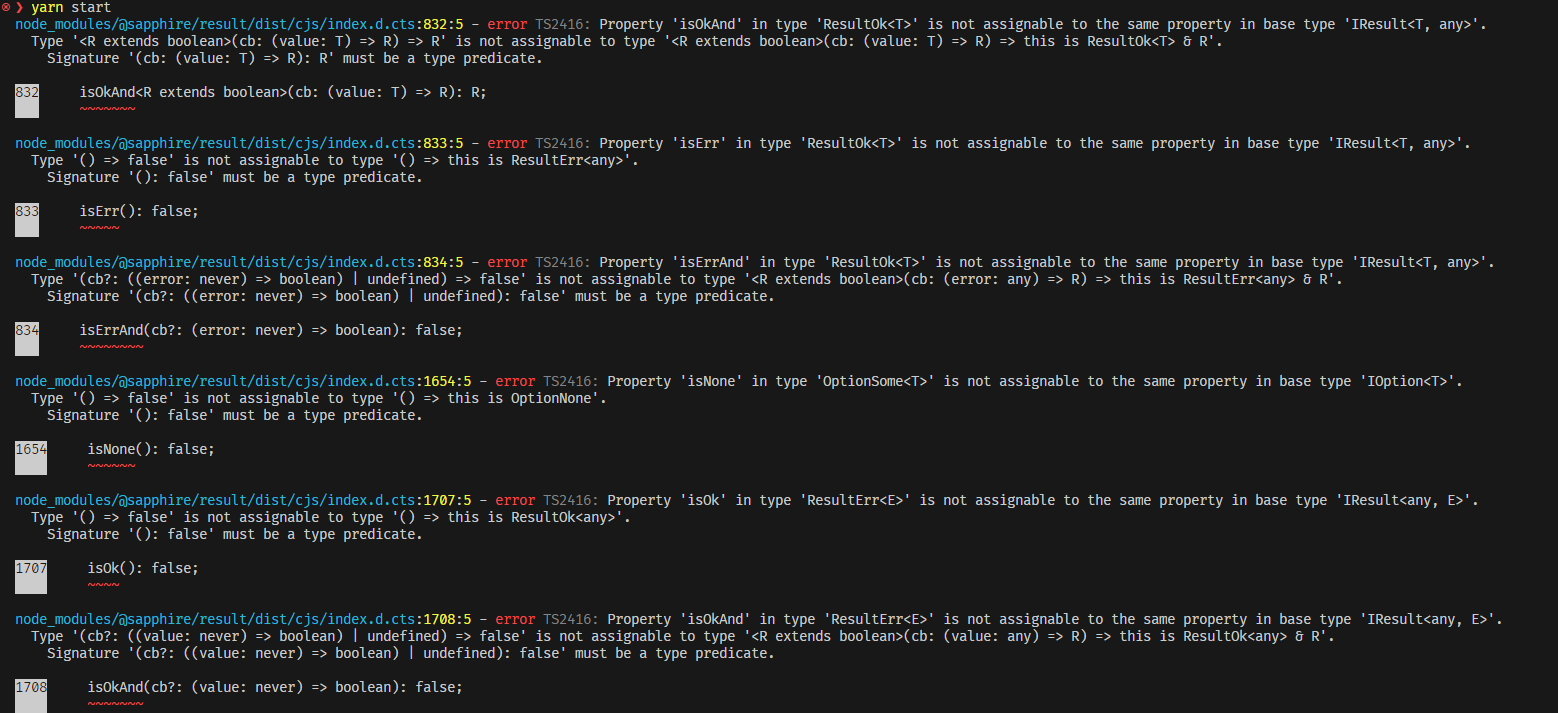
Assigning ID hints outside of builders
Is it possible to assign id hints outside of builders? Currently it's impossible to juggle id hints between the dev bot and the main bot and I was wondering if there's a way to do this outside of the builder, maybe before the client is initialized
Solution:
You can use environment variables specifyig individual id hints or a separate file like
config.ts
which will have this:
```ts
const prodHints = {
hint1: "id hint 1",
hint2: "id hint 2",...idhint & command id
Is sapphire framework supposed to assign command id to every command whether it is global or guild-only?
also, can we put idHints directly in the slash commands or not? if not, what do you suggest...
Solution:
first question is a yes because that's just how the Discord API works (as well as databases in general for that matter). Every entity has an id. A message (like this), a user, a guild (server), channel, thread, forum post, etc, they all have ids.
For your second question, I'm not exactly sure what you're asking. There is only 1 valid place to put the
idHints
and that's as part of the registerChatInputCommand
/ registerContextMenuCommand
function's second paramater (options)
```ts
public override registerApplicationCommands(registry: Command.Registry) {...Cooldown filter precondition
I wish to create a cooldown for a command but instead of filtering by user (
cooldownFilteredUsers
), I want to filter it with a precondition (ie user has a certain role in the guild). What is the best way to do this?
(I don't want to deny the command usage, just apply a cooldown for users who do not meet the precondition.)...Solution:
Yeah i realised afterwards that it didn't work as intended. and the precondition didn't run π¦
I wanted to extend the cooldown precondition method because I'm not sure how to code the cooldown myself. So i thought to just add to the super method...
API Authentification
Does sapphire have a way to implement authentification to the api routes so that for, example, the api can only be accessed thru the bot dashboard?
PM2 issues
Hello. I'm trying to use PM2 (installed globally) with NVM and I'm having trouble getting it to work. Any idea what I might be doing wrong? Is it because of TSX?
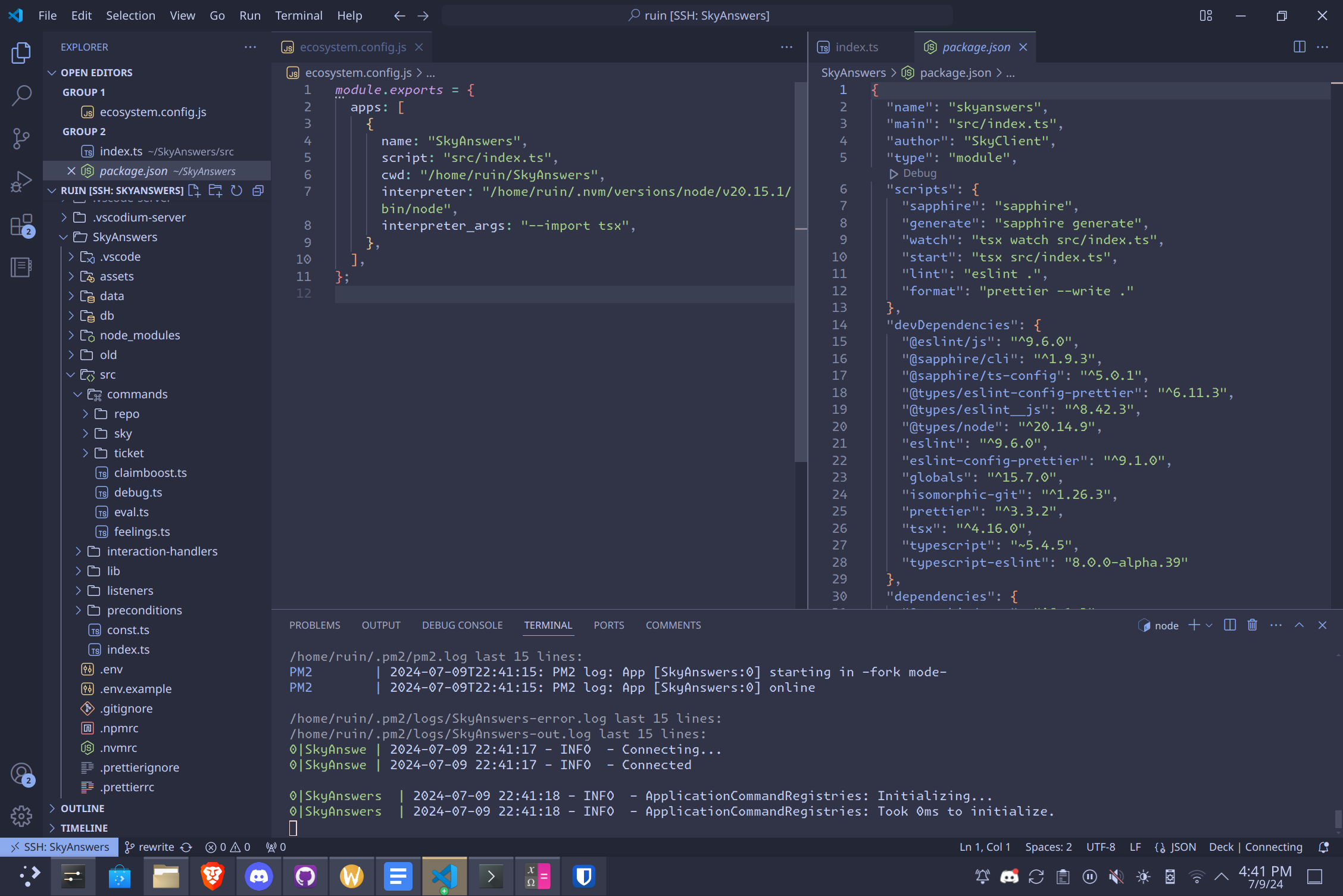
Help with manually setting path for stores
I am using Nx, a monorepo framework. The way it configures it's builds, makes it so that it is not possible to set the
main
entry in package.json
, because Nx also uses that entry to do some preprocessing/validation before running SapphireJS' entry main.js
I tried manually setting the path via container.stores.registerPath('./main.js')
where SapphireJS client logs in, but with no luck. Any advice/direction would be much appreciated.
registerPath()...Solution:
You can also set the root directory so it doesn't rely on the
main
entry in package.json
and is instead a path relative to the main files' directory (import.meta.url
in ESM or __dirname
in CJS).
However, if you want to load the pieces programmatically, you can use this guide: https://sapphirejs.dev/docs/Guide/additional-information/registering-virtual-pieces
If you go for the latter, you can use the Sapphire CLI, you can write sapphire gl
in your terminal and it'll generate all the _load.js
(or _load.ts
depending on your configuration) files ready for usage...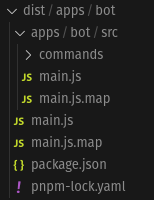
Slash commands failing to register
I deleted my dist folder because I wanted to 'refresh' the compiled code, and right after I noticed that only 2 of my commands are registering now, them being escalation-manager and shortcut-manager. I don't get what went wrong since it was working fine earlier but maybe someone can shine some light into this?
Solution:
I fixed it by just merging message commands with slash commands
Chat input command IDs
I have a function that needs to get the id of a chat input command to process some logic, and I was wondering how I could get the id? Obviously it's stored in container.stores.get('commands') but I don't know where to go from there.
Solution:...
import { ApplicationCommandRegistries } from '@sapphire/framework';
ApplicationCommandRegistries.acquire('commandNameHere').globalChatInputCommandIds
import { ApplicationCommandRegistries } from '@sapphire/framework';
ApplicationCommandRegistries.acquire('commandNameHere').globalChatInputCommandIds
Interaction create and custom commands
I have a system with shortcuts (basically a command that punishes a user with a defined reason and duration, e.g something like ,spam <user>) and I'm trying to port them to my sapphire bot, however, I'm unsure if adding an interaction create event for chat input commands would mess with sapphire's handlers. My guess is that It won't if it can't find a command that matches the name of a global command but I'd still like to make sure. Can anyone confirm this?
Solution:
Ah no you can't do that through sapphire command classes but you can implement the Events. UnknownChatInputCommand event instead of interactionCreate which will ensure that the interaction is at least a chat input command and not another type of interaction.
Errors and their listeners
In my commands when I want to throw an error I just use
throw '<message>'
and then send an embed with that error using the MessageCommandError event listener. This works fine however It also logs to my console, which is really annoying me. Is there a way to disable this?Preconditions Help
preconditions: ['AllStaff', 'BreakRoom']
preconditions: ['AllStaff', 'BreakRoom']
Solution:
Yes
PaginatedMessage - change select menu string names
Hi, I want to change the string names in the paginated message select menu.
Instead of "page 1" "page 2" I want a custom string based on the embed content.
I've looked through the documents and don't see any string to change under
PaginatedMessage.defaultActions
.
...got error idk whatβs wrong with my code
Here this what i meant
Solution:
As for solution to the original question:
Discordjs changed the intent variable case. Either use
GatewayIntentBit
enum or specify intents in UpperCamelCase...Terminal Crash
Every time I stop a sapphire project using watch my terminal crash
Solution:
Terminal version was the problem thank you !
Preconditions in InteractionHandler
Can I execute a precondition in an Interaction Handler?
Solution:
that's what the parse method is for. Also keep in mind that preconditions are just glorified if checks, you can extract the logic to a seperate function and execute that function in both.
Commands aren't being updated on Discord's end
Hello. I'm having trouble with commands not being updated on Discord's end. I added options to one of my commands, but the option isn't showing up in Discord. I've also tried removing the command completely from the
dist
folder, but that just results in a command that times out, instead of saying the command is outdated.Solution:
You can name it βsnarky-remarkβ
Slash command registered, but not displaying.
I've got a test slash command that is getting registered, but not displaying in Discord.
```js
import {Command, container} from "@sapphire/framework";
...
Solution:
Can you try configuring the default behaviour to BulkOverwrite? https://sapphirejs.dev/docs/Guide/commands/application-commands/application-command-registry/advanced/setting-global-behavior-when-not-identical